UVA-548Tree(二叉树的递归遍历)
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
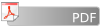
You are to determine the value of the leaf node in a given binary tree that is the terminal node of a path of least value from the root of the binary tree to any leaf. The value of a path is the sum of values of nodes along that path.
Input
The input file will contain a description of the binary tree given as the inorder and postorder traversal sequences of that tree. Your program will read two line (until end of file) from the input file. The first line will contain the sequence of values associated with an inorder traversal of the tree and the second line will contain the sequence of values associated with a postorder traversal of the tree. All values will be different, greater than zero and less than 10000. You may assume that no binary tree will have more than 10000 nodes or less than 1 node.
Output
For each tree description you should output the value of the leaf node of a path of least value. In the case of multiple paths of least value you should pick the one with the least value on the terminal node.
Sample Input
3 2 1 4 5 7 6
3 1 2 5 6 7 4
7 8 11 3 5 16 12 18
8 3 11 7 16 18 12 5
255
255
Sample Output
1
3
255
题解:给出了一个二叉树的中序和后序遍历,让求树枝的最小总长的最小枝节点;思路是把这个二叉树求出来,然后找最小值,找最小值的地方错了好多次;必须要先找最小总长,再找相等的情况下最小枝节点;另外后序遍历其实倒过来就是先序遍历;在后序遍历的最后一个点其实就是根节点,根据这个在中序遍历里面递归找左右树;
代码:
#include<cstdio>
#include<cstring>
#include<cmath>
#include<iostream>
#include<algorithm>
#include<queue>
using namespace std;
#define SI(x) scanf("%d",&x)
#define mem(x,y) memset(x,y,sizeof(x))
#define PI(x) printf("%d",x)
#define P_ printf(" ")
const int INF=0x3f3f3f3f;
typedef long long LL;
const int MAXN=100010;
int v1[MAXN],v2[MAXN];
char s[MAXN];
int ans,minlength;
struct Node{
int v;
Node *L,*R;
Node(){
L=NULL;R=NULL;
}
};
int init(char *s,int *v){
int k=0;
for(int i=0;s[i];i++){
while(isdigit(s[i]))v[k]=v[k]*10+s[i++]-'0';
k++;
}
// for(int i=0;i<k;i++)printf("%d ",v[i]);puts("");
return k;
}
int find(int n,int *v,int t){
for(int i=n-1;i>=0;i--)
if(v[i]==t)return i;
return 0;
}
Node* build(int n,int *v1,int *v2){
Node *root;
if(n<=0)return NULL;
root=new Node;
root->v=v2[n-1];
int p=find(n,v1,v2[n-1]);
root->L=build(p,v1,v2);
root->R=build(n-p-1,v1+p+1,v2+p);
return root;
}
void dfs(Node *root,int length){
if(root==NULL)return;
length+=root->v;
if(root->L==NULL&&root->R==NULL){
if(minlength>length)
{
minlength=length;
ans=root->v;
}
else if(minlength==length)
ans=min(ans,root->v);
return;
}
dfs(root->L,length);
dfs(root->R,length);
}
int main(){
while(gets(s)){
mem(v1,0);mem(v2,0);
init(s,v1);
gets(s);
int k=init(s,v2);
Node *root=build(k,v1,v2);
ans=minlength=INF;
dfs(root,0);
printf("%d\n",ans);
}
return 0;
}
UVA-548Tree(二叉树的递归遍历)的更多相关文章
- UVa 548 (二叉树的递归遍历) Tree
题意: 给出一棵由中序遍历和后序遍历确定的点带权的二叉树.然后找出一个根节点到叶子节点权值之和最小(如果相等选叶子节点权值最小的),输出最佳方案的叶子节点的权值. 二叉树有三种递归的遍历方式: 先序遍 ...
- Uva 548 二叉树的递归遍历lrj 白书p155
直接上代码... (另外也可以在递归的时候统计最优解,不过程序稍微复杂一点) #include <iostream> #include <string> #include &l ...
- 二叉树的递归遍历 The Falling Leaves UVa 699
题意:对于每一棵树,每一个结点都有它的水平位置,左子结点在根节点的水平位置-1,右子节点在根节点的位置+1,从左至右输出每个水平位置的节点之和 解题思路:由于上题所示的遍历方式如同二叉树的前序遍历,与 ...
