poj1066 Treasure Hunt【计算几何】
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 8192 | Accepted: 3376 |
Description
An example is shown below:
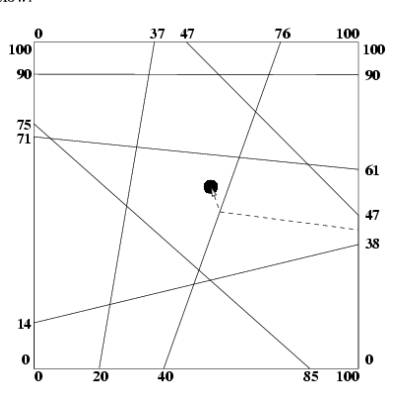
Input
Output
Sample Input
7
20 0 37 100
40 0 76 100
85 0 0 75
100 90 0 90
0 71 100 61
0 14 100 38
100 47 47 100
54.5 55.4
Sample Output
Number of doors = 2
Source
题意:
一个正方形中有n面墙,告诉你一个宝藏所在的坐标。问最少要砸穿多少墙才能到达宝藏。
思路:
枚举宝藏坐标和所有的墙的端点构成一个线段。判断这个线段和多少墙相交,因为这个就是从某一个点进入的最短距离,相交的个数就是穿墙数。
这个端点就是最开始进入的点。
相当于那些墙的端点把外围墙划分成了很多区域,我们从某一个点进去。两个端点之间任何一个位置进去都是一样的,所以枚举端点就行了。
找一个最小值就行了。要注意有可能是从角进去的。
刚开始inter函数写错了。
#include <iostream>
#include <set>
#include <cmath>
#include <stdio.h>
#include <cstring>
#include <algorithm>
#include <vector>
#include <queue>
#include <map>
//#include <bits/stdc++.h>
using namespace std;
typedef long long LL;
#define inf 0x7f7f7f7f const double eps = 1e-;
int sgn(double x)
{
if(fabs(x) < eps)return ;
if(x < )return -;
else return ;
} struct point{
double x, y;
point(){}
point(double _x, double _y)
{
x = _x;
y = _y;
}
point operator -(const point &b)const
{
return point(x - b.x, y - b.y);
}
point operator +(const point &b)const
{
return point(x + b.x, y + b.y);
}
point operator /(double d)const
{
return point(x / d, y / d);
}
double operator ^(const point &b)const
{
return x * b.y - y * b.x;
}
double operator *(const point &b)const
{
return x * b.x + y * b.y;
}
//绕原点旋转B
void transXY(double b)
{
double tx = x, ty = y;
x = tx * cos(b) - ty * sin(b);
y = tx * sin(b) + ty * cos(b);
}
};
struct line{
point s, e;
double k;
line(){}
line(point _s, point _e)
{
s = _s;
e = _e;
k = atan2(e.y - s.y, e.x - s.x);
}
pair<int, point>operator &(const line &b)const
{
point res = s;
if(sgn((s - e) ^ (b.s - b.e)) == ){
if(sgn((s - b.e) ^ (b.s - b.e)) == ){
return make_pair(, res);
}
else return make_pair(, res);
}
double t = ((s - b.s) ^ (b.s - b.e)) / ((s - e) ^ (b.s - b.e));
res.x += (e.x - s.x) * t;
res.y += (e.y - s.y) * t;
return make_pair(, res);
}
}; double dist(point a, point b)
{
return sqrt((a - b) * (a - b));
} bool inter(line l1,line l2)
{
return
max(l1.s.x,l1.e.x) >= min(l2.s.x,l2.e.x) &&
max(l2.s.x,l2.e.x) >= min(l1.s.x,l1.e.x) &&
max(l1.s.y,l1.e.y) >= min(l2.s.y,l2.e.y) &&
max(l2.s.y,l2.e.y) >= min(l1.s.y,l1.e.y) &&
sgn((l2.s-l1.s)^(l1.e-l1.s))*sgn((l2.e-l1.s)^(l1.e-l1.s)) <= &&
sgn((l1.s-l2.s)^(l2.e-l1.s))*sgn((l1.e-l2.s)^(l2.e-l2.s)) <= ;
} const int maxn = ;
line l[maxn];
point treasure, p[maxn];
int n; int main()
{
l[] = line(point(, ), point(, ));
l[] = line(point(,), point(, ));
l[] = line(point(, ), point(, ));
l[] = line(point(, ), point(, ));
while(scanf("%d", &n) != EOF){
for(int i = ; i <= n + ; i++){
scanf("%lf%lf%lf%lf", &l[i].s.x, &l[i].s.y, &l[i].e.x, &l[i].e.y);
//[2 * i - 1] = l[i].s;
//p[2 * i] = l[i].e;
}
scanf("%lf%lf", &treasure.x, &treasure.y); int ans = inf;
for(int i = ; i <= ; i++){
line l1 = line(treasure, l[i].s), l2 = line(treasure, l[i].e);
int cnt1 = , cnt2 = ;
for(int j = ; j <= n + ; j++){
if(inter(l1, l[j])){
cnt1++;
//cout<<cnt1<<endl;
}
if(inter(l2, l[j])){
cnt2++;
//cout<<cnt1<<endl;
}
}
ans = min(ans, cnt1 + );
ans = min(ans, cnt2 + );
}
for(int i = ; i <= n + ; i++){
line l1 = line(treasure, l[i].s), l2 = line(treasure, l[i].e);
int cnt1 = , cnt2 = ;
for(int j = ; j <= n + ; j++){
if(inter(l1, l[j])){
cnt1++;
}
if(inter(l2, l[j])){
cnt2++;
}
}
ans = min(ans, cnt1);
ans = min(ans, cnt2);
}
/*for(int i = 1; i <= n * 2; i++){
line l1 = line(treasure, p[i]);
int cnt = 0;
for(int j = 1; j <= n; j++){
if(inter(l1, l[j])){
cnt++;
}
}
ans = min(ans, cnt);
//cout<<ans<<endl;
}
line l1 = line(treasure, point(0, 0));
int cnt = 0;
for(int i = 1; i <= n; i++){
if(inter(l1, l[i])){
cnt++;
}
}
ans = min(ans, cnt + 1);
cnt = 0;
l1 = line(treasure, point(0, 100));
for(int i = 1; i <= n; i++){
if(inter(l1, l[i])){
cnt++;
}
}
ans = min(ans, cnt + 1);
cnt = 0;
l1 = line(treasure, point(100, 0));
for(int i = 1; i <= n; i++){
if(inter(l1, l[i])){
cnt++;
}
}
ans = min(ans, cnt + 1);
cnt = 0;
l1 = line(treasure, point(100, 100));
for(int i = 1; i <= n; i++){
if(inter(l1, l[i])){
cnt++;
}
}
ans = min(ans, cnt + 1);*/
printf("Number of doors = %d\n", ans);
}
return ;
}
poj1066 Treasure Hunt【计算几何】的更多相关文章
- POJ1066 Treasure Hunt
嘟嘟嘟 题意看题中的图就行:问你从给定的点出发最少需要穿过几条线段才能从正方形中出去(边界也算). 因为\(n\)很小,可以考虑比较暴力的做法.枚举在边界中的哪一个点离开的.也就是枚举四周的点\((x ...
