HDU4272LianLianKan(dfs)
Now we have a number stack, and we should link and pop the same element pairs from top to bottom. Each time, you can just link the top element with one same-value element. After pop them from stack, all left elements will fall down. Although the game seems to be interesting, it's really naive indeed.
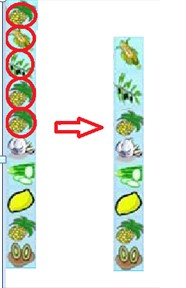
To prove I am a wisdom among my friend, I add an additional rule to the game: for each top element, it can just link with the same-value element whose distance is less than 6 with it.
Before the game, I want to check whether I have a solution to pop all elements in the stack.
The first line is an integer N indicating the number of elements in the stack initially. (1 <= N <= 1000)
The next line contains N integer ai indicating the elements from bottom to top. (0 <= ai <= 2,000,000,000)
注意给的顺序是从底部到顶部。
开始想直接模拟,不可行,因为在下面5个里可能有一个或多个相同的元素,每个选择都可能对后面造成影响,因此要dfs。
其中还巧妙的用了map,当然改用set来存然后看里面到底有没有奇数个的元素也是可以的,这点的优化很重要。
#include<stdio.h>
#include<iostream>
#include<string>
#include<cstring>
#include<algorithm>
#include<queue>
#include<set>
#include<map> using namespace std; int vis[],a[]; int dfs(int n)
{
int i=,j=n-;
while(n>=&&vis[n]) --n;
if(n==-) return ;
if(n==&&!vis[]) return ;
while(i<=)
{
if(j<) return ;
if(vis[j]) --j;
if(a[n]==a[j])
{
vis[j]=;
if(dfs(n-)) return ;
vis[j]=;
}
++i;
--j;
}
return ;
} int main()
{
int k,m,q,t,p,n;
int T;
map<int,int> mp;
map<int,int>::iterator it; while(cin>>n)
{
t=;
for(int i=;i<n;++i)
{
scanf("%d",&a[i]);
vis[i]=;
mp[a[i]]++;
} for(it=mp.begin();it!=mp.end();++it)
{
if((*it).second%)
{
t=;
break;
}
} if(t)
{
cout<<<<endl;
}else
{
cout<<dfs(n-)<<endl;
} }
return ;
}
HDU4272LianLianKan(dfs)的更多相关文章
- LeetCode Subsets II (DFS)
题意: 给一个集合,有n个可能相同的元素,求出所有的子集(包括空集,但是不能重复). 思路: 看这个就差不多了.LEETCODE SUBSETS (DFS) class Solution { publ ...
- LeetCode Subsets (DFS)
题意: 给一个集合,有n个互不相同的元素,求出所有的子集(包括空集,但是不能重复). 思路: DFS方法:由于集合中的元素是不可能出现相同的,所以不用解决相同的元素而导致重复统计. class Sol ...
- HDU 2553 N皇后问题(dfs)
N皇后问题 Time Limit:1000MS Memory Limit:32768KB 64bit IO Format:%I64d & %I64u Description 在 ...
- 深搜(DFS)广搜(BFS)详解
图的深搜与广搜 一.介绍: p { margin-bottom: 0.25cm; direction: ltr; line-height: 120%; text-align: justify; orp ...
- 【算法导论】图的深度优先搜索遍历(DFS)
关于图的存储在上一篇文章中已经讲述,在这里不在赘述.下面我们介绍图的深度优先搜索遍历(DFS). 深度优先搜索遍历实在访问了顶点vi后,访问vi的一个邻接点vj:访问vj之后,又访问vj的一个邻接点, ...
- 深度优先搜索(DFS)与广度优先搜索(BFS)的Java实现
1.基础部分 在图中实现最基本的操作之一就是搜索从一个指定顶点可以到达哪些顶点,比如从武汉出发的高铁可以到达哪些城市,一些城市可以直达,一些城市不能直达.现在有一份全国高铁模拟图,要从某个城市(顶点) ...
- 深度优先搜索(DFS)和广度优先搜索(BFS)
深度优先搜索(DFS) 广度优先搜索(BFS) 1.介绍 广度优先搜索(BFS)是图的另一种遍历方式,与DFS相对,是以广度优先进行搜索.简言之就是先访问图的顶点,然后广度优先访问其邻接点,然后再依次 ...
- 图的 储存 深度优先(DFS)广度优先(BFS)遍历
图遍历的概念: 从图中某顶点出发访遍图中每个顶点,且每个顶点仅访问一次,此过程称为图的遍历(Traversing Graph).图的遍历算法是求解图的连通性问题.拓扑排序和求关键路径等算法的基础.图的 ...
- 搜索——深度优先搜索(DFS)
设想我们现在身处一个巨大的迷宫中,我们只能自己想办法走出去,下面是一种看上去很盲目但实际上会很有效的方法. 以当前所在位置为起点,沿着一条路向前走,当碰到岔道口时,选择其中一个岔路前进.如果选择的这个 ...
随机推荐
- MyEclipse使用总结——使用MyEclipse打包带源码的jar包
平时开发中,我们喜欢将一些类打包成jar包,然后在别的项目中继续使用,不过由于看不到jar包里面的类的源码了,所以也就无法调试,要想调试,那么就只能通过关联源代码的形式,这样或多或少也有一些不方便,今 ...
- AVR 定点数运算程序设计及数制转换
AVR 单片机有加法和减法指令,可以直接调用相关指令来达到目的. 这里列出了16位加法.16位带立即数加法. 16位减法.16位带立即数减法. 16位比较.16位带立即数比较程序和16位取补程序. a ...
- CSS复合样式
关于font OK,我们先从font来谈起. 如下一段代码: div{ font-size: 14px; font-family: '\5FAE\8F6F\96C5\9ED1'; font-weigh ...
- Ember学习(8):REOPENING CLASSES AND INSTANCES
英文原址:http://emberjs.com/guides/object-model/reopening-classes-and-instances/ 你不须要一次就完毕类所有内容的定义,通过reo ...
- 【转】深入浅出REST
转自:http://www.infoq.com/cn/articles/rest-introduction 不知你是否意识到,围绕着什么才是实现异构的应用到应用通信的“正确”方式,一场争论正进行的如火 ...
- 学渣告诉你,到底神马是傅里叶级数!转自 新浪@工程师style
- Codeforces Round #310 (Div. 1) B. Case of Fugitive set
B. Case of Fugitive Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://codeforces.com/contest/555/p ...
- 了解PHP中Stream(流)的概念与用法(转)
Stream是PHP开发里最容易被忽视的函数系列(SPL系列,Stream系列,pack函数,封装协议)之一,但其是个很有用也很重要的函数.Stream可以翻译为“流”,在Java里,流是一个很重要的 ...
- Device Pixel Ratio & Media Queries
一些重要的名词解释: CSS pixels(CSS 像素):详见http://www.w3.org/TR/css3-values/#reference-pixe CSS声明的像素值,可随着放大缩小而放 ...
- Spring JdbcTemplate的queryForList(String sql , Class<T> elementType)易错使用--转载
原文地址: http://blog.csdn.net/will_awoke/article/details/12617383 一直用ORM,今天用JdbcTemplate再次抑郁了一次. 首先看下这个 ...