react router @4 和 vue路由 详解(一)vue路由基础和使用
完整版:https://www.cnblogs.com/yangyangxxb/p/10066650.html
1、vue路由基础和使用
a、大概目录
我这里建了一个router文件夹,文件夹下有index.html
b、准备工作:
npm install vue-router
或者 yarn add vue-router
c、配置
必须要通过 Vue.use() 明确地安装路由功能:
在你的文件夹下的 src 文件夹下的 main.js 文件内写入以下代码
import Vue from 'vue' import VueRouter from 'vue-router' Vue.use(VueRouter)
附上我的代码:我是将router的内容写在了我的router文件夹下的index.html中,然后暴露出去,在main.js中引入
router文件夹下的index.html
import Vue from 'vue'
import VueRouter from 'vue-router'
Vue.use(VueRouter) import Home from 'pages/Home'
import Map from 'components/Map'
import Home1 from 'components/Home1'
import Find from 'components/Find'
import Mine from 'components/Mine'
import Type from 'components/Type'
import Publish from 'components/Publish'
import Search from 'components/Search'
import Success from 'components/Success'
import Need from 'components/Need'
import Position0 from 'components/Position'
import Like from 'components/scrollX/Like'
import S1 from 'components/scrollX/1'
import S2 from 'components/scrollX/2'
import Listall from 'components/mine/Listall'
import Listone from 'components/mine/Listone'
import Listchange from 'components/mine/Listchange' const routes = [
{
path:'/',
redirect:'/ho'
},
{
path: '/ho',
redirect:'/ho/home',
component: Home,
children: [
{
name: 'home',
path: 'home',
component: Home1,
redirect:'/ho/home/like',
children :[
{
name: 'like',
path: 'like',
component: Like
},
{
name: '2000001',
path: '2000001',
component: S1
},
{
name: '2000022',
path: '2000022',
component: S2
}
]
},
{
name: 'type',
path: 'type',
component: Type
},
{
name: 'need',
path: 'need',
component: Need
},
{
name: 'find',
path: 'find',
component: Find
},
{
name: 'mine',
path: 'mine',
component: Mine
}
]
},
{
name: 'search',
path: '/search',
component: Search
},
{
name: 'position',
path: '/position',
component: Position0
},
{
name: 'publish',
path: '/publish',
component: Publish
},
{
name: 'success',
path: '/success',
component: Success
},
{
name: 'listall',
path: '/listall',
component: Listall
},
{
name: 'listone',
path: '/listone',
component: Listone
},
{
name: 'listchange',
path: '/listchange',
component: Listchange
},
{
name: 'map',
path: '/map',
component: Map
}
] const router = new VueRouter({
mode: 'history',
routes
}) export default router

main.js

import Vue from 'vue'
import App from './App.vue'
import router from './router' Vue.use(MintUI)
Vue.use(ElementUI); Vue.config.productionTip = false new Vue({
router,
render: h => h(App)
}).$mount('#app')

d、常规使用
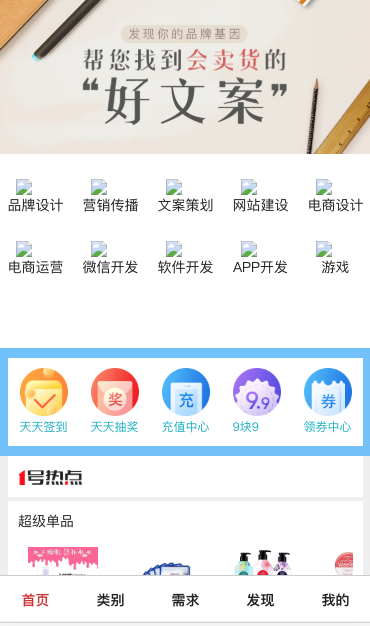

<template>
<div class="home">
<router-view></router-view>
<Ibar></Ibar>
</div>
</template>

那么在Ibar页面中如何切换路由呢?