- zoj Treasure Hunt IV
Treasure Hunt IV Time Limit: 2 Seconds Memory Limit: 65536 KB Alice is exploring the wonderland ...
- POJ 1066 Treasure Hunt(线段相交判断)
Treasure Hunt Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 4797 Accepted: 1998 Des ...
- ZOJ3629 Treasure Hunt IV(找到规律,按公式)
Treasure Hunt IV Time Limit: 2 Seconds Memory Limit: 65536 KB Alice is exploring the wonderland ...
- POJ 1066 Treasure Hunt(相交线段&&更改)
Treasure Hunt 大意:在一个矩形区域内.有n条线段,线段的端点是在矩形边上的,有一个特殊点,问从这个点到矩形边的最少经过的线段条数最少的书目,穿越仅仅能在中点穿越. 思路:须要巧妙的转换一 ...
- Treasure Hunt
Treasure Hunt time limit per test 1 second memory limit per test 256 megabytes input standard input ...
- zoj 3629 Treasure Hunt IV 打表找规律
H - Treasure Hunt IV Time Limit:2000MS Memory Limit:65536KB 64bit IO Format:%lld & %llu ...
- ZOJ 3626 Treasure Hunt I 树上DP
E - Treasure Hunt I Time Limit:2000MS Memory Limit:65536KB Description Akiba is a dangerous country ...
- 湖南大学ACM程序设计新生杯大赛(同步赛)I - Piglet treasure hunt Series 1
题目描述 Once there was a pig, which was very fond of treasure hunting. The treasure hunt is risky, and ...
随机推荐
- memcache和redis的区别
1.定义 Redis是一个开源的使用ANSI C语言编写.支持网络.可基于内存亦可持久化的日志型.Key-Value数据库,并提供多种语言的API Memcache是一个高性能的分布式的内存对象缓存系 ...
- C#------Aspose.cells使用方法
转载: http://www.cnblogs.com/muer/p/yaxle.html 代码: public ActionResult ImportData(HttpPostedFileBase f ...
- Bypass X-WAF SQL注入防御(多姿势)
0x00 前言 X-WAF是一款适用中.小企业的云WAF系统,让中.小企业也可以非常方便地拥有自己的免费云WAF. 本文从代码出发,一步步理解WAF的工作原理,多姿势进行WAF Bypass. ...
- Explaining Delegates in C# - Part 4 (Asynchronous Callback - Way 1)
So far, I have discussed about Callback, Multicast delegates, Events using delegates, and yet anothe ...
- Ajax 分析方法
我们如何查看到 Ajax 请求: 以 https://m.weibo.cn/u/2830678474 这个网页为例,按 F12,加载网页,然后选择资源类型为 XHR 的就可以看到 Ajax 请求了 我 ...
- C++中class与struct的区别(struct的类型名同时可以作为变量名)
通常我们知道的区别: (一)默认继承权限.如果不明确指定,来自class的继承按照private继承处理,来自struct的继承按照public继承处理: (二)成员的默认访问权限.class的成员默 ...
- 游戏服务器学习笔记 3———— firefly 的代码结构,逻辑
注:以下所有代码都是拿暗黑来举例,由于本人能力有限很多地方还没有看透彻,所以建议大家只是参考.有不对的地方非常欢迎指正. 一.结构 系统启动命令是,python statmaster.py,启 ...
- Sencha Touch 实战开发培训 视频教程 第二期 第二节
2014.4.9晚上8:00分开课. 本节课耗时接近1个半小时,需要一点耐心来观看. 本期培训一共八节,前两节免费,后面的课程需要付费才可以观看. 本节内容: 了解Container: 了解card布 ...
- IIS6配置后仍然无法解析json文件解决办法
两台服务器,都是Windows Server2003,照着以下办法设置后,一台可以访问到json文件,一台不可以. 1. MIME设置: 在IIS的站点属性的HTTP头设置里,选MIME 映射中点击” ...
- jconsole连接远程Tomcat应用
一.环境信息 远程tomcat:linux 64位 centos 7 上tomcat 8 本机:windows7 二.步骤 linux上,在tomcat安装目录的bin下,新建setenv.sh,内容 ...