python s12 day3
python s12 day3
深浅拷贝
对于 数字 和 字符串 而言,赋值、浅拷贝和深拷贝无意义,因为其永远指向同一个内存地址。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
import copy # ######### 数字、字符串 ######### n1 = 123 # n1 = "i am alex age 10" print ( id (n1)) # ## 赋值 ## n2 = n1 print ( id (n2)) # ## 浅拷贝 ## n2 = copy.copy(n1) print ( id (n2)) # ## 深拷贝 ## n3 = copy.deepcopy(n1) print ( id (n3)) |
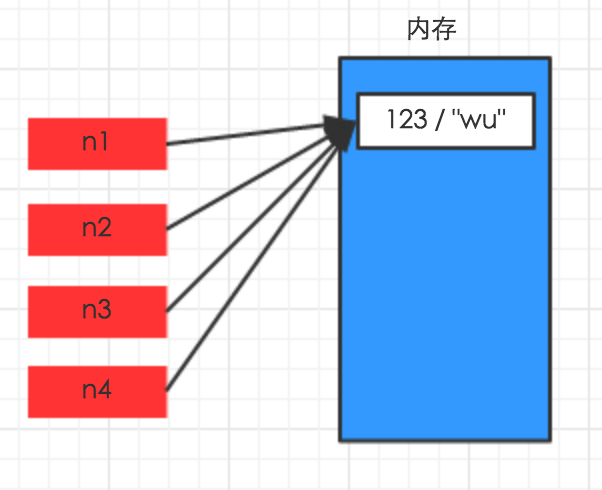
对于字典、元祖、列表 而言,进行赋值、浅拷贝和深拷贝时,其内存地址的变化是不同的。
赋值,只是创建一个变量,该变量指向原来内存地址,如:
1
2
3
|
n1 = { "k1" : "wu" , "k2" : 123 , "k3" : [ "alex" , 456 ]} n2 = n1 |
浅拷贝,在内存中只额外创建第一层数据
1
2
3
4
5
|
import copy n1 = { "k1" : "wu" , "k2" : 123 , "k3" : [ "alex" , 456 ]} n3 = copy.copy(n1) |
深拷贝,在内存中将所有的数据重新创建一份(排除最后一层,即:python内部对字符串和数字的优化)
1
2
3
4
5
|
import copy n1 = { "k1" : "wu" , "k2" : 123 , "k3" : [ "alex" , 456 ]} n4 = copy.deepcopy(n1) |
函数
一、背景
在学习函数之前,一直遵循:面向过程编程,即:根据业务逻辑从上到下实现功能,其往往用一长段代码来实现指定功能,开发过程中最常见的操作就是粘贴复制,也就是将之前实现的代码块复制到现需功能处,如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
while True : if cpu利用率 > 90 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 if 硬盘使用空间 > 90 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 if 内存占用 > 80 % : #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 |
腚眼一看上述代码,if条件语句下的内容可以被提取出来公用,如下:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
def 发送邮件(内容) #发送邮件提醒 连接邮箱服务器 发送邮件 关闭连接 while True : if cpu利用率 > 90 % : 发送邮件( 'CPU报警' ) if 硬盘使用空间 > 90 % : 发送邮件( '硬盘报警' ) if 内存占用 > 80 % : |
对于上述的两种实现方式,第二次必然比第一次的重用性和可读性要好,其实这就是函数式编程和面向过程编程的区别:
- 函数式:将某功能代码封装到函数中,日后便无需重复编写,仅调用函数即可
- 面向对象:对函数进行分类和封装,让开发“更快更好更强...”
函数式编程最重要的是增强代码的重用性和可读性
二、定义和使用
1
2
3
4
5
|
def 函数名(参数): ... 函数体 ... |
函数的定义主要有如下要点:
- def:表示函数的关键字
- 函数名:函数的名称,日后根据函数名调用函数
- 函数体:函数中进行一系列的逻辑计算,如:发送邮件、计算出 [11,22,38,888,2]中的最大数等...
- 参数:为函数体提供数据
- 返回值:当函数执行完毕后,可以给调用者返回数据。
以上要点中,比较重要有参数和返回值:
1、返回值
函数是一个功能块,该功能到底执行成功与否,需要通过返回值来告知调用者。
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
|
def 发送短信(): 发送短信的代码... if 发送成功: return True else : return False while True : # 每次执行发送短信函数,都会将返回值自动赋值给result # 之后,可以根据result来写日志,或重发等操作 result = 发送短信() if result = = False : 记录日志,短信发送失败... |
2、参数
为什么要有参数?

