Web UI开发神器—Kendo UI for jQuery数据管理网格编辑操作
Kendo UI目前最新提供Kendo UI for jQuery、Kendo UI for Angular、Kendo UI Support for React和Kendo UI Support for Vue四个控件。Kendo UI for jQuery是创建现代Web应用程序的最完整UI库。
编辑是Kendo UI网格的基本功能,可让您操纵其数据的显示方式。
Kendo UI Grid提供以下编辑模式:
- 批量编辑
- 内联编辑
- 弹出式编辑
- 自定义编辑
批量编辑
网格使您能够进行和保存批量更新。要在网格中启用批处理编辑操作,请将数据源的批处理选项设置为true。
<!DOCTYPE html>
<html>
<head><title></title><link rel="stylesheet" href="styles/kendo.common.min.css" /><link rel="stylesheet" href="styles/kendo.default.min.css" /><link rel="stylesheet" href="styles/kendo.default.mobile.min.css" />
<script src="js/jquery.min.js"></script>
<script src="js/kendo.all.min.js"></script>
</head>
<body>
<div id="example">
<div id="grid"></div>
<script>
$(document).ready(function () {
var crudServiceBaseUrl = "https://demos.telerik.com/kendo-ui/service",
dataSource = new kendo.data.DataSource({
transport: {
read: {
url: crudServiceBaseUrl + "/Products",
dataType: "jsonp"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function(options, operation) {
if (operation !== "read" && options.models) {
return {models: kendo.stringify(options.models)};
}
}
},
batch: true,
pageSize: 20,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true } }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
navigatable: true,
pageable: true,
height: 550,
toolbar: ["create", "save", "cancel"],
columns: [
"ProductName",
{ field: "UnitPrice", title: "Unit Price", format: "{0:c}", width: 120 },
{ field: "UnitsInStock", title: "Units In Stock", width: 120 },
{ field: "Discontinued", width: 120, editor: customBoolEditor },
{ command: "destroy", title: " ", width: 150 }],
editable: true
});
});
function customBoolEditor(container, options) {
var guid = kendo.guid();
$('<input class="k-checkbox" id="' + guid + '" type="checkbox" name="Discontinued" data-type="boolean" data-bind="checked:Discontinued">').appendTo(container);
$('<label class="k-checkbox-label" for="' + guid + '"></label>').appendTo(container);
}
</script>
</div>
</body>
</html>
内联编辑
当用户单击一行时,网格提供用于内联编辑其数据的选项。要启用内联编辑操作,请将Grid的editable选项设置为inline。
<!DOCTYPE html>
<html>
<head><title></title><link rel="stylesheet" href="styles/kendo.common.min.css" /><link rel="stylesheet" href="styles/kendo.default.min.css" /><link rel="stylesheet" href="styles/kendo.default.mobile.min.css" />
<script src="js/jquery.min.js"></script>
<script src="js/kendo.all.min.js"></script>
</head>
<body>
<div id="example">
<div id="grid"></div>
<script>
$(document).ready(function () {
var crudServiceBaseUrl = "https://demos.telerik.com/kendo-ui/service",
dataSource = new kendo.data.DataSource({
transport: {
read: {
url: crudServiceBaseUrl + "/Products",
dataType: "jsonp"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function(options, operation) {
if (operation !== "read" && options.models) {
return {models: kendo.stringify(options.models)};
}
}
},
batch: true,
pageSize: 20,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true } }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
pageable: true,
height: 550,
toolbar: ["create"],
columns: [
"ProductName",
{ field: "UnitPrice", title: "Unit Price", format: "{0:c}", width: "120px" },
{ field: "UnitsInStock", title:"Units In Stock", width: "120px" },
{ field: "Discontinued", width: "120px", editor: customBoolEditor },
{ command: ["edit", "destroy"], title: " ", width: "250px" }],
