POJ 2286 The Rotation Game(IDA*)
Time Limit: 15000MS | Memory Limit: 150000K | |
Total Submissions: 6396 | Accepted: 2153 |
Description
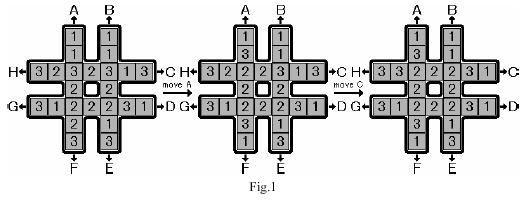
Initially, the blocks are placed on the board randomly. Your task is to move the blocks so that the eight blocks placed in the center square have the same symbol marked. There is only one type of valid move, which is to rotate one of the four lines, each consisting of seven blocks. That is, six blocks in the line are moved towards the head by one block and the head block is moved to the end of the line. The eight possible moves are marked with capital letters A to H. Figure 1 illustrates two consecutive moves, move A and move C from some initial configuration.
Input
Output
Sample Input
1 1 1 1 3 2 3 2 3 1 3 2 2 3 1 2 2 2 3 1 2 1 3 3
1 1 1 1 1 1 1 1 2 2 2 2 2 2 2 2 3 3 3 3 3 3 3 3
0
Sample Output
AC
2
DDHH
2
题目链接:POJ 2286
第一道IDA*题目,由于用的是递归的写法,代码量实际上不会很大,只要在dfs入口处做好各种判断就可以了 ,能用IDA*的前提至少答案要存在,如果不存在的话搜索深度会无限加深就没有意义了,然后每一次都用估价函数剪枝即可,再加一个防止来回的剪枝速度可以快一倍
大致伪代码如下:
void dfs(state, dep)
{
计算此时状态下的估价函数值h(state)
if(h(state)==0)
已到终点,返回true
else if(h(state)+dep>Maxdep)
在规定的Maxdep内一定走不到终点,返回false
else
{
for....
{
得到新状态n_state
if(dfs(n_state,dep))
return 1;
}
}
return 0;
}
代码:
#include <stdio.h>
#include <iostream>
#include <algorithm>
#include <cstdlib>
#include <sstream>
#include <numeric>
#include <cstring>
#include <bitset>
#include <string>
#include <deque>
#include <stack>
#include <cmath>
#include <queue>
#include <set>
#include <map>
using namespace std;
#define INF 0x3f3f3f3f
#define LC(x) (x<<1)
#define RC(x) ((x<<1)+1)
#define MID(x,y) ((x+y)>>1)
#define CLR(arr,val) memset(arr,val,sizeof(arr))
#define FAST_IO ios::sync_with_stdio(false);cin.tie(0);
typedef pair<int, int> pii;
typedef long long LL;
const double PI = acos(-1.0);
const int N = 25; int arr[N], cnt[4];
int Max_dep, Num;
char ans[1000];
int top,Back[8]={5,4,7,6,1,0,3,2};//Back数组,减掉正拉动后又马上反向拉动的无意义搜索 int pos[8][7] =
{
{1, 3, 7, 12, 16, 21, 23}, //A0
{2, 4, 9, 13, 18, 22, 24}, //B1
{11, 10, 9, 8, 7, 6, 5}, //C2
{20, 19, 18, 17, 16, 15, 14}, //D3
{24, 22, 18, 13, 9, 4, 2},//E4
{23, 21, 16, 12, 7, 3, 1},//F5
{14, 15, 16, 17, 18, 19, 20},//G6
{5, 6, 7, 8, 9, 10, 11},//H7
};
int Need(int cur[])
{
int i;
cnt[1] = cnt[2] = cnt[3] = 0;
for (i = 7; i <= 9; ++i)
++cnt[cur[i]];
for (i = 12; i <= 13; ++i)
++cnt[cur[i]];
for (i = 16; i <= 18; ++i)
++cnt[cur[i]];
int Need_1 = 8 - cnt[1], Need_2 = 8 - cnt[2], Need_3 = 8 - cnt[3];
int Min_Need = min(Need_1, min(Need_2, Need_3));
return Min_Need;
}
int IDA_star(int dep, int Arr[],int pre)
{
int Need_cur = Need(Arr);
if (Need_cur == 0)
{
Num = Arr[7];
return 1;
}
if (Need_cur + dep > Max_dep)
return 0;
int Temp_arr[N]; for (int Opsid = 0; Opsid < 8; ++Opsid)
{
if(Opsid==pre)
continue;
ans[top++] = 'A' + Opsid; for (int i = 1; i <= 24; ++i)
Temp_arr[i] = Arr[i];
for (int i = 0; i < 7; ++i)
Temp_arr[pos[Opsid][i]] = Arr[pos[Opsid][(i + 1) % 7]]; if (IDA_star(dep + 1, Temp_arr, Back[Opsid]))
return 1;
else
--top;
}
return 0;
}
int main(void)
{
while (~scanf("%d", &arr[1]) && arr[1])
{
for (int i = 2; i <= 24; ++i)
scanf("%d", &arr[i]);
if (Need(arr) == 0)
{
puts("No moves needed");
printf("%d\n", arr[7]);
}
else
{
top = 0;
Max_dep = 1;
Num = -1;
while (!IDA_star(0, arr, -1))
++Max_dep;
ans[top] = '\0';
puts(ans);
printf("%d\n", Num);
}
}
return 0;
}
POJ 2286 The Rotation Game(IDA*)的更多相关文章
- POJ - 2286 - The Rotation Game (IDA*)
IDA*算法,即迭代加深的A*算法.实际上就是迭代加深+DFS+估价函数 题目传送:The Rotation Game AC代码: #include <map> #include < ...
