SpringBoot+idea搭建微服务简化流程
个人微信公众号:程序猿的月光宝盒
[toc]
# 1.新建普通maven工程
2.在父级pom中按需修改
3.删除父级src
目录
4.创建公共模块common,里面只有service接口和实体类
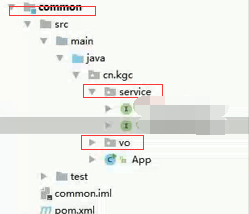
5.构建微服务模块,provider
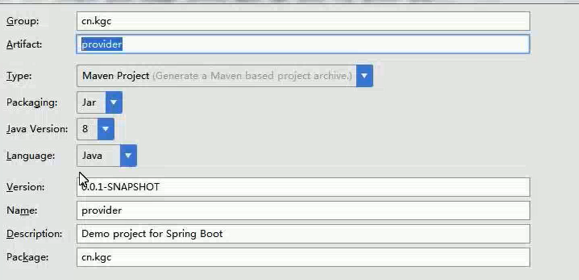
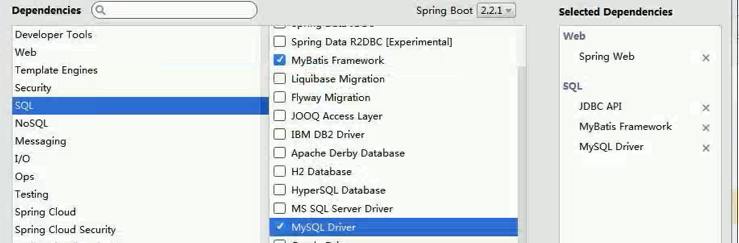
6.引用Zookeeper和Dubbo的依赖
在这个provider中修改pom文件坐标
<dependencies>
<!--引入公共模块项目-->
<dependency>
<groupId>cn.kgc</groupId>
<artifactId>common</artifactId>
<version>1.0-SNAPSHOT</version>
</dependency>
<!--这是微服dubbo的核心服务包-->
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>dubbo</artifactId>
<version>2.5.3</version>
<exclusions>
<exclusion>
<artifactId>spring</artifactId>
<groupId>org.springframework</groupId>
</exclusion>
</exclusions>
</dependency>
<!--这是zookeeper的服务操作包 但主要这里的版本最好和你的zookeeper版本一致-->
<dependency>
<groupId>org.apache.zookeeper</groupId>
<artifactId>zookeeper</artifactId>
<version>3.4.6</version>
<exclusions>
<exclusion>
<artifactId>slf4j-log4j12</artifactId>
<groupId>org.slf4j</groupId>
</exclusion>
</exclusions>
</dependency>
<!--这里是zookeeper的zkCli的操作包 我们读写服务全靠这个包-->
<dependency>
<groupId>com.github.sgroschupf</groupId>
<artifactId>zkclient</artifactId>
<version>0.1</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-jdbc</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>1.3.2</version>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.38</version>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
</dependencies>
7.在provider中写mapper和service的实现
8.在启动类上添加mapper扫描注解
9.在resources下建这两个文件,用于注册
9.1application.properties
修改需要的url参数和分页插件参数
server.port=9090
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
spring.datasource.url=jdbc:mysql://localhost:3306/kgc
spring.datasource.username=root
spring.datasource.password=ok
mybatis.type-aliases-package=cn.kgc.vo
mybatis.mapper-locations=mapper/*.xml
pagehelper.helper-dialect=mysql
9.2spring-provider.xml
根据需要修改用户服务接口
中的参数,作用是将service的接口注入到Zookeeper
中去
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://code.alibabatech.com/schema/dubbo
http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<!-- 提供方应用信息,用于计算依赖关系 -->
