【拓扑排序】【DFS】Painting A Board
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 3902 | Accepted: 1924 |
Description
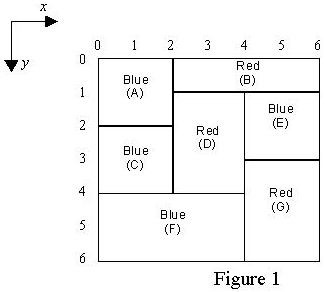
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
Input
Note that:
- Color-code is an integer in the range of 1 .. 20.
- Upper left corner of the board coordinates is always (0,0).
- Coordinates are in the range of 0 .. 99.
- N is in the range of 1..15.
Output
Sample Input
1
7
0 0 2 2 1
0 2 1 6 2
2 0 4 2 1
1 2 4 4 2
1 4 3 6 1
4 0 6 4 1
3 4 6 6 2
Sample Output
3
Source
#include<iostream>
#include<cstring>
#include<cstdio>
#include<algorithm>
using namespace std;
inline int read(){
int x=0,f=1;char c=getchar();
for(;!isdigit(c);c=getchar()) if(c=='-') f=-1;
for(;isdigit(c);c=getchar()) x=x*10+c-'0';
return x*f;
}
int T;
bool dis[41][41]; int x1[41],y1[41],x2[41],y2[41],col[41];
int N,res,ans=999999;
int tu[41];
bool vis[41];
int que[41]; void dfs(int st){
if(st==N) {
res=0;
for(int k=1;k<=N;k++) if(que[k]!=que[k-1]) res++;
ans=min(ans,res);
return ;
}
for(int i=1;i<=N;i++){
if(!tu[i]&&!vis[i]){
for(int j=1;j<=N;j++) if(dis[i][j]) tu[j]--;
vis[i]=true;que[st+1]=col[i];
dfs(st+1);
vis[i]=false;
for(int j=1;j<=N;j++) if(dis[i][j]) tu[j]++;
}
}
return ;
} int main(){
T=read();
while(T--){
ans=999999;
memset(tu,0,sizeof(tu));
memset(dis,false,sizeof(dis));
N=read();
for(int i=1;i<=N;i++){
x1[i]=read(),y1[i]=read();
x2[i]=read(),y2[i]=read();
col[i]=read();
}
for(int i=1;i<=N;i++){
for(int j=1;j<=N;j++)
if(i!=j&&x2[j]==x1[i]&&((y1[i]>=y1[j]&&y1[i]<=y2[j])||(y2[i]<=y2[j]&&y2[i]>=y1[j])||(y1[i]<=y1[j]&&y2[i]>=y2[j])||(y1[i]>=y1[j]&&y2[i]<=y2[j]))) dis[j][i]=true,tu[i]++;
}
dfs(0);
printf("%d\n",ans);
}
}
【拓扑排序】【DFS】Painting A Board的更多相关文章
- ACM/ICPC 之 拓扑排序+DFS(POJ1128(ZOJ1083)-POJ1270)
两道经典的同类型拓扑排序+DFS问题,第二题较第一题简单,其中的难点在于字典序输出+建立单向无环图,另外理解题意是最难的难点,没有之一... POJ1128(ZOJ1083)-Frame Stacki ...
- 拓扑排序+DFS(POJ1270)
[日后练手](非解题) 拓扑排序+DFS(POJ1270) #include<stdio.h> #include<iostream> #include<cstdio> ...
- 拓扑排序-DFS
拓扑排序的DFS算法 输入:一个有向图 输出:顶点的拓扑序列 具体流程: (1) 调用DFS算法计算每一个顶点v的遍历完成时间f[v] (2) 当一个顶点完成遍历时,将该顶点放到一个链表的最前面 (3 ...
- Ordering Tasks(拓扑排序+dfs)
Ordering Tasks John has n tasks to do. Unfortunately, the tasks are not independent and the executio ...
