spring 使用@AspectJ注解开发Spring AOP
选择切点
代码清单:打印角色接口
package com.ssm.chapter11.aop.service; import com.ssm.chapter11.game.pojo.Role; public interface RoleService { // public void printRole(Role role); public void printRole(Role role, int sort); }
代码清单:RoleService实现类
package com.ssm.chapter11.aop.service.impl; import org.springframework.stereotype.Component;
import com.ssm.chapter11.aop.service.RoleService;
import com.ssm.chapter11.game.pojo.Role; @Component
public class RoleServiceImpl implements RoleService { // @Override
// public void printRole(Role role) {
// System.out.println("{id: " + role.getId() + ", " + "role_name : " + role.getRoleName() + ", " + "note : " + role.getNote() + "}");
// } public void printRole(Role role, int sort) {
System.out.println("{id: " + role.getId() + ", " + "role_name : " + role.getRoleName() + ", " + "note : " + role.getNote() + "}");
System.out.println(sort);
} }
这个类没什么特别的,只是这个时候如果把printRole作为AOP的切点,那么用动态代理的语言就是要为类RoleServi-ceImpl生成代理对象,然后拦截printRole方法,于是可以产生各种AOP通知方法。
创建切面
package com.ssm.chapter11.aop.aspect; import com.ssm.chapter11.aop.verifier.RoleVerifier;
import com.ssm.chapter11.aop.verifier.impl.RoleVerifierImpl;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.*; @Aspect
public class RoleAspect { @DeclareParents(value = "com.ssm.chapter11.aop.service.impl.RoleServiceImpl+", defaultImpl = RoleVerifierImpl.class)
public RoleVerifier roleVerifier; @Pointcut("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))")
public void print() {
} // @Before("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))")
// @Before("execution(* com.ssm.chapter11.*.*.*.*.printRole(..)) && within(com.ssm.chapter11.aop.service.impl.*)")
@Before("print()")
// @Before("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..)) && args(role, sort)")
public void before() {
System.out.println("before ....");
} @After("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))")
public void after() {
System.out.println("after ....");
} @AfterReturning("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))")
public void afterReturning() {
System.out.println("afterReturning ....");
} @AfterThrowing("execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))")
public void afterThrowing() {
System.out.println("afterThrowing ....");
} @Around("print()")
public void around(ProceedingJoinPoint jp) {
System.out.println("around before ....");
try {
jp.proceed();
} catch (Throwable e) {
e.printStackTrace();
}
System.out.println("around after ....");
} }
连接点
execution(* com.ssm.chapter11.aop.service.impl.RoleServiceImpl.printRole(..))
依次对这个表达式做出分析。
•execution:代表执行方法的时候会触发。
•*:代表任意返回类型的方法。
•com.ssm.chapter11.aop.service.impl.RoleServiceImpl:代表类的全限定名。
•printRole:被拦截方法名称。
•(..):任意的参数。
显然通过上面的描述,全限定名为com.ssm.chapter11.aop.service.impl.RoleServiceImpl的类的printRole方法被拦截了,这样它就按照AOP通知的规则把方法织入流程中。
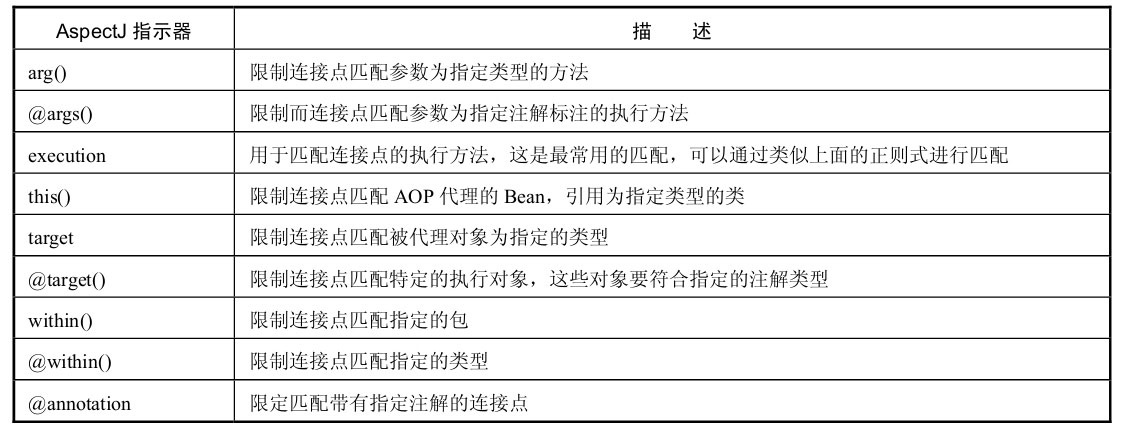
测试AOP
package com.ssm.chapter11.aop.config; import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.ComponentScan;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
import com.ssm.chapter11.aop.aspect.RoleAspect; @Configuration
@EnableAspectJAutoProxy
@ComponentScan("com.ssm.chapter11.aop")
public class AopConfig { @Bean
public RoleAspect getRoleAspect() {
return new RoleAspect();
} }
Spring还提供了XML的方式,这里就需要使用AOP的命名空间了
<?xml version='1.0' encoding='UTF-8' ?>
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context" xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-4.0.xsd
http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-4.0.xsd
http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-4.0.xsd"> <aop:aspectj-autoproxy/>
<bean id="roleAspect" class="com.ssm.chapter11.aop.aspect.RoleAspect"/>
<bean id="roleService" class="com.ssm.chapter11.aop.service.impl.RoleServiceImpl"/> </beans>
无论用XML还是用Java的配置,都能使Spring产生动态代理对象,从而组织切面,把各类通知织入到流程当中
代码清单:测试AOP流程
package com.ssm.chapter11.aop.main; import com.ssm.chapter11.aop.verifier.RoleVerifier;
import com.ssm.chapter11.aop.verifier.impl.RoleVerifierImpl;
import org.aspectj.lang.annotation.DeclareParents;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import org.springframework.context.annotation.AnnotationConfigApplicationContext;
import com.ssm.chapter11.aop.config.AopConfig;
import com.ssm.chapter11.aop.service.RoleService;
import com.ssm.chapter11.game.pojo.Role; public class Main { public static void main(String[] args) { ApplicationContext ctx = new AnnotationConfigApplicationContext(AopConfig.class);
// 使用XML使用ClassPathXmlApplicationContext作为IoC容器
// ApplicationContext ctx = new ClassPathXmlApplicationContext("ssm/chapter11/spring-cfg3.xml");
RoleService roleService = ctx.getBean(RoleService.class); Role role = new Role();
role.setId(1L);
role.setRoleName("role_name_1");
role.setNote("note_1"); RoleVerifier roleVerifier = (RoleVerifier) roleService;
if (roleVerifier.verify(role)) {
roleService.printRole(role, 1);
}
System.out.println("####################");
//测试异常通知
// role = null;
// roleService.printRole(role);
} }
spring 使用@AspectJ注解开发Spring AOP的更多相关文章
- Spring学习之旅(八)Spring 基于AspectJ注解配置的AOP编程工作原理初探
由小编的上篇博文可以一窥基于AspectJ注解配置的AOP编程实现. 本文一下未贴出的相关代码示例请关注小编的上篇博文<Spring学习之旅(七)基于XML配置与基于AspectJ注解配置的AO ...
