wx.Frame
wx.Frame
A frame is a window whose size and position can (usually) be changed by the user.
It usually has thick borders and a title bar, and can optionally contain a menu bar, toolbar and status bar. A frame can contain any window that is not a frame or dialog.
A frame that has a status bar and toolbar, created via the CreateStatusBar
and CreateToolBar
functions, manages these windows and adjusts the value returned by GetClientSize
to reflect the remaining size available to application windows.
Default event processing
wx.Frame processes the following events:
wxEVT_SIZE:
if the frame has exactly one child window, not counting the status and toolbar, this child is resized to take the entire frame client area. If two or more windows are present, they should be laid out explicitly either by manually handlingwxEVT_SIZE
or using sizers;wxEVT_MENU_HIGHLIGHT:
the default implementation displays the help string associated with the selected item in the first pane of the status bar, if there is one.
Window Styles
This class supports the following styles:
wx.DEFAULT_FRAME_STYLE
: Defined aswx.MINIMIZE_BOX
|wx.MAXIMIZE_BOX
|wx.RESIZE_BORDER
|wx.SYSTEM_MENU
|wx.CAPTION
|wx.CLOSE_BOX
|wx.CLIP_CHILDREN
.wx.ICONIZE
: Display the frame iconized (minimized). Windows only.wx.CAPTION
: Puts a caption on the frame. Notice that this flag is required bywx.MINIMIZE_BOX
,wx.MAXIMIZE_BOX
andwx.CLOSE_BOX
on most systems as the corresponding buttons cannot be shown if the window has no title bar at all. I.e. ifwx.CAPTION
is not specified those styles would be simply ignored.wx.MINIMIZE
: Identical towx.ICONIZE
. Windows only.wx.MINIMIZE_BOX
: Displays a minimize box on the frame.wx.MAXIMIZE
: Displays the frame maximized. Windows and GTK+ only.wx.MAXIMIZE_BOX
: Displays a maximize box on the frame. Notice that under wxGTKwx.RESIZE_BORDER
must be used as well or this style is ignored.wx.CLOSE_BOX
: Displays a close box on the frame.wx.STAY_ON_TOP
: Stay on top of all other windows, see alsowx.FRAME_FLOAT_ON_PARENT
.wx.SYSTEM_MENU
: Displays a system menu containing the list of various windows commands in the window title bar. Unlikewx.MINIMIZE_BOX
,wx.MAXIMIZE_BOX
andwx.CLOSE_BOX
styles this style can be used withoutwx.CAPTION
, at least under Windows, and makes the system menu available without showing it on screen in this case. However it is recommended to only use it together withwx.CAPTION
for consistent behaviour under all platforms.wx.RESIZE_BORDER
: Displays a resizable border around the window.wx.FRAME_TOOL_WINDOW
: Causes a frame with a small title bar to be created; the frame does not appear in the taskbar under Windows or GTK+.wx.FRAME_NO_TASKBAR
: Creates an otherwise normal frame but it does not appear in the taskbar under Windows or GTK+ (note that it will minimize to the desktop window under Windows which may seem strange to the users and thus it might be better to use this style only withoutwx.MINIMIZE_BOX
style). In wxGTK, the flag is respected only if the window manager supports_NET_WM_STATE_SKIP_TASKBAR
hint.wx.FRAME_FLOAT_ON_PARENT
: The frame will always be on top of its parent (unlikewx.STAY_ON_TOP
). A frame created with this style must have a notNone
parent.wx.FRAME_SHAPED
: Windows with this style are allowed to have their shape changed with theSetShape
method.
