POJ3126 Prime Path —— BFS + 素数表
题目链接:http://poj.org/problem?id=3126
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 22936 | Accepted: 12706 |
Description
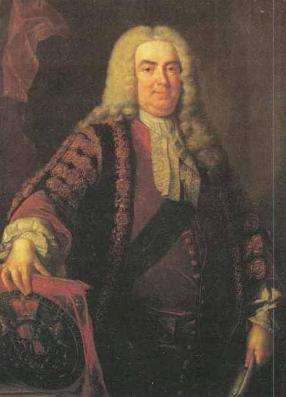
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033
1733
3733
3739
3779
8779
8179
The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
Input
Output
Sample Input
3
1033 8179
1373 8017
1033 1033
Sample Output
6
7
0
Source
题解:
1.打印素数表。
2.由于只有四位数,直接枚举不会超时。由于要求的是“最少步数”,所以用BFS进行搜索。
写法一:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cmath>
#include <algorithm>
#include <vector>
#include <queue>
#include <stack>
#include <map>
#include <string>
#include <set>
#define ms(a,b) memset((a),(b),sizeof((a)))
using namespace std;
typedef long long LL;
const int INF = 2e9;
const LL LNF = 9e18;
const int MOD = 1e9+;
const int MAXN = +; struct node //num为素数,dig[]为这个素数的每个位上的数,便于操作。
{
int num, dig[], step;
}; int vis[], pri[]; queue<node>que;
int bfs(node s, node e)
{
ms(vis,);
while(!que.empty()) que.pop();
s.step = ;
vis[s.num] = ;
que.push(s); node now, tmp;
while(!que.empty())
{
now = que.front();
que.pop(); if(now.num==e.num)
return now.step; for(int i = ; i<; i++) //枚举位数
for(int j = ; j<; j++) //枚举数字
{
if(i== && j==) continue; //首位不能为0
tmp = now;
tmp.dig[i] = j; //第i为变为j
tmp.num = tmp.dig[] + tmp.dig[]*+tmp.dig[]*+tmp.dig[]*;
if(!pri[tmp.num] && !vis[tmp.num]) //num为素数并且没有被访问
{
vis[tmp.num] = ;
tmp.step++;
que.push(tmp);
}
}
}
return -;
} void init() //素数表,pri[]==0的为素数
{
int m = sqrt(+0.5);
ms(pri,);
pri[] = ;
for(int i = ; i<=m; i++) if(!pri[i])
for(int j = i*i; j<=; j += i)
pri[j] = ;
} int main()
{
init();
int T;
scanf("%d",&T);
while(T--)
{
int n, m;
node s, e;
scanf("%d%d",&n,&m);
s.num = n; e.num = m;
for(int i = ; i<; i++, n /= ) s.dig[i] = n%;
for(int i = ; i<; i++, m /= ) e.dig[i] = m%; int ans = bfs(s,e);
if(ans==-)
puts("Impossible");
else
printf("%d\n",ans);
}
}
写法二:
#include <iostream>
#include <cstdio>
#include <cstring>
#include <cmath>
#include <algorithm>
#include <vector>
#include <queue>
#include <stack>
#include <map>
#include <string>
#include <set>
#define ms(a,b) memset((a),(b),sizeof((a)))
using namespace std;
typedef long long LL;
const int INF = 2e9;
const LL LNF = 9e18;
const int MOD = 1e9+;
const int MAXN = +; struct node
{
int num, step;
}; int vis[], dig[];
int pri[]; queue<node>que;
int bfs(int s, int e)
{
ms(vis,);
while(!que.empty()) que.pop(); node now, tmp;
now.num = s;
now.step = ;
vis[now.num] = ;
que.push(now); while(!que.empty())
{
now = que.front();
que.pop(); if(now.num==e)
return now.step; dig[] = now.num%;
dig[] = (now.num/)%;
dig[] = (now.num/)%;
dig[] = (now.num/)%;
for(int i = ; i<; i++)
for(int j = ; j<; j++)
{
if(i== && j==) continue;
tmp.num = now.num + (j- dig[i])*pow(,i); //pow前面不要加上强制类型(int)
// tmp.num = now.num + (j- dig[i])* (int)pow(10,i);
// (int)pow(10,2) 居然等于99, 看来还是不要依赖这些函数
if(!pri[tmp.num] && !vis[tmp.num])
{
vis[tmp.num] = ;
tmp.step = now.step + ;
que.push(tmp);
}
}
}
return -;
} void init()
{
int m = sqrt(+0.5);
ms(pri,);
pri[] = ;
for(int i = ; i<=m; i++) if(!pri[i])
for(int j = i*i; j<=; j += i)
pri[j] = ;
} int main()
{
init();
int T;
scanf("%d",&T);
while(T--)
{
int n, m;
scanf("%d%d",&n,&m);
int ans = bfs(n,m);
if(ans==-)
puts("Impossible");
else
printf("%d\n",ans);
}
}
POJ3126 Prime Path —— BFS + 素数表的更多相关文章
- [HDU 1973]--Prime Path(BFS,素数表)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1973 Prime Path Time Limit: 5000/1000 MS (Java/Others ...
