Unity3D_(API)场景切换SceneManager
Unity场景切换SceneManager 官方文档:传送门
静态方法
创建场景
GetActiveScene Gets the currently active Scene.
GetSceneAt Get the Scene at index in the SceneManager's list of loaded Scenes.
GetSceneByName Searches through the Scenes loaded for a Scene with the given name.
GetSceneByPath Searches all Scenes loaded for a Scene that has the given asset path.
LoadScene Loads the Scene by its name or index in Build Settings.
LoadSceneAsync Loads the Scene asynchronously in the background.
MergeScenes This will merge the source Scene into the destinationScene.
MoveGameObjectToScene Move a GameObject from its current Scene to a new Scene.
SetActiveScene Set the Scene to be active.
UnloadSceneAsync Destroys all GameObjects associated with the given Scene and removes the Scene from the SceneManager
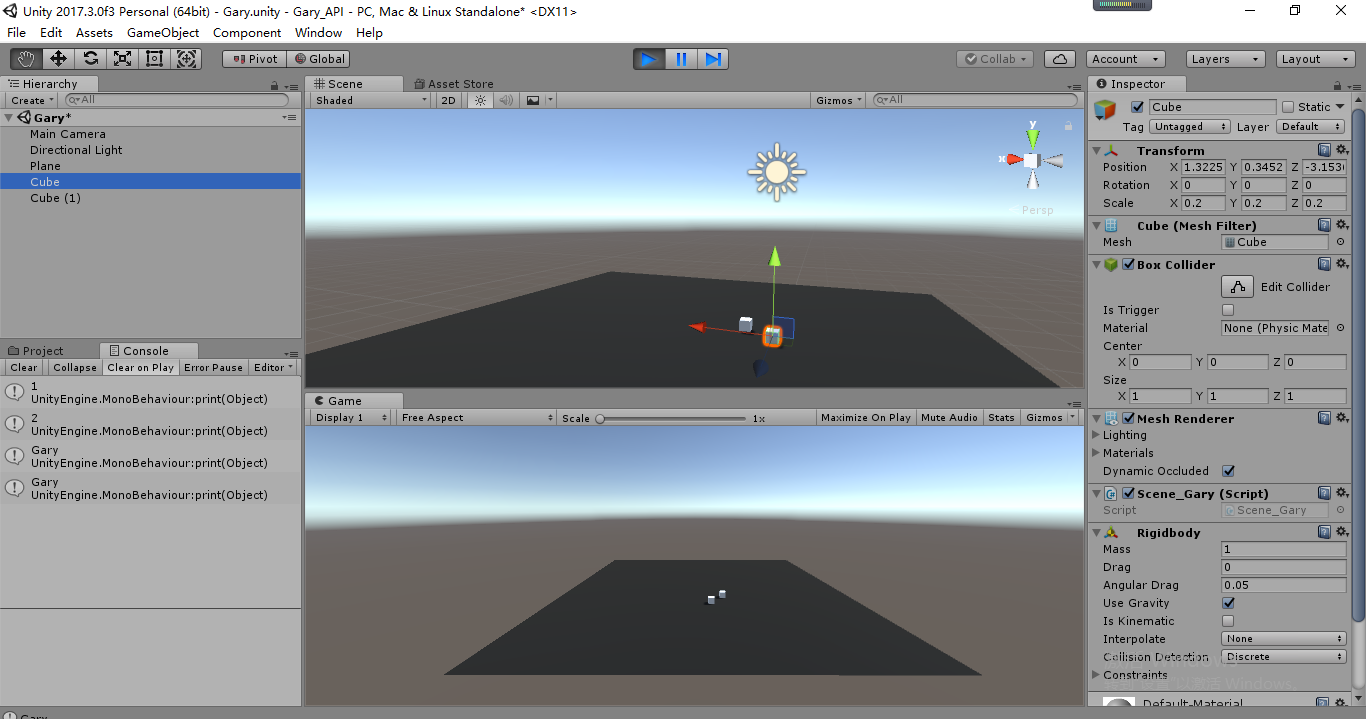
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement; public class Scene_Gary : MonoBehaviour { // Use this for initialization
void Start () {
//获得当前场景中的数量
print(SceneManager.sceneCount);
//获得BuildSettings中的名字
print(SceneManager.sceneCountInBuildSettings); //获得当前激活场景名字
print(SceneManager.GetActiveScene().name);
print(SceneManager.GetSceneAt().name); SceneManager.activeSceneChanged += OnActiveSceneChanged;
SceneManager.sceneLoaded += OnSceneLoaded;
} void OnActiveSceneChanged(Scene a, Scene b)
{
print(a.name);
print(b.name);
} void OnSceneLoaded(Scene a ,LoadSceneMode mode)
{
print(a.name+" "+mode);
} // Update is called once per frame
void Update () {
if (Input.GetKeyDown(KeyCode.Space))
{
//SceneManager.LoadScene(0);
SceneManager.LoadScene(SceneManager.GetSceneByBuildIndex().name);
}
}
}
Scene_Gary.cs
//获得当前场景中的数量
print(SceneManager.sceneCount);
//获得BuildSettings中的名字
print(SceneManager.sceneCountInBuildSettings); //获得当前激活场景名字
print(SceneManager.GetActiveScene().name);
print(SceneManager.GetSceneAt().name); SceneManager.activeSceneChanged += OnActiveSceneChanged;
SceneManager.sceneLoaded += OnSceneLoaded;
void OnActiveSceneChanged(Scene a, Scene b)
{
print(a.name);
print(b.name);
} void OnSceneLoaded(Scene a ,LoadSceneMode mode)
{
print(a.name+" "+mode);
}
//重新加载场景
//SceneManager.LoadScene(0);
SceneManager.LoadScene(SceneManager.GetSceneByBuildIndex().name);
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement; public class Scene_Gary : MonoBehaviour { // Use this for initialization
