vueJs开发音乐播放器第二篇(点击歌单跳出详情页)
(1.使用router定义跳转链接,2. 使用axios得到音乐第三方数据,并渲染到页面上,3.组件之间传值(props))
1.接下来使用了vue-router路由动态传值,父子嵌套式路由
this.$router.push({
path: `/recommend/${item.id}`
});
2.router入口文件定义路由路径:
3. 此时需要渲染路由在父组件中:
B.使用vuex进行状态管理:
npm install vuex --save
import Vue from 'vue'
import Vuex from 'vuex'
Vue.use(Vuex)
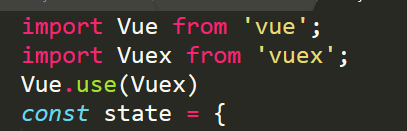
定义一个state,getters,mutations,方便组件中使用:
export 导出vuex定义模块
第二栏效果图:
主要代码:
<template>
<div >
<div class="wrapper" ref='wrapper'>
<div class="content">
<listview
title="推荐歌单"
:showPlayCount="true"
:showSinger="false"
:limit="6"
:resources="recommendList"
@select="selectedList"></listview>
<listview
title="最In音乐"
:showPlayCount="false"
:showSinger="true"
:limit="6"
:resources="newList"
@select="selectedList"></listview>
<listview
title="主播电台"
:showPayCount="false"
:showSinger="true"
:limit="6"
:resources="djrecommend"
@select="selectedList"></listview>
</div>
<router-view></router-view>
</div> </div>
</template>
<script>
import Listview from '@/components/listview/listview'
import {mapGetters, mapMutations} from 'vuex'
import store from "@/store/store.js";
export default{
name:"recommend",
store,
data(){
return {
list:null
} },
components:{
Listview, },
methods: {
//定义selectedList方法,router.push路由跳转
selectedList(item) {
this.$router.push({
path: `/recommend/${item.id}`
});
console.log(item);
this.setSongList(item);
},
...mapMutations([
'setSongList'
]) },
created(){
/*this.axios
.get('http://localhost:3000/top/playlist/highquality')
.then((response)=>{
this.list = response.data.playlists // console.log(this.list)
})
.catch((response)=>{
console.log(response)
})*/
this.recommendList = 'http://localhost:3000/top/playlist/highquality';
this.newList='http://localhost:3000/top/playlist?order=hot';
this.djrecommend='http://localhost:3000/personalized/djprogram';
} }
</script>
<style lang="scss" rel="style/scss"> </style>
歌单列表页
<template>
<transition name="fade">
<music-list :songs="songs" :id="id">
</music-list>
</transition>
</template> <script>
import {mapGetters} from 'vuex';
import musicList from '@/components/music-list/music-list' export default {
name: "list-detail",
data() {
return {
songs: {},
id: ''
}
},
created() {
this._getSongList();
},
methods: {
_getSongList() {
if (!this.songList || !this.songList.id) {
this.$router.push('/recommend');
return;
}
//使用vuex定义的数据得到父组件的相关值
this.songs = this.songList;
this.id = this.songList.id;
},
},
computed: {
...mapGetters([
'songList'
])
},
components: {
musicList
}, }
</script> <style lang="scss">
.fade-enter-active, .fade-leave-active {
transition: all 0.3s;
} .fade-enter, .fade-leave-to {
opacity: 0;
} </style>
子组件传值给歌曲列表页
<template>
<div class="music-list">
<div class="header">
<div class="header-img">
<img :src="songs.coverImgUrl">
<div class="play-count"><i class="icon iconfont icon-headset"></i>{{songs.playCount|unitConvert}}</div>
<div class="detail-btn"><i class="icon iconfont icon-detail"></i></div>
</div>
<div class="header-content">
<div class="name">{{songs.name}}</div>
<div class="tags" v-show="songs.tags">
<span>标签: </span><span class="tag" v-for="(tag,idx1) in songs.tags" :key="idx1">{{tag}}</span>
</div>
<div class="subname" v-if="songs.subscribers && songs.subscribers.length > 0">
{{songs.subscribers[0].nickname}}<span class="create-time">创建于{{songs.createTime}}</span>
</div>
</div>
