hdu3095-Eleven puzzle(双向搜索+哈希)
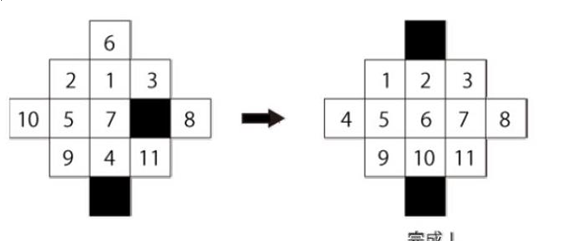
The tile in black means it’s empty
Each step you can move only one tile.
Here comes the problem.How many steps at least it required to done the game.
Input
Every case contains five lines to describe the initial status of the board. 0 means empty.
It’s confirmed that the board is legal.
Output
Sample Input
Sample Output
#include<cstdio>
#include<cstring>
#include<string>
#include<iostream>
#include<sstream>
#include<algorithm>
#include<utility>
#include<vector>
#include<set>
#include<map>
#include<queue>
#include<cmath>
#include<iterator>
#include<stack>
using namespace std;
const int INF=1e9+;
const double eps=1e-;
const int mod=;
const int maxn=;
int f[],r[]; //双向bfs的两个队列的队首队尾指针
int dx[]={-,,,},dy[]={,-,,}; //方向数组
bool in(int x,int y){ return x>=&&x<&&y>=&&y<; } //是否越界
struct node
{
int px[],py[]; //保存2个空格的位置和整个数组
int A[][];
}nod[][maxn]; //0是前面搜,1是后面搜
int B[][]={ //最终状态
-,-,,-,-,
-,,,,-,
,,,,,
-,,,,-,
-,-,,-,-
};
bool Same(int A[][]) //判断该状态是否与最终的状态相等
{
for(int i=;i<;i++)
for(int j=;j<;j++)
if(A[i][j]!=B[i][j]) return false;
return true;
}
int F[]; //保存2^i
void GetF()
{
F[]=;
for(int i=;i<;i++) F[i]=F[i-]*;
}
int Get(int A[][]) //得到哈希值
{
int ret=,k=;
for(int i=;i<;i++)
for(int j=;j<;j++) if(A[i][j]>) ret+=F[k++]*A[i][j]; //乘上一个权值
return ret;
}
struct Hash
{
int v,next,nid,k; //是哈希值,next指向下一个节点,nid和k分别保存是下标和0或1
}ha[mod+maxn];
int hash_id;
bool check(int a,int k1,int b,int k2) //判断是否完全相等
{
for(int i=;i<;i++)
for(int j=;j<;j++)
if(nod[k1][a].A[i][j]!=nod[k2][b].A[i][j]) return false;
return true;
}
int Insert_Hash(int v,int nid,int k) //插入
{
int a=v%mod;
int p=ha[a].next;
while(p!=-)
{
if(ha[p].v==v&&check(ha[p].nid,ha[p].k,nid,k)) return ha[p].k;//有相同的状态
p=ha[p].next;
}
p=++hash_id; //没有则增加新节点,前插法
ha[p].v=v; ha[p].nid=nid; ha[p].k=k;
ha[p].next=ha[a].next; ha[a].next=p;
return -; //-1代表插入了新节点
}
bool AddNode(node& t,int i,int j,int k)
{
int x=t.px[i],y=t.py[i];
int nx=x+dx[j],ny=y+dy[j];
if(!in(nx,ny)||t.A[nx][ny]<=) return false;
node& tt=nod[k][r[k]];
tt=t;
swap(tt.A[x][y],tt.A[nx][ny]); //交换
tt.px[i]=nx; tt.py[i]=ny;
int a=Insert_Hash(Get(tt.A),r[k],k);
if(a==-){ r[k]++; return false; } //队尾指加1
else if(a==k) return false; //自己原来访问过的状态
else return true; //相遇了,找到了解
}
void Print(node& t)
{
for(int i=;i<;i++)
{
for(int j=;j<;j++) printf("%d ",t.A[i][j]);
puts("");
}
puts("=========");
getchar();
}
bool bfs(int k)
{
int& be=f[k];
int en=r[k];
while(be<en)
{
node& t=nod[k][be++];
//Print(t);
for(int i=;i<;i++)
for(int j=;j<;j++) if(AddNode(t,i,j,k)) return true;
}
return false;
}
int solve()
{
if(Same(nod[][].A)) return ;
for(int i=;i<;i++)
for(int j=;j<;j++) nod[][].A[i][j]=B[i][j]; //最终状态
nod[][].px[]=; nod[][].py[]=;
nod[][].px[]=; nod[][].py[]=;
int step=;
f[]=f[]=,r[]=r[]=;
for(int i=;i<mod;i++) ha[i].next=-;
hash_id=mod-;
while(f[]<r[]||f[]<r[]) //双向搜
{
step++;
if(bfs()) return step;
step++;
if(bfs()) return step;
if(step>=) return -;
}
return -;
}
int main()
{
int T;
GetF();
scanf("%d",&T);
while(T--)
{
int k=;
for(int i=;i<;i++)
for(int j=;j<;j++)//得到初始状态
{
if(B[i][j]==-) { nod[][].A[i][j]=-; continue; }
scanf("%d",&nod[][].A[i][j]);
if(nod[][].A[i][j]==)
{ nod[][].px[k]=i; nod[][].py[k++]=j; }
}
int ans=solve();
if(ans==-) printf("No solution!\n");
else printf("%d\n",ans);
}
return ;
}
hdu3095-Eleven puzzle(双向搜索+哈希)的更多相关文章
- Eleven puzzle_hdu_3095(双向广搜).java
Eleven puzzle Time Limit: 20000/5000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) T ...