void Start () {
SceneManager.sceneLoaded += this.OnSceneLoader;
} //当场景加载出来调用触发的事件
void OnSceneLoader(Scene scene,LoadSceneMode mode)
{
Debug.Log("Hello Gary~");
} // Update is called once per frame
void Update () {
//加载场景
SceneManager.LoadScene("Gary2");
//获得当前场景
Scene scene = SceneManager.GetActiveScene();
//重新加载当前场景
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
Scene_Gary.cs
void Start () {
//将OnSceneLoader()方法添加到场景管理类
SceneManager.sceneLoaded += this.OnSceneLoader;
} //当场景加载出来调用触发的事件
void OnSceneLoader(Scene scene,LoadSceneMode mode)
{
Debug.Log("Hello Gary~");
}
CreateScene | Create an empty new Scene at runtime with the given name. |
GetActiveScene | Gets the currently active Scene. |
GetSceneAt | Get the Scene at index in the SceneManager's list of loaded Scenes. |
GetSceneByBuildIndex | Get a Scene struct from a build index. |
GetSceneByName | Searches through the Scenes loaded for a Scene with the given name. |
GetSceneByPath | Searches all Scenes loaded for a Scene that has the given asset path. |
LoadScene | Loads the Scene by its name or index in Build Settings. |
LoadSceneAsync | Loads the Scene asynchronously in the background. |
MergeScenes | This will merge the source Scene into the destinationScene. |
MoveGameObjectToScene | Move a GameObject from its current Scene to a new Scene. |
SetActiveScene | Set the Scene to be active. |
UnloadSceneAsync | Destroys all GameObjects associated with the given Scene and removes the Scene from the SceneManager. |
场景构建
File->Build Settings
将场景放到Scenes In Build中,默认第一个场景index下标是0
按下空格,加载下标为0的场景(Gary.scene)
通过OnSceneLoader()方法,触发加载场景触发的事件
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.SceneManagement; public class Scene_Gary : MonoBehaviour { // Use this for initialization
void Start () {
//将OnSceneLoader()方法添加到场景管理类
SceneManager.sceneLoaded += this.OnSceneLoader;
} //当场景加载出来调用触发的事件
void OnSceneLoader(Scene scene,LoadSceneMode mode)
{
Debug.Log("Hello Gary~");
} // Update is called once per frame
void Update () {
if (Input.GetKeyDown(KeyCode.Space))
{
SceneManager.LoadScene();
}
}
}
Scene_Gary.cs
Unity3D_(API)场景切换SceneManager的更多相关文章
- Unity 之 场景切换
Application.LoadLevel();//场景名称或索引,删除掉原场景的所有东西 Application.LoadLevelAdditive()//添加并加载场景,不删除当前场景的物体, ...
- Unity 中场景切换
Unity游戏开发中,单个Scene解决所有问题似乎不可能,那么多个Scene之间的切换是必然存在.如果仅仅是切换,似乎什么都好说,但是在场景比较大的时候不想让玩家等待加载或者说场景与场景之间想通过一 ...
