Ultra-QuickSort
Description
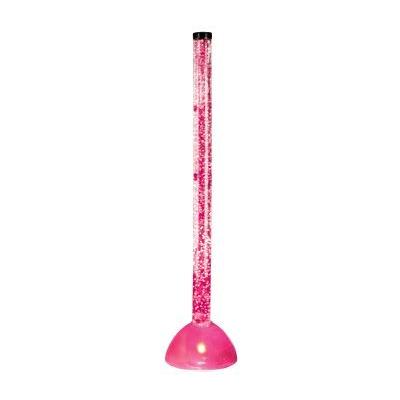
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
代码提交结果:compile error
困惑,不知道为什么
#include <stdio.h>
#include <stdlib.h>
void merging(int *list1,int list1_size,int *list2,int list2_size,int num,int *count)
{
int i,j,k,m;
i=j=k=0;
int temp[num],a,b;
while (i<list1_size && j<list2_size) {
a=list1[i];
b=list2[j];
if(list1[i]<list2[j])
{
temp[k++]=list1[i++];
}else{
temp[k++]=list2[j++];
(*count)=(*count)+list1_size-i;
}
}
while (i<list1_size) {
temp[k]=list1[i];
k++;
i++;
}
while (j<list2_size) {
temp[k]=list2[j];
k++;
j++;
}
for (m=0; m<list1_size+list2_size; m++) {
list1[m]=temp[m];
}
}
void sort(int *p,int num,int *count)
{
if (num>1) {
int *list1=p;
int list1_size=num/2;
int *list2=p+num/2;
int list2_size=num-list1_size;
sort(list1, list1_size,count);
sort(list2, list2_size,count);
merging(list1,list1_size,list2,list2_size,num,count);
}
}
int main() {
int num;
int *p;
int i;
int *count;
int result;
while (scanf("%d",&num)&&num!=0) {
result=0;
count=&result;
p=(int *)malloc(num*sizeof(int));
for (i=0; i<num; i++) {
scanf("%d",&p[i]);
}
sort(p,num,count);
printf("%d\n",(*count));
}
return 0;
}
0
Ultra-QuickSort的更多相关文章
- quickSort算法导论版实现
本文主要实践一下算法导论上的快排算法,活动活动. 伪代码图来源于 http://www.cnblogs.com/dongkuo/p/4827281.html // imp the quicksort ...
- Javascript算法系列之快速排序(Quicksort)
原文出自: http://www.nczonline.net/blog/2012/11/27/computer-science-in-javascript-quicksort/ https://gis ...
- JavaScript 快速排序(Quicksort)
"快速排序"的思想很简单,整个排序过程只需要三步: (1)在数据集之中,选择一个元素作为"基准"(pivot). (2)所有小于"基准"的元 ...
- QuickSort 快速排序 基于伪代码实现
本文原创,转载请注明地址 http://www.cnblogs.com/baokang/p/4737492.html 伪代码 quicksort(A, lo, hi) if lo < hi p ...
- quicksort
快排.... void quicksort(int *a,int left,int right){ if(left >= right){ return ; } int i = left; int ...
- 随手编程---快速排序(QuickSort)-Java实现
背景 快速排序,是在上世纪60年代,由美国人东尼·霍尔提出的一种排序方法.这种排序方式,在当时已经是非常快的一种排序了.因此在命名上,才将之称为"快速排序".这个算法是二十世纪的七 ...
- Teleport Ultra 下载网页修复
1 三个基本正则替换 tppabs="h[^"]*"/\*tpa=h[^"]*/javascript:if\(confirm\('h[^"]*[Ult ...
- Ultra Video Splitter & Converter
1. Video Splitter http://www.aone-soft.com/splitter.htm Ultra Video Splitter 是一款视频分割工具.可将一个巨大的AVI/Di ...
- 算法实例-C#-快速排序-QuickSort
算法实例 ##排序算法Sort## ### 快速排序QuickSort ### bing搜索结果 http://www.bing.com/knows/search?q=%E5%BF%AB%E9%80% ...
- mac 激活Ultra Edit16
一.文本编辑器UltraEdit 参照Ultra Edit16.10 Mac 破解下载,或者官方下载 Ultra Edit16即可 printf of=/Applications/UltraEdit. ...
随机推荐
- PHP中设置时区方法小结
找到原因后,在网上搜索到了一些关于PHP的时区设置方法: 1.修改php.ini,在php.ini中找到data.timezone =去掉它前面的;号,然后设置data.timezone = “Asi ...
- android 基本布局(RelativeLayout、TableLayout等)使用方法及各种属性
本文介绍 Android 界面开发中最基本的四种布局LinearLayout.RelativeLayout.FrameLayout.TableLayout 的使用方法及这四种布局中常用的属性. ...
- Hello BIEE
这篇文章提供了一个Hello World式的例子,讲述如何创建一个最简单的BIEE资料库.本文使用的示例数据可以在从此链接下载:http://www.zw1840.com . 目录 创建资料库 创 ...
- Parameter 'id' not found. Available parameters are [1, 0, param1, param2]异常
出现此类异常可能的原因:Mapper.xml文件中的parameterType的类型与传入的参数类型不匹配
- 4、解决native库不兼容
解决native库不兼容 现象: 报警告 [root@hadoop1 hadoop-]# bin/hdfs dfs -ls /input // :: WARN util.NativeCodeLoade ...
- SQL中JOIN 的用法
关于sql语句中的连接(join)关键字,是较为常用而又不太容易理解的关键字,下面这个例子给出了一个简单的解释 --建表table1,table2:create table table1(id int ...
- FFMPEG ./configure 参数及意义
FFMPEG版本:2.6.2,编译环境:ubuntu 14.4. 不同版本的FFMPEG参数可能不同,可在FFMPEG目录下使用以下命令查看 ./configure --help --help pri ...
- (转)yii流程,入口文件下的准备工作
yii流程 一 目录文件 |-framework 框架核心库 |--base 底层类库文件夹,包含CApplication(应用类,负责全局的用户请求处理,它管理的应用组件集, ...
- 随笔:近期仍在流行的QQ盗号网页简析
前言:被盗号的人们,你们的防护意识有那么弱吗? 声明:本文提到的技术,仅可用作网络安全加固等合法正当目的.本文作者无法鉴别判断读者阅读本文的真实目的,敬请读者在本国法律所允许范围内阅读本文,读者一旦因 ...
- Docker+OpenvSwitch搭建VxLAN实验环境
一.概述 1.环境:我这里是2台linux机器(host1和host2),发行版是kali2.0, ...