POJ 1330 Nearest Common Ancestors(Tarjan离线LCA)
Description
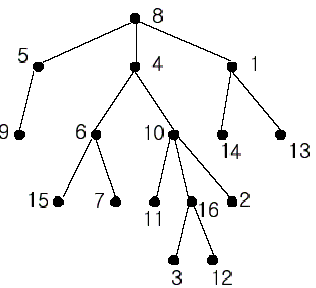
In the figure, each node is labeled with an integer from {1, 2,...,16}. Node 8 is the root of the tree. Node x is an ancestor of node y if node x is in the path between the root and node y. For example, node 4 is an ancestor of node 16. Node 10 is also an ancestor
of node 16. As a matter of fact, nodes 8, 4, 10, and 16 are the ancestors of node 16. Remember that a node is an ancestor of itself. Nodes 8, 4, 6, and 7 are the ancestors of node 7. A node x is called a common ancestor of two different nodes y and z if node
x is an ancestor of node y and an ancestor of node z. Thus, nodes 8 and 4 are the common ancestors of nodes 16 and 7. A node x is called the nearest common ancestor of nodes y and z if x is a common ancestor of y and z and nearest to y and z among their common
ancestors. Hence, the nearest common ancestor of nodes 16 and 7 is node 4. Node 4 is nearer to nodes 16 and 7 than node 8 is.
For other examples, the nearest common ancestor of nodes 2 and 3 is node 10, the nearest common ancestor of nodes 6 and 13 is node 8, and the nearest common ancestor of nodes 4 and 12 is node 4. In the last example, if y is an ancestor of z, then the nearest
common ancestor of y and z is y.
Write a program that finds the nearest common ancestor of two distinct nodes in a tree.
Input
N. Each of the next N -1 lines contains a pair of integers that represent an edge --the first integer is the parent node of the second integer. Note that a tree with N nodes has exactly N - 1 edges. The last line of each test case contains two distinct integers
whose nearest common ancestor is to be computed.
Output
Sample Input
2
16
1 14
8 5
10 16
5 9
4 6
8 4
4 10
1 13
6 15
10 11
6 7
10 2
16 3
8 1
16 12
16 7
5
2 3
3 4
3 1
1 5
3 5
Sample Output
4
3
#include<iostream>
#include<cstdio>
#include<cstring>
#include<algorithm>
#include<limits.h>
#include<vector>
typedef long long LL;
using namespace std;
#define REPF( i , a , b ) for ( int i = a ; i <= b ; ++ i )
#define REP( i , n ) for ( int i = 0 ; i < n ; ++ i )
#define CLEAR( a , x ) memset ( a , x , sizeof a )
const int maxn=10005;
int n,uu,vv;
vector<int>v[maxn];
int pre[maxn],vis[maxn];
bool root[maxn];
int find_root(int x)
{
if(pre[x]!=x)
x=find_root(pre[x]);
return pre[x];
}
void Union(int x,int y)
{
x=find_root(x);
y=find_root(y);
if(x!=y) pre[y]=x;
}
void LCA(int x)
{
for(int i=0;i<v[x].size();i++)
{
LCA(v[x][i]);
Union(x,v[x][i]);
}
vis[x]=1;
if(x==uu&&vis[vv]==1)
{
printf("%d\n",find_root(vv));
return ;
}
if(x==vv&&vis[uu]==1)
{
printf("%d\n",find_root(uu));
return ;
}
}
void init()
{
REP(i,maxn)
{
v[i].clear();
pre[i]=i;
root[i]=true;
vis[i]=0;
}
}
void solve()
{
REPF(i,1,n)
{
if(root[i]==true)
{
LCA(i);
break;
}
}
// for(int i=1;i<=n;i++)
// printf("222222 %d\n",pre[i]);
}
int main()
{
int t,a,b;
scanf("%d",&t);
while(t--)
{
scanf("%d",&n);
init();
REPF(i,1,n-1)
{
scanf("%d%d",&a,&b);
v[a].push_back(b);
root[b]=false;
}
scanf("%d%d",&uu,&vv);
solve();
}
return 0;
}
POJ 1330 Nearest Common Ancestors(Tarjan离线LCA)的更多相关文章
- POJ - 1330 Nearest Common Ancestors(基础LCA)
POJ - 1330 Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000KB 64bit IO Format: %l ...
- poj 1330 Nearest Common Ancestors 单次LCA/DFS
Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 19919 Accept ...