The default frame style is for normal, resizable frames. To create a frame which cannot be resized by user, you may use the following combination of styles:
style = wx.DEFAULT_FRAME_STYLE & ~(wx.RESIZE_BORDER | wx.MAXIMIZE_BOX)
Window Extra Styles
See also the Window Styles. This class supports the following extra styles:
wx.FRAME_EX_CONTEXTHELP
: Under Windows, puts a query button on the caption. When pressed, Windows will go into a context-sensitive help mode and wxWidgets will send awxEVT_HELP
event if the user clicked on an application window. Note that this is an extended style and must be set by calling SetExtraStyle before Create is called (two-step construction). You cannot use this style together withwx.MAXIMIZE_BOX
orwx.MINIMIZE_BOX
, so you should usewx.DEFAULT_FRAME_STYLE
~ (wx``wx.MINIMIZE_BOX`` |wx.MAXIMIZE_BOX
) for the frames having this style (the dialogs don’t have a minimize or a maximize box by default)wx.FRAME_EX_METAL
: On Mac OS X, frames with this style will be shown with a metallic look. This is an extra style.
Events Emitted by this Class
Event macros for events emitted by this class:
- EVT_CLOSE: Process a
wxEVT_CLOSE_WINDOW
event when the frame is being closed by the user or programmatically (seewx.Window.Close
). The user may generate this event clicking the close button (typically the ‘X’ on the top-right of the title bar) if it’s present (see theCLOSE_BOX
style). See wx.CloseEvent. - EVT_ICONIZE: Process a
wxEVT_ICONIZE
event. See wx.IconizeEvent. - EVT_MENU_OPEN: A menu is about to be opened. See wx.MenuEvent.
- EVT_MENU_CLOSE: A menu has been just closed. See wx.MenuEvent.
- EVT_MENU_HIGHLIGHT: The menu item with the specified id has been highlighted: used to show help prompts in the status bar by wx.Frame. See wx.MenuEvent.
- EVT_MENU_HIGHLIGHT_ALL: A menu item has been highlighted, i.e. the currently selected menu item has changed. See wx.MenuEvent.
Note
An application should normally define an wx.CloseEvent handler for the frame to respond to system close events, for example so that related data and subwindows can be cleaned up.
See also
wx.MDIParentFrame, wx.MDIChildFrame, wx.MiniFrame, wx.Dialog
Class Hierarchy

Control Appearance
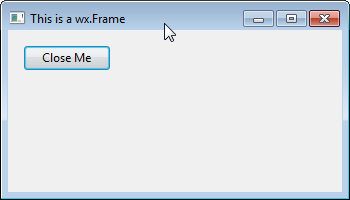
wxMSW
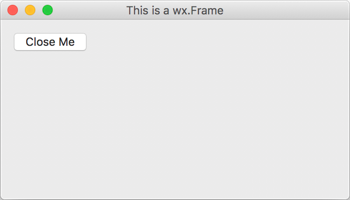
wxMAC

wxGTK
Known Subclasses
wx.aui.AuiMDIParentFrame, DocChildFrame , DocParentFrame ,wx.html.HtmlHelpFrame, wx.MDIChildFrame, wx.MDIParentFrame, wx.MiniFrame,wx.PreviewFrame, wx.adv.SplashScreen
Methods Summary
__init__ |
Default constructor. |
Centre |
Centres the frame on the display. |
Create |
Used in two-step frame construction. |
CreateStatusBar |
Creates a status bar at the bottom of the frame. |
CreateToolBar |
Creates a toolbar at the top or left of the frame. |
GetClientAreaOrigin |
Returns the origin of the frame client area (in client coordinates). |
GetMenuBar |
Returns a pointer to the menubar currently associated with the frame (if any). |
GetStatusBar |
Returns a pointer to the status bar currently associated with the frame (if any). |
GetStatusBarPane |
Returns the status bar pane used to display menu and toolbar help. |
GetToolBar |
Returns a pointer to the toolbar currently associated with the frame (if any). |
OnCreateStatusBar |
Virtual function called when a status bar is requested by CreateStatusBar . |
OnCreateToolBar |
Virtual function called when a toolbar is requested by CreateToolBar . |
PopStatusText |
|
ProcessCommand |
Simulate a menu command. |
PushStatusText |
|
SetMenuBar |
Tells the frame to show the given menu bar. |
SetStatusBar |
Associates a status bar with the frame. |
SetStatusBarPane |
Set the status bar pane used to display menu and toolbar help. |
SetStatusText |
Sets the status bar text and redraws the status bar. |
SetStatusWidths |
Sets the widths of the fields in the status bar. |
SetToolBar |
Associates a toolbar with the frame. |
Properties Summary
MenuBar |
See GetMenuBar and SetMenuBar |
StatusBar |
See GetStatusBar and SetStatusBar |
StatusBarPane |
See GetStatusBarPane and SetStatusBarPane |
ToolBar |
See GetToolBar and SetToolBar |
Class API
- class
wx.
