jQuery操纵DOM元素属性 attr()和removeAtrr()方法使用详解
jQuery操纵DOM元素属性 attr()和removeAtrr()方法使用详解
attr()方法 读操作
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("button").click(function () {
alert($("p").attr("title"));//获取属性
// this code can only get the first element's attribute.
});
});
</script>
</head>
<body>
<p title="title1">paragraph 1</p>
<p title="title2">paragraph 2</p>
<br/>
<button>get title</button>
</body>
</html>
<script type="text/javascript">
$(document).ready(function () {
$("button").click(function () {
//get attribute for every element in selection.
$("p").each(function () {
alert($(this).attr("title"));
});
});
});
</script>
即可分别获取每个元素的属性.
attr()方法 写操作
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$("#button1").click(function(){
$("p").attr("title","Hello World"); });
});
</script>
</head>
<body>
<input type="button" id="button1" value="button1"></input>
<p>This is a paragraph.</p>
<div>This is a div.</div>
<p>This is another paragraph.</p>
<div>This is another div.</div>
</body>
</html>
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("#button1").click(function () {
$("p").attr("title", "Hello World");
});
$("#button2").click(function () {
$("div").attr({"title": "some title for div", "hello": "World"});
});
});
</script>
</head>
<body>
<input type="button" id="button1" value="button1"></input>
<input type="button" id="button2" value="button2"></input>
<p>This is a paragraph.</p>
<div>This is a div.</div>
<p>This is another paragraph.</p>
<div>This is another div.</div>
</body>
</html>

<!DOCTYPE html>
<html>
<head>
<style>
div {
color: blue;
}
span {
color: red;
}
b {
font-weight: bolder;
}
</style>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("div")
.attr("id", function (index, oldAttr) {
if (oldAttr) {
return "div-id" + index + oldAttr;
} else {
return "div-id" + index;
} })
.each(function () {
$("span", this).html("(id = '<b>" + this.id + "</b>')");
});
}); </script>
</head>
<body> <div id="someId">Zero-th <span></span></div>
<div>First <span></span></div>
<div>Second <span></span></div> </body>
</html>
上面的例子,对应的页面结果如下:
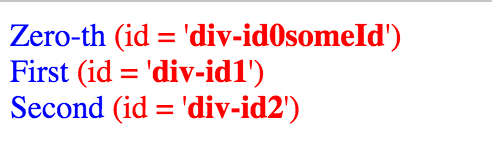
<script type="text/javascript">
$(document).ready(function () {
$("div").attr("id", function (index, oldAttr) {
return undefined;
}).each(function () {
$("span", this).html("(id = '<b>" + this.id + "</b>')");
});
});
</script>
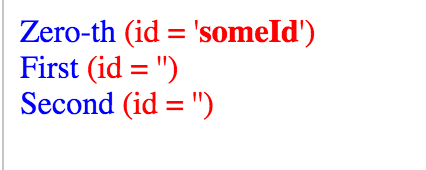
removeAttr()方法
<!DOCTYPE html>
<html>
<head>
<script type="text/javascript" src="/jquery/jquery.js"></script>
<script type="text/javascript">
$(document).ready(function () {
$("input[type=button]").click(function () {
$("div").removeAttr("title");
});
});
</script>
</head>
<body>
<input type="button" value="ClickMe"></input> <div title="hello">Zero</div>
</body>
</html>
$element.prop( "onclick", null );
console.log( "onclick property: ", $element[ 0 ].onclick );
参考资料
jQuery操纵DOM元素属性 attr()和removeAtrr()方法使用详解的更多相关文章
- jquery中dom元素的attr和prop方法的理解
一.背景 在编写使用高版本[ jQuery 1.6 开始新增了一个方法 prop()]的jquery插件进行编写js代码的时候,经常不知道dom元素的attr和prop方法到底有什么区别?各自有什么应 ...
- JQuery处理DOM元素-属性操作
JQuery处理DOM元素-属性操作 //操作元素的属性: $('*').each(function(n){ this.id = this.tagName + n; }) //获取属性值: $('') ...
- jQuery对html元素的取值与赋值实例详解
jQuery对html元素的取值与赋值实例详解 转载 2015-12-18 作者:欢欢 我要评论 这篇文章主要介绍了jQuery对html元素的取值与赋值,较为详细的分析了jQuery针对常 ...
