ACM学习历程—HDU 5326 Work(树形递推)
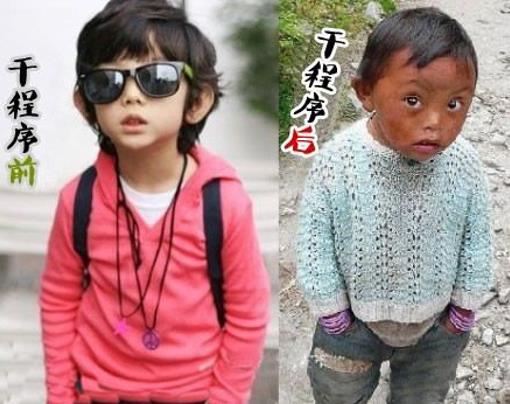
It’s an interesting experience to move from ICPC to work, end my college life and start a brand new journey in company.
As
is known to all, every stuff in a company has a title, everyone except
the boss has a direct leader, and all the relationship forms a tree. If
A’s title is higher than B(A is the direct or indirect leader of B), we
call it A manages B.
Now, give you the relation of a company, can you calculate how many people manage k people.
Each test case begins with two integers n and k, n indicates the number of stuff of the company.
Each of the following n-1 lines has two integers A and B, means A is the direct leader of B.
1 <= n <= 100 , 0 <= k < n
1 <= A, B <= n
这个题目是个递推,不过由于是树形的,需要dfs来完成递推的过程。
关键在于p[now] += p[to]+1;如果now能manage to的话。
此处采用链式前向星来保存关系图。
代码:
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <cmath>
#include <set>
#include <map>
#include <queue>
#include <string>
#include <algorithm>
#define LL long long using namespace std; const int maxN = ; struct Edge
{
int to, next;
}edge[maxN]; int head[maxN], cnt; void addEdge(int u, int v)
{
edge[cnt].to = v;
edge[cnt].next = head[u];
head[u] = cnt;
cnt++;
} void initEdge()
{
memset(head, -, sizeof(head));
cnt = ;
} int n, k;
int fa[maxN], p[maxN]; void input()
{
initEdge();
memset(p, -, sizeof(p));
int u, v;
for (int i = ; i < n; ++i)
{
scanf("%d%d", &u, &v);
addEdge(u, v);
}
} void dfs(int now)
{
p[now] = ;
int to;
for (int i = head[now]; i != -; i = edge[i].next)
{
to = edge[i].to;
if (p[to] == -)
dfs(to);
p[now] += p[to]+;
}
} void work()
{
int ans = ;
for (int i = ; i <= n; ++i)
{
if (p[i] != -)
{
if (p[i] == k)
ans++;
}
else
{
dfs(i);
if (p[i] == k)
ans++;
}
}
printf("%d\n", ans);
} int main()
{
//freopen("test.in", "r", stdin);
while (scanf("%d%d", &n, &k) != EOF)
{
input();
work();
}
return ;
}
ACM学习历程—HDU 5326 Work(树形递推)的更多相关文章
- ACM学习历程—HDU1041 Computer Transformation(递推 && 大数)
Description A sequence consisting of one digit, the number 1 is initially written into a computer. A ...
- ACM学习历程—ZOJ3777 Problem Arrangement(递推 && 状压)
Description The 11th Zhejiang Provincial Collegiate Programming Contest is coming! As a problem sett ...
- ACM学习历程——HDU4472 Count(数学递推) (12年长春区域赛)
Description Prof. Tigris is the head of an archaeological team who is currently in charge of an exca ...
- ACM学习历程—HDU 5451 Best Solver(Fibonacci数列 && 快速幂)(2015沈阳网赛1002题)
Problem Description The so-called best problem solver can easily solve this problem, with his/her ch ...
- ACM学习历程—HDU 5459 Jesus Is Here(递推)(2015沈阳网赛1010题)
Sample Input 9 5 6 7 8 113 1205 199312 199401 201314 Sample Output Case #1: 5 Case #2: 16 Case #3: 8 ...
- ACM学习历程—HDU 5512 Pagodas(数学)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=5512 学习菊苣的博客,只粘链接,不粘题目描述了. 题目大意就是给了初始的集合{a, b},然后取集合里 ...
- ACM学习历程—HDU 3915 Game(Nim博弈 && xor高斯消元)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=3915 题目大意是给了n个堆,然后去掉一些堆,使得先手变成必败局势. 首先这是个Nim博弈,必败局势是所 ...
- ACM学习历程—HDU 5536 Chip Factory(xor && 字典树)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=5536 题目大意是给了一个序列,求(si+sj)^sk的最大值. 首先n有1000,暴力理论上是不行的. ...
- ACM学习历程—HDU 5534 Partial Tree(动态规划)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=5534 题目大意是给了n个结点,让后让构成一个树,假设每个节点的度为r1, r2, ...rn,求f(x ...
随机推荐
- java Map 实现类的对比
java为数据结构中的映射定义了一个接口 java.util.Map ,他有四个实现类
- Laravel 5.4的本地化
简介 Laravel 的本地化功能提供方便的方法来获取多语言的字符串,让你的网站可以简单的支持多语言. 语言包存放在 resources/lang 目录下的文件里.在此目录中应该有应用对应支持的语言并 ...
- 数据结构(Java语言)——Stack简单实现
栈是限制插入和删除仅仅能在一个位置上进行的表.该位置是表的末端,叫做栈的顶top.对栈的基本操作有进栈push和出栈pop,前者相当于插入.后者这是删除最后插入的元素. 栈有时又叫先进先出FIFO表. ...
- Struts2实现input数据回显
/** 修改页面 */ public String editUI() { //准备回显得数据 Role role = roleService.getById(id); ...
- 编写mipsel mt7620 Led驱动(一)
1.看原理图中知芯片上66引脚控制一个LED 2.在Datasheet中找出GPIO pin 3.在ProgrammingGuid System Contrl中找到GPIO控制寄存器地址: 4.控制 ...
- WinDbg调试分析 net站点 CPU100%问题
WinDbg调试分析 asp.net站点 CPU100%问题 公司为了节省成本,最近有一批服务器降了配置,CPU从8核降到了2核.本身是小站点,访问量也不高,CPU总是会飙到100%而且可以一直持续几 ...
- EasyUI触发方法、触发事件、创建对象的格式??
创建对象 $("选择器").组件名({ 属性名 : 值, 属性名 : 值 }); 触发方法 $("选择器").组件名("方法名",参数); ...
- JavaScript -- 没事看看
@.delete 原文:https://developer.mozilla.org/zh-CN/docs/Web/JavaScript/Reference/Operators/delete
- NVR硬件录像机web无插件播放方案功能实现之相关接口注意事项说明
该篇博文主要用来说明EasyNVR硬件录像回放版本的相关接口说明和调用的demo: 方便用户的二次开发和集成. 软件根目录会包含接口文档的,因此,本文主要是对一些特定接口的说明和接口实现功能的讲解以及 ...
- Python --- Scrapy 命令(转)
Scrapy 命令 分为两种: 全局命令 和 项目命令 . 全局命令:在哪里都能使用. 项目命令:必须在爬虫项目里面才能使用. 全局命令 C:\Users\AOBO>scrapy -h Scra ...