Google Maps API的使用
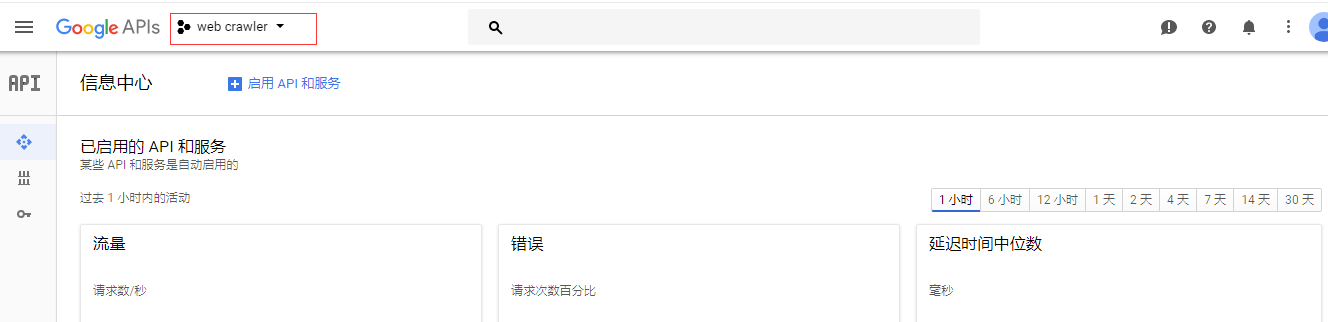

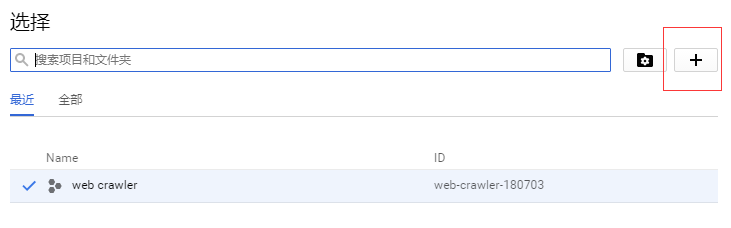
.png)
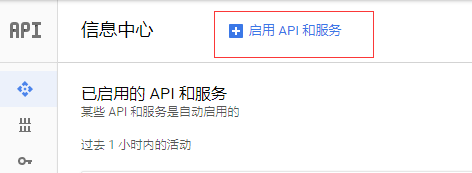
.png)
.png)
.png)
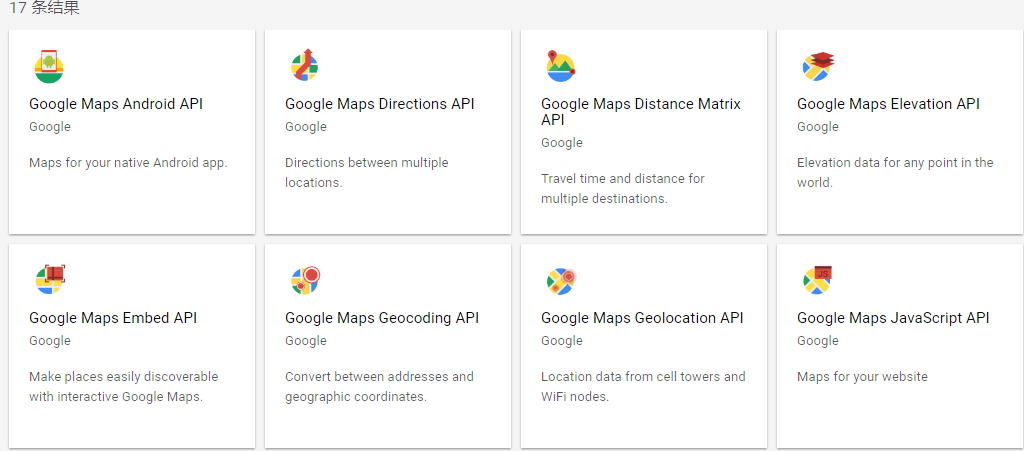
.png)
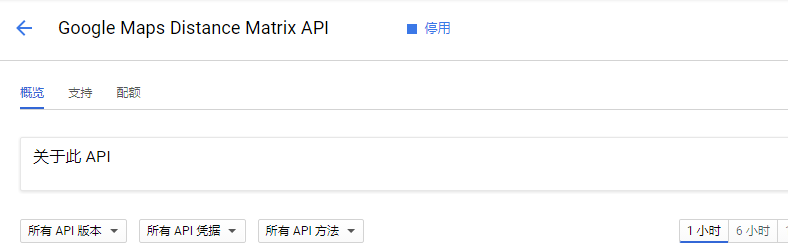
.png)
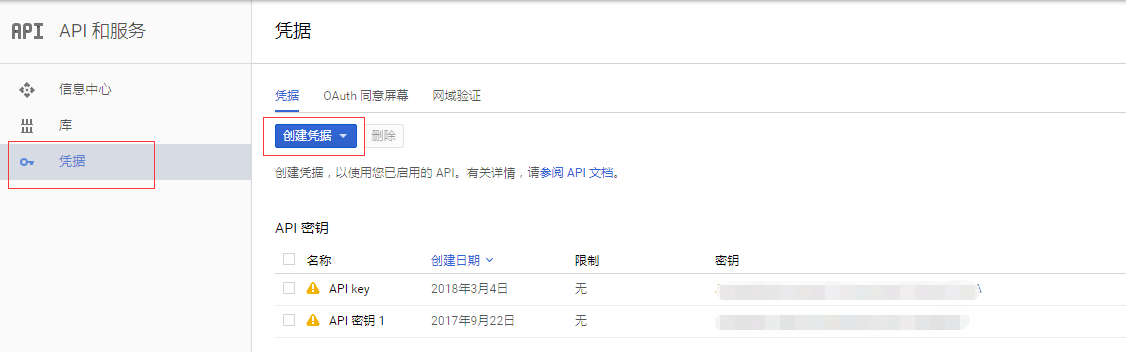
.png)
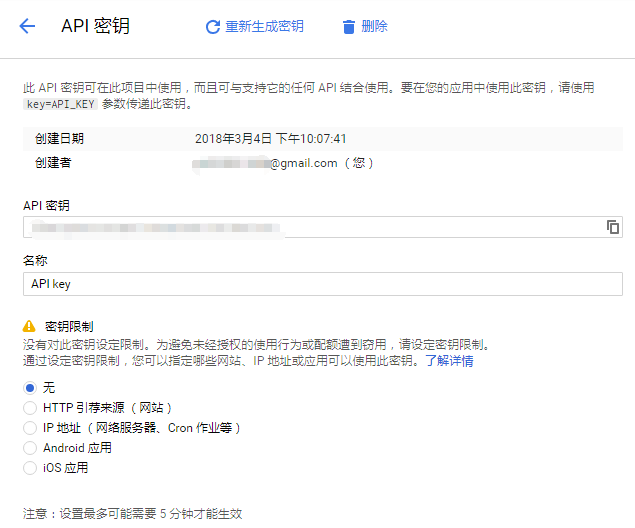
import googlemaps
from datetime import datetime gmaps = googlemaps.Client(key='Add Your Key here') # Geocoding an address
geocode_result = gmaps.geocode('1600 Amphitheatre Parkway, Mountain View, CA')
print(geocode_result[0]['geometry']['location']) # Look up an address with reverse geocoding
reverse_geocode_result = gmaps.reverse_geocode((40.714224, -73.961452))
print(reverse_geocode_result[0]['address_components'][1]['long_name'])
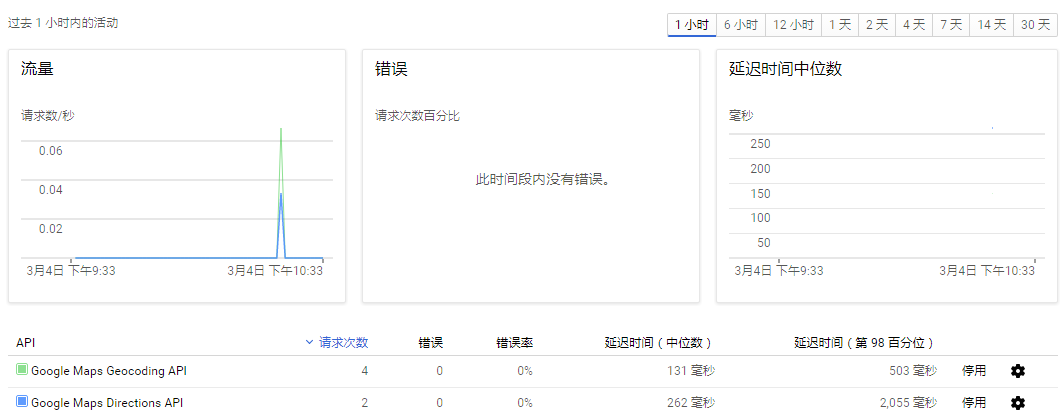
.png)
import googlemaps
from datetime import datetime gmaps = googlemaps.Client(key='Add Your Key here')
# Request directions via public transit
now = datetime.now()
directions_result = gmaps.directions("Sydney Town Hall",
"Parramatta, NSW",
mode="transit",
departure_time=now)
print(directions_result)
import googlemaps
from datetime import datetime
import os
import csv
