面试题:Concurrenthashmap原理分析 有用
一、背景:
线程不安全的HashMap
效率低下的HashTable容器
锁分段技术
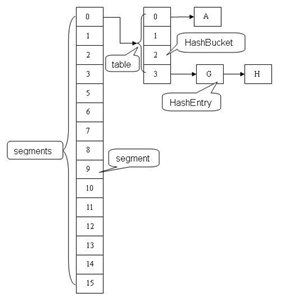
二、应用场景
三、源码解读
- /**
- * The segments, each of which is a specialized hash table
- */
- final Segment<K,V>[] segments;
不变(Immutable)和易变(Volatile)
- static final class HashEntry<K,V> {
- final K key;
- final int hash;
- volatile V value;
- final HashEntry<K,V> next;
- }
其它
定位操作:
- final Segment<K,V> segmentFor(int hash) {
- return segments[(hash >>> segmentShift) & segmentMask];
- }
- final Segment<K,V> segmentFor(int hash) {
- return segments[(hash >>> segmentShift) & segmentMask];
- }
数据结构

- static final class Segment<K,V> extends ReentrantLock implements Serializable {
- /**
- * The number of elements in this segment's region.
- */
- transient volatileint count;
- /**
- * Number of updates that alter the size of the table. This is
- * used during bulk-read methods to make sure they see a
- * consistent snapshot: If modCounts change during a traversal
- * of segments computing size or checking containsValue, then
- * we might have an inconsistent view of state so (usually)
- * must retry.
- */
- transient int modCount;
- /**
- * The table is rehashed when its size exceeds this threshold.
- * (The value of this field is always <tt>(int)(capacity *
- * loadFactor)</tt>.)
- */
- transient int threshold;
- /**
- * The per-segment table.
- */
- transient volatile HashEntry<K,V>[] table;
- /**
- * The load factor for the hash table. Even though this value
- * is same for all segments, it is replicated to avoid needing
- * links to outer object.
- * @serial
- */
- final float loadFactor;
- }

删除操作remove(key)
- public V remove(Object key) {
- hash = hash(key.hashCode());
- return segmentFor(hash).remove(key, hash, null);
- }

- V remove(Object key, int hash, Object value) {
- lock();
- try {
- int c = count - 1;
- HashEntry<K,V>[] tab = table;
- int index = hash & (tab.length - 1);
- HashEntry<K,V> first = tab[index];
- HashEntry<K,V> e = first;
- while (e != null && (e.hash != hash || !key.equals(e.key)))
- e = e.next;
- V oldValue = null;
- if (e != null) {
- V v = e.value;
- if (value == null || value.equals(v)) {
- oldValue = v;
- // All entries following removed node can stay
- // in list, but all preceding ones need to be
- // cloned.
- ++modCount;
- HashEntry<K,V> newFirst = e.next;
- *for (HashEntry<K,V> p = first; p != e; p = p.next)
- *newFirst = new HashEntry<K,V>(p.key, p.hash,
- newFirst, p.value);
- tab[index] = newFirst;
- count = c; // write-volatile
- }
- }
- return oldValue;
- } finally {
- unlock();
- }
- }


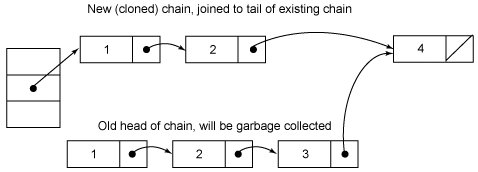
get操作

- V get(Object key, int hash) {
- if (count != 0) { // read-volatile 当前桶的数据个数是否为0
- HashEntry<K,V> e = getFirst(hash); 得到头节点
- while (e != null) {
- if (e.hash == hash && key.equals(e.key)) {
- V v = e.value;
- if (v != null)
- return v;
- return readValueUnderLock(e); // recheck
- }
- e = e.next;
- }
- }
- returnnull;
- }


- V readValueUnderLock(HashEntry<K,V> e) {
- lock();
- try {
- return e.value;
- } finally {
- unlock();
- }
- }

put操作

- V put(K key, int hash, V value, boolean onlyIfAbsent) {
- lock();
- try {
- int c = count;
- if (c++ > threshold) // ensure capacity
- rehash();
- HashEntry<K,V>[] tab = table;
- int index = hash & (tab.length - 1);
- HashEntry<K,V> first = tab[index];
- HashEntry<K,V> e = first;
- while (e != null && (e.hash != hash || !key.equals(e.key)))
- e = e.next;
- V oldValue;
- if (e != null) {
- oldValue = e.value;
- if (!onlyIfAbsent)
- e.value = value;
- }
- else {
- oldValue = null;
- ++modCount;
- tab[index] = new HashEntry<K,V>(key, hash, first, value);
- count = c; // write-volatile
- }
- return oldValue;
- } finally {
- unlock();
- }
- }