Frame
(TopLevelWindow) -
Possible constructors:
Frame() Frame(parent, id=ID_ANY, title="", pos=DefaultPosition,
size=DefaultSize, style=DEFAULT_FRAME_STYLE, name=FrameNameStr)A frame is a window whose size and position can (usually) be changed by the user.
Methods
__init__
(self, *args, **kw)-
Overloaded Implementations:
__init__ (self)
Default constructor.
__init__ (self, parent, id=ID_ANY, title=””, pos=DefaultPosition, size=DefaultSize, style=DEFAULT_FRAME_STYLE, name=FrameNameStr)
Constructor, creating the window.
Parameters: - parent (wx.Window) – The window parent. This may be, and often is,
None
. If it is notNone
, the frame will be minimized when its parent is minimized and restored when it is restored (although it will still be possible to minimize and restore just this frame itself). - id (wx.WindowID) – The window identifier. It may take a value of -1 to indicate a default value.
- title (string) – The caption to be displayed on the frame’s title bar.
- pos (wx.Point) – The window position. The value DefaultPosition indicates a default position, chosen by either the windowing system or wxWidgets, depending on platform.
- size (wx.Size) – The window size. The value DefaultSize indicates a default size, chosen by either the windowing system or wxWidgets, depending on platform.
- style (long) – The window style. See wx.Frame class description.
- name (string) – The name of the window. This parameter is used to associate a name with the item, allowing the application user to set Motif resource values for individual windows.
Note
For Motif,
MWM
(the Motif Window Manager) should be running for any window styles to work (otherwise all styles take effect).See also
- parent (wx.Window) – The window parent. This may be, and often is,
Centre
(self, direction=BOTH)-
Centres the frame on the display.
Parameters: direction (int) – The parameter may be wx.HORIZONTAL
,wx.VERTICAL
orwx.BOTH
.
Create
(self, parent, id=ID_ANY, title="", pos=DefaultPosition, size=DefaultSize, style=DEFAULT_FRAME_STYLE, name=FrameNameStr)-
Used in two-step frame construction.
See wx.Frame for further details.
Parameters: Return type: bool
CreateStatusBar
(self, number=1, style=STB_DEFAULT_STYLE, id=0, name=StatusBarNameStr)-
Creates a status bar at the bottom of the frame.
Parameters: - number (int) – The number of fields to create. Specify a value greater than 1 to create a multi-field status bar.
- style (long) – The status bar style. See wx.StatusBar for a list of valid styles.
- id (wx.WindowID) – The status bar window identifier. If -1, an identifier will be chosen by wxWidgets.
- name (string) – The status bar window name.
Return type: Returns: A pointer to the status bar if it was created successfully,
None
otherwise.Note
The width of the status bar is the whole width of the frame (adjusted automatically when resizing), and the height and text size are chosen by the host windowing system.
See also
CreateToolBar
(self, style=TB_DEFAULT_STYLE, id=ID_ANY, name=ToolBarNameStr)-
Creates a toolbar at the top or left of the frame.
Parameters: - style (long) – The toolbar style. See wx.ToolBar for a list of valid styles.
- id (wx.WindowID) – The toolbar window identifier. If -1, an identifier will be chosen by wxWidgets.
- name (string) – The toolbar window name.
Return type: Returns: A pointer to the toolbar if it was created successfully,
None
otherwise.Note
By default, the toolbar is an instance of wx.ToolBar. To use a different class, override
OnCreateToolBar
. When a toolbar has been created with this function, or made known to the frame withwx.Frame.SetToolBar
, the frame will manage the toolbar position and adjust the return value fromwx.Window.GetClientSize
to reflect the available space for application windows. Under PocketPC
, you should always use this function for creating the toolbar to be managed by the frame, so that wxWidgets can use a combined menubar and toolbar. Where you manage your own toolbars, create a wx.ToolBar as usual.See also
GetClientAreaOrigin
(self)-
Returns the origin of the frame client area (in client coordinates).
