Unity3D学习笔记(八):四元素和书籍推荐
书籍推荐:
- 3D数学基础:图形与游戏开发——游戏软件开发专家系列(美)邓恩
- Unity Shader入门精要 冯乐乐(92年)
- 数据结构(Python语言描述)
- 数据结构、算法与应用(C++语言描述)
- 算法导论 第3版
- 黑客与画家
- 程序员生存之道
- 流体力学:八叉数空间划分
- using System.Collections;
- using System.Collections.Generic;
- using UnityEngine;
- public class RotateVector : MonoBehaviour {
- // Use this for initialization
- void Start () {
- Vector3 dir = new Vector3(,,);
- Debug.DrawLine(Vector3.zero,dir,Color.red,);
- //单位四元数表示无旋转
- Debug.Log(Quaternion.identity);
- //四元数的逆
- Quaternion q = Quaternion.AngleAxis(, Vector3.up);
- Quaternion tempQ = Quaternion.Inverse(q);
- Debug.Log(q);
- Debug.Log(tempQ);
- //旋转四元数
- Quaternion rotateQ = Quaternion.AngleAxis(, Vector3.up);
- Vector3 newVector = rotateQ * dir;
- Debug.DrawLine(Vector3.zero, newVector, Color.green, );
- //自己写一个RotationAround
- //RotateAround(Vector3 point, Vector3 axis, float angle);
- }
- // Update is called once per frame
- void Update () {
- }
- }
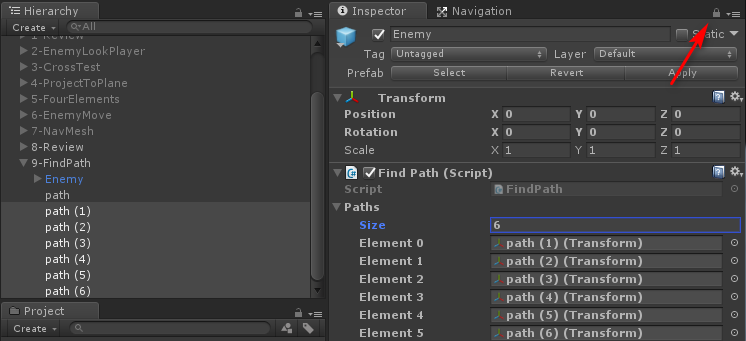
- using System.Collections;
- using System.Collections.Generic;
- using UnityEngine;
- public class RotateAroundScript : MonoBehaviour {
- public Transform sphere;
- public float rotateSpeed = ;
- // Use this for initialization
- void Start () {
- }
- // Update is called once per frame
- void Update () {
- //调用RotateAround
- RotateAround(sphere.position, Vector3.up, rotateSpeed);
- }
- public void myRotateAround(Vector3 point, Vector3 axis, float angle)
- {
- Vector3 A = transform.position - point;
- //Vector3 n = Vector3.Project();
- //Vector3.Dot(axis, A) = | n | * axis.magnitude;
- //| n | = Vector3.Dot(axis, A) / axis.magnitude;
- //Vector3 n = axis.normalized * | n |;
- Vector3 n = axis.normalized * Vector3.Dot(axis, A) / axis.magnitude;
- Vector3 a = A - n;
- Quaternion q = Quaternion.AngleAxis(angle, axis);
- Vector3 b = q * a;
- }
- }
复习
- using System.Collections;
- using System.Collections.Generic;
- using UnityEngine;
- public class w06d4 : MonoBehaviour {
- public Transform target;
- public Vector3 cubeSize;
- //欧拉角是如何表示旋转的
- //使用x y z来表示旋转 x表示绕x轴旋转多少度 y表示绕y轴旋转多少度 z表示绕z轴旋转多少度
- //四元数是如何表示旋转的
- //使用x y z w来表示旋转 通过轴角对来表示旋转 n轴转45度 x = n.x * sin<theta/2> , y = n.y * sin<theta/2>, z = n.z * sin<theta/2>, w = cos<theta/2>
- //欧拉角和四元数的优缺点
- //欧拉角
- // 优点: 直观,容易理解
- // 缺点: 有万向节锁
- // 只能绕固定轴旋转
- // 不能实现旋转的平滑差值
- //四元数
- // 优点: 可以实现任何角度的旋转