<dubbo:application name="myprovider" />
<!-- 使用zookeeper注册中心暴露服务地址,我的zookeeper是架在本地的 -->
<dubbo:registry protocol="zookeeper" address="127.0.0.1:2181" timeout="60000"/>
<!-- 用dubbo协议在20880端口暴露服务 -->
<dubbo:protocol name="dubbo" port="20880" />
<!-- 用户服务接口 -->
<dubbo:service interface="cn.kgc.service.TableToOneService" ref="tableToOneService"/>
<bean id="tableToOneService" class="cn.kgc.service.TableToOneServiceImpl"/>
<dubbo:service interface="cn.kgc.service.TableToManyService" ref="tableToManyService"/>
<bean id="tableToManyService" class="cn.kgc.service.TableToManyServiceImpl"/>
</beans>
10再修改启动项,添加导入资源注解
11再创建微服务模块,consumer
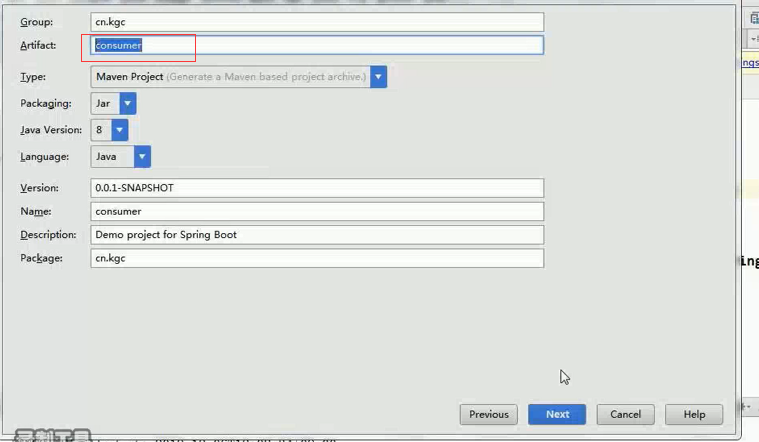
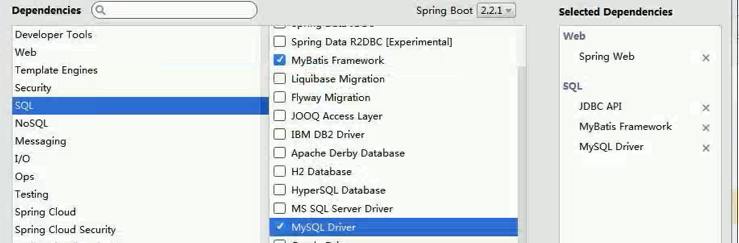
12.把provider中pom的坐标拷贝到consumer
13.把provider中的application文件拷贝到consumer,并按需修改(端口号等)
14.编写controller类和添加spring-consumer.xml
14.1spring-consumer.xml
按需修改service名
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:dubbo="http://code.alibabatech.com/schema/dubbo"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://code.alibabatech.com/schema/dubbo http://code.alibabatech.com/schema/dubbo/dubbo.xsd">
<dubbo:application name="dubbo-consumer"/>
<dubbo:registry check="false" address="zookeeper://127.0.0.1:2181"/>
<dubbo:reference interface="cn.kgc.service.TableToOneService" id="tableToOneService"/>
<dubbo:reference interface="cn.kgc.service.TableToManyService" id="tableToManyService"/>
</beans>
15再启动类上添加导入资源注解
全部代码写完
然后开启Zookeeper服务器,然后开启provider启动类,再开启consumer启动类,最后用postman检测接口是否通畅,根据错误信息修改代码..
整体流程
结束
SpringBoot+idea搭建微服务简化流程的更多相关文章
- Spring-boot:快速搭建微服务框架
前言: Spring Boot是为了简化Spring应用的创建.运行.调试.部署等而出现的,使用它可以做到专注于Spring应用的开发,而无需过多关注XML的配置. 简单来说,它提供了一堆依赖打包,并 ...
- 十分钟搭建微服务框架(SpringBoot +Dubbo+Docker+Jenkins源码)
本文将以原理+实战的方式,首先对“微服务”相关的概念进行知识点扫盲,然后开始手把手教你搭建这一整套的微服务系统. 这套微服务框架能干啥? 这套系统搭建完之后,那可就厉害了: 微服务架构 你的整个应用程 ...
- 【译文】用Spring Cloud和Docker搭建微服务平台
by Kenny Bastani Sunday, July 12, 2015 转自:http://www.kennybastani.com/2015/07/spring-cloud-docker-mi ...
- Spring Cloud和Docker搭建微服务平台
用Spring Cloud和Docker搭建微服务平台 This blog series will introduce you to some of the foundational concepts ...
