(1)第一个ASP.NET Web API
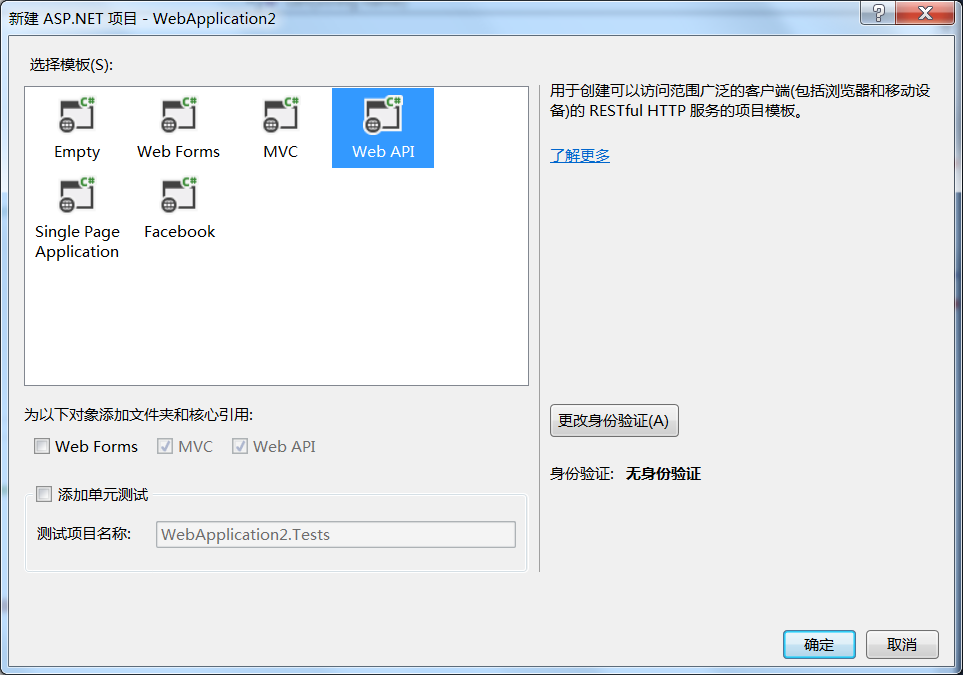
。
protected void Application_Start()
{
AreaRegistration.RegisterAllAreas();
//配置API路由
GlobalConfiguration.Configure(WebApiConfig.Register);
FilterConfig.RegisterGlobalFilters(GlobalFilters.Filters);
RouteConfig.RegisterRoutes(RouteTable.Routes);
BundleConfig.RegisterBundles(BundleTable.Bundles);
}
App_Start/WebApiConfig.cs
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API configuration and services
// Web API routes
config.MapHttpAttributeRoutes();
config.Routes.MapHttpRoute(
name: "DefaultApi",
routeTemplate: "{controller}/{id}",
defaults: new { id = RouteParameter.Optional }
);
}
}
Storages.cs
using System;
using System.Collections.Generic;
using System.Linq;
using System.Web;
namespace TmplWebApiDemo.Models
{
public class Storages
{
public static IEnumerable<Student> Students { get; set; }
public static IEnumerable<Teacher> Teachers { get; set; }
static Storages()
{
Students = new List<Student>
{
new Student{Id =1,Name="张三",Age =11,Gender=false},
new Student{Id =2,Name="李四",Age =21,Gender=true},
new Student{Id =3,Name="王五",Age =22,Gender=false},
new Student{Id =4,Name="赵飞燕",Age =25,Gender=true},
