HDU-1035 Robot Motion
http://acm.hdu.edu.cn/showproblem.php?pid=1035
Robot Motion
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)
Total Submission(s): 5591 Accepted Submission(s): 2604
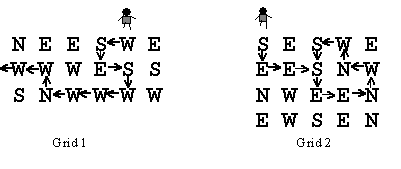
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
#include<stdio.h>
#include<string.h>
int n,m,s,num,num1;
char str[][];
int mark[][];
void dfs(int x,int y)
{
int x1,y1;
if(str[x][y]=='W')
{ x1=x;
y1=y-;
if(x1<||x1>n||y1<||y1>m||mark[x1][y1]==)
return ;
if(mark[x1][y1]==)
{
num1++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
else
{
num++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
}
if(str[x][y]=='S')
{ x1=x+;
y1=y;
if(x1<||x1>n||y1<||y1>m||mark[x1][y1]==)
return ;
if(mark[x1][y1]==)
{
num1++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
else
{
num++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
}
if(str[x][y]=='E')
{ x1=x;
y1=y+;
if(x1<||x1>n||y1<||y1>m||mark[x1][y1]==)
return ; if(mark[x1][y1]==)
{
num1++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
else
{ num++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
}
if(str[x][y]=='N')
{
x1=x-;
y1=y;
if(x1<||x1>n||y1<||y1>m||mark[x1][y1]==)
return ;
if(mark[x1][y1]==)
{
num1++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
else
{
num++;
mark[x1][y1]=;
dfs(x1,y1);
// mark[x1][y1]=0;
}
}
}
int main()
{
int i,j;
while(~scanf("%d%d%d",&n,&m,&s))
{
num=,num1=;
if(n==&&m==&&s==)
break;
memset(mark,,sizeof(mark));
getchar();
for(i=;i<=n;i++)
{
for(j=;j<=m;j++)
scanf("%c",&str[i][j]);
getchar();
}
dfs(,s);
if(num1==)
printf("%d step(s) to exit\n",num+);
else if(==num+-num1)
printf("0 step(s) before a loop of %d step(s)\n",num1);
else
printf("%d step(s) before a loop of %d step(s)\n",num+-num1,num1); }
return ;
}
HDU-1035 Robot Motion的更多相关文章
- HDOJ(HDU).1035 Robot Motion (DFS)
HDOJ(HDU).1035 Robot Motion [从零开始DFS(4)] 点我挑战题目 从零开始DFS HDOJ.1342 Lotto [从零开始DFS(0)] - DFS思想与框架/双重DF ...
- [ACM] hdu 1035 Robot Motion (模拟或DFS)
Robot Motion Problem Description A robot has been programmed to follow the instructions in its path. ...
- hdu 1035 Robot Motion(dfs)
虽然做出来了,还是很失望的!!! 加油!!!还是慢慢来吧!!! >>>>>>>>>>>>>>>>> ...
- HDU 1035 Robot Motion(dfs + 模拟)
嗯... 题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1035 这道题比较简单,但自己一直被卡,原因就是在读入mp这张字符图的时候用了scanf被卡. ...
- hdu 1035 Robot Motion(模拟)
Problem Description A robot has been programmed to follow the instructions in its path. Instructions ...
- 题解报告:hdu 1035 Robot Motion(简单搜索一遍)
Problem Description A robot has been programmed to follow the instructions in its path. Instructions ...
- (step 4.3.5)hdu 1035(Robot Motion——DFS)
题目大意:输入三个整数n,m,k,分别表示在接下来有一个n行m列的地图.一个机器人从第一行的第k列进入.问机器人经过多少步才能出来.如果出现了循环 则输出循环的步数 解题思路:DFS 代码如下(有详细 ...
- hdoj 1035 Robot Motion
Robot Motion Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Tota ...
- hdu1035 Robot Motion (DFS)
Robot Motion Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Tot ...
随机推荐
- vs2015中ctrl+shift+F进行“在文件中查找”,有时候无效?
搜狗拼音的默认简繁切换快捷键是ctrl+shift+F,改掉以后vs就可以收到这个按键消息了
- javascript类继承系列五(其他方式继承)
除了前面学习的三种继承外,还有另外三种:原型继承寄生继承,寄生组合继承都是以: function object(o) { function F() { } F.prototype = o; retur ...
- c语言学习之基础知识点介绍(七):循环结构
本节主要介绍循环结构 一.while循环 /* 语法: while(表达式){ //循环体; } 注意:循环变量.循环条件和循环控制语句三者缺一不可. 例如: */ ; //循环变量 ){ //循环条 ...
- iOS-assign、copy 、retain等关键字的含义
iOS中assign.copy .retain等关键字的含义 assign: 简单赋值,不更改索引计数 copy: 建立一个索引计数为1的对象,然后释放旧对象 retain:释放旧的对象,将旧对象的值 ...
- Object-C — KVO & oc通知
键值观察(KVO)是基于键值编码的一种技术. 利用键值观察可以注册成为一个对象的观察者,在该对象的某个属性变化时收到通知. 被观察对象需要编写符合KVC标准的存取方法,编写键值观察分为以下三步: (1 ...
- html-----003
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/ ...
- [学习笔记]设计模式之Decorator
写在前面 为方便读者,本文已添加至索引: 设计模式 学习笔记索引 Decorator(装饰)模式,可以动态地给一个对象添加一些额外的职能.为了更好地理解这个模式,我们将时间线拉回Bridge模式笔记的 ...
- Django练习项目之搭建博客
背景:自从今年回家过年后,来到公司给我转了试用,我的学习效率感觉不如从前,而且刚步入社会我总是想要怎么想明白想清楚一些事,这通常会花掉,消耗我大量的精力,因为我想把我的生活管理规划好了,而在it技术学 ...
- python细节
1.assert 断言语句,可判断一句话真假,若为假会抛出AssertionError. eg. assert 1==1 assert 1==2则AssertionError 2.单引号双引号 ...
- 谈memcache和memcached的区别
用了段时间的memcache和memcached总结下认识,看很多人在用cache的时候,刚刚都没有搞清楚memcache 和 memcached的区别,还有就是使用的时候基本都是 get/set ...