import pandas as pd
import matplotlib.pyplot as plt
import math # 将已知的多个txt文件中的内容放到一个CSV文件下
def txt2Csv(dataPath, csvname):
fileList = os.listdir(dataPath)
csvFile = open(dataPath + '\\' + csvname, 'w+')
writer = csv.writer(csvFile)
for fileName in fileList:
with open(dataPath + '\\' + fileName) as fileObj:
lines = fileObj.readlines()
for line in lines:
line = line.split(',')
line[-1] = line[-1][0:-1]
writer.writerow((line))
csvFile.close() # 根据经纬度获取两地之间的距离及花费的时间
def getDistanceDuration(key, path, csvName):
gmaps = googlemaps.Client(key=key)
df = pd.read_csv(path + '\\' + csvName)
df.columns = ['id', 'time', 'longitude', 'latitude']
durationList = []
distanceList = []
try:
for i in range(1, 1000):
now = datetime.now()
# 调取google API的directions:
directions_result = gmaps.directions((df.iloc[i, 3], df.iloc[i, 2]),
(df.iloc[i+1, 3], df.iloc[i+1, 2]),
mode="driving",
departure_time=now)
# 按照返回的格式,找出distance及duration,追加到列表中并返回
distanceList.append(directions_result[0]['legs'][0]['distance']['value'])
durationList.append(directions_result[0]['legs'][0]['duration']['value'])
except googlemaps.exceptions._RetriableRequest:
pass