- POJ 1330 Nearest Common Ancestors(裸LCA)
Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 39596 Accept ...
- poj 1330 Nearest Common Ancestors 裸的LCA
#include<iostream> #include<cstdio> #include<cstring> #include<algorithm> #i ...
- POJ 1330 Nearest Common Ancestors / UVALive 2525 Nearest Common Ancestors (最近公共祖先LCA)
POJ 1330 Nearest Common Ancestors / UVALive 2525 Nearest Common Ancestors (最近公共祖先LCA) Description A ...
- POJ.1330 Nearest Common Ancestors (LCA 倍增)
POJ.1330 Nearest Common Ancestors (LCA 倍增) 题意分析 给出一棵树,树上有n个点(n-1)条边,n-1个父子的边的关系a-b.接下来给出xy,求出xy的lca节 ...
- LCA POJ 1330 Nearest Common Ancestors
POJ 1330 Nearest Common Ancestors Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 24209 ...
- POJ 1330 Nearest Common Ancestors(lca)
POJ 1330 Nearest Common Ancestors A rooted tree is a well-known data structure in computer science a ...
- POJ 1330 Nearest Common Ancestors 倍增算法的LCA
POJ 1330 Nearest Common Ancestors 题意:最近公共祖先的裸题 思路:LCA和ST我们已经很熟悉了,但是这里的f[i][j]却有相似却又不同的含义.f[i][j]表示i节 ...
随机推荐
- “快的打车”创始人陈伟星的新项目招人啦,高薪急招Java服务端/Android/Ios 客户端研发工程师/ mysql DBA/ app市场推广专家,欢迎大家加入我们的团队! - V2EX
"快的打车"创始人陈伟星的新项目招人啦,高薪急招Java服务端/Android/Ios 客户端研发工程师/ mysql DBA/ app市场推广专家,欢迎大家加入我们的团队! - ...
- [置顶] HMM Tutorial 隐马尔科夫模型
有一个月没有写博客了,这一个月系统的学习了HMM model. 上周周五做了个report 感觉还好. 所以把Slide贴上来.
- WinDbg分析DUMP文件
1. 如何生成dump文件? 原理:通过SetUnhandledExceptionFilter设置捕获dump的入口,然后通过MiniDumpWriteDump生成dump文件: ...
- BZOJ 1578: [Usaco2009 Feb]Stock Market 股票市场( 背包dp )
我们假设每天买完第二天就卖掉( 不卖出也可以看作是卖出后再买入 ), 这样就是变成了一个完全背包问题了, 股票价格为体积, 第二天的股票价格 - 今天股票价格为价值.... 然后就一天一天dp... ...
- 回归基础从新认识——HTML+CSS
前言 这段时间工作没那么繁杂,索性就想说来套系统的学习,之前去面试的时候,有被问及些基础的知识,居然回答不上来,也不能说是回答不上吧,回答的不全面.前端群上问了那个机构比较好,选择了慕课网.看了一段时 ...
- asp.net 生成、解析条形码和二维码
原文 asp.net 生成.解析条形码和二维码 一.条形码 一维码,俗称条形码,广泛的用于电子工业等行业.比如我们常见的书籍背面就会有条形码,通过扫描枪等设备扫描就可以获得书籍的ISBN(Intern ...
- [ASP.NET]以iTextSharp手绘表格并产生PDF下载
原文 [ASP.NET]以iTextSharp手繪表格並產生PDF下載 大家使用iTextSharp的機緣都不太一樣, 由於單位Crystal Report的License數量有限主管要我去找一個免費 ...
- 搭建Windows SVN服务器及TortoiseSVN使用帮助和下载
搭建Windows SVN服务器: 用的SVN服务器通常为外部,例如Google Code的服务器,不过,做为一个程序开发人员,就算自己一个人写程序,也应该有一个SVN版本控制系统,以便对开发代码进行 ...
- 正确处理Windows电源事件
简介为系统挂起与恢复而进行的应用准备步骤 曾几何时,当您正要通过应用提交或发布一些重要数据时,突然遇到一些急事需要处理,而且会耽误很长时间.当您完成任务回到电脑前时,发现电脑已经自动进入 了挂起状态, ...
- HDU 2159 二维费用背包问题
一个关于打怪升级的算法问题.. 题意:一个人在玩游戏老是要打怪升级,他愤怒了,现在,还差n经验升级,还有m的耐心度(为零就删游戏不玩了..),有m种怪,有一个最大的杀怪数s(杀超过m只也会删游戏的.. ...