editable: "inline"
});
});
function customBoolEditor(container, options) {
var guid = kendo.guid();
$('<input class="k-checkbox" id="' + guid + '" type="checkbox" name="Discontinued" data-type="boolean" data-bind="checked:Discontinued">').appendTo(container);
$('<label class="k-checkbox-label" for="' + guid + '"></label>').appendTo(container);
}
</script>
</div>
</body>
</html>
弹出式编辑
网格提供用于在弹出窗口中编辑其数据的选项。要启用弹出窗口编辑操作,请将Grid的editable选项设置为popup。
<!DOCTYPE html>
<html>
<head><title></title><link rel="stylesheet" href="styles/kendo.common.min.css" /><link rel="stylesheet" href="styles/kendo.default.min.css" /><link rel="stylesheet" href="styles/kendo.default.mobile.min.css" />
<script src="js/jquery.min.js"></script>
<script src="js/kendo.all.min.js"></script>
</head>
<body>
<div id="example">
<div id="grid"></div>
<script>
$(document).ready(function () {
var crudServiceBaseUrl = "https://demos.telerik.com/kendo-ui/service",
dataSource = new kendo.data.DataSource({
transport: {
read: {
url: crudServiceBaseUrl + "/Products",
dataType: "jsonp"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function(options, operation) {
if (operation !== "read" && options.models) {
return {models: kendo.stringify(options.models)};
}
}
},
batch: true,
pageSize: 20,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true } }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
pageable: true,
height: 550,
toolbar: ["create"],
columns: [
{ field:"ProductName", title: "Product Name" },
{ field: "UnitPrice", title:"Unit Price", format: "{0:c}", width: "120px" },
{ field: "UnitsInStock", title:"Units In Stock", width: "120px" },
{ field: "Discontinued", width: "120px", editor: customBoolEditor },
{ command: ["edit", "destroy"], title: " ", width: "250px" }],
editable: "popup"
});
});
function customBoolEditor(container, options) {
var guid = kendo.guid();
$('<input class="k-checkbox" id="' + guid + '" type="checkbox" name="Discontinued" data-type="boolean" data-bind="checked:Discontinued">').appendTo(container);
$('<label class="k-checkbox-label" for="' + guid + '"></label>').appendTo(container);
}
</script>
</div>
</body>
</html>
自定义编辑
网格使您可以实现自定义列编辑器,并指定在用户编辑数据时适用的验证规则。
实施自定义编辑器
要在网格中实现自定义编辑器,请指定相应列的编辑器字段。 该字段的值将指向JavaScript函数,该函数将实例化对应列单元格的列编辑器。
<!DOCTYPE html>
<html>
<head><title></title><link rel="stylesheet" href="styles/kendo.common.min.css" /><link rel="stylesheet" href="styles/kendo.default.min.css" /><link rel="stylesheet" href="styles/kendo.default.mobile.min.css" />
<script src="js/jquery.min.js"></script>
<script src="js/kendo.all.min.js"></script>
</head>
<body>
<script src="../content/shared/js/products.js"></script>
<div id="example">
<div id="grid"></div>
<script>
$(document).ready(function () {
var dataSource = new kendo.data.DataSource({
pageSize: 20,
data: products,
autoSync: true,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: { validation: { required: true } },
Category: { defaultValue: { CategoryID: 1, CategoryName: "Beverages"} },
UnitPrice: { type: "number", validation: { required: true, min: 1} }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
pageable: true,
height: 550,
toolbar: ["create"],
columns: [
{ field:"ProductName",title:"Product Name" },
{ field: "Category", title: "Category", width: "180px", editor: categoryDropDownEditor, template: "#=Category.CategoryName#" },