- HDU 5438 拓扑排序+DFS
Ponds Time Limit: 1500/1000 MS (Java/Others) Memory Limit: 131072/131072 K (Java/Others)Total Sub ...
- POJ1128 Frame Stacking(拓扑排序+dfs)题解
Description Consider the following 5 picture frames placed on an 9 x 8 array. ........ ........ ... ...
- poj1270Following Orders(拓扑排序+dfs回溯)
题目链接: 啊哈哈.点我点我 题意是: 第一列给出全部的字母数,第二列给出一些先后顺序. 然后按字典序最小的方式输出全部的可能性.. . 思路: 整体来说是拓扑排序.可是又非常多细节要考虑.首先要按字 ...
- Codeforces Round #292 (Div. 2) D. Drazil and Tiles [拓扑排序 dfs]
传送门 D. Drazil and Tiles time limit per test 2 seconds memory limit per test 256 megabytes Drazil cre ...
- 拓扑排序/DFS HDOJ 4324 Triangle LOVE
题目传送门 题意:判三角恋(三元环).如果A喜欢B,那么B一定不喜欢A,任意两人一定有关系连接 分析:正解应该是拓扑排序判环,如果有环,一定是三元环,证明. DFS:从任意一点开始搜索,搜索过的点标记 ...
- CodeForces-1217D (拓扑排序/dfs 判环)
题意 https://vjudge.net/problem/CodeForces-1217D 请给一个有向图着色,使得没有一个环只有一个颜色,您需要最小化使用颜色的数量. 思路 因为是有向图,每个环两 ...
随机推荐
- vue数组操作不触发前端重新渲染
暂时使用给数组先赋值 [ ] ,然后重新赋值的方式解决. 此外,能够监听的数组变异方法 https://cn.vuejs.org/v2/guide/list.html#%E5%8F%98%E5%BC% ...
- 关于might_sleep的一点说明【转】
转自:http://blog.csdn.net/chen_chuang_/article/details/48462575 这个函数我在看代码时基本上是直接忽略的(因为我知道它实际上不干什么事),不过 ...
- selenium===介绍
selenium 是支持java.python.ruby.php.C#.JavaScript . 从语言易学性来讲,首选ruby ,python 从语言应用广度来讲,首选java.C#.php. 从语 ...
- android 与JS之间的交互
在页面布局很复杂并且是动态的时候,android本身的控件就变得不是那么地灵活了,只有借助于网页的强大布局能力才能实现,但是在操作html页面的同时也需要与android其它的组件存在交互,比如说 在 ...
- [How to]Cloudera manager 离线安装手册
2016-01-1910:54:05 增加kafka 1.简介 本文介绍在离线环境下安装Cloudera manager和简单使用方法 2.环境 OS:CentOS 6.7 Cloudera man ...
- Unknown character set: 'utf8mb4'
出现Unknown character set: 'utf8mb4'该错误是因为你的mysql-connector-java版本太高了,现在的mysql编码方式utf8mb4 然而老版本的却是utf ...
- 尽量用const,enum,inline代替define
在读<Effective C++>之前,我确实不知道const,enum,inline会和define扯上什么关系,看完感觉收获很大,记录之. define: 宏定义. 在编译预处理时,对 ...
- 使用cmd(黑窗口)敲命令使用远程数据库
C:\Users\gzz>mysql -h 10.27.104.176 -u root -p mysql
- c语言中数组,指针数组,数组指针,二维数组指针
1.数组和指针 ] = {,,,,};// 定义数组 // 1. 指针和数组的关系 int * pa = array; pa = array; // p[0] == *(p+0) == array[0 ...
- 怎么制作CHM格式电子书
CHM格式的帮助文件相信大家都不陌生,CHM文件形式多样,使用方便,深受大家喜爱. 今天给大家介绍一种把文本文件转换为CHM格式电子书的方法. 前期准备过程 1 下载QuickCHM v2.6文件 去 ...