- def CPU报警邮件()
- #发送邮件提醒
- 连接邮箱服务器
- 发送邮件
- 关闭连接
- def 硬盘报警邮件()
- #发送邮件提醒
- 连接邮箱服务器
- 发送邮件
- 关闭连接
- def 内存报警邮件()
- #发送邮件提醒
- 连接邮箱服务器
- 发送邮件
- 关闭连接
- while True:
- if cpu利用率 > 90%:
- CPU报警邮件()
- if 硬盘使用空间 > 90%:
- 硬盘报警邮件()
- if 内存占用 > 80%:
- 内存报警邮件()


- def 发送邮件(邮件内容)
- #发送邮件提醒
- 连接邮箱服务器
- 发送邮件
- 关闭连接
- while True:
- if cpu利用率 > 90%:
- 发送邮件("CPU报警了。")
- if 硬盘使用空间 > 90%:
- 发送邮件("硬盘报警了。")
- if 内存占用 > 80%:
- 发送邮件("内存报警了。")

函数的有三中不同的参数:
- 普通参数
- 默认参数
- 动态参数

- # ######### 定义函数 #########
- # name 叫做函数func的形式参数,简称:形参
- def func(name):
- print name
- # ######### 执行函数 #########
- # 'wupeiqi' 叫做函数func的实际参数,简称:实参
- func('wupeiqi')


- def func(name, age = 18):
- print "%s:%s" %(name,age)
- # 指定参数
- func('wupeiqi', 19)
- # 使用默认参数
- func('alex')
- 注:默认参数需要放在参数列表最后


- def func(*args):
- print args
- # 执行方式一
- func(11,33,4,4454,5)
- # 执行方式二
- li = [11,2,2,3,3,4,54]
- func(*li)


- def func(**kwargs):
- print args
- # 执行方式一
- func(name='wupeiqi',age=18)
- # 执行方式二
- li = {'name':'wupeiqi', age:18, 'gender':'male'}
- func(**li)

- def func(*args, **kwargs):
- print args
- print kwargs
扩展:发送邮件实例
1
2
3
4
5
6
7
8
9
10
11
12
13
14
|
import smtplib from email.mime.text import MIMEText from email.utils import formataddr msg = MIMEText( '邮件内容' , 'plain' , 'utf-8' ) msg[ 'From' ] = formataddr([ "武沛齐" , 'wptawy@126.com' ]) msg[ 'To' ] = formataddr([ "走人" , '424662508@qq.com' ]) msg[ 'Subject' ] = "主题" server = smtplib.SMTP( "smtp.126.com" , 25 ) server.login( "wptawy@126.com" , "邮箱密码" ) server.sendmail( 'wptawy@126.com' , [ '424662508@qq.com' ,], msg.as_string()) server.quit() |
内置函数
注:查看详细猛击这里
open函数,该函数用于文件处理
操作文件时,一般需要经历如下步骤:
- 打开文件
- 操作文件
一、打开文件
1
|
文件句柄 = open ( '文件路径' , '模式' ) |
打开文件时,需要指定文件路径和以何等方式打开文件,打开后,即可获取该文件句柄,日后通过此文件句柄对该文件操作。
打开文件的模式有:
- r,只读模式(默认)。
- w,只写模式。【不可读;不存在则创建;存在则删除内容;】
- a,追加模式。【可读; 不存在则创建;存在则只追加内容;】
"+" 表示可以同时读写某个文件
- r+,可读写文件。【可读;可写;可追加】
- w+,写读
- a+,同a
"U"表示在读取时,可以将 \r \n \r\n自动转换成 \n (与 r 或 r+ 模式同使用)
- rU
- r+U
"b"表示处理二进制文件(如:FTP发送上传ISO镜像文件,linux可忽略,windows处理二进制文件时需标注)
- rb
- wb
- ab
二、操作