<div class="back" @click="back">
<i class="icon iconfont icon-close"></i>
</div>
<div class="background">
<img :src="songs.coverImgUrl">
</div>
</div>
<div class="body">
<div class="header-bar">
<i class="icon iconfont icon-play"></i><span class="playAll">播放全部<i
class="count">(共{{detail.length}}首)</i></span>
<span class="collect"><i class="icon iconfont icon-add"></i>收藏({{subscribedCount|unitConvert}})</span>
</div>
<div class="songList-wrapper" ref="listWrapper">
<ul class="songlist">
<li class="song-item" v-for="(item, idx) in detail" :key="idx" @click="selectItem(item, idx, $event)">
<div class="line-number">
<span>{{idx+1}}</span>
</div>
<div class="item-content">
<div class="songname">{{item.name}}</div>
<div class="songer">{{item.ar[0].name}} - {{item.al.name}}</div>
</div>
<div class="tool">
<i class="icon iconfont icon-tool"></i>
</div>
</li>
</ul> </div> </div> </div>
</template> <script> import {unitConvert} from '@/common/js/unitConvert'
import {mapGetters, mapActions} from 'vuex' export default {
name: "music-list",
//得到list-detail传过来的id,并获取第三方数据,渲染到页面中
props: {
songs: {
type: Object
},
id: {
type: [String, Number]
}
},
data() {
return {
detail: [],
subscribedCount: ''
}
},
created() {
if (this.id) {
let url = `http://localhost:3000/playlist/detail?id=${this.id}`;
this.axios.get(url).then((res) => {
this.detail = res.data.playlist.tracks;
this.subscribedCount = res.data.playlist.subscribedCount; })
} },
methods: {
back(){ },
...mapActions([
'selectPlay'
])
},
filters: {
unitConvert(num) {
return unitConvert(num);
}
}
}
</script> <style lang="scss">
@function px2rem($px) {
@return $px / 30 + rem;
}
.music-list {
position: fixed;
z-index: 200;
top: 0;
bottom: 0;
left: 0;
right: 0;
background: #fff;
.header {
position: relative;
display: flex;
width: 100%;
height: px2rem(400);
align-items: center;
overflow: hidden;
background-color: rgba(0, 0, 0, 0.2);
.header-img {
display: flex;
position: relative;
justify-content: center;
flex: 0 0 px2rem(300);
width: px2rem(300);
height: px2rem(250);
img {
width: px2rem(250);
height: px2rem(250);
}
.play-count {
position: absolute;
top: 0;
right: px2rem(40);
color: #fff;
font-size: px2rem(24);
font-weight: bold;
.icon-headset {
color: #fff;
font-size: px2rem(24);
font-weight: bold;
margin-right: px2rem(8);
}
}
.detail-btn {
position: absolute;
bottom: 0;
right: px2rem(42);
color: #fff;
.icon-detail {
font-size: px2rem(36);
font-weight: bold; }
}
}
.header-content {
flex: 1;
height: px2rem(250);
padding-right: px2rem(25);
color: #fff;
.name {
margin: px2rem(20) 0;
height: px2rem(90);
font-size: px2rem(32);
font-weight: bold;
}
.tags {
margin-bottom: px2rem(24);
font-size: px2rem(26);
line-height: px2rem(26);
height: px2rem(26);
.tag {
padding: 0 px2rem(12);
border: 2px solid #fff;
border-radius: px2rem(8);
margin-left: px2rem(12);
}
}
.subname {
font-size: px2rem(26);
line-height: px2rem(26);
height: px2rem(26);
.create-time {
margin-left: px2rem(10);
} } }
.icon-close {
position: absolute;
top: 0;
right: px2rem(16);
padding: px2rem(20);
color: #fff;
font-size: px2rem(32);
font-weight: bold;
}
.background {
position: absolute;
top: 0;
left: 0;
width: 100%;
height: px2rem(400);
z-index: -1;
filter: blur(px2rem(100));
overflow: hidden; }
}
.body {
.header-bar {
display: flex;
line-height: px2rem(80);
height: px2rem(80);
border-bottom: 1px solid #eee;