- POJ3126 Prime Path (bfs+素数判断)
POJ3126 Prime Path 一开始想通过终点值双向查找,从最高位开始依次递减或递增,每次找到最接近终点值的素数,后来发现这样找,即使找到,也可能不是最短路径, 而且代码实现起来特别麻烦,后来 ...
- POJ3126 Prime Path(BFS)
题目链接. AC代码如下: #include <iostream> #include <cstdio> #include <cstring> #include &l ...
- poj3126 Prime Path 广搜bfs
题目: The ministers of the cabinet were quite upset by the message from the Chief of Security stating ...
- POJ2126——Prime Path(BFS)
Prime Path DescriptionThe ministers of the cabinet were quite upset by the message from the Chief of ...
- POJ 3126 Prime Path(BFS 数字处理)
意甲冠军 给你两个4位质数a, b 每次你可以改变a个位数,但仍然需要素数的变化 乞讨a有多少次的能力,至少修改成b 基础的bfs 注意数的处理即可了 出队一个数 然后入队全部能够由这个素 ...
- poj 3126 Prime Path bfs
题目链接:http://poj.org/problem?id=3126 Prime Path Time Limit: 1000MS Memory Limit: 65536K Total Submi ...
- poj3126 Prime Path(c语言)
Prime Path Description The ministers of the cabinet were quite upset by the message from the Chief ...
- [POJ]P3126 Prime Path[BFS]
[POJ]P3126 Prime Path Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 35230 Accepted: ...
随机推荐
- maven配置中国下载源【转:http://www.cnblogs.com/libingbin/p/5949483.html】
修改 配置文件 maven 安装 路径 F:\apache-maven-3.3.9\conf 修改 settings.xml或者在.m2文件夹下新建一个settings.xml 阿里源 <mir ...
- PHP中使用GD库方式画时钟
<!--demo.html中内容--> <body> <img id="time" src="test.php" /> &l ...
- HTML 文档之 Head 最佳实践--摘抄
HTML 文档之 Head 最佳实践 story 01-10 阅读 353 收藏 0 收藏 这篇文章整理了作者认可的一些最佳实践,写在这里与各位分享 阅读原文折叠收起 HTML 文档之 Head 最佳 ...
- es6 递归 tree
function loop(data) { let office = data.map(item => { if(item.type == '1' ||item.type == '2') { i ...
- avi视频文件提取与合并
最近在做一个avi视频文件的提取与合并,花了几天熟悉avi文件格式.制作了一个提取与合并的动态库,不过仅限于提取视频,视频的合并还没添加一些额外判断,可能导致不同分辨率的视频文件合成后不能播放.欢迎大 ...
- Codeforces 123 E Maze
Discription A maze is represented by a tree (an undirected graph, where exactly one way exists betwe ...
- Docker资源限制实现——cgroup
摘要 随着Docker技术被越来越多的个人.企业所接受,其用途也越来越广泛.Docker资源管理包含对CPU.内存.IO等资源的限制,但大部分Docker使用者在使用资源管理接口时往往还比较模糊. 本 ...
- poj1870--Bee Breeding(模拟)
题目链接:点击打开链接 题目大意:给出一个蜂窝,也就是有六边形组成,从内向外不断的循环(如图).给出两个数的值u,v按六边形的走法,由中心向六个角走.问由u到v的的最小步数. 首先处理处每个数的坐标, ...
- reorder-list——链表、快慢指针、逆转链表、链表合并
Given a singly linked list L: L0→L1→…→Ln-1→Ln,reorder it to: L0→Ln→L1→Ln-1→L2→Ln-2→… You must do thi ...
- MySql视频教程——百度云下载路径
百度云分享MySql视频教程给大家.祝大家事业进步! MySql视频教程:http://pan.baidu.com/s/1gdCHX79 password:n46i