- POJ2286 The Rotation Game(IDA*)
The Rotation Game Time Limit: 15000MS Memory Limit: 150000K Total Submissions: 5691 Accepted: 19 ...
- UVA-1343 The Rotation Game (IDA*)
题目大意:数字1,2,3都有八个,求出最少的旋转次数使得图形中间八个数相同.旋转规则:对于每一长行或每一长列,每次旋转就是将数据向头的位置移动一位,头上的数放置到尾部.若次数相同,则找出字典序最小旋转 ...
- 【UVa】1343 The Rotation Game(IDA*)
题目 题目 分析 lrj代码.... 还有is_final是保留字,害的我CE了好几发. 代码 #include <cstdio> #include <algorit ...
- POJ 1979 Red and Black (红与黑)
POJ 1979 Red and Black (红与黑) Time Limit: 1000MS Memory Limit: 30000K Description 题目描述 There is a ...
- POJ 3268 Silver Cow Party (最短路径)
POJ 3268 Silver Cow Party (最短路径) Description One cow from each of N farms (1 ≤ N ≤ 1000) convenientl ...
- POJ.3087 Shuffle'm Up (模拟)
POJ.3087 Shuffle'm Up (模拟) 题意分析 给定两个长度为len的字符串s1和s2, 接着给出一个长度为len*2的字符串s12. 将字符串s1和s2通过一定的变换变成s12,找到 ...
- POJ.1426 Find The Multiple (BFS)
POJ.1426 Find The Multiple (BFS) 题意分析 给出一个数字n,求出一个由01组成的十进制数,并且是n的倍数. 思路就是从1开始,枚举下一位,因为下一位只能是0或1,故这个 ...
- Booksort POJ - 3460 (IDA*)
Description The Leiden University Library has millions of books. When a student wants to borrow a ce ...
随机推荐
- js 实现纯前端将数据导出excel两种方式,亲测有效
由于项目需要,需要在不调用后台接口的情况下,将json数据导出到excel表格,兼容chrome没问题,其他还没有测试过 通过将json遍历进行字符串拼接,将字符串输出到csv文件,输出的文件不会再是 ...
- python_26_dictionary
#key-value 字典无下标 所以乱序,key值尽量不要取中文 info={ 'stu1101':'Liu Guannan', 'stu1102':'Wang Ruipu', 'stu1103': ...
- Java环境变量搭建(Windows环境)
变量名:JAVA_HOME 变量值:C:\Program Files (x86)\Java\jdk1.8.0_91 // 要根据自己的实际路径配置 变量名:CLASSPATH 变量值:. ...
- DongDong跳一跳
题目连接:https://ac.nowcoder.com/acm/contest/904/C 题意很好理解,思路想歪了,本来一道很简单的题,写了好久没写出来. 思路就是找每一个高度最大值的时候就是找“ ...
- Ubuntu安装mysql和简单使用
一.安装mysql sudo apt-get install mysql-server sudo apt-get isntall mysql-client sudo apt-get install l ...
- ZJOI2019Round#1
考的这么差二试基本不用去了 不想说什么了.就把这几天听课乱记的东西丢上来吧 这里是二试乱听课笔记ZJOI2019Round#2 ZJOI Round#1 Day1 M.<具体数学>选讲 罗 ...
- Python 正则表达式 利用括号分组
如果想把区号从匹配的电话号码中分离,可以添加括号在正则表达式中创建分组,再使用group()方法,从一个分组中获取匹配的文本 正则表达式字符串中,第一个括号是第一组,第二个括号是第二组.向group( ...
- 课时21.img标签(掌握)
1.img标签中的img其实是英文image的缩写,所以img标签的作用,就是告诉浏览器我们需要显示一张图片 2.img标签格式:<img src=" "> img是 ...
- python 2.7版本解决TypeError: 'encoding' is an invalid keyword argument for this function
今天在用yaml处理数据时,由于yaml.load可接收一个byte字符串,unicode字符串,打开的二进制文件或文本文件对象,但字节字符串和文件必须是utf-8,utf-16-be或utf-16- ...
- javascript隐藏和显示元素以及清空textarea
当前希望写一个单选框,选中“paste”则显示粘贴框,选中“upload”则提示选择文件. 因为这两种情况只是显示不同,所以只需要用javascript来进行显示和隐藏. 最后的结果大概这样: 初始时 ...