- 使用@AspectJ注解开发Spring AOP
一.实体类: Role public class Role { private int id; private String roleName; private String note; @Overr ...
- Spring Aop(二)——基于Aspectj注解的Spring Aop简单实现
转发地址:https://www.iteye.com/blog/elim-2394762 2 基于Aspectj注解的Spring Aop简单实现 Spring Aop是基于Aop框架Aspectj实 ...
- Spring学习之旅(七)基于XML配置与基于AspectJ注解配置的AOP编程比较
本篇博文用一个稍复杂点的案例来对比一下基于XML配置与基于AspectJ注解配置的AOP编程的不同. 相关引入包等Spring AOP编程准备,请参考小编的其他博文,这里不再赘述. 案例要求: 写一 ...
- spring boot纯注解开发模板
简介 spring boot纯注解开发模板 创建项目 pom.xml导入所需依赖 点击查看源码 <dependencies> <dependency> <groupId& ...
- Spring:基于注解的Spring MVC
什么是Spring MVC Spring MVC框架是一个MVC框架,通过实现Model-View-Controller模式来很好地将数据.业务与展现进行分离.从这样一个角度来说,Spring MVC ...
- Spring自动装配----注解装配----Spring自带的@Autowired注解
Spring自动装配----注解装配----Spring自带的@Autowired注解 父类 package cn.ychx; public interface Person { public voi ...
- Spring使用AspectJ注解和XML配置实现AOP
本文演示的是Spring中使用AspectJ注解和XML配置两种方式实现AOP 下面是使用AspectJ注解实现AOP的Java Project首先是位于classpath下的applicationC ...
- Spring注解开发系列Ⅵ --- AOP&事务
注解开发 --- AOP AOP称为面向切面编程,在程序开发中主要用来解决一些系统层面上的问题,比如日志,事务,权限等待,Struts2的拦截器设计就是基于AOP的思想,横向重复,纵向抽取.详细的AO ...
随机推荐
- hbase实践之协处理器Coprocessor
HBase客户端查询存在的问题 Scan 用Get/Scan查询数据, Filter 用Filter查询特定数据 以上情况只适合几千行数据以及不是很多的列的"小数据". 当表扩展为 ...
- 配置jdk和环境变量
1.官网下载jdk1.8,默认安装即可 2.JAVE_HOME:jdk安装目录 path:C:;%JAVA_HOME%\bin; C:;%JAVA_HONE%\jre\bin;(当dos界面输入命令 ...
- select下拉选中显示对应的div隐藏不相关的div
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title> ...
- go内置的反向代理
package main import ( "log" "net/http" "net/http/httputil" "net/u ...
- django 学习第二天
今日内容 一.Django MVC和MTV框架 MVC controller:路由分发 用urls里面放置不同路径 执行不同函数 model 数据库信xi view #views 逻辑相关里面,写函数 ...
- 011——MATLAB清除工作控件变量
(一):参考文献:https://zhidao.baidu.com/question/234530287.html 清除当前工作空间全部变量:clear: 清除当前工作空间某些变量:clear 变量名 ...
- Kafka 通过python简单的生产消费实现
使用CentOS6.5.python3.6.kafkaScala 2.10 - kafka_2.10-0.8.2.2.tgz (asc, md5) 一.下载kafka 下载地址 https://ka ...
- Kafka 幂等生产者和事务生产者特性(讨论基于 kafka-python | confluent-kafka 客户端)
Kafka 提供了一个消息交付可靠性保障以及精确处理一次语义的实现.通常来说消息队列都提供多种消息语义保证 最多一次 (at most once): 消息可能会丢失,但绝不会被重复发送. 至少一次 ( ...
- fgets()函数
声明: char *fgets(char *str,int n,FILE* stream) 参数: str—这是指向一个字符数组的指针,该数组存储了要读取的字符串 n – 这是要读取的最大字符数(包括 ...
- const关键字与数组、指针
目录 const关键字 const修饰数组 const修饰指针 用两个const修饰指针 @ 开始回顾C基础知识.C中使用指针是很危险的事情,一个不慎就会造成程序崩溃,因此对于传入函数的参数进行保护就 ...