new Student{Id =5,Name="王刚",Age =31,Gender=true}
};
Teachers = new List<Teacher>
{
new Teacher{Id =1,Name="老师1",Age =11,Gender=false},
new Teacher{Id =2,Name="老师2",Age =21,Gender=true},
new Teacher{Id =3,Name="老师3",Age =22,Gender=false},
new Teacher{Id =4,Name="老师4",Age =25,Gender=true}
};
}
}
public class Person
{
public int Id { get; set; }
public string Name { get; set; }
public int Age { get; set; }
public bool Gender { get; set; }
}
public class Student : Person
{
}
public class Teacher : Person
{
}
}
/// <summary>
/// 学生资源集合
/// </summary>
public class StudentsController : ApiController
{
//c r u d
/// <summary>
/// GET / Students/
/// </summary>
public IEnumerable<Student> Get()
{
return Storages.Students;
}
/// <summary>
/// GET / students/zhangsan return entity
/// </summary>
/// <returns></returns>
public Student Get(string name)
{
return Storages.Students.FirstOrDefault(s => s.Name.Equals(name, StringComparison.InvariantCultureIgnoreCase));
}
}
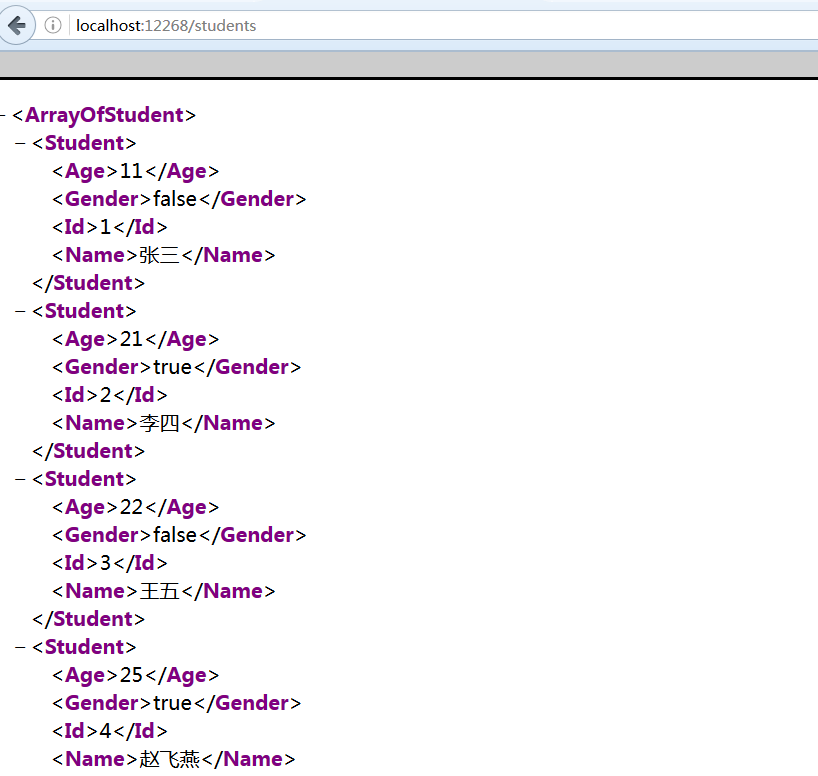
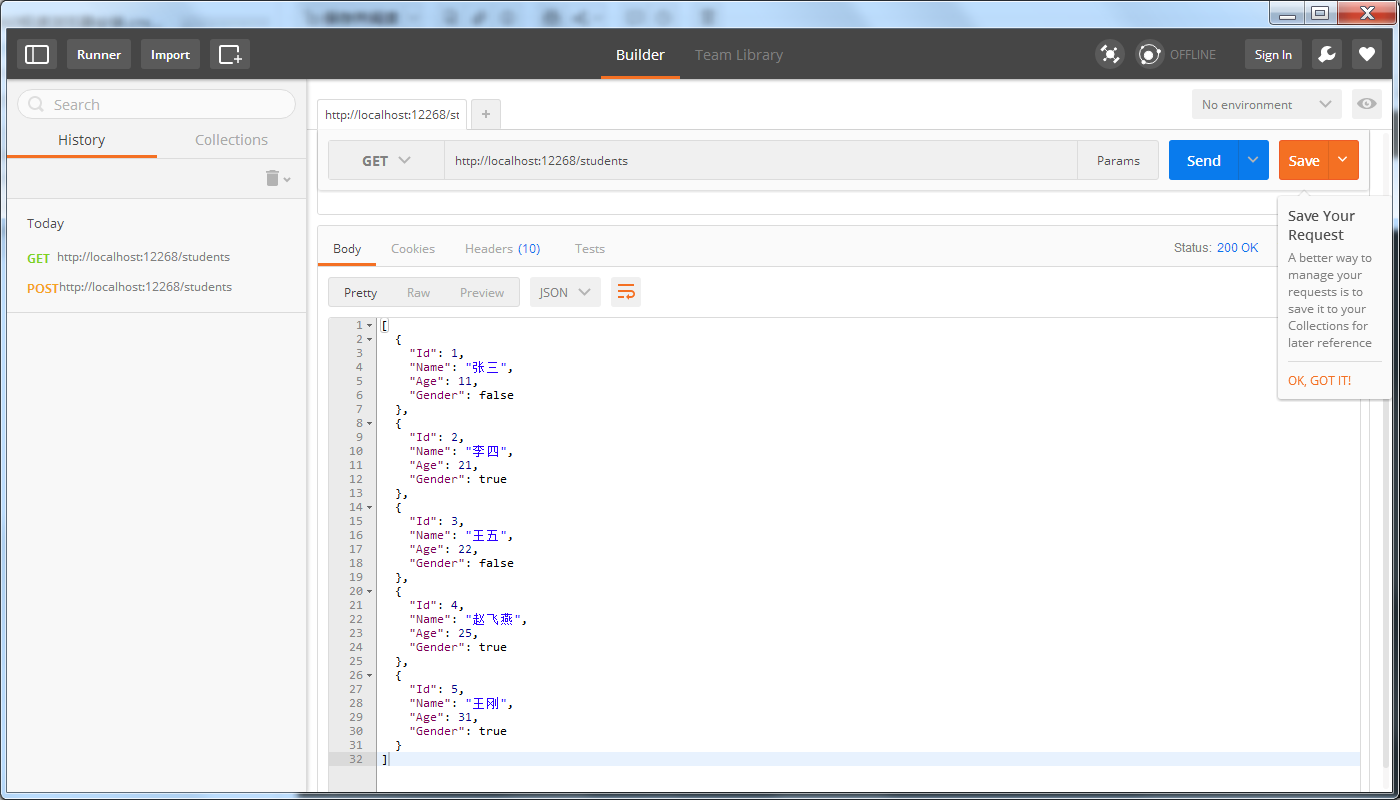
(1)第一个ASP.NET Web API的更多相关文章
- 【ASP.NET Web API教程】1.1 第一个ASP.NET Web API
Your First ASP.NET Web API (C#)第一个ASP.NET Web API(C#) By Mike Wasson|January 21, 2012作者:Mike Wasson ...