return distanceList, durationList path = 'D:\\Learnning\\python\\scrape\\taxiData\\T-drive Taxi Trajectories\\release\\taxi_log_2008_by_id'
txt2Csv(path, 'geodata.csv') distanceList, durationList = getDistanceDuration('AIzaSyD8X6tJx6Ap5TVHlqwSso8iTwZfDWcFsOA', path, 'geodata.csv')
# 对返回数据的单位做转换, 并使用math.ceil对数据向上取整
distanceList = [math.ceil(dis/1000) for dis in distanceList]
durationList = [math.ceil(dis/60) for dis in durationList] totalDistance = 0
totalDuration = 0
# 计算总路程,并画出每段路程的距离在总路程中的占比:
for distance in distanceList:
totalDistance += distance
distancePropo = [distance/totalDistance for distance in distanceList]
plt.bar(distanceList, distancePropo)
plt.title("Distance interval")
plt.xlabel("Km")
plt.ylabel("Proportion")
plt.show() # 计算总时间,并画出每段路程花费的时间在总时间中的占比:
for duration in durationList:
totalDuration += duration
durationPropo = [duration/totalDuration for duration in durationList]
plt.bar(durationList, durationPropo)
plt.title("Time interval")
plt.xlabel("Min")
plt.ylabel("Proportion")
plt.show()
.png)
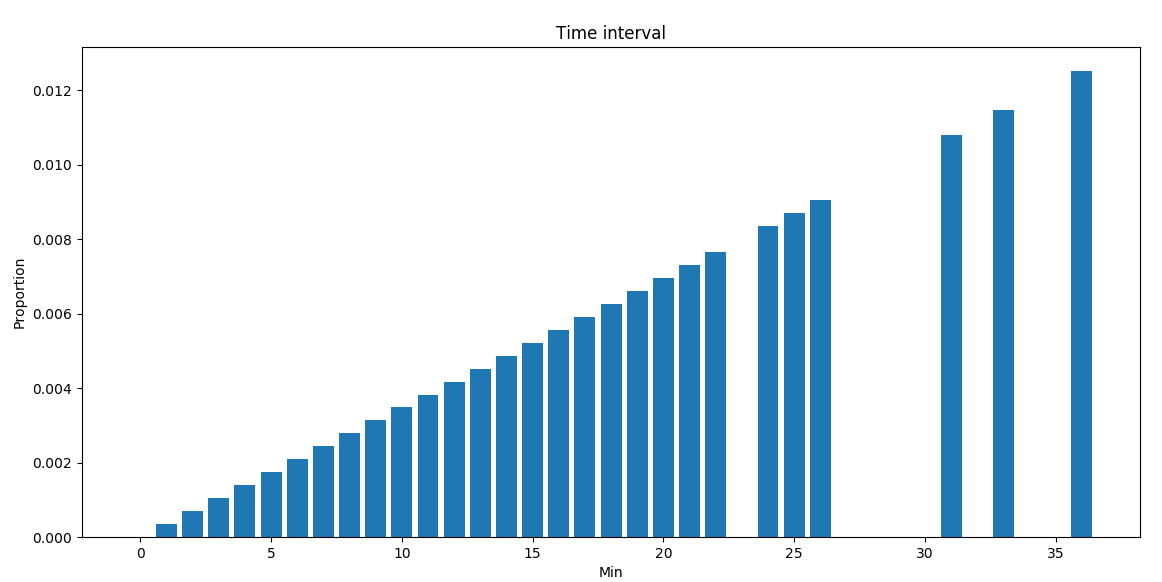
按行驶距离分布:
.png)
Google Maps API的使用的更多相关文章
- Google Maps API V3 之绘图库 信息窗口
Google官方教程: Google 地图 API V3 使用入门 Google 地图 API V3 针对移动设备进行开发 Google 地图 API V3 之事件 Google 地图 API V3 ...
