hdu3567 八数码2(康托展开+多次bfs+预处理)
Eight II
Time Limit: 4000/2000 MS (Java/Others) Memory Limit: 130000/65536 K (Java/Others)
Total Submission(s): 4103 Accepted Submission(s): 878
In this game, you are given a 3 by 3 board and 8 tiles. The tiles are numbered from 1 to 8 and each covers a grid. As you see, there is a blank grid which can be represented as an 'X'. Tiles in grids having a common edge with the blank grid can be moved into that blank grid. This operation leads to an exchange of 'X' with one tile.
We use the symbol 'r' to represent exchanging 'X' with the tile on its right side, and 'l' for the left side, 'u' for the one above it, 'd' for the one below it.

A state of the board can be represented by a string S using the rule showed below.
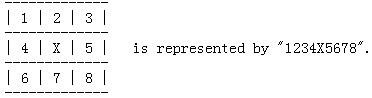
The problem is to operate an operation list of 'r', 'u', 'l', 'd' to turn the state of the board from state A to state B. You are required to find the result which meets the following constrains:
1. It is of minimum length among all possible solutions.
2. It is the lexicographically smallest one of all solutions of minimum length.
The input of each test case consists of two lines with state A occupying the first line and state B on the second line.
It is guaranteed that there is an available solution from state A to B.
The first line is in the format of "Case x: d", in which x is the case number counted from one, d is the minimum length of operation list you need to turn A to B.
S is the operation list meeting the constraints and it should be showed on the second line.
12X453786
12345678X
564178X23
7568X4123
dd
Case 2: 8
urrulldr
题目很好理解,就是个华容道的游戏,唯一要注意的就是要输出最短路径,长度相同时字典序要最小。
这道题和hdu1430方法一样,只不过1430是一次bfs+康托展开保存所有状态,而这个起点有九种,所以只要九次bfs就可以了,另外路径不能直接暴力保存,我是用了ans[x][e]来表示起点为x,走到康托值为e的操作字符,再用一个pre[x][e]来表示这个操作的前一个的康托值。另外这道题卡时间卡了一个减枝,就是如果前一步是u,那这一步就不需要d了,其他方向也一样,另外再赞一下这种把函数写在结构体里面的方法,真是太好用了!!
对了,还有由于每次输入的起点不一样,还有其他数字的位子也不一样,所以要用一个p来保存x的位置,(bfs过程中也要用p来表示x到哪里了,并且记得更新),然后用一个re[]数组来表示出了x以外的其他数字的对应关系,然后就可以了。
建议先做一下hdu1430,这是1430的题解。点击打开链接
上代码。
#include<iostream>
#include<algorithm>
#include<cstdlib>
#include<sstream>
#include<cstring>
#include<bitset>
#include<cstdio>
#include<string>
#include<deque>
#include<stack>
#include<cmath>
#include<queue>
#include<set>
#include<map>
using namespace std;
#define INF 0x3f3f3f3f
#define CLR(x,y) memset(x,y,sizeof(x))
#define LC(x) (x<<1)
#define RC(x) ((x<<1)+1)
#define MID(x,y) ((x+y)>>1)
typedef pair<int,int> pii;
typedef long long LL;
const double PI=acos(-1.0);
const int N=362880+10;
int fact[10]= {1,1,2,6,24,120,720,5040,40320,362880};
struct node {
char arr[10];
int val;
int p;
string step;
void contor() {
val=0;
for(int i=0; i<9; i++) {
int t=0;
