Spark: Best practice for retrieving big data from RDD to local machine
've got big RDD(1gb) in yarn cluster. On local machine, which use this cluster I have only 512 mb. I'd like to iterate over values in RDD on my local machine. I can't use collect(), because it would create too big array locally which more then my heap. I need some iterative way. There is method iterator(), but it requires some additional information, I can't provide. UDP: commited toLocalIterator method |
|||||||||
|
Update: TL;DR And the original answer might give a rough idea how it works: First of all, get the array of partition indexes:
Then create smaller rdds filtering out everything but a single partition. Collect the data from smaller rdds and iterate over values of a single partition:
I didn't try this code, but it should work. Please write a comment if it won't compile. Of cause, it will work only if the partitions are small enough. If they aren't, you can always increase the number of partitions with |
|||||||||||||
|
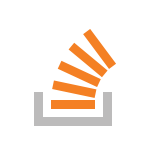
Did you find this question interesting? Try our newsletter
Sign up for our newsletter and get our top new questions delivered to your inbox (see an example).
Wildfire answer seems semantically correct, but I'm sure you should be able to be vastly more efficient by using the API of Spark. If you want to process each partition in turn, I don't see why you can't using
Or this
|
|||||||||||||
|
Here is the same approach as suggested by @Wildlife but written in pyspark. The nice thing about this approach - it lets user access records in RDD in order. I'm using this code to feed data from RDD into STDIN of the machine learning tool's process.
Produces output:
|
||||
Map/filter/reduce using Spark and download the results later? I think usual Hadoop approach will work. Api says that there are map - filter - saveAsFile commands:https://spark.incubator.apache.org/docs/0.8.1/scala-programming-guide.html#transformations |
|||||||||||||
|
For Spark 1.3.1 , the format is as follows
|
Spark: Best practice for retrieving big data from RDD to local machine的更多相关文章
- Why Apache Spark is a Crossover Hit for Data Scientists [FWD]
Spark is a compelling multi-purpose platform for use cases that span investigative, as well as opera ...
- [Spark] 02 - Practice Spark
开发环境 教学视频:Spark的环境搭建,需安装配置环境:Java, Hadoop 环境配置:玩转大数据分析!Spark2.X+Python 精华实战课程(免费)[其实只是环境搭建] 进入pyspar ...
- spark SQL (四)数据源 Data Source----Parquet 文件的读取与加载
spark SQL Parquet 文件的读取与加载 是由许多其他数据处理系统支持的柱状格式.Spark SQL支持阅读和编写自动保留原始数据模式的Parquet文件.在编写Parquet文件时,出于 ...
- Spark菜鸟学习营Day1 从Java到RDD编程
Spark菜鸟学习营Day1 从Java到RDD编程 菜鸟训练营主要的目标是帮助大家从零开始,初步掌握Spark程序的开发. Spark的编程模型是一步一步发展过来的,今天主要带大家走一下这段路,让我 ...
- The ‘Microsoft.ACE.OLEDB.12.0′ provider is not registered on the local machine. (System.Data)
When you try to import Excel 2007 or later “.xlsx” files into an SQL Server 2008 database you may ge ...
- Microsoft SQL Server 17导出xlsx文件时报错:The 'Microsoft.ACE.OLEDB.12.0' provider is not registered on the local machine. (System.Data)
导出数据时报错: 如果你是导出office 2007格式 TITLE: SQL Server Import and Export Wizard ---------------------------- ...
- Spark学习之键值对(pair RDD)操作(3)
Spark学习之键值对(pair RDD)操作(3) 1. 我们通常从一个RDD中提取某些字段(如代表事件时间.用户ID或者其他标识符的字段),并使用这些字段为pair RDD操作中的键. 2. 创建 ...
- <Spark><Programming><Loading and Saving Your Data>
Motivation Spark是基于Hadoop可用的生态系统构建的,因此Spark可以通过Hadoop MapReduce的InputFormat和OutputFormat接口存取数据. Spar ...
- spark SQL (五)数据源 Data Source----json hive jdbc等数据的的读取与加载
1,JSON数据集 Spark SQL可以自动推断JSON数据集的模式,并将其作为一个Dataset[Row].这个转换可以SparkSession.read.json()在一个Dataset[Str ...
随机推荐
- cookie 设置有效期 检测cookie
设置cookie 函数直接上代码: function setCookie(name, value, days) { //设置cookie var d = new Date(); d.setTime(d ...
- DB2建库简单例子
--重启数据库 FORCE APPLICATION ALL DB2STOP DB2START --创建数据库 TERRITORY US COLLATE USING SYSTEM ) CONNECT T ...
- HTTP协议详解之User Agent篇
•User Agent:用户代理 指浏览器他的信息包括硬件平台.系统软件.应用软件和用户个人偏好.用户代理不仅仅指浏览器,还包括搜索引擎. •为什么所有浏览器的User Agent都带有Mozilla ...
- LINUX下一款不错的网站压力测试工具webbench
LINUX下一款不错的网站压力测试工具webbench 分类: Linux 2014-07-03 09:10 220人阅读 评论(0) 收藏 举报 [html] view plaincopy wget ...
- More Effective C++:通过引用捕获异常
当你写一个catch子句时,必须确定让异常通过何种方式传递到catch子句里.你可以有三个选择:与你给函数传递参数一样,通过指针(by pointer),通过传值(by value)或通过引用(by ...
- esp8266烧录Html文件,实现内置网页控制设备!
代码地址如下:http://www.demodashi.com/demo/14321.html 一.前言: 这个月也快结束了,时间真快,我服务器知识自学依然在路途中,这几天听到热点网页配置esp826 ...
- 使用nginx生成缩略图
nginx中可以使用 --with-http_image_filter_module 这个模块,今天发现在github上发现国人开发的一款模块 模块同时支持 Nginx 和 tengine 本ngin ...
- nexus 介绍
http://juvenshun.iteye.com/blog/349534
- c:forEach标签
//varStat代表 遍历typeListDesc集合所用到的方法 <!-- stat当前迭代的第几项 --> <c:forEach var="type" it ...
- Numpy库应用实例——GPS定位
背景介绍 定位系统 GPS全球定位系统(Global Positioning System)以GPS系统为例介绍卫星定位的计算方法 GPS定位的基本原理 GPS定位的基本原理是根据高速运动卫星的 ...