- Google Maps API V3 之 图层
Google官方教程: Google 地图 API V3 使用入门 Google 地图 API V3 针对移动设备进行开发 Google 地图 API V3 之事件 Google 地图 API V3 ...
- Google Maps API V3 之 路线服务
Google官方教程: Google 地图 API V3 使用入门 Google 地图 API V3 针对移动设备进行开发 Google 地图 API V3 之事件 Google 地图 API V3 ...
- google maps api申请的问题
现在已经改由统一的GOOGLE API控制台进行所有GOOGLE API的管理了. 方法是使用Google帐号登入 https://code.google.com/apis/console. 然后在所 ...
- Google maps API开发(一)(转)
一.加载Google maps API <script type="text/javascript" src="http://ditu.google.com/map ...
- Google maps API开发(二)(转)
这一篇主要实现怎么调用Google maps API中的地址解析核心类GClientGeocoder: 主要功能包括地址解析.反向解析.本地搜索.周边搜索等, 我这里主要有两个实例: 实例一.当你搜索 ...
- Google Maps API Web Services
原文:Google Maps API Web Services 摘自:https://developers.google.com/maps/documentation/webservices/ Goo ...
- Google maps API开发
原文:Google maps API开发 Google maps API开发(一) 最近做一个小东西用到google map,突击了一下,收获不小,把自己学习的一些小例子记录下来吧 一.加载Googl ...
- Google Maps API Key申请办法(最新)
之前的Google Maps Api的API Key很容易申请,只需要按照一个简单的表单提交部署的网站地址即可,自动生成API Key并给出引用的路径. 但是最近在处理另外一个项目的时候发现之前的这种 ...
- 如何插入谷歌地图并获取javascript api 秘钥--Google Maps API error: MissingKeyMapError
参考:https://blog.csdn.net/klsstt/article/details/51744866 Google Maps API error: MissingKeyMapError h ...
随机推荐
- C语言_指针变量的赋值与运算,很详细
指针变量的赋值 指针变量同普通变量一样,使用之前不仅要定义说明, 而且必须赋予具体的值.未经赋值的指针变量不能使用, 否则将造成系统混乱,甚至死机.指针变量的赋值只能赋予地址, 决不能赋予任何其它数据 ...
- vector动态数组
vector是STL模板库中的序列式容器,利用它可以有效地避免空间的浪费. 创建vector容器 vector< int >v:vector< char >:vector< ...
- SpringBoot实战 之 接口日志篇
在本篇文章中不会详细介绍日志如何配置.如果切换另外一种日志工具之类的内容,只用于记录作者本人在工作过程中对日志的几种处理方式. 1. Debug 日志管理 在开发的过程中,总会遇到各种莫名其妙的问题, ...
- c#(控制台应用程序)实现排序算法的研究总结
前言:闲来无事,便研究起来对数组的排序算法,怕过后遗忘,特地总结一下,也希望能帮到大家 概要: 总结的算法: 冒泡排序.插入排序.选择排序 要排序的一列数(从小到大): 1, 5, 3, 83, 4 ...
- linux远程控制
linux远程控制 SSH协议:为客户机提供安全的shell环境,默认端口22OpenSSH服务服务名称:sshd主程序:/usr/sbin/sshd ,/usr/bin/ssh配置文件:/etc/s ...
- 使用dlib中的深度残差网络(ResNet)实现实时人脸识别
opencv中提供的基于haar特征级联进行人脸检测的方法效果非常不好,本文使用dlib中提供的人脸检测方法(使用HOG特征或卷积神经网方法),并使用提供的深度残差网络(ResNet)实现实时人脸识别 ...
- PHP XML简介
php xml文件编程. xml简介 XML作用 1.可以作为程序间通讯的标准(ajax text xml) 2.可以作为配置文件 3.可以作为小型数据库 XML语法 一个xml文件应该包括以下几个内 ...
- freemarker报错之九
1.错误描述 五月 30, 2014 11:52:04 下午 freemarker.log.JDK14LoggerFactory$JDK14Logger error 严重: Template proc ...
- Java和Flex整合报错(一)
1.错误描述 at org.springframework.beans.factory.support.DefaultListableBeanFactory.resolveDependency(Def ...
- 利用scrapy模拟登录知乎
闲来无事,写一个模拟登录知乎的小demo. 分析网页发现:登录需要的手机号,密码,_xsrf参数,验证码 实现思路: 1.获取验证码 2.获取_xsrf 参数 3.携带参数,请求登录 验证码url : ...