- Eight(bfs+全排列的哈希函数)
Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 22207 Accepted: 9846 Special Judge ...
- poj1200Crazy Search (哈希)
转载请注明出处: http://www.cnblogs.com/fraud/ ——by fraud Crazy Search Time Limit: 1000MS Memory ...
- POJ 1200:Crazy Search(哈希)
Crazy Search Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 32483 Accepted: 8947 Des ...
- POJ-3131-Cubic Eight-Puzzle(双向BFS+哈希)
Description Let's play a puzzle using eight cubes placed on a 3 × 3 board leaving one empty square. ...
- Crazy Search POJ - 1200 (字符串哈希hash)
Many people like to solve hard puzzles some of which may lead them to madness. One such puzzle could ...
- [PHP内核探索]PHP中的哈希表
在PHP内核中,其中一个很重要的数据结构就是HashTable.我们常用的数组,在内核中就是用HashTable来实现.那么,PHP的HashTable是怎么实现的呢?最近在看HashTable的数据 ...
- java单向加密算法小结(2)--MD5哈希算法
上一篇文章整理了Base64算法的相关知识,严格来说,Base64只能算是一种编码方式而非加密算法,这一篇要说的MD5,其实也不算是加密算法,而是一种哈希算法,即将目标文本转化为固定长度,不可逆的字符 ...
- Java 哈希表运用-LeetCode 1 Two Sum
Given an array of integers, find two numbers such that they add up to a specific target number. The ...
随机推荐
- 算法导论(第三版)Exercises4.2(第四章二节)
4.2-1(计算结果) 18 14 62 66 4.2-2(Strassen算法计算矩阵乘法) void multiplyMatrix(int a[], int b[], int n, int r ...
- 深入理解linux网络技术内幕读书笔记(一)--简介
Table of Contents 1 基本术语 1.1 本书常用的缩写 2 引用计数 2.1 引用计数函数 3 垃圾回收 3.1 异步 3.2 同步 4 函数指针 4.1 缺点 5 goto语句 5 ...
- Spring事务管理器分类
Spring并不直接管理事务,事实上,它是提供事务的多方选择.你能委托事务的职责给一个特定的平台实现,比如用JTA或者是别的持久机制.Spring的事务管理器可以用下表表示: 事务管理器的实例 目标 ...
- THREE.JS + Blender(obj、mtl加载代码)
2016-11-04 09:23:17 THREE.REVISION "81dev" Blender "2.78" 1.加载OBJ.MTL文件 // T ...
- Java IO教程 导读
Java IO是一套java 用来读写数据(输入和输出)的API.大部分程序都要处理一些输入,并有输入产生一些输出.Java为此提供了java.io包. 如果你浏览下java.io包,会对其中各样的类 ...
- 分享几个社交类网站常用并且也是最好用的jquery类库
官网都有详细的文档说明,大家自行百度谷歌哈! artZoom:常用于微博,支持图片放大缩小旋转 AutoComplete:自动完成 BackTop:当内容多时出现“返回顶部” CFUpload:批量上 ...
- 解决 Google 重定向,体验 Google 本味
想要体验原汁原味的 Google(google.com),下面的方案是我用过的较方便的方案. 欢迎更正及补充 Chrome 扩展 Chrone 商店有一款禁止重定向的扩展 NoCountryRedir ...
- (转)[老老实实学WCF] 第四篇 初探通信--ChannelFactory
第四篇 初探通信--ChannelFactory 通过前几篇的学习,我们简单了解了WCF的服务端-客户端模型,可以建立一个简单的WCF通信程序,并且可以把我们的服务寄宿在IIS中了.我们不禁感叹WCF ...
- Windows 不能在 本地计算机 启动 SQL Server(MSSQLSERVER)。错误码126
结合自己的解决方案和网络上搜到的内容,现总结如下: 首先你要知道问题出在了什么地方才能针对性处理. 1.打开事件查看器 计算机右击——管理 右侧会出现错误列表,在其中找到SQL server有关的查看 ...
- Jboss基础及简单的应用
初学Jboss,对于Jboss的基础认识以及配置做一些记录 Jboss基础: JBoss是什么–基于J2EE的应用服务器–开放源代码–JBoss核心服务不包括支持servlet/JSP的WEB容器,一 ...