justify-content: center;
text-align: center;
.icon-play {
width: px2rem(80);
font-size: px2rem(48);
}
.playAll {
flex: 1;
font-size: px2rem(26);
text-align: left;
.count {
font-size: px2rem(24);
font-style: normal;
color: #7e8c8d;
}
}
.collect {
padding: 0 px2rem(24);
background: #F93021;
color: #fff;
font-size: px2rem(26);
font-weight: bold;
text-align: center;
.icon-add {
font-size: px2rem(26);
color: #fff;
font-weight: bold;
}
}
}
.songList-wrapper {
position: absolute;
top: px2rem(480);
bottom: 0;
left: 0;
width: 100%;
overflow: hidden;
.songlist {
background: #fff;
.song-item {
display: flex;
&.list-complete-enter-active, &.list-complete-leave-active {
transition: all 0.2s linear;
}
&.list-complete-enter, &.list-complete-leave-to {
opacity: 0;
transform: translateY(px2rem(30));
}
.line-number {
flex: 0 0 px2rem(80);
width: px2rem(80);
height: px2rem(80);
line-height: px2rem(80);
text-align: center;
font-size: px2rem(30);
color: #7e8c8d; }
.item-content {
flex: 1;
border-bottom: 1px solid #eee;
width: 80%;
.songname {
font-size: px2rem(28);
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis; }
.songer {
font-size: px2rem(22);
color: #7e8c8d;
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis; } }
.tool {
flex: 0 0 px2rem(80);
width: px2rem(80);
line-height: px2rem(80);
border-bottom: 1px solid #eee;
text-align: center;
.icon-tool {
font-size: px2rem(30); }
} }
}
} }
} </style>
歌曲列表页
router的相关定义:
import Vue from 'vue'
import Router from 'vue-router'
import Helloworld from '@/components/HelloWorld'
import Recommend from '@/page/recommend'
import HotRecommend from '@/page/hotrecommend'
import Search from '@/page/search'
//引入子组件
import recommendList from '@/page/list-detail' Vue.use(Router) export default new Router({
routes: [
{
path: '/',
component: Helloworld
},
{
path: '/recommend',
component: Recommend,
//子组件路由
children: [
{
path: ':id',
component: recommendList
}
]
},
{
path: '/Hotrecommend',
component: HotRecommend
},
{
path: '/search',
component: Search
}
]
})
嵌套式路由实用
vuex-- store.js设定:
import Vue from 'vue';
import Vuex from 'vuex';
Vue.use(Vuex)
const state = {
// count:1,
songList:null
}
const getters = {
songList:function(state) {
return state.songList;
}
}
const mutations = {
/*increment(state){
state.count++
},
decrement(state){
state.count--
},*/
setSongList(state,item) {
state.songList=item;
}
}
const actions={
/*increment: ({commit})=>{
commit('increment')
},
decrement: ({commit})=>{
commit('decrement')
},*/
/* selectPlay: ({commit, state}, {list, index})=>{
commit('setPlayList',list)
},
*/
} export default new Vuex.Store({
state,
mutations,
getters,
actions
})
state,mutations,getters定义
vueJs开发音乐播放器第二篇(点击歌单跳出详情页)的更多相关文章
- Linux终端音乐播放器cmus攻略: 操作歌单
目录 1. 安装 2. 操作说明 2.1. *PlayList歌单 2.2. 其他 3. 视图切换 4. 使响应Media/play按键 4.1. 编译安装 cmus是一款开源的终端音乐播放器.它小巧 ...
- android开发音乐播放器--Genres和Art album的获取
最近在做一个项目,其中涉及到音乐播放器.当用到Genres和Art album时花费了一些时间才搞定,今天把方法草草列出,以供自己以后忘记时查看,也希望可以帮助碰到同样问题的道友!! 一.Genres ...
- python音乐播放器第二版
此代码是上一期的改版 需要用到的Python库有 .pygame 2.time 3.xmusic(我自己写的用来做音乐索引) .colorama(美观) 推荐使用pip安装 方法: pip ins ...
- 用Vue来实现音乐播放器(九):歌单数据接口分析
z这里如果我们和之前获取轮播图的数据一样来获取表单的数据 发现根本获取不到 原因是qq音乐在请求头里面加了authority和refer等 但是如果我们通过jsonp实现跨域来请求数据的话 是根本 ...
- iOS开发拓展篇—音频处理(音乐播放器3)
iOS开发拓展篇—音频处理(音乐播放器3) 说明:这篇文章主要介绍音频工具类和播放工具类的封装. 一.控制器间数据传递 1.两个控制器之间数据的传递 第一种方法:self.parentViewCont ...