- Uploadify 3.2 参数属性、事件、方法函数详解
一.属性 属性名称 默认值 说明 auto true 设置为true当选择文件后就直接上传了,为false需要点击上传按钮才上传 . buttonClass ” 按钮样式 buttonCursor ‘ ...
- (转)Uploadify 3.2 参数属性、事件、方法函数详解
转自http://blog.sina.com.cn/s/blog_5079086b0101fkmh.html Hallelujah博客 一.属性 属性名称 默认值 说明 auto true 设置为tr ...
- Uploadify 3.2 参数属性、事件、方法函数详解以及配置
一.属性 属性名称 默认值 说明 auto true 设置为true当选择文件后就直接上传了,为false需要点击上传按钮才上传 . buttonClass ” 按钮样式 buttonCursor ‘ ...
- web进阶之jQuery操作DOM元素&&MySQL记录操作&&PHP面向对象学习笔记
hi 保持学习数量和质量 1.jQuery操作DOM元素 ----使用attr()方法控制元素的属性 attr()方法的作用是设置或者返回元素的属性,其中attr(属性名)格式是获取元素属性名的值,a ...
- JQUERY选择和操作DOM元素(利用正则表达式的方法匹配字符串中的一部分)
JQUERY选择和操作DOM元素(利用正则表达式的方法匹配字符串中的一部分) 1.匹配属性的开头 $("[attributeName^='value']"); 2.匹配属性的结尾 ...
- JS操作DOM元素属性和方法
Dom元素基本操作方法API,先记录下,方便以后使用. W3C DOM和JavaScript很容易混淆不清.DOM是面向HTML和XML文档的API,为文档提供了结构化表示,并定义了如何通过脚本来访 ...
随机推荐
- Qt on Android:创建可伸缩界面
使用 Qt 来开发 Android 应用,也需要适配不同移动设备,适配多种多样的屏幕和分辨率.这次我们大概来讲一下如何使用 Qt 提供的机制来创建可伸缩的界面. DPI 必须要解释一下 DPI . D ...
- JavaScript Prototype
function Obj () { } Obj.a=0; Obj.fn=function(){ } console.log(Obj.a); console.log(typeof Obj.fn);//f ...
- Util应用程序框架公共操作类(四):验证公共操作类
为了能够验证领域实体,需要一个验证公共操作类来提供支持.由于我将使用企业库(Enterprise Library)的验证组件来完成这项任务,所以本文也将演示对第三方框架的封装要点. .Net提供了一个 ...
- Ubuntu杂记——Apache+PHP+MySQL的安装
昨天晚上,参考博客园的另一篇文章,在自己的Ubuntu上搭建了一个Apache+PHP+MySQL的服务器,在此谨记,以备不时之需. 一.安装Apache sudo apt-get install a ...
- YII 的源码分析(-)
做为源码分析的首秀,我就挑了yii(读作歪依依而不是歪爱爱):它的赞美之词我就不多说了,直接入正题.先准备材料,建议直从官网下载yii的源码包(1.1.15). 在demos里边有一个最简单的应用—h ...
- jsonp跨域+ashx(示例)
前言 做B/S项目的时候,我们一般使用jquery+ashx来实现异步的一些操作,比如后台获取一些数据到前台,但是如果ashx文件不在本项目下,引用的是别的域下的文件,这时候就访问不了.关于jsonp ...
- ubunt14.04 安装JDK
1.到 Sun 的官网下载 http://www.oracle.com/technetwork/java/javase/downloads/jdk8-downloads-2133151.html 选择 ...
- LeetCode - 72. Edit Distance
最小编辑距离,动态规划经典题. Given two words word1 and word2, find the minimum number of steps required to conver ...
- 读谭浩强C语言数据结构有感(1)
1.什么是数据结构? 数据结构,就是我们计算机内部的运算,编程语言的基础工作模式吧,个人总结的 = = !! 数据:说简单一点,就是计算机二进制机器码,然后通过一些复杂的操作,变为复杂的语言. 数据元 ...
- react入门(5)
对前面四篇内容进行简单的回顾: react入门(1):jsx,组件,css写法 react入门(2):事件,this.props.children,props,...other,map循环 react ...