- class file(object)
- def close(self): # real signature unknown; restored from __doc__
- 关闭文件
- """
- close() -> None or (perhaps) an integer. Close the file.
- Sets data attribute .closed to True. A closed file cannot be used for
- further I/O operations. close() may be called more than once without
- error. Some kinds of file objects (for example, opened by popen())
- may return an exit status upon closing.
- """
- def fileno(self): # real signature unknown; restored from __doc__
- 文件描述符
- """
- fileno() -> integer "file descriptor".
- This is needed for lower-level file interfaces, such os.read().
- """
- return 0
- def flush(self): # real signature unknown; restored from __doc__
- 刷新文件内部缓冲区
- """ flush() -> None. Flush the internal I/O buffer. """
- pass
- def isatty(self): # real signature unknown; restored from __doc__
- 判断文件是否是同意tty设备
- """ isatty() -> true or false. True if the file is connected to a tty device. """
- return False
- def next(self): # real signature unknown; restored from __doc__
- 获取下一行数据,不存在,则报错
- """ x.next() -> the next value, or raise StopIteration """
- pass
- def read(self, size=None): # real signature unknown; restored from __doc__
- 读取指定字节数据
- """
- read([size]) -> read at most size bytes, returned as a string.
- If the size argument is negative or omitted, read until EOF is reached.
- Notice that when in non-blocking mode, less data than what was requested
- may be returned, even if no size parameter was given.
- """
- pass
- def readinto(self): # real signature unknown; restored from __doc__
- 读取到缓冲区,不要用,将被遗弃
- """ readinto() -> Undocumented. Don't use this; it may go away. """
- pass
- def readline(self, size=None): # real signature unknown; restored from __doc__
- 仅读取一行数据
- """
- readline([size]) -> next line from the file, as a string.
- Retain newline. A non-negative size argument limits the maximum
- number of bytes to return (an incomplete line may be returned then).
- Return an empty string at EOF.
- """
- pass
- def readlines(self, size=None): # real signature unknown; restored from __doc__
- 读取所有数据,并根据换行保存值列表
- """
- readlines([size]) -> list of strings, each a line from the file.
- Call readline() repeatedly and return a list of the lines so read.
- The optional size argument, if given, is an approximate bound on the
- total number of bytes in the lines returned.
- """
- return []
- def seek(self, offset, whence=None): # real signature unknown; restored from __doc__
- 指定文件中指针位置
- """
- seek(offset[, whence]) -> None. Move to new file position.
- Argument offset is a byte count. Optional argument whence defaults to
- (offset from start of file, offset should be >= 0); other values are 1
- (move relative to current position, positive or negative), and 2 (move
- relative to end of file, usually negative, although many platforms allow
- seeking beyond the end of a file). If the file is opened in text mode,
- only offsets returned by tell() are legal. Use of other offsets causes
- undefined behavior.
- Note that not all file objects are seekable.
- """
- pass
- def tell(self): # real signature unknown; restored from __doc__
- 获取当前指针位置
- """ tell() -> current file position, an integer (may be a long integer). """
- pass
- def truncate(self, size=None): # real signature unknown; restored from __doc__
- 截断数据,仅保留指定之前数据
- """
- truncate([size]) -> None. Truncate the file to at most size bytes.
- Size defaults to the current file position, as returned by tell().
- """
- pass
- def write(self, p_str): # real signature unknown; restored from __doc__
- 写内容
- """
- write(str) -> None. Write string str to file.
- Note that due to buffering, flush() or close() may be needed before
- the file on disk reflects the data written.
- """
- pass
- def writelines(self, sequence_of_strings): # real signature unknown; restored from __doc__
- 将一个字符串列表写入文件
- """
- writelines(sequence_of_strings) -> None. Write the strings to the file.
- Note that newlines are not added. The sequence can be any iterable object
- producing strings. This is equivalent to calling write() for each string.
- """
- pass
- def xreadlines(self): # real signature unknown; restored from __doc__
- 可用于逐行读取文件,非全部
- """
- xreadlines() -> returns self.
- For backward compatibility. File objects now include the performance
- optimizations previously implemented in the xreadlines module.
- """
- pass