- iOS开发拓展篇—音频处理(音乐播放器1)
iOS开发拓展篇—音频处理(音乐播放器1) 说明:该系列文章通过实现一个简单的音乐播放器来介绍音频处理的相关知识点,需要重点注意很多细节的处理. 一.调整项目的结构,导入必要的素材 调整后的项目结构如 ...
- iOS开发拓展篇—音频处理(音乐播放器2)
iOS开发拓展篇—音频处理(音乐播放器2) 说明:该文主要介绍音乐播放界面的搭建. 一.跳转 1.跳转到音乐播放界面的方法选择 (1)使用模态跳转(又分为手动的和自动的) (2)使用xib并设置跳转 ...
- iOS开发拓展篇—音频处理(音乐播放器4)
iOS开发拓展篇—音频处理(音乐播放器4) 说明:该文主要介绍音乐播放器实现过程中的一些细节控制. 实现的效果: 一.完整的代码 YYPlayingViewController.m文件 // // Y ...
- iOS开发拓展篇—音频处理(音乐播放器5)
iOS开发拓展篇—音频处理(音乐播放器5) 实现效果: 一.半透明滑块的设置 /** *拖动滑块 */ - (IBAction)panSlider:(UIPanGestureRecognizer *) ...
- iOS开发拓展篇—音频处理(音乐播放器6)
iOS开发拓展篇—音频处理(音乐播放器6) 一.图片处理 说明: Aspect表示按照原来的宽高比进行缩放. Aspectfit表示按照原来的宽高比缩放,要求看到全部图片,后果是不能完全覆盖窗口,会留 ...
随机推荐
- 鸿蒙HarmonyOS实战-ArkUI组件(Swiper)
一.Swiper 1.概述 Swiper可以实现手机.平板等移动端设备上的图片轮播效果,支持无缝轮播.自动播放.响应式布局等功能.Swiper轮播图具有使用简单.样式可定制.功能丰富.兼容性好等优点, ...
- #博弈论#HDU 1847 Good Luck in CET-4 Everybody!
题目 有\(n\)个石子,每次只能取2的自然数幂个, 取完石子的人获胜,问先手是否必胜 分析 如果不是3的倍数,那么取完一次一定能剩下3的倍数个, 反之亦然,那么3的倍数为必败状态 代码 #inclu ...
- OpenHarmony—内核对象事件之源码详解
近年来,国内开源实现跨越式发展,并成为企业提升创新能力.生产力.协作和透明度的关键.作为 OpenAtom OpenHarmony(以下简称"OpenHarmony")开源项目共建 ...
- Go 语言函数、参数和返回值详解
函数是一组语句,可以在程序中重复使用.函数不会在页面加载时自动执行.函数将通过调用函数来执行. 创建函数 要创建(通常称为声明)一个函数,请执行以下操作: 使用 func 关键字. 指定函数的名称,后 ...
- opengauss-jdbc问题整理
opengauss-jdbc问题整理(更新中) 问题 1 jdbc 批量执行 insert 语句时返回结果不符合 Spring jpa 预期 问题描述: jdbc 执行查询时,可以使用prepares ...
- MogDB/opengauss触发器简介(1)
MogDB/opengauss 触发器简介(1) 触发器是对应用动作的响应机制,当应用对一个对象发起 DML 操作时,就会产生一个触发事件(Event).如果该对象上拥有该事件对应的触发器,那么就会检 ...
- Centos 6.4 配置网页服务器
Centos 6.4 配置网页服务器 (2013-08-08 22:59:09) 转载▼ 分类:linux系统 今天值班,在单位找一台电脑安装了Centos 6.4操作系统. 一.安装软件 yum ...
- 实验k8s ————— k8s 搭建[一]
前言 以前学习k8s记录的.这里简单整理一下搭建,当时是我们学习环境的搭建,正式环境得专门的运维人员来,毕竟人家考虑的东西不一样. 正文 这里用kubeadm进行搭建,更加详细信息,在这里: http ...
- oracle 数据库连接
前言 关于oracle 数据库如何连接,我一开始以为和mysql 和 sql server一样,写好连接语句然后调用相应的dll. 知道我遇到了两个错误: 1.64位程序不能去驱动32位客户端 2.O ...
- VulnHub-Jangow-01-1.0.1打靶记录
知识点 NMAP参数 -sV 获取系统信息 -sT TCP扫描可能会留下日志记录 -sC 使用默认脚本(在-A模式下不需要) -p1-xxx 扫描端口号 -p- ==>等价于 -p1-65535 ...