for(int j=i+1; j<9; j++) {
if(arr[j]<arr[i])t++;
}
val+=t*fact[9-i-1];
}
val++;
}
void change_d(int x) {
char temp=arr[x];
arr[x]=arr[x+3];
arr[x+3]=temp;
p=x+3;
step+='d';
}
void change_l(int x) {
p=x-1;
char temp=arr[x];
arr[x]=arr[x-1];
arr[x-1]=temp;
step+='l';
}
void change_r(int x) {
p=x+1;
char temp=arr[x];
arr[x]=arr[x+1];
arr[x+1]=temp;
step+='r';
}
void change_u(int x) {
p=x-3;
char temp=arr[x];
arr[x]=arr[x-3];
arr[x-3]=temp;
step+='u';
}
node() {}
node(char *s) {
int i,j;
for(i = 0; i<strlen(s); i++) {
arr[i] = s[i];
}
}
};
int vis[N];
int pre[9][N];
char ans[9][N];
char re[10];
node s,t;
void bfs(int x) {
memset(pre[x],-1,sizeof(pre[x]));
memset(vis,0,sizeof(vis));
s.contor();
s.p=x;
queue<node>q;
vis[s.val]=1;
q.push(s);
node now,v;
while(!q.empty()) {
now=q.front();
q.pop();
v=now;
int e=v.val;
int len=v.step.length();
//d
if(v.p<6&&ans[x][e]!='u') {
v.change_d(v.p);
v.contor();
if(!vis[v.val]) {
vis[v.val]=1;
ans[x][v.val]='d';
pre[x][v.val]=e;
q.push(v);
}
}
//l
v=now;
if(v.p!=0&&v.p!=3&&v.p!=6&&ans[x][e]!='r') {
v.change_l(v.p);
v.contor();
if(!vis[v.val]) {
vis[v.val]=1;
ans[x][v.val]='l';
pre[x][v.val]=e;
q.push(v);
}
}
//r
v=now;
if(v.p!=2&&v.p!=5&&v.p!=8&&ans[x][e]!='l') {
v.change_r(v.p);
v.contor();
if(!vis[v.val]) {
vis[v.val]=1;
ans[x][v.val]='r';
pre[x][v.val]=e;
q.push(v);
}
}
//u
v=now;
if(v.p>2&&ans[x][e]!='d') {
v.change_u(v.p);
v.contor();
if(!vis[v.val]) {
vis[v.val]=1;
ans[x][v.val]='u';
pre[x][v.val]=e;
q.push(v);
}
}
}
}
int main() {
s = node("X12345678");
bfs(0);
s = node("1X2345678");
bfs(1);
s = node("12X345678");
bfs(2);
s = node("123X45678");
bfs(3);
s = node("1234X5678");
bfs(4);
s = node("12345X678");
bfs(5);
s = node("123456X78");
bfs(6);
s = node("1234567X8");
bfs(7);
s = node("12345678X");
bfs(8);
char ss[10];
int T;
cin>>T;
int cas=1;
while(T--) {
scanf("%s",ss);
int p;
for(int i=0,j=0; i<9; i++) {
if(ss[i]=='X'){
p=i;
}else{
re[ss[i]-'0']=++j; //记住 是j
}
}
scanf("%s",ss);
for(int i=0; i<9; i++) {
if(ss[i]!='X')
t.arr[i]=re[ss[i]-'0'];
else t.arr[i]='X';
}
t.contor();
int sum=t.val;
string aa;
aa="";
while(sum!=-1){
aa+=ans[p][sum];
sum=pre[p][sum];
}
printf("Case %d: %d\n",cas++,aa.size()-1);//为什么要-1呢?因为第一种状态是空字符,不是路径
for(int i=aa.size()-2;i>=0;i--)
printf("%c",aa[i]);
printf("\n");
}
}
hdu3567 八数码2(康托展开+多次bfs+预处理)的更多相关文章
- HDU 1043 Eight (BFS·八数码·康托展开)
题意 输出八数码问题从给定状态到12345678x的路径 用康托展开将排列相应为整数 即这个排列在全部排列中的字典序 然后就是基础的BFS了 #include <bits/stdc++.h ...
- hdu1043Eight (经典的八数码)(康托展开+BFS)
建议先学会用康托展开:http://blog.csdn.net/u010372095/article/details/9904497 Problem Description The 15-puzzle ...
- 八数码问题(一) 暴力BFS + STL
八数码问题是一个经典的人工智能问题.具体问题不累述了. 思路:由于存在多组测试数据,可以考虑“打表法“.所谓打表法,即枚举所有的初始情况,记录其到达终点的路径.而在这个题目中,顺序打表会调用很多次BF ...