- C++编程练习(17)----“二叉树非递归遍历的实现“
二叉树的非递归遍历 最近看书上说道要掌握二叉树遍历的6种编写方式,之前只用递归方式编写过,这次就用非递归方式编写试一试. C++编程练习(8)----“二叉树的建立以及二叉树的三种遍历方式“(前序遍历 ...
- 二叉树的递归遍历 Tree UVa548
题意:给一棵点带权的二叉树的中序和后序遍历,找一个叶子使得他到根的路径上的权值的和最小,如果多解,那该叶子本身的权值应该最小 解题思路:1.用getline()输入整行字符,然后用stringstre ...
- Trees on the level UVA - 122 (二叉树的层次遍历)
题目链接:https://vjudge.net/problem/UVA-122 题目大意:输入一颗二叉树,你的任务是按从上到下,从左到右的顺序输出各个结点的值.每个结点都按照从根节点到它的移动序列给出 ...
- 数据结构之二叉树篇卷三 -- 二叉树非递归遍历(With Java)
Nonrecursive Traversal of Binary Tree First I wanna talk about why we should <code>Stack</c ...
- UVa 548 Tree【二叉树的递归遍历】
题意:给出一颗点带权的二叉树的中序和后序遍历,找一个叶子使得它到根的路径上的权和最小. 学习的紫书:先将这一棵二叉树建立出来,然后搜索一次找出这样的叶子结点 虽然紫书的思路很清晰= =可是理解起来好困 ...
- UVA - 548 Tree(二叉树的递归遍历)
题意:已知中序后序序列,求一个叶子到根路径上权和最小,如果多解,则叶子权值尽量小. 分析:已知中序后序建树,再dfs求从根到各叶子的权和比较大小 #include<cstdio> #inc ...
- UVa 122 (二叉树的层次遍历) Trees on the level
题意: 输入一颗二叉树,按照(左右左右, 节点的值)的格式.然后从上到下从左到右依次输出各个节点的值,如果一个节点没有赋值或者多次赋值,则输出“not complete” 一.指针方式实现二叉树 首先 ...
随机推荐
- artdialog关闭弹出窗口
打开 function opentree(){ var dialog = art.dialog({ title: '选择提交部门', content:jQuery("#my ...
- PHP中date函数参数详解
date函数输出当前的时间echo date('Y-m-d H:i:s', time()); // 格式:xxxx-xx-xx xx:xx:xx 第一个参数的格式分别表示: a - "am& ...
- javascript学习笔记(1) 简单html语法
<html> <head><meta http-equiv="content-type" content="text/html" ...
- 得到IP包的数据意义(简单实现例子)
#include <stdio.h> #include <unistd.h> #include <linux/if_ether.h> #include <li ...
- [摘]ASP.Net标准控件(TextBox控件)
TextBox控件 TextBox控件又称文本框控件,为用户提供输入文本的功能. 1.属性 TextBox控件的常用属性及说明如表1所示. 表1 TextBox控件常用属性及说明 属 性 说 ...
- Adobe Acrobat XI Pro 官方下载及安装破解
Adobe公司推出的PDF 格式是一种全新的电子文档格式.借助 Acrobat ,您几乎可以用便携式文档格式 (Portable Document Format ,简称 PDF) 出版所有的文档. P ...
- WPF中的触发器简单总结
原文 http://blog.sina.com.cn/s/blog_5f2ed5cb0100p3ab.html 触发器,从某种意义上来说它也是一种Style,因为它包含有一个Setter集合,并根据一 ...
- c# 多显示器设置主屏幕(Set primary screen for multiple monitors)
原文 http://www.cnblogs.com/coolkiss/archive/2013/09/18/3328854.html 经过google加各种百度,终于找到了一个有效的解决方案,下面是两 ...
- 一些User-Agent
"Mozilla/4.0 (compatible; MSIE 7.0; Windows NT 6.0)", "Mozilla/4.0 (compatible; MSIE ...
- cdoj 847 方老师与栈 火车进出战问题
//其实我是不想写这题的,但是这题让我想起了我年轻的时候 解法:直接模拟栈就好. //另外我年轻时候做的那题数据范围比较小,原理也不一样. //对于序列中的任何一个数其后面所有比它小的数应该是倒序的, ...