It may be different from (0, 0) if the frame has a toolbar.
Return type: wx.Point
GetMenuBar
(self)-
Returns a pointer to the menubar currently associated with the frame (if any).
Return type: wx.MenuBar See also
GetStatusBar
(self)-
Returns a pointer to the status bar currently associated with the frame (if any).
Return type: wx.StatusBar See also
GetStatusBarPane
(self)-
Returns the status bar pane used to display menu and toolbar help.
Return type: int See also
GetToolBar
(self)-
Returns a pointer to the toolbar currently associated with the frame (if any).
Return type: wx.ToolBar See also
OnCreateStatusBar
(self, number, style, id, name)-
Virtual function called when a status bar is requested by
CreateStatusBar
.Parameters: - number (int) – The number of fields to create.
- style (long) – The window style. See wx.StatusBar for a list of valid styles.
- id (wx.WindowID) – The window identifier. If -1, an identifier will be chosen by wxWidgets.
- name (string) – The window name.
Return type: Returns: A status bar object.
Note
An application can override this function to return a different kind of status bar. The default implementation returns an instance of wx.StatusBar.
See also
OnCreateToolBar
(self, style, id, name)-
Virtual function called when a toolbar is requested by
CreateToolBar
.Parameters: - style (long) – The toolbar style. See wx.ToolBar for a list of valid styles.
- id (wx.WindowID) – The toolbar window identifier. If -1, an identifier will be chosen by wxWidgets.
- name (string) – The toolbar window name.
Return type: Returns: A toolbar object.
Note
An application can override this function to return a different kind of toolbar. The default implementation returns an instance of wx.ToolBar.
See also
PopStatusText
(self, number=0)-
Parameters: number (int) –
ProcessCommand
(self, id)-
Simulate a menu command.
Parameters: id (int) – The identifier for a menu item. Return type: bool
PushStatusText
(self, text, number=0)-
Parameters: - text (string) –
- number (int) –
SetMenuBar
(self, menuBar)-
Tells the frame to show the given menu bar.
Parameters: menuBar (wx.MenuBar) – The menu bar to associate with the frame. Note
If the frame is destroyed, the menu bar and its menus will be destroyed also, so do not delete the menu bar explicitly (except by resetting the frame’s menu bar to another frame or
None
). Under Windows, a size event is generated, so be sure to initialize data members properly before callingSetMenuBar
. Note that on some platforms, it is not possible to call this function twice for the same frame object.See also
SetStatusBar
(self, statusBar)-
Associates a status bar with the frame.
If statusBar is
None
, then the status bar, if present, is detached from the frame, but not deleted.Parameters: statusBar (wx.StatusBar) – See also
SetStatusBarPane
(self, n)-
Set the status bar pane used to display menu and toolbar help.
Using -1 disables help display.
Parameters: n (int) –
SetStatusText
(self, text, number=0)-
Sets the status bar text and redraws the status bar.
Parameters: - text (string) – The text for the status field.
- number (int) – The status field (starting from zero).
Note
Use an empty string to clear the status bar.
See also
SetStatusWidths
(self, widths)-
Sets the widths of the fields in the status bar.
Parameters: widths (list of integers) – Must contain an array of n integers, each of which is a status field width in pixels. A value of -1 indicates that the field is variable width; at least one field must be -1. You should delete this array after calling SetStatusWidths
.
SetToolBar
(self, toolBar)-
Associates a toolbar with the frame.
Parameters: toolBar (wx.ToolBar) –
Properties
MenuBar
-
See
GetMenuBar
andSetMenuBar
StatusBar
-
See
GetStatusBar
andSetStatusBar
StatusBarPane
-
See
GetStatusBarPane
andSetStatusBarPane
ToolBar
-
See
GetToolBar
andSetToolBar
wx.Frame的更多相关文章
- wx.ListCtrl简单使用例子
效果图: 示例代码: #! /usr/bin/env python #coding=utf-8 import wx import sys packages = [('jessica alba', 'p ...