{ field: "UnitPrice", title:"Unit Price", format: "{0:c}", width: "130px" },
{ command: "destroy", title: " ", width: "150px" }],
editable: true
});
});
function categoryDropDownEditor(container, options) {
$('<input required name="' + options.field + '"/>')
.appendTo(container)
.kendoDropDownList({
autoBind: false,
dataTextField: "CategoryName",
dataValueField: "CategoryID",
dataSource: {
type: "odata",
transport: {
read: "https://demos.telerik.com/kendo-ui/service/Northwind.svc/Categories"
}
}
});
}
</script>
</div>
</body>
</html>
设置验证规则
要为编辑操作定义验证规则,请在数据源的架构中配置验证选项。
<!DOCTYPE html>
<html>
<head><title></title><link rel="stylesheet" href="styles/kendo.common.min.css" /><link rel="stylesheet" href="styles/kendo.default.min.css" /><link rel="stylesheet" href="styles/kendo.default.mobile.min.css" />
<script src="js/jquery.min.js"></script>
<script src="js/kendo.all.min.js"></script>
</head>
<body>
<div id="example">
<div id="grid"></div>
<script>
$(document).ready(function () {
var crudServiceBaseUrl = "https://demos.telerik.com/kendo-ui/service",
dataSource = new kendo.data.DataSource({
transport: {
read: {
url: crudServiceBaseUrl + "/Products",
dataType: "jsonp"
},
update: {
url: crudServiceBaseUrl + "/Products/Update",
dataType: "jsonp"
},
destroy: {
url: crudServiceBaseUrl + "/Products/Destroy",
dataType: "jsonp"
},
create: {
url: crudServiceBaseUrl + "/Products/Create",
dataType: "jsonp"
},
parameterMap: function (options, operation) {
if (operation !== "read" && options.models) {
return { models: kendo.stringify(options.models) };
}
}
},
batch: true,
pageSize: 20,
schema: {
model: {
id: "ProductID",
fields: {
ProductID: { editable: false, nullable: true },
ProductName: {
validation: {
required: true,
productnamevalidation: function (input) {
if (input.is("[name='ProductName']") && input.val() != "") {
input.attr("data-productnamevalidation-msg", "Product Name should start with capital letter");
return /^[A-Z]/.test(input.val());
}
return true;
}
}
},
UnitPrice: { type: "number", validation: { required: true, min: 1} },
Discontinued: { type: "boolean" },
UnitsInStock: { type: "number", validation: { min: 0, required: true} }
}
}
}
});
$("#grid").kendoGrid({
dataSource: dataSource,
pageable: true,
height: 550,
toolbar: ["create"],
columns: [
"ProductName",
{ field: "UnitPrice", title: "Unit Price", format: "{0:c}", width: "120px" },
{ field: "UnitsInStock", title: "Units In Stock", width: "120px" },
{ field: "Discontinued", width: "120px" },
{ command: ["edit", "destroy"], title: " ", width: "250px"}],
editable: "inline"
});
});
</script>
</div>
</body>
</html>
了解最新Kendo UI最新资讯,请关注Telerik中文网!
扫描关注慧聚IT微信公众号,及时获取最新动态及最新资讯
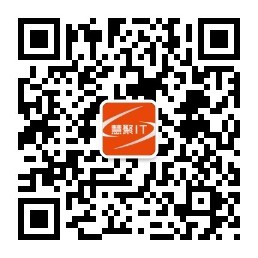
Web UI开发神器—Kendo UI for jQuery数据管理网格编辑操作的更多相关文章
- Web UI开发神器—Kendo UI for jQuery数据管理之过滤操作
Kendo UI for jQuery最新试用版下载 Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support f ...
- [置顶] Kendo UI开发教程: Kendo UI 示例及总结
前面基本介绍完Kendo UI开发的基本概念和开发步骤,Kendo UI的示例网站为http://demos.kendoui.com/ ,包含了三个部分 Web DemoMobile DemoData ...
- HTML5 Web app开发工具Kendo UI Web中如何绑定网格到远程数据
在前面的文章中对于Kendo UI中的Grid控件的一些基础的配置和使用做了一些介绍,本文来看看如何将Kendo UI 中的Grid网格控件绑定到远程数据. 众所周知Grid网格控件是用户界面的一个重 ...
- HTML5 Web app开发工具Kendo UI Web中Grid网格控件的使用
Kendo UI Web中的Grid控件不仅可以显示数据,并对数据提供了丰富的支持,包括分页.排序.分组.选择等,同时还有着大量的配置选项.使用Kendo DataSource组件,可以绑定到本地的J ...