- // 可以实现旋转叠加
- // 没有万向节锁 、
- // 可以绕任意轴旋转
- // 可以实现旋转的平滑差值
- // 缺点: 不直观,不容易理解
- //API
- //使当前游戏物体的旋转变为 绕x轴旋转90
- //1)、使用欧拉角的形式赋值 //transform.eulerAngles = new Vector3(90, 0, 0);
- // 使用四元数的形式赋值 //transform.rotation = Quaternion.AngleAxis(90, Vector3.right);
- //2)、使当前游戏物体直接看向目标
- transform.LookAt(target);
- transform.rotation = Quaternion.LookRotation(target.position - transform.position);
- //3)、使当前游戏物体缓缓看向目标
- transform.rotation = Quaternion.Slerp(transform.rotation,
- Quaternion.LookRotation(target.position - transform.position),
- /Quaternion.Angle(transform.rotation, Quaternion.LookRotation(target.position - transform.position)));
- //4)、把一个欧拉角转换为四元数
- transform.rotation = Quaternion.Euler(, , );
- //5)、使用鼠标的水平轴值控制当前物体旋转(使用四元数叠加)
- float mouseX = Input.GetAxis("Mouse X");
- //自身的 绕Y轴转 XX 度
- transform.rotation *= Quaternion.Euler(, mouseX, );
- //世界的 绕Y轴转 XX 度
- transform.rotation = Quaternion.Euler(, mouseX, ) * transform.rotation;
- void Start () {
- transform.rotation = Quaternion.AngleAxis(, Vector3.right);
- transform.eulerAngles = new Vector3(, , );
- transform.LookAt(target);
- transform.rotation = Quaternion.LookRotation(target.position - transform.position);
- transform.rotation = Quaternion.Euler(, , );
- }
- void Update () {
- transform.rotation = Quaternion.Slerp(
- transform.rotation,
- Quaternion.LookRotation(target.position - transform.position),
- / Quaternion.Angle(transform.rotation, Quaternion.LookRotation(target.position - transform.position)));
- float mouseX = Input.GetAxis("Mouse X");
- // 自身的 绕Y轴转 XX 度
- transform.rotation *= Quaternion.Euler(, mouseX, );
- // 世界的 绕Y轴转 XX 度
- transform.rotation = Quaternion.Euler(, mouseX, ) * transform.rotation;
- }
- private void OnDrawGizmos()
- {
- Gizmos.color = new Color(, , , 0.2f);
- Gizmos.DrawSphere(transform.position, );
- }
- private void OnDrawGizmosSelected()
- {
- Gizmos.color = Color.red;
- Gizmos.DrawCube(transform.position, cubeSize);
- }
- }
Unity3D学习笔记(八):四元素和书籍推荐的更多相关文章
- Unity3D学习笔记(四)Unity的网络基础(C#)
一 网络下载可以使用WWW类下载资源用法:以下载图片为例WWW date = new WWW("<url>");yield return date;texture = ...
- [转]Unity3D学习笔记(四)天空、光晕和迷雾
原文地址:http://bbs.9ria.com/thread-186942-1-1.html 作者:江湖风云 六年前第一次接触<魔兽世界>的时候,被其绚丽的画面所折服,一个叫做贫瘠之地的 ...
- Unity3D学习笔记(四):物理系统碰撞和预制体
Rigidbody(刚体组件):加了此组件游戏物体就变成刚体了 ----Mass(质量,单位kg):重力G = 质量m * 重力加速度g(g=9.81 m/s^2) --------冲量守恒定理 动量 ...