- wxPython安装错误问题:No module named wx
今天心血来潮安装wxPython,本机win7,且已经安装Python,版本为2.7.3,然后IDE使用的PyCharm,然后wxPython下载的版本为:wxPython2.8-win32-unic ...
- wxPython 基本框架与运行原理 -- App 与 Frame
<wxPython in Action> chapter 1.2 笔记 wxPython 是 wxWidgets 的 Python 实现,“w” for Microsoft Windows ...
- PIL Image 转成 wx.Image、wx.Bitmap
import wx from PIL import Image def ConvertToWxImage(): pilImage = Image.open('1.png') image = wx.Em ...
- wx
wx The classes in this module are the most commonly used classes for wxPython, which is why they hav ...
- wx.Dialog
wx.Dialog A dialog box is a window with a title bar and sometimes a system menu, which can be moved ...
- wx.ToolBar
wx.ToolBar A toolbar is a bar of buttons and/or other controls usually placed below the menu bar in ...
- wx模块小实例
功能介绍: 查询数据库表数据,提取数据并显示 main.py(执行文件) #coding:gbk __author__ = 'Hito' import querySmscode import wx c ...
- Python中wx.FlexGridSizer
FlexGridSizer是GridSizer的一个更灵活的版本.它与标准的GridSizer几乎相同,除了下面3点例外: 1.每行和每列可以有各自的尺寸.2.默认情况下,当尺寸调整时,它行和列整体改 ...
随机推荐
- hightchart or hightstock 格式Y数据
hightchart or hightstock 格式Y数据,鼠标放在上面显示两位小数 方法一: tooltip: { shared: true, crosshairs: true , formatt ...
- MinGW 使用 msvcr90.dll
MinGW 编译出来的程序总是使用 VC6 的 msvcrt.dll ,VC8,9,10有很多新的API(仅限于c runtime),想使用怎么办? 比如:boost 对 MinGW 最低要求就是 m ...
- 【男性身材计算】胸围=身高*0.48(如:身高175cm的标准胸围=175cm*0.61=84cm);腰围=身高*0.47(如:身高175c… - 李峥 - 价值中国网
[男性身材计算]胸围=身高*0.48(如:身高175cm的标准胸围=175cm*0.61=84cm):腰围=身高*0.47(如:身高175c- - 李峥 - 价值中国网 李峥:[男性身材计算]胸围=身 ...
- linux底半部机制在视频采集驱动中的应用
最近在做一个arm+linux平台的视频驱动.本来这个驱动应该是做板子的第三方提供的,结果对方软件实力很差,自己做不了这个东西,外包给了一个暑期兼职的在读博士.学生嘛,只做过实验,没做过产品,给出的东 ...
- Oracle表空间常用操作
--创建表空间 create tablespace test datafile 'E:\test2_data.dbf' SIZE 20M autoextend on next 5M maxsize 5 ...
- Longge的问题(欧拉,思维)
Longge的问题 Submit Status Practice HYSBZ 2705 Description Longge的数学成绩非常好,并且他非常乐于挑战高难度的数学问题.现在问题来了:给定一 ...
- Introduction to neural network —— 该“神经网络” 下拉“祭坛”
Introduction to neural network 不能自欺欺人. 实干兴邦,空谈误国. -------------------------------------------------- ...
- WebConfig 配置文件详解
<?xml version="1.0"?><!--注意: 除了手动编辑此文件以外,您还可以使用 Web 管理工具来配置应用程序的设置.可以使用 Visual St ...
- 从头开始-03.C语言中数据类型
基本数据类型 整形: Int 4字节 %d / %i Short 2字节 %hd Long 8字节 %ld Longlong 8字节 %lld Unsigned 4字节 % 浮点型 单精度 Float ...
- SQL 时间格式化函数
1 取值后格式化 {0:d}小型:如2005-5-6 {0:D}大型:如2005年5月6日 {0:f}完整型 2 当前时间获取 DateTime.Now.ToShortDateString 3 取值中 ...