- Web前端开发神器--WebStorm(JavaScript 开发工具) 8.0.3 中文汉化破解版
WebStorm(JavaScript 开发工具) 8.0.3 中文汉化破解版 http://www.jb51.net/softs/171905.html WebStorm 是jetbrains公司旗 ...
- Web UI开发速速种草—Kendo UI for jQuery网格编辑操作概述
Kendo UI for jQuery最新试用版下载 Kendo UI目前最新提供Kendo UI for jQuery.Kendo UI for Angular.Kendo UI Support f ...
- Web 前端开发精华文章集锦(jQuery、HTML5、CSS3)【系列十八】
<Web 前端开发精华文章推荐>2013年第六期(总第十八期)和大家见面了.梦想天空博客关注 前端开发 技术,分享各种增强网站用户体验的 jQuery 插件,展示前沿的 HTML5 和 C ...
- Web 前端开发精华文章推荐(jQuery、HTML5、CSS3)【系列十二】
2012年12月12日,[<Web 前端开发人员和设计师必读文章>系列十二]和大家见面了.梦想天空博客关注 前端开发 技术,分享各种增强网站用户体验的 jQuery 插件,展示前沿的 HT ...
- Web 前端开发精华文章集锦(jQuery、HTML5、CSS3)【系列二十】
<Web 前端开发精华文章推荐>2013年第八期(总第二十期)和大家见面了.梦想天空博客关注 前端开发 技术,分享各种增强网站用户体验的 jQuery 插件,展示前沿的 HTML5 和 C ...
随机推荐
- CEC、ARC功能介绍
众所周知,HDMI作为一个数字化视频音频的接收标准,是可以同时传输视频和音频的,当然随着HDMI版本的提升,它的功能也一直在增强.事实上HDMI升级到1.3时,人们就发现了HDMI多了一个CEC功能. ...
- laydate年份选择,关闭底框,点击指定年份就选择然后关闭控件,翻页不选择也不关闭控件
如下图,翻页不选择也不关闭.点击指定年份时再选择和关闭控件 代码如下 // 默认没有选择,把判断赋值当前时间 var iYearCode = parseInt(new Date().getFullYe ...
- PAT甲级 散列题_C++题解
散列 PAT (Advanced Level) Practice 散列题 目录 <算法笔记> 重点摘要 1002 A+B for Polynomials (25) 1009 Product ...
- PHP和Memcached - Memcached的安装
1.现有扩展对比 memcache memcached 实现方式 原生 局域libmemcached的类库,性能高 编程方式 面向过程.对象 面向对象 CAS命令 NO YES php7 NO Y ...
- 【数据结构】P1165 日志分析
题目描述 MM 海运公司最近要对旗下仓库的货物进出情况进行统计.目前他们所拥有的唯一记录就是一个记录集装箱进出情况的日志.该日志记录了两类操作:第一类操作为集装箱入库操作,以及该次入库的集装箱重量:第 ...
- prometheus+grafana+Alertmanager邮箱告警
环境 系统:CentOS 7 软件:alertmanager-0.18.0.linux-amd64.tar.gz 安装 下载二进制包 地址:https://prometheus.io/download ...
- RMAN执行crosscheck archive报错ORA-19633问题处理
一.问题现象 RMAN connect target /; run { crosscheck archivelog all; } ORA-: control file record is out of ...
- springboot 整合 web 项目找不到 jsp 文件
今天遇到一个问题,就是使用springboot整合web项目的时候,怎么都访问不到 \webapp\WEB-INF\jsp\index.jsp 页面.这个问题搞了半天,试了各种方式.最后是因为在启动的 ...
- (十)easyUI之折叠面板+选项卡+树完成系统布局
一.效果 二 .编码 数据库设计 数据库函数设计,该函数根据父节点id 查询出所有字节点(包括孙子节点) BEGIN #声明两个临时变量 ); ); '; SET tempChd=CAST(rootI ...
- 【es6】将2个数组合并为一个数组
//第一种 一个数组中的值为key 一个数组中的值为value let arr1 = ['内存','颜色','尺寸']; let arr2 = [1,2,3]; let temp = arr1.map ...