- spring cloud+dotnet core搭建微服务架构:Api授权认证(六)
前言 这篇文章拖太久了,因为最近实在太忙了,加上这篇文章也非常长,所以花了不少时间,给大家说句抱歉.好,进入正题.目前的项目基本都是前后端分离了,前端分Web,Ios,Android...,后端也基本 ...
- spring cloud+.net core搭建微服务架构:Api授权认证(六)
前言 这篇文章拖太久了,因为最近实在太忙了,加上这篇文章也非常长,所以花了不少时间,给大家说句抱歉.好,进入正题.目前的项目基本都是前后端分离了,前端分Web,Ios,Android...,后端也基本 ...
- 【微服务】使用spring cloud搭建微服务框架,整理学习资料
写在前面 使用spring cloud搭建微服务框架,是我最近最主要的工作之一,一开始我使用bubbo加zookeeper制作了一个基于dubbo的微服务框架,然后被架构师否了,架构师曰:此物过时.随 ...
- 手把手教你使用spring cloud+dotnet core搭建微服务架构:服务治理(-)
背景 公司去年开始使用dotnet core开发项目.公司的总体架构采用的是微服务,那时候由于对微服务的理解并不是太深,加上各种组件的不成熟,只是把项目的各个功能通过业务层面拆分,然后通过nginx代 ...
- spring cloud+dotnet core搭建微服务架构:服务发现(二)
前言 上篇文章实际上只讲了服务治理中的服务注册,服务与服务之间如何调用呢?传统的方式,服务A调用服务B,那么服务A访问的是服务B的负载均衡地址,通过负载均衡来指向到服务B的真实地址,上篇文章已经说了这 ...
随机推荐
- 微信小程序——e.target与e.currentTarget的区别
在小程序的点击事件中,我们经常使用这两个属性来传参,看起来效果一样,查了官方文档如下: target:事件源组件对象 currentTarget:当前组件对象 什么意思?我刚开始就有点不懂,那就直接上 ...
- request获取路径
1.request.getRequestURL() 返回的是完整的url,包括Http协议,端口号,servlet名字和映射路径,但它不包含请求参数. 2.request.getRequestURI( ...
- iNeuOS 工业互联网 从网关到云端一体化解决方案。教你如何做PPT。
iNeuOS 专注打造云端操作系统,提供全新解决方案 (凑够150字) 核心组件包括:边缘网关(iNeuLink).设备容器(iNeuKernel).视图建模(iNeuView).机器 ...
- springboot执行延时任务-DelayQueue的使用
DelayQueue简介 在很多场景我们需要用到延时任务,比如给客户异步转账操作超时后发通知告知用户,还有客户下单后多长时间内没支付则取消订单等等,这些都可以使用延时任务来实现. jdk中DelayQ ...
- 【JS】370- 总结异步编程的六种方式
点击上方"前端自习课"关注,学习起来~ 作者:Aima https://segmentfault.com/a/1190000019188824 众所周知 JavaScript 是 ...
- 【NPM】361- 10个 NPM 使用技巧
点击上方"前端自习课"关注,学习起来~ 对于一个项目,常用的一些npm简单命令包含的功能有: 初始化一个文件夹( npm init ) 下载npm模块( npm install ) ...
- UWP 使用SSL证书,保证数据安全
事情是这样的,我们后端的小伙伴升级了用户会员系统,使用了全新的GraphQL登录机制,并且采用SSL加密的方式来实现阻止陌生客户端请求的案例. GraphQL在UWP端的实现,以后有时间会单独写一篇文 ...
- Oracle常用函数(略微少了点 不过是自己稍微整理的)
DECODE DECODE(value ,if 1, then 1,if 2,then 2, ....,else) 解析: if 条件=1 return (value 1) if条 ...
- cannot resolve symbol AppCompatActivity
记一次配置性的问题 今天复习自定义控件的时候新建一个project,生成的代码冒红,可把我给郁闷了.我知道我的配置是正确的,可是... 它出现了cannot resolve symbol AppCom ...
- python基础知识第七篇(练习)
# a. 获取内容相同的元素列表 l1 = [11,22,33] l2 = [22,33,44] for l in l1: if l in l2: print(l) # b. 获取 l1 中有, l2 ...