Codeforces Round #533 C. Ayoub and Lost Array
题面:
题目描述:
题目分析:
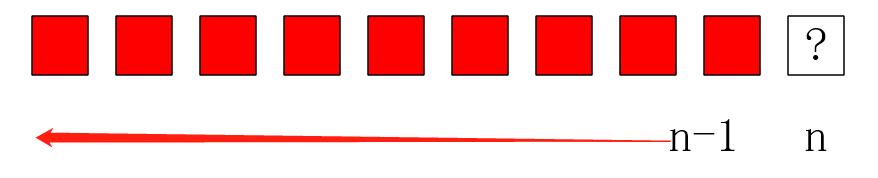
当p == 0时:dp[0][n] += dp[0][n-1] * (第n位选被3模后余数为0的数的个数)dp[0][n] += dp[1][n-1] * (第n位选被3模后余数为2的数的个数)dp[0][n] += dp[2][n-1] * (第n位选被3模后余数为1的数的个数)当p == 1时:dp[0][n] += dp[0][n-1] * (第n位选被3模后余数为1的数的个数)dp[0][n] += dp[1][n-1] * (第n位选被3模后余数为0的数的个数)dp[0][n] += dp[2][n-1] * (第n位选被3模后余数为2的数的个数)当p == 2时:dp[0][n] += dp[0][n-1] * (第n位选被3模后余数为2的数的个数)dp[0][n] += dp[1][n-1] * (第n位选被3模后余数为1的数的个数)dp[0][n] += dp[2][n-1] * (第n位选被3模后余数为0的数的个数)
1-x中,被3取模后余数为0的数的个数:f0(x) = x / 31-x中,被3取模后余数为1的数的个数:f1(x) = (x + 2) / 31-x中,被3取模后余数为2的数的个数:f2(x) = (x + 1) / 3
1 #include <cstdio>
2 #include <cstring>
3 #include <iostream>
4 #include <cmath>
5 #include <algorithm>
6 using namespace std;
7 const long long mod = 1e9+7;
8 const long long maxn = 2e5+5;
9 long long n, l, r;
10
11 long long dp[5][maxn];
12
13 const int yu[3][3] = {{0, 2, 1}, {1, 0, 2}, {2, 1, 0}};
14
15
16 int main(){
17 cin >> n >> l >> r;
18 int t;
19
20 //注意初始化
21 for(int i = 0; i < 3; i++){
22 t = (3-i)%3;
23 dp[i][1] = ( (r+t)/3-(l+t-1)/3 );
24 }
25
26
27 for(int i = 2; i <= n; i++){
28 for(int k = 0; k < 3; k++){
29 for(int p = 0; p < 3; p++){
30 t = (3-yu[k][p]) % 3;
31 dp[k][i] += dp[p][i-1]*( (r+t)/3-(l+t-1)/3 ) % mod;
32 dp[k][i] %= mod;
33 }
34 }
35 }
36
37 cout << dp[0][n] << endl;
38
39 return 0;
40 }
Codeforces Round #533 C. Ayoub and Lost Array的更多相关文章
- Codeforces Round #533 (Div. 2)题解
link orz olinr AK Codeforces Round #533 (Div. 2) 中文水平和英文水平都太渣..翻译不准确见谅 T1.给定n<=1000个整数,你需要钦定一个值t, ...
- Codeforces Round #533 (Div. 2) C. Ayoub and Lost Array 【dp】
传送门:http://codeforces.com/contest/1105/problem/C C. Ayoub and Lost Array time limit per test 1 secon ...
- Codeforces Round #533 (Div. 2) C.思维dp D. 多源BFS
题目链接:https://codeforces.com/contest/1105 C. Ayoub and Lost Array 题目大意:一个长度为n的数组,数组的元素都在[L,R]之间,并且数组全 ...
- Codeforces Round #533 (Div. 2) Solution
A. Salem and Sticks 签. #include <bits/stdc++.h> using namespace std; #define N 1010 int n, a[N ...
- Codeforces Round #533(Div. 2) C.Ayoub and Lost Array
链接:https://codeforces.com/contest/1105/problem/C 题意: 给n,l,r. 一个n长的数组每个位置可以填区间l-r的值. 有多少种填法,使得数组每个位置相 ...
- Codeforces Round #533 (Div. 2) C. Ayoub and Lost Array(递推)
题意: 长为 n,由 l ~ r 中的数组成,其和模 3 为 0 的数组数目. 思路: dp[ i ][ j ] 为长为 i,模 3 为 j 的数组数目. #include <bits/stdc ...
- Codeforces Round #258 (Div. 2) . Sort the Array 贪心
B. Sort the Array 题目连接: http://codeforces.com/contest/451/problem/B Description Being a programmer, ...
- Codeforces Round #555 (Div. 3) E. Minimum Array 【数据结构 + 贪心】
一 题面 E. Minimum Array 二 分析 注意前提条件:$0 \le a_{i} \lt n$ 并且 $0 \le b_{i} \lt n$.那么,我们可以在$a_{i}$中任取一个数 ...
- Codeforces Round #533 (Div. 2) B. Zuhair and Strings 【模拟】
传送门:http://codeforces.com/contest/1105/problem/B B. Zuhair and Strings time limit per test 1 second ...
随机推荐
- Verilog基础语法总结
去年小学期写的,push到博客上好了 Verilog 的基本声明类型 wire w1; // 线路类型 reg [-3:4] r1; // 八位寄存器 integer mem[0:2047]; // ...
- mysql(二)--mysql索引剖析
1.1. 索引是什么 1.1.1.索引图解 维基百科对数据库索引的定义: 数据库索引,是数据库管理系统(DBMS)中一个排序的数据结构,以协助快速查询.更新数据库表中数据. 怎么理解这个定义呢? 首 ...
- Adaptive Threshold
Adaptive Threshold 1. Otsu's Binarization: Using a discriminant analysis to partition the image into ...
- macOS utils
macOS utils dr.unarchiver https://dr-unarchiver.en.softonic.com/mac https://dr-unarchiver.en.softoni ...
- css ::selection 选择文本改变样式
.p1::selection{ background: red; color: #fff; }
- react 遍历 object
@observable obj = { name: "ajanuw", age: 22, }; @computed get list() { return _.toPairs(th ...
- Flutter: 下拉刷新,上拉加载更多
import 'package:flutter/material.dart'; import 'package:english_words/english_words.dart'; void main ...
- Python算法_三种斐波那契数列算法
斐波那契数列(Fibonacci sequence),又称黄金分割数列.因数学家列昂纳多·斐波那契(Leonardoda Fibonacci)以兔子繁殖为例子而引入,故又称为"兔子数列&qu ...
- Django Admin 实现三级联动的示例代码(省市区)===>小白级
一 使用环境 开发系统: windows IDE: pycharm 数据库: msyql,navicat 编程语言: python3.7 (Windows x86-64 executable in ...
- Django简单的使用及一些基础方法
目录 一.静态文件配置 1. 什么是静态文件 2. 静态文件的用法 3. 静态文件的动态绑定 二.请求方式与相应 1. get请求 2. post请求 3. Django后端视图函数处理请求 三.re ...