HDU 2193 AVL Tree
AVL Tree
Definition of an AVL tree
An AVL tree is a binary search tree which has the following properties:
1. The sub-trees of every node differ in height by at most one.
2. Every sub-tree is an AVL tree.
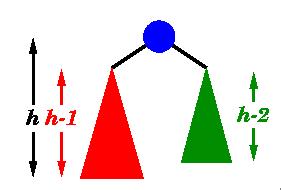
Balance requirement for an AVL tree: the left and right sub-trees differ by at most 1 in height.An AVL tree of n nodes can have different height.
For example, n = 7:
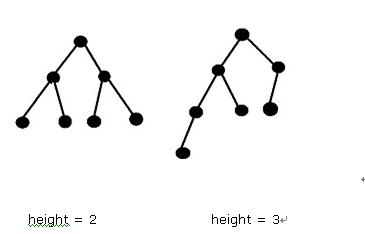
So the maximal height of the AVL Tree with 7 nodes is 3.
Given n,the number of vertices, you are to calculate the maximal hight of the AVL tree with n nodes.
Input
Input file contains multiple test cases. Each line of the input is an integer n(0<n<=10^9).
A line with a zero ends the input.
Output
An integer each line representing the maximal height of the AVL tree with n nodes.Sample Input
1
2
0
Sample Output
0
1
解题思路:
本题给出一个整数,要求输出其能建立的最高的平衡二叉树的高度。
关于平衡二叉树最小节点最大高度有一个公式,设height[i]为高度为i的平衡二叉树的最小结点数,则height[i] = height[i - 1] + height[i - 2] + 1;
因为高度为0时平衡二叉树:
#
高度为1时平衡二叉树:
0 # 或 #
/ \
1 # #
高度为2时平衡二叉树:
0 # 或 #
/ \ / \
1 # # # #
/ \
2 # #
高度为i时平衡二叉树:
# 或 #
/ \ / \
i - 2 i - 1 i - 1 i - 2
所以只需要将10^9内的数据记录后让输入的数据与之比较就可得到答案。(高度不会超过46)
#include <cstdio>
using namespace std;
const int maxn = ;
int height[maxn];
int main(){
height[] = ;
height[] = ;
for(int i = ; i < maxn; i++){ //记录1 - 50层最小需要多少节点
height[i] = height[i - ] + height[i - ] + ;
}
int n;
while(scanf("%d", &n) != EOF){ //输入数据
if(n == ) //如果为0结束程序
break;
int ans = -;
for(int i = ; i < maxn; i++){ //从第0层开始比较
if(n >= height[i]) //只要输入的数据大于等于该点的最小需求答案高度加一
ans++;
else
break; //否则结束循环
}
printf("%d\n", ans); //输出答案
}
return ;
}
HDU 2193 AVL Tree的更多相关文章
- HDU 5513 Efficient Tree
HDU 5513 Efficient Tree 题意 给一个\(N \times M(N \le 800, M \le 7)\)矩形. 已知每个点\((i-1, j)\)和\((i,j-1)\)连边的 ...
- 04-树5 Root of AVL Tree
平衡二叉树 LL RR LR RL 注意画图理解法 An AVL tree is a self-balancing binary search tree. In an AVL tree, the he ...
- 1066. Root of AVL Tree (25)
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child sub ...
- 1066. Root of AVL Tree
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child su ...
- 树的平衡 AVL Tree
本篇随笔主要从以下三个方面介绍树的平衡: 1):BST不平衡问题 2):BST 旋转 3):AVL Tree 一:BST不平衡问题的解析 之前有提过普通BST的一些一些缺点,例如BST的高度是介于lg ...
- AVL Tree Insertion
Overview AVL tree is a special binary search tree, by definition, any node, its left tree height and ...
- 1123. Is It a Complete AVL Tree (30)
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child sub ...
- A1123. Is It a Complete AVL Tree
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child sub ...
- A1066. Root of AVL Tree
An AVL tree is a self-balancing binary search tree. In an AVL tree, the heights of the two child sub ...
随机推荐
- Bug报告提交规范
首先声明,bug的测试规范应该在公司的正式文档建立.本建议非正式文档,有些内容可能不正确,有些内容可能需要继续商榷,甚至有些内容同公司规范有冲突.如果发现问题,直接忽略本文相应内容.本帖本意仅就工作中 ...
- 手动编译安装LAMP之httpd
安装前准备: 开发环境:Development Libraries 和 Development Tools httpd环境包:apr-1.4.6.tar.bz2 和 apr-util-1.4.1.ta ...
- ajaxfileupload插件上传图片功能,用MVC和aspx做后台各写了一个案例
HTML代码 和js 代码 @{ Layout = null; } <!DOCTYPE html> <html> <head> <meta name=&quo ...
- C# task和timer实现定时操作
C#中,定时器,或者叫作间隔器,每隔一段时间执行一个操作. 1.Timer本身就是多线程 C#中为不同场合下使用定时器,提供了不同的Timer类,在asp.net中一般使用System.Timers. ...
- VUE环境安装和创建项目
1.首先要安装nodejs和npm. 下载nodejs安装,下载地址:https://nodejs.org/en/ 安装很简单一路next即可. 安装完成后可以在cmd窗口输入node -v 和 np ...
- JS 面向对象详解
面向对象详解1 OO1.html <!DOCTYPE html> <html> <head> <meta charset="utf-8" ...
- WCF快速上手(二)
服务端是CS程序,客户端(调用者)是BS程序 一.代码结构: 二.服务接口Contract和实体类Domain INoticeService: using Domain; using System; ...
- 基于注解的Spring容器源码分析
从spring3.0版本引入注解容器类之后,Spring注解的使用就变得异常的广泛起来,到如今流行的SpringBoot中,几乎是全部使用了注解.Spring的常用注解有很多,有@Bean,@Comp ...
- P1642 规划
题目链接 题意分析 一看就知道是一道\(01\)分数规划的题 我们二分值之后 跑树形背包就可以了 CODE: #include<iostream> #include<cstdio&g ...
- TX2 安装v4l
在TX2上使用v4l2-ctl --all -d /dev/video0查看相机参数时报错: v4l2-ctl :command not found 手动安装: sudo apt-get instal ...