- 动画间隔AnimationInterval 场景切换、图层叠加
从这一个月的学习进度上来看算比较慢的了,从开始学习C++到初试cocos,这也是我做过的比较大的决定,从工作中里挤出时间来玩玩自己喜欢的游戏开发也是一件非常幸福的事情,虽然现在对cocos的了解还只是 ...
- unity 之 场景切换进度条显示
一.UI.建立slider适当更改即可: 二.新增loadScene脚本,用来进行场景切换,将其绑定任意物体上面.博主以放置主相机为例.参数分别为进度条(用来设置value值),显示进度文本text: ...
- 【Cocos2d-x 3.x】 场景切换生命周期、背景音乐播放和场景切换原理与源码分析
大部分游戏里有很多个场景,场景之间需要切换,有时候切换的时候会进行背景音乐的播放和停止,因此对这块内容进行了总结. 场景切换生命周期 场景切换用到的函数: bool Setting::init() { ...
- texturepacker打包图片,场景切换时背景图有黑边
在使用TexturePacker打包图片之后,背景图在场景切换(有切换动画)时,明显能看到有黑边,在百度之后解决了. 知乎上边有网友贴出了两种解决方法,我抄过来如下: 第一种: 修改 ccConfig ...
- cocos2d-x 帧循环不严谨造成场景切换卡顿
最近在用cocos2d-x做引导界面,2dx版本是2.2.3,场景切换加上了效果,所有资源都已经使用texturepacker打包预加载,但是在实际运行调试中,场景切换相当卡顿. 各种纠结后,无意中将 ...
- 自制Unity小游戏TankHero-2D(5)声音+爆炸+场景切换+武器弹药
自制Unity小游戏TankHero-2D(5)声音+爆炸+场景切换+武器弹药 我在做这样一个坦克游戏,是仿照(http://game.kid.qq.com/a/20140221/028931.htm ...
- JavaScript强化教程 -- cocosjs场景切换
场景切换 在main.js,将StartScene作为我们初始化运行的场景,代码如下: cc.LoaderScene.preload(g_resources, function () { cc.dir ...
随机推荐
- 正则爬取某段子网站前20页段子(request库)
首先还是谷歌浏览器抓包对该网站数据进行分析,结果如下: 该网站地址:http://www.budejie.com/text 该网站数据都是通过html页面进行展示,网站url默认为第一页,http:/ ...
- Java中创建的对象多了,必然影响内存和性能
1, Java中创建的对象多了,必然影响内存和性能,所以对象的创建越少越好,最后还要记得销毁.
- CSS(下)
目录 CSS(下) CSS属性相关 宽和高 字体属性 背景属性 边框 border-radius display属性 CSS盒子模型 margin外边距 padding内填充 浮动(float) 限制 ...
- iis 8.0 HTTP 错误 404.3 server 2012
最近在学习WCF,发现将网站WCF服务放到IIS上时不能正常运行,从网上搜了一下: 解决方法,以管理员身份进入命令行模式,运行: "%windir%\Microsoft.NET\Framew ...
- SpringMVC整体架构
总结: 1. 用户发起请求到前端控制器(DispatchServlet): 2. 前端控制器没有处理业务逻辑的能力,需要找到具体的模型对象处理(Handler),到处理器映射器中查找Handler对象 ...
- CSS---解决文本溢出,换行
当我们设置我的的div,或者其它文本框固定宽度之后,文本内容过多就会出文本溢出(显示在区域外面,不换行的情况). 这时我们可以使用Css中的几个属于来解.有以下的三个属于可以解决问题: 1,word- ...
- 01 Linux常用基本命令(一)
1.远程连接服务器 Xshell为例: ssh 用户名@IP地址 (ssh root@192.168.119.139) 查看服务器的IP地址: ifconfig (ip addr) 2.命令 1.ls ...
- 基于Zabbix 3.2.6版本的low-level-discover(lld)
个人使用理解: 1.使用一个返回值是JSON的KEY,在Templates或者Hosts中创建一个Discovery规则.该key的返回值类似于: 索引key -- value 类型 ...
- AngularJs 初级入门 学习笔记
刚学angular, 做一些笔记方便自己翻看. ng-app: 填写模块的名称 ng-init: 初始化数据(一般通过控制器初始化) ng-model: 填写数据模型 ng-bind: 绑定数据模型, ...
- 织梦多个栏目arclist调用副栏目不显示的解决办法
织梦arclist调用副栏目不显示,网上关于这个问题的解决办法有很多,其中一种是: 打开/include/taglib/arclist.lib.php,代码约位于295-296行(我目前用的DedeC ...