- python3.4学习笔记(十四) 网络爬虫实例代码,抓取新浪爱彩双色球开奖数据实例
python3.4学习笔记(十四) 网络爬虫实例代码,抓取新浪爱彩双色球开奖数据实例 新浪爱彩双色球开奖数据URL:http://zst.aicai.com/ssq/openInfo/ 最终输出结果格 ...
- X-Cart 学习笔记(四)常见操作
目录 X-Cart 学习笔记(一)了解和安装X-Cart X-Cart 学习笔记(二)X-Cart框架1 X-Cart 学习笔记(三)X-Cart框架2 X-Cart 学习笔记(四)常见操作 五.常见 ...
- C++Primer第5版学习笔记(四)
C++Primer第5版学习笔记(四) 第六章的重难点内容 你可以点击这里回顾第四/五章的内容 第六章是和函数有关的知识,函数就是命名了的代码块,可以处理不同的情况,本章内 ...
- Go语言学习笔记八: 数组
Go语言学习笔记八: 数组 数组地球人都知道.所以只说说Go语言的特殊(奇葩)写法. 我一直在想一个人参与了两种语言的设计,但是最后两种语言的语法差异这么大.这是自己否定自己么,为什么不与之前统一一下 ...
- 【转】 Pro Android学习笔记(四十):Fragment(5):适应不同屏幕或排版
目录(?)[-] 设置横排和竖排的不同排版风格 改写代码 对于fragment,经常涉及不同屏幕尺寸和不同的排版风格.我们在基础小例子上做一下改动,在横排的时候,仍是现实左右两个fragment,在竖 ...
- 【opencv学习笔记八】创建TrackBar轨迹条
createTrackbar这个函数我们以后会经常用到,它创建一个可以调整数值的轨迹条,并将轨迹条附加到指定的窗口上,使用起来很方便.首先大家要记住,它往往会和一个回调函数配合起来使用.先看下他的函数 ...
随机推荐
- python count()
count() 描述 Python count() 方法用于统计字符串里某个字符出现的次数.可选参数为在字符串搜索的开始与结束位置. 语法 count()方法语法: str.count(sub, st ...
- 【Espruino】NO.07 获取电压值
http://blog.csdn.net/qwert1213131/article/details/27985645 本文属于个人理解,能力有限,纰漏在所难免.还望指正! [小鱼有点电] 前几节的内容 ...
- 文件上传 - iframe上传
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/ ...
- [js]ext.js探索
Ext JS 经常会遇到布局等头疼的问题,一直在用bootstrap,但是我不喜欢这玩意出的效果想找个合适的js架构入手 http://examples.sencha.com/extjs/6.6.0/ ...
- [django]JsonResponse序列化数据
def home(request): data = { 'name': 'maotai', 'age': 22 } import json return HttpResponse(json.dumps ...
- CentOS6.5 升级 Python 2.7 版本
转载请注明出处http://write.blog.csdn.net/mdeditor 目录 目录 前言 安装Python-279 解决YUM与Python279的兼容问题 前言 CentOS 6.5中 ...
- PHP自定义函数返回多个值
PHP自定义函数只允许用return语句返回一个值,当return执行以后,整个函数的运行就会终止. 有时要求函数返回多个值时,用return是不可以把值一个接一个地输出的. return语句可以返回 ...
- VB.net 与线程
Imports System.Threading Imports System Public Class Form1 Dim th1, th2 As Thread Public Sub Method1 ...
- shell应用技巧
Shell 应用技巧 Shell是一个命令解释器,是在内核之上和内核交互的一个层面. Shell有很多种,我们所使用的的带提示符的那种属于/bin/bash,几乎所有的linux系统缺省就是这种she ...
- python ( EOL while scanning string literal)
python错误: EOL while scanning string literal: 这个异常造成的原因是字符串,引号没有成对出现 参考:http://www.jb51.net/article/6 ...