Robot Motion - poj 1573
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 11269 | Accepted: 5486 |
Description
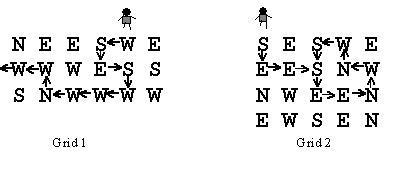
A robot has been programmed to follow the instructions in its path. Instructions for the next direction the robot is to move are laid down in a grid. The possible instructions are
N north (up the page)
S south (down the page)
E east (to the right on the page)
W west (to the left on the page)
For example, suppose the robot starts on the north (top) side of Grid 1 and starts south (down). The path the robot follows is shown. The robot goes through 10 instructions in the grid before leaving the grid.
Compare what happens in Grid 2: the robot goes through 3 instructions only once, and then starts a loop through 8 instructions, and never exits.
You are to write a program that determines how long it takes a robot to get out of the grid or how the robot loops around.
Input
Output
Sample Input
3 6 5
NEESWE
WWWESS
SNWWWW
4 5 1
SESWE
EESNW
NWEEN
EWSEN
0 0 0
Sample Output
10 step(s) to exit
3 step(s) before a loop of 8 step(s)
#include <iostream>
#include<string.h>
using namespace std; int main() {
int row,col,x,y; int pos[][];
char ins[][];
int step;
int flag=-;
cin>>row>>col>>x;
while(row&&col&&x){
memset(pos,,sizeof(int)*);
y=;
step=;
flag=-;
for(int i=;i<row;i++){
for(int j=;j<col;j++){
cin>>ins[i][j];
}
}
pos[y-][x-]=;
for(int i=;i<row*col;i++){
switch(ins[y-][x-]){
case 'N':
y--;
break;
case 'E':
x++;
break;
case 'S':
y++;
break;
case 'W':
x--;
break;
} if(y>row||y<||x>col||x<){
flag=;
break;
}else if(pos[y-][x-]!=){
flag=;
break;
}else{
++step;
pos[y-][x-]=step;
} }
if(flag==){
cout<<step<<" step(s) to exit"<<endl;
}else{
cout<<(pos[y-][x-]-)<<" step(s) before a loop of "<<(step-pos[y-][x-]+)<<" step(s)"<<endl;
}
cin>>row>>col>>x;
}
return ;
}
Robot Motion - poj 1573的更多相关文章
- 模拟 POJ 1573 Robot Motion
题目地址:http://poj.org/problem?id=1573 /* 题意:给定地图和起始位置,robot(上下左右)一步一步去走,问走出地图的步数 如果是死循环,输出走进死循环之前的步数和死 ...
- POJ 1573 Robot Motion(BFS)
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 12856 Accepted: 6240 Des ...
- Poj OpenJudge 百练 1573 Robot Motion
1.Link: http://poj.org/problem?id=1573 http://bailian.openjudge.cn/practice/1573/ 2.Content: Robot M ...
- POJ 1573 Robot Motion(模拟)
题目代号:POJ 1573 题目链接:http://poj.org/problem?id=1573 Language: Default Robot Motion Time Limit: 1000MS ...
- POJ 1573 Robot Motion
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 12978 Accepted: 6290 Des ...
- poj 1573 Robot Motion【模拟题 写个while循环一直到机器人跳出来】
...
- 【POJ - 1573】Robot Motion
-->Robot Motion 直接中文 Descriptions: 样例1 样例2 有一个N*M的区域,机器人从第一行的第几列进入,该区域全部由'N' , 'S' , 'W' , 'E' ,走 ...
- POJ 1573:Robot Motion
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 11324 Accepted: 5512 Des ...
- Robot Motion 分类: POJ 2015-06-29 13:45 11人阅读 评论(0) 收藏
Robot Motion Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 11262 Accepted: 5482 Descrip ...
随机推荐
- 洛谷 P3804 后缀自动机
题目描述 给定一个只包含小写字母的字符串SS , 请你求出 SS 的所有出现次数不为 11 的子串的出现次数乘上该子串长度的最大值. 输入输出格式 输入格式: 一行一个仅包含小写字母的字符串SS 输出 ...
- lua的table.sort
local aa = {{a=11},{a=44},{a=33},{a=2} } table.sort(aa,function(a,b) return a.a>b.a end) for k, v ...
- 【转载】 GNU GCC 选项说明
GCC 1 Section: GNU Tools (1) Updated: 2003/12/05 Sponsor: GCC Casino Winning Content NAME gcc,g++-GN ...
- UVa 816 (BFS求最短路)
/*816 - Abbott's Revenge ---代码完全参考刘汝佳算法入门经典 ---strchr() 用来查找某字符在字符串中首次出现的位置,其原型为:char * strchr (cons ...
- vs2015安装VAssistX以后,去除中文注释会有红色下划线方法
---恢复内容开始--- 环境:Visual Studio 2015 问题:代码中出现中文后会带下划线,不舒服-----解决办法. 1.安装完Visual Assist X后会在VS2015的菜单栏出 ...
- Preference Learning——Object Ranking
Basics About Orders Object Ranking应用: 量化的受訪者的感觉或印象(quantification of respondents' sensations or impr ...
- jmap -histo pid 输出的[C [B [I [S methodKlass的含义
转载于https://yq.aliyun.com/articles/43542 摘要: jmap -histo pid 输出结果样式 num #instances #byte ...
- asp.net显示评论的时候为几天前的格式
自己做的一个小项目实现的功能,做个记录先~ 效果如图: 代码如下: public static class TimerHelper { public static string GetTimeSpan ...
- Javascript modules--js 模块化
https://medium.freecodecamp.org/javascript-modules-a-beginner-s-guide-783f7d7a5fcc 这个网站也是非常好:https:/ ...
- Sublime Text 3 文档
中文版:http://feliving.github.io/Sublime-Text-3-Documentation/ 英文版:http://www.sublimetext.com/docs/3/