<template>
<div class="ibar">
<router-link to="/ho/home" tag="span" active-class="active">首页</router-link>
<router-link to="/ho/type" tag="span" active-class="active">类别</router-link>
<router-link to="/ho/need" tag="span" active-class="active">需求</router-link>
<router-link to="/ho/find" tag="span" active-class="active">发现</router-link>
<router-link to="/ho/mine" tag="span" active-class="active">我的</router-link>
</div>
</template>

注意:此处的tag=“span”代表这个按钮是个span标签,你可以写样式的时候直接写span标签的样式即可
此处的active-class="active"代表点击哪个按钮哪个按钮高亮
此时我们详细看一下router文件夹下的index.js

//引入vue
import Vue from 'vue' //引入路由
import VueRouter from 'vue-router' //把路由挂载到vue上
Vue.use(VueRouter)
//引入我各个路由对应的component组件
import Home from 'pages/Home'
import Map from 'components/Map'
import Home1 from 'components/Home1'
import Find from 'components/Find'
import Mine from 'components/Mine'
import Type from 'components/Type'
import Publish from 'components/Publish'
import Search from 'components/Search'
import Success from 'components/Success'
import Need from 'components/Need'
import Position0 from 'components/Position'
import Like from 'components/scrollX/Like'
import S1 from 'components/scrollX/1'
import S2 from 'components/scrollX/2'
import Listall from 'components/mine/Listall'
import Listone from 'components/mine/Listone'
import Listchange from 'components/mine/Listchange'
const routes = [
{ //path是路由的路径
path:'/', //redirect代表重定向,因为当前路径'/'并没有对应的组件,所以需要重定向到其他路由页面
redirect:'/ho'
},
{
path: '/ho',
redirect:'/ho/home', //当不需要重定向的时候,需要component写上当前路由对应的组件页面
component: Home, //有些路由还有子路由,需要用到children[],
//当访问的时候,<router-link>的属性to的时候要把所有的父组件都带上
//如:此处的/ho/home/like
children: [
{
name: 'home',
path: 'home',
component: Home1,
redirect:'/ho/home/like',
children :[
{
name: 'like',
path: 'like',
component: Like
},
{
name: '2000001',
path: '2000001',
component: S1
},
{
name: '2000022',
path: '2000022',
component: S2
}
]
},
{
name: 'type',
path: 'type',
component: Type
},
{
name: 'need',
path: 'need',
component: Need
},
{
name: 'find',
path: 'find',
component: Find
},
{
name: 'mine',
path: 'mine',
component: Mine
}
]
},
{
name: 'search',
path: '/search',
component: Search
},
{
name: 'position',
path: '/position',
component: Position0
},
{
name: 'publish',
path: '/publish',
component: Publish
},
{
name: 'success',
path: '/success',
component: Success
},
{
name: 'listall',
path: '/listall',
component: Listall
},
{
name: 'listone',
path: '/listone',
component: Listone
},
{
name: 'listchange',
path: '/listchange',
component: Listchange
},
{
name: 'map',
path: '/map',
component: Map
}
] const router = new VueRouter({ //此处设置mode为history,即不带#号,在处理数据的时候会更方便一些
mode: 'history', //ES6的写法,即routes:routes的简写,当key和value名字一样时,可简写
routes
})
//把你创建的路由暴露出去,使得main.js可以将其引入并使用
export default router

引申1:
路由有一个meta属性
可以给该路由挂载一些信息
设置一些自己title、显示隐藏、左右滑动的方向之类的
meta: {
title: "HelloWorld", 要现实的title
show: true 设置导航隐藏显示
}
使用的时候:this.$route.meta.show
<Bottom v-show=
"this.$route.meta.show"
></Bottom>
引申2:
动态路由
{
path:"/two/:id",
component:Two,
}
获取数据this.$route.params.动态路由的名字
此处是:this.$route.params.id
引申3:
路由别名alias

{
path: '/a',
component: A,
alias: '/b'
}
// /a 的别名是 /b
//意味着,当用户访问 /b 时,URL 会保持为 /b,但是路由匹配则为 /a
//就像用户访问 /a 一样
//简单的说就是给 /a 起了一个外号叫做 /b ,但是本质上还是 /a