- class TextIOWrapper(_TextIOBase):
- """
- Character and line based layer over a BufferedIOBase object, buffer.
- encoding gives the name of the encoding that the stream will be
- decoded or encoded with. It defaults to locale.getpreferredencoding(False).
- errors determines the strictness of encoding and decoding (see
- help(codecs.Codec) or the documentation for codecs.register) and
- defaults to "strict".
- newline controls how line endings are handled. It can be None, '',
- '\n', '\r', and '\r\n'. It works as follows:
- * On input, if newline is None, universal newlines mode is
- enabled. Lines in the input can end in '\n', '\r', or '\r\n', and
- these are translated into '\n' before being returned to the
- caller. If it is '', universal newline mode is enabled, but line
- endings are returned to the caller untranslated. If it has any of
- the other legal values, input lines are only terminated by the given
- string, and the line ending is returned to the caller untranslated.
- * On output, if newline is None, any '\n' characters written are
- translated to the system default line separator, os.linesep. If
- newline is '' or '\n', no translation takes place. If newline is any
- of the other legal values, any '\n' characters written are translated
- to the given string.
- If line_buffering is True, a call to flush is implied when a call to
- write contains a newline character.
- """
- def close(self, *args, **kwargs): # real signature unknown
- 关闭文件
- pass
- def fileno(self, *args, **kwargs): # real signature unknown
- 文件描述符
- pass
- def flush(self, *args, **kwargs): # real signature unknown
- 刷新文件内部缓冲区
- pass
- def isatty(self, *args, **kwargs): # real signature unknown
- 判断文件是否是同意tty设备
- pass
- def read(self, *args, **kwargs): # real signature unknown
- 读取指定字节数据
- pass
- def readable(self, *args, **kwargs): # real signature unknown
- 是否可读
- pass
- def readline(self, *args, **kwargs): # real signature unknown
- 仅读取一行数据
- pass
- def seek(self, *args, **kwargs): # real signature unknown
- 指定文件中指针位置
- pass
- def seekable(self, *args, **kwargs): # real signature unknown
- 指针是否可操作
- pass
- def tell(self, *args, **kwargs): # real signature unknown
- 获取指针位置
- pass
- def truncate(self, *args, **kwargs): # real signature unknown
- 截断数据,仅保留指定之前数据
- pass
- def writable(self, *args, **kwargs): # real signature unknown
- 是否可写
- pass
- def write(self, *args, **kwargs): # real signature unknown
- 写内容
- pass
- def __getstate__(self, *args, **kwargs): # real signature unknown
- pass
- def __init__(self, *args, **kwargs): # real signature unknown
- pass
- @staticmethod # known case of __new__
- def __new__(*args, **kwargs): # real signature unknown
- """ Create and return a new object. See help(type) for accurate signature. """
- pass
- def __next__(self, *args, **kwargs): # real signature unknown
- """ Implement next(self). """
- pass
- def __repr__(self, *args, **kwargs): # real signature unknown
- """ Return repr(self). """
- pass
- buffer = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- closed = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- encoding = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- errors = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- line_buffering = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- name = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- newlines = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- _CHUNK_SIZE = property(lambda self: object(), lambda self, v: None, lambda self: None) # default
- _finalizing = property(lambda self: object(), lambda self, v: None, lambda self: None) # default

三、管理上下文
为了避免打开文件后忘记关闭,可以通过管理上下文,即:
1
2
3
|
with open ( 'log' , 'r' ) as f: ... |
如此方式,当with代码块执行完毕时,内部会自动关闭并释放文件资源。
在Python 2.7 后,with又支持同时对多个文件的上下文进行管理,即:
1
2
|
with open ( 'log1' ) as obj1, open ( 'log2' ) as obj2: pass |
lambda表达式
学习条件运算时,对于简单的 if else 语句,可以使用三元运算来表示,即:
1
2
3
4
5
6
7
8
|
# 普通条件语句 if 1 = = 1 : name = 'wupeiqi' else : name = 'alex' # 三元运算 name = 'wupeiqi' if 1 = = 1 else 'alex' |
对于简单的函数,也存在一种简便的表示方式,即:lambda表达式
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
|
# ###################### 普通函数 ###################### # 定义函数(普通方式) def func(arg): return arg + 1 # 执行函数 result = func( 123 ) # ###################### lambda ###################### # 定义函数(lambda表达式) my_lambda = lambda arg : arg + 1 # 执行函数 result = my_lambda( 123 ) |
lambda存在意义就是对简单函数的简洁表示
递归
利用函数编写如下数列:
斐波那契数列指的是这样一个数列 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233,377,610,987,1597,2584,4181,6765,10946,17711,28657,46368...
1
2
3
4
5
6
7
8
|
def func(arg1,arg2): if arg1 = = 0 : print arg1, arg2 arg3 = arg1 + arg2 print arg3 func(arg2, arg3) func( 0 , 1 ) |
装饰器
装饰器是函数,只不过该函数可以具有特殊的含义,装饰器用来装饰函数或类,使用装饰器可以在函数执行前和执行后添加相应操作。
1
2
3
4
5
6
7
8
9
10
|
def wrapper(func): def result(): print 'before' func() print 'after' return result @wrapper def foo(): print 'foo' |

