POJ1941 The Sierpinski Fractal
Description
For this problem, you are to outline the Sierpinski Triangle up to a
certain recursion depth, using just ASCII characters. Since the drawing
resolution is thus fixed, you'll need to grow the picture
appropriately. Draw the smallest triangle (that is not divided any
further) with two slashes, to backslashes and two underscores like this:
- /\
/__\
To see how to draw larger triangles, take a look at the sample output.
Input
input contains several testcases. Each is specified by an integer n.
Input is terminated by n=0. Otherwise 1<=n<=10 indicates the
recursion depth.
Output
characters. Align your output to the left, that is, print the bottom
leftmost slash into the first column. The output must not contain any
trailing blanks. Print an empty line after each test case.
Sample Input
- 3
- 2
- 1
- 0
Sample Output
- /\
- /__\
- /\ /\
- /__\/__\
- /\ /\
- /__\ /__\
- /\ /\ /\ /\
- /__\/__\/__\/__\
- /\
- /__\
- /\ /\
- /__\/__\
- /\
- /__\
Hint
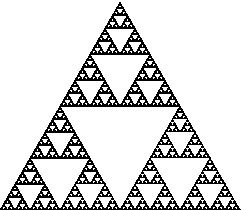
The Sierpinski-Triangle up to recursion depth 7
Source
- //It is made by jump~
- #include <iostream>
- #include <cstdlib>
- #include <cstring>
- #include <cstdio>
- #include <cmath>
- #include <algorithm>
- #include <ctime>
- #include <vector>
- #include <queue>
- #include <map>
- #include <set>
- using namespace std;
- typedef long long LL;
- char ch[][];
- int n;
- inline int getint()
- {
- int w=,q=; char c=getchar();
- while((c<'' || c>'') && c!='-') c=getchar(); if(c=='-') q=,c=getchar();
- while (c>='' && c<='') w=w*+c-'', c=getchar(); return q ? -w : w;
- }
- inline void solve(int x,int y,int now){
- if(now==) {
- ch[x][y]='/'; ch[x][y+]='_'; ch[x][y+]='_'; ch[x][y+]='\\';
- ch[x-][y+]='/'; ch[x-][y+]='\\';
- return ;
- }
- int du=(<<now);//拆分成三个小三角形
- solve(x,y,now-); solve(x,y+du,now-); solve(x-du/,y+du/,now-);
- }
- inline void work(){
- while() {
- n=getint(); if(n==) break;
- memset(ch,,sizeof(ch)); int mi=(<<n);
- solve(mi,,n); int last;//记录最后一个
- for(int i=;i<=mi;i++) {
- for(int j=;j<mi*;j++) if(ch[i][j]) last=j;
- for(int j=;j<last;j++) if(!ch[i][j]) ch[i][j]=' ';
- }
- for(int i=;i<=mi;i++) printf("%s\n",ch[i]);
- printf("\n");
- }
- }
- int main()
- {
- work();
- return ;
- }
POJ1941 The Sierpinski Fractal的更多相关文章
- POJ 1941 The Sierpinski Fractal
总时间限制: 1000ms 内存限制: 65536kB 描述 Consider a regular triangular area, divide it into four equal triangl ...
- poj 1941 The Sierpinski Fractal 递归
//poj 1941 //sep9 #include <iostream> using namespace std; const int maxW=2048; const int maxH ...
- POJ 1941 The Sierpinski Fractal ——模拟
只需要开一个数组,记录一下这个图形. 通过一番计算,发现最大的面积大约是2k*2k的 然后递归下去染三角形. 需要计算出左上角的坐标. 然后输出的时候需要记录一下每一行最远延伸的地方,防止行末空格过多 ...
- POJ 题目分类(转载)
Log 2016-3-21 网上找的POJ分类,来源已经不清楚了.百度能百度到一大把.贴一份在博客上,鞭策自己刷题,不能偷懒!! 初期: 一.基本算法: (1)枚举. (poj1753,poj2965 ...
- HDU——PKU题目分类
HDU 模拟题, 枚举1002 1004 1013 1015 1017 1020 1022 1029 1031 1033 1034 1035 1036 1037 1039 1042 1047 1048 ...
- (转)POJ题目分类
初期:一.基本算法: (1)枚举. (poj1753,poj2965) (2)贪心(poj1328,poj2109,poj2586) (3)递归和分治法. (4)递推. ...
- poj分类
初期: 一.基本算法: (1)枚举. (poj1753,poj2965) (2)贪心(poj1328,poj2109,poj2586) (3)递归和分治法. ( ...
- 转载 ACM训练计划
leetcode代码 利用堆栈:http://oj.leetcode.com/problems/evaluate-reverse-polish-notation/http://oj.leetcode. ...
- poj 题目分类(1)
poj 题目分类 按照ac的代码长度分类(主要参考最短代码和自己写的代码) 短代码:0.01K--0.50K:中短代码:0.51K--1.00K:中等代码量:1.01K--2.00K:长代码:2.01 ...
随机推荐
- javascript中的时间处理
var myDate = new Date(); myDate.getYear(); //获取当前年份(2位) myDate.getFullYear(); //获取完整的年份(4位,1970-???? ...
- gridpanel分组汇总
[ExtJS5学习笔记]第三十节 sencha extjs 5表格gridpanel分组汇总 2015-05-31 86 本文地址:http://blog.csdn.net/sushengmi ...
- C语言 百炼成钢13
//题目37:将一个数组逆序输出.用第一个与最后一个交换. #include<stdio.h> #include<stdlib.h> #include<math.h> ...
- 通过 SQL Server 视图访问另一个数据库服务器表的方法
今天项目经理跑过来对我大吼大叫说什么之前安排让我做一大堆接口为什么没做,我直接火了,之前明明没有这个事情…… 不过事情还要解决,好在两个项目都是用的sqlserver,可以通过跨数据库视图来快速解决问 ...
- 2015国产犯罪传记《暴力天使》HD720P.泰语中字
导演: 吴强编剧: 阮明玉主演: 张玉英 / 金理 / 至宝类型: 传记语言:泰语制片国家/地区: 中国大陆上映日期: 2016年3月25日片长: 92分钟又名: Huong Ga暴力天使的剧情简介 ...
- 流媒体技术之RTSP
流媒体技术之RTSP 标签: RTSP技术移动流媒体 2016-06-19 18:48 38人阅读 评论(0) 收藏 举报 分类: 流媒体相关技术 版权声明:本文为博主原创文章,未经博主允许不得转载 ...
- 第10章 系统级I/O
第10章 系统级I/O 10.1 Unix I/O 一个Unix文件就是一个m个字节的序列:B0,B1,…,BK,…,Bm-1 Unix I/O:一种将设备优雅地映射为文件的方式,允许Unix内核引出 ...
- HTML5 全屏特性
全屏功能是浏览器很早就支持的一项功能了,可以让你页面中的video, image ,div 等等子元素实现全屏浏览,从而带来更好的视觉体验,来看看怎么使用吧.先来看看有哪些API和事件支持. API ...
- 20145215《Java程序设计》第10周学习总结
20145215<Java程序设计>第十周学习总结 教材学习内容总结 网络编程 网络概述 网络编程就是在两个或两个以上的设备(例如计算机)之间传输数据.程序员所作的事情就是把数据发送到指定 ...
- IT男的”幸福”生活"系列暂停更新通知
首先谢谢博客园,这里给了我很多快乐.更给了大家一个学习的好地方. 在这几天更新过程中,看到了很多哥们的关注,在这里我谢谢你们,是你们给了我动力,是你们又一次给了我不一样的幸福. 在续5中我已回复了,博 ...