react router @4 和 vue路由 详解(一)vue路由基础和使用的更多相关文章
- react router @4 和 vue路由 详解(四)vue如何在路由里面定义一个子路由
完整版:https://www.cnblogs.com/yangyangxxb/p/10066650.html 6.vue如何在路由里面定义一个子路由? 给父路由加一个 children:[] 参考我 ...
- react router @4 和 vue路由 详解(八)vue路由守卫
完整版:https://www.cnblogs.com/yangyangxxb/p/10066650.html 13.vue路由守卫 a.beforeEach 全局守卫 (每个路由调用前都会触发,根据 ...
- react router @4 和 vue路由 详解(六)vue怎么通过路由传参?
完整版:https://www.cnblogs.com/yangyangxxb/p/10066650.html 8.vue怎么通过路由传参? a.通配符传参数 //在定义路由的时候 { path: ' ...
- angular路由详解六(路由守卫)
路由守卫 CanActivate: 处理导航到某个路由的情况. CanDeactivate:处理从当前路由离开的情况. Resole:在路由激活之前获取路由数据. 1.CanActivate: 处理导 ...
- angular路由详解三(路由参数传递)
我们经常用路由传递参数,路由主要有三种方式: 第一种:在查询参数中传递数据 {path:"address/:id"} => address/1 => Activa ...
- vue技术栈进阶(02.路由详解—基础)
路由详解(一)--基础: 1)router-link和router-view组件 2)路由配置 3)JS操作路由
- Vue 路由详解
Vue 路由详解 对于前端来说,其实浏览器配合超级连接就很好的实现了路由功能.但是对于单页面应用来说,浏览器和超级连接的跳转方式已经不能适用,所以各大框架纷纷给出了单页面应用的解决路由跳转的方案. V ...
- 每天记录一点:NetCore获得配置文件 appsettings.json vue-router页面传值及接收值 详解webpack + vue + node 打造单页面(入门篇) 30分钟手把手教你学webpack实战 vue.js+webpack模块管理及组件开发
每天记录一点:NetCore获得配置文件 appsettings.json 用NetCore做项目如果用EF ORM在网上有很多的配置连接字符串,读取以及使用方法 由于很多朋友用的其他ORM如S ...
- Ocelot简易教程(三)之主要特性及路由详解
作者:依乐祝 原文地址:https://www.cnblogs.com/yilezhu/p/9664977.html 上篇<Ocelot简易教程(二)之快速开始2>教大家如何快速跑起来一个 ...
随机推荐
- [osg]osgcallback各种回调使用的例子介绍
观察MyReadFileCallback结构体的内容,可以发现它继承自osgDB::Registry::ReadFileCallback,并重载了一个函数readNode,分析源代码可知,该函数在os ...
- 如何备份MySQL数据库
在MySQL中进行数据备份的方法有两种: 1. mysqlhotcopy 这个命令会在拷贝文件之前会把表锁住,并把数据同步到数据文件中,以避免拷贝到不完整的数据文件,是最安全快捷的备份方法. 命令的使 ...
- Basic Calculator 基本计算器
2018-09-27 22:02:36 一.Basic Calculator II 问题描述: 问题求解: sign用来保存前一个符号,用num来记录数字,如果碰到一个符号或者到达结尾,则需要进行入栈 ...
- English trip Spoken English & Word List(updating...)
Word List 词汇 Square 英 [skweə] 美 [skwɛr] adj. 平方的:正方形的:直角的:正直的. 使成方形:与…一致 vi. 一致:成方形 n. 平方:广场:正方形 ...
- Lab 3-2
Analyze the malware found in the file Lab03-02.dll using basic dynamic analysis tools. Questions and ...
- hdu-5985 概率DP
http://acm.hdu.edu.cn/showproblem.php?pid=5985 作为队里负责动态规划的同学,做不出来好无奈啊.思考了一个下午,最好还是参考了别人的思想才写出来,数学啊!! ...
- js,vue.js一些方法的总结
push() 可向数组的末尾添加一个或多个元素 pop() 删除并返回数组的最后一个元素 shift()删除并返回数组的第一个元素 unshift() 添加并返回数组的第一个元素 sort()对数组的 ...
- 小程序传id值
xml文件 <view class='bgcf bsbb pl30 pr30 pt30 pb30 df fww' > <block wx:for="{{intr ...
- 将本地 项目文件托管到 github
1.新建一个本地 repository文件夹 2.将想要 托管的项目或文件 复制到repository 文件夹下 2. 右键 git bash here 输入命令 git init 生成本地仓库 4. ...
- nginx-exporter安装使用
一.没有vts的启动方式 #nginx_exporter -telemetry.address=:9113 -nginx.scrape_uri="http://127.0.0.1:100 ...