- import functools
- def wrapper(func):
- @functools.wraps(func)
- def wrapper():
- print 'before'
- func()
- print 'after'
- return wrapper
- @wrapper
- def foo():
- print 'foo'

详细猛击这里
python s12 day3的更多相关文章
- Python s12 Day3 笔记及作业
1. Set集合 old_dict = { "#1":{ 'hostname':'c1', 'cpu_count':2, 'mem_capicity':16}, "#2& ...
- python2.0 s12 day3
s12 day3 视频每节的内容 03 python s12 day3 本节内容概要 第三天的主要内容 上节没讲完的: 6.集合 7.collections 1)计数器 2)有序字典 3)默认字典 4 ...
- python笔记 - day3
python笔记 - day3 参考:http://www.cnblogs.com/wupeiqi/articles/5453708.html set特性: 1.无序 2.不重复 3.可嵌套 函数: ...
- python s12 day2
python s12 day2 入门知识拾遗 http://www.cnblogs.com/wupeiqi/articles/4906230.html 基本数据类型 注:查看对象相关成员 var, ...
- python学习 day3 (3月4日)---字符串
字符串: 下标(索引) 切片[起始:终止] 步长[起始:终止:1] 或者-1 从后往前 -1 -2 -3 15个专属方法: 1-6 : 格式:大小写 , 居中(6) s.capitalize() s ...
- python基础 Day3
python Day3 1.作业回顾 设定一个理想的数字比如88,让用户输入数字,如果比88大,则显示猜测的结果大:如果比66小,则显示猜测的结果小了,给用户三次猜测机会,如果显示猜测正确退出循环,如 ...
- Python学习day3作业
days3作业 作业需求 HAproxy配置文件操作 根据用户输入,输出对应的backend下的server信息 可添加backend 和sever信息 可修改backend 和sever信息 可删除 ...
- python基础:day3作业
修改haproxy配置文件 基本功能:1.获取记录2.添加记录3.删除记录 代码结构:三个函数一个主函数 知识点:1.python简单数据结构的使用:列表.字典等 2.python两个模块的使用:os ...
- python学习-day3
今天是第三天学习,加油! 第一部分 集合 一.集合 1.什么是集合以及特性? 特性:无序的,不重复的序列,可嵌套. 2.创建集合 方法一:创建空集合 s1 = set() print(type(s1) ...
随机推荐
- 【Java】Java Platform
The Java platform has two components: The Java Virtual Machine The Java Application Programming Inte ...
- BZOJ 3122 随机数生成器
http://www.lydsy.com/JudgeOnline/problem.php?id=3122 题意:给出p,a,b,x1,t 已知xn=a*xn-1+b%p,求最小的n令xn=t 首先,若 ...
- ViewHolder VS HolderView ?
ViewHolder 模式在 Android 中大家应该都不陌生了,特别是在 ListView 中通过 ViewHolder 来减少 findViewById 的调用和 类型的转换. 而 Holder ...
- Java中如何创建进程(转)
在Java中,可以通过两种方式来创建进程,总共涉及到5个主要的类. 第一种方式是通过Runtime.exec()方法来创建一个进程,第二种方法是通过ProcessBuilder的start方法来创建进 ...
- 几种交换两个数函数(swap函数)的写法和解析
#include <iostream> using namespace std; /*值传递,局部变量a和b的值确实在调用swap0时变化了,当结束时,他们绳命周期结束*/ void sw ...
- linux下USB串口,minicom
[一].驱动相关说明: 如果直接使用串口线,而没有用到USB转串口设备,就不需要安装驱动. 如果使用了USB转串口,一般情况下也不需要安装驱动了,目前linux系统已经包含了该驱动,可以自动识别,亦可 ...
- Python异常处理实例
#coding=utf-8 #---异常处理--- # 写一个自己定义的异常类 class MyInputException(Exception): def __init__(self, length ...
- lazyman学习
1.安装: gem install lazyman 2.建立工程: cd到工程目录下 lazyman new 工程名 3.打开调试命令 lazyman c lazyman调用selenium-webd ...
- Qt 与 JavaScript 通信
使用QWebView加载网页后,解决Qt与JavaScript通信的问题: The QtWebKit Bridge :http://qt-project.org/doc/qt-4.8/qtwebkit ...
- 适配器模式 java
结构模式:将类和对象结合在一起构成更大的结构,就像是搭积木. 1.适配器模式 源接口---适配器--目标接口 2.使用场景: 现在你有一个很古老的类,里面的一些方法很有用,你如何使用这些方法? 当然你 ...