- \old),如果超过阀值,数组进行扩容。值得一提的是,Segment的扩容判断比HashMap更恰当,因为HashMap是在插入元素后判断元素是否已经到达容量的,如果到达了就进行扩容,但是很有可能扩容之后没有新元素插入,这时HashMap就进行了一次无效的扩容。
- Concurrenthashmap如何扩容。扩容的时候首先会创建一个两倍于原容量的数组,然后将原数组里的元素进行再hash后插入到新的数组里。为了高效ConcurrentHashMap不会对整个容器进行扩容,而只对某个segment进行扩容。

- boolean containsKey(Object key, int hash) {
- if (count != 0) { // read-volatile
- HashEntry<K,V> e = getFirst(hash);
- while (e != null) {
- if (e.hash == hash && key.equals(e.key))
- returntrue;
- e = e.next;
- }
- }
- returnfalse;
- }

size()操作
面试题:Concurrenthashmap原理分析 有用的更多相关文章
- ConcurrentHashMap原理分析(1.7与1.8)-put和 get 需要执行两次Hash
ConcurrentHashMap 与HashMap和Hashtable 最大的不同在于:put和 get 两次Hash到达指定的HashEntry,第一次hash到达Segment,第二次到达Seg ...
- 干货 MySQL常见的面试题 + 索引原理分析
常见的面试必备之MySQL索引底层原理分析: MySQL索引的本质 MySQL索引的底层原理 MySQL索引的实战经验 面试 1)问题:数据库中最常见的慢查询优化方式是什么? 回答:加索引 2)问题: ...
- [转载] ConcurrentHashMap原理分析
转载自http://blog.csdn.net/liuzhengkang/article/details/2916620 集合是编程中最常用的数据结构.而谈到并发,几乎总是离不开集合这类高级数据结构的 ...
- Java集合:ConcurrentHashMap原理分析
集合是编程中最常用的数据结构.而谈到并发,几乎总是离不开集合这类高级数据结构的支持.比如两个线程需要同时访问一个中间临界区(Queue),比如常会用缓存作为外部文件的副本(HashMap).这篇文章主 ...
- 【Java并发编程】1、ConcurrentHashMap原理分析
集合是编程中最常用的数据结构.而谈到并发,几乎总是离不开集合这类高级数据结构的支持.比如两个线程需要同时访问一个中间临界区(Queue),比如常会用缓存作为外部文件的副本(HashMap).这篇文章主 ...
- Java 中 ConcurrentHashMap 原理分析
一.Java并发基础 当一个对象或变量可以被多个线程共享的时候,就有可能使得程序的逻辑出现问题. 在一个对象中有一个变量i=0,有两个线程A,B都想对i加1,这个时候便有问题显现出来,关键就是对i加1 ...
- ConcurrentHashMap原理分析(二)-扩容
概述 在上一篇文章中介绍了ConcurrentHashMap的存储结构,以及put和get方法,那本篇文章就介绍一下其扩容原理.其实说到扩容,无非就是新建一个数组,然后把旧的数组中的数据拷贝到新的数组 ...
- ConcurrentHashMap原理分析
当我们享受着jdk带来的便利时同样承受它带来的不幸恶果.通过分析Hashtable就知道,synchronized是针对整张Hash表的,即每次锁住整张表让线程独占,安全的背后是巨大的浪费,而现在的解 ...
- ConcurrentHashMap 原理分析
1 为什么有ConcurrentHashMap hashmap是非线程安全的,hashtable是线程安全的,但是所有的写和读方法都有synchronized,所以同一时间只有一个线程可以持有对象,多 ...
随机推荐
- python sort() sorted() 与argsort()函数的区别
1.python的内建排序函数有 sort.sorted两个 sort函数只定义在list中,sorted函数对于所有的可迭代序列都可以定义. for example: ls = list([5, 2 ...
- python_判断字符串编码的方法
1. 安装chardet 在命令行中,进入Python27\Scripts目录,输入以下的命令:easy_install chardet 2. 操作 import chardet f = open(' ...
- Codeforces Round #286 (Div. 2)A. Mr. Kitayuta's Gift(暴力,string的应用)
由于字符串的长度很短,所以就暴力枚举每一个空每一个字母,出现行的就输出.这么