- 一个ASP.NET Web API 2.0应用
在一个空ASP.NET Web项目上创建一个ASP.NET Web API 2.0应用 由于ASP.NET Web API具有与ASP.NET MVC类似的编程方式,再加上目前市面上专门介绍ASP.N ...
- 在一个空ASP.NET Web项目上创建一个ASP.NET Web API 2.0应用
由于ASP.NET Web API具有与ASP.NET MVC类似的编程方式,再加上目前市面上专门介绍ASP.NET Web API 的书籍少之又少(我们看到的相关内容往往是某本介绍ASP.NET M ...
- 第一个ASP.NET Web API (C#)程序
本文翻自http://www.asp.net/web-api/overview/getting-started-with-aspnet-web-api 绝对手工制作,如有雷同,实属巧合. 转载请注明. ...
- 如何创建一个Asp .Net Web Api项目
1.点击文件=>新建=>项目 2.创建一个Asp .NET Web项目 3.选择Empty,然后选中下面的MVC和Web Api,也可以直接选择Web Api选项,注意将身份验证设置为无身 ...
- ASP.NET Web API 记录请求响应数据到日志的一个方法
原文:http://blog.bossma.cn/dotnet/asp-net-web-api-log-request-response/ ASP.NET Web API 记录请求响应数据到日志的一个 ...
- How ASP.NET Web API 2.0 Works?[持续更新中…]
一.概述 RESTful Web API [Web标准篇]RESTful Web API [设计篇] 在一个空ASP.NET Web项目上创建一个ASP.NET Web API 2.0应用 二.路由 ...
- ASP.NET Web API中的依赖注入
什么是依赖注入 依赖,就是一个对象需要的另一个对象,比如说,这是我们通常定义的一个用来处理数据访问的存储,让我们用一个例子来解释,首先,定义一个领域模型如下: namespace Pattern.DI ...
- OData services入门----使用ASP.NET Web API描述
http://www.cnblogs.com/muyoushui/archive/2013/01/27/2878844.html ODate 是一种应用层协议,设计它的目的在于提供一组通过HTTP的交 ...
随机推荐
- iOS 简单音乐播放器 界面搭建
如图搭建一个音乐播放器界面,具备以下几个简单功能: 1,界面协调,整洁. 2,点击播放,控制进度条. 3.三收藏歌曲,点击收藏,心形收藏标志颜色加深. 4,左右按钮,切换歌曲图片和标题. 5,点击中间 ...
- BestCoder Round #61 1001 Numbers
Problem Description There are n numbers A1,A2....An{A}_{1},{A}_{2}....{A}_{n}A1,A2....An,yo ...
- easyui datagrid中datetime字段的显示和增删改查问题
datagrid中datetime字段的异常显示: 使用过easyui datagrid的应该都知道,如果数据库中的字段是datetime类型,绑定在datagrid显式的时候会不正常显示,一般需要借 ...
- Minimum Adjustment Cost
Given an integer array, adjust each integers so that the difference of every adjacent integers are n ...
- [转载]Python-第三方库requests详解
Requests 是用Python语言编写,基于 urllib,采用 Apache2 Licensed 开源协议的 HTTP 库.它比 urllib 更加方便,可以节约我们大量的工作,完全满足 HTT ...
- XPath 教程
http://www.w3school.com.cn/xpath/xpath_syntax.asp
- 【转】你可能不知道的Shell
本文转自http://coolshell.cn/articles/8619.html,只摘取了其中的一部分. 再分享一些可能你不知道的shell用法和脚本,简单&强大! 在阅读以下部分前,强烈 ...
- Match:Seek the Name, Seek the Fame(POJ 2752)
追名逐利 题目大意:给定一个字符串S,要你找到S的所有前缀后缀数组 还是Kmp的Next数组的简单应用,但是这一题有一个BUG,那就是必须输出字符串的长度(不输出就WA),然而事实上对于abcbab, ...
- Mysql 基础 高级查询
在西面内容中 car 和 nation 都表示 表名 1.无论 高级查询还是简单查询 都用 select.. from..语句 from 后面 加表名 可以使一张表也可以是 ...
- dhtmlxTree介绍(转载)
dhtmlxTree 是树菜单,允许我们快速开发界面优美,基于Ajax的javascript库. 她允许在线编辑,拖拽,三种状态(全选.不选.半选),复选框等模式.同时在加载大数据量的时候,仍然 可以 ...