- hdu3567 八数码(搜索)--预处理
题意:为你两个状态,求a到b 的最小路径,要求字典序最小. 思路: 最开始想的是目标状态是变化的,所以打表应该不行,然后直接上A*,但是TLE了- -(瞬间无语) 然后看了下别人的思路,预处理出9个状 ...
- Aizu0121 Seven Puzzle(bfs+康托展开)
https://vjudge.net/problem/Aizu-0121 比八数码要水的多,bfs. 但是做的时候我把康托展开记错了,wa了好几次. 附上康托展开博客详解:https://blog.c ...
- HDU1430;魔板(BFS+康托展开)
传送门 题意 给出初始序列与终止序列,给出三种操作,问最少经过几次操作能使初始->终止,输出操作(字典序最小) 分析 字符串只有8个字符,使用康托展开. 1.BFS将所有序列从"123 ...
- 双向广搜+hash+康托展开 codevs 1225 八数码难题
codevs 1225 八数码难题 时间限制: 1 s 空间限制: 128000 KB 题目等级 : 钻石 Diamond 题目描述 Description Yours和zero在研究A*启 ...
- hdu-1043(八数码+bfs打表+康托展开)
参考文章:https://www.cnblogs.com/Inkblots/p/4846948.html 康托展开:https://blog.csdn.net/wbin233/article/deta ...
- HDU1043 Eight(八数码:逆向BFS打表+康托展开)题解
Eight Time Limit: 10000/5000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total Sub ...
随机推荐
- C++知识点总结(6)
1.double和float的存储方式 float遵从的是IEEE R32.24 ,而double 遵从的是R64.53.无论是单精度还是双精度在存储中都分为三个部分: 符号位(Sign) : 0代表 ...
- 每天一道算法题(14)——N个降序数组,找到最大的K个数
题目: 假定有20个有序数组,每个数组有500个数字,降序排列,数字类型32位uint数值,现在需要取出这10000个数字中最大的500个. 思路 (1).建立大顶堆,维度为数组的个数,这里为20( ...
- C++用指针变量作为函数的参数接受数组的值的问题的总结
实参和形参的四种结合方式 实参 形参 实例 数组名 数组名 1.1 数组名 指针变量 1.2 指针变量 数组名 1.3 指针变量 指针变量 1.4 本文以输入10个整数,然后对其进行排序,然后输出的程 ...
- 写一个函数封装printf用作trace
转自http://blog.csdn.net/coder_weisong/article/details/10285291 写一个函数封装printf用作trace 方法一: #inc ...
- Entity Framework Tutorial Basics(9):Entity Relationships
这篇前面已经转载出来了,地址:http://www.cnblogs.com/purplefox2008/p/5646466.html
- 20169219《Linux内核原理与分析》第十一周作业
设备与模块 关于设备驱动和设备管理的四种内核成分: 设备类型:为了统一普通设备的操作所采用的分类. 模块:用于按需加载和卸载目标码的机制. 内核对象:内核数据结构中支持面向对象的简单操作,还支持维护对 ...
- Android简单的monkey测试
Android中的monkey测试是比较常用的工具了,设定好monkey之后,让手机跑一晚上,第二天分析日志,这样能更加有效率的工作. monkey测试的工具比较多,基本的方法都差不多. 抓取日志: ...
- 《Linux内核设计与实现》读书笔记(四)- 进程的调度
主要内容: 什么是调度 调度实现原理 Linux上调度实现的方法 调度相关的系统调用 1. 什么是调度 现在的操作系统都是多任务的,为了能让更多的任务能同时在系统上更好的运行,需要一个管理程序来管理计 ...
- JavaScript prototype原型链介绍
javascript 是一种基于原型的编程 (prototype based programming) 的语言, 而与我们通常的基于类编程 (class based programming) 有很大的 ...
- easyui textbox 设置只读不可编辑状态
在使用easyul的时候,发现输入框内容及不容易获取与设置,用jQuery的方式大部分失效.依稀记得好像是因为easyul会在原页面的基础上,生成了一些新的独有样式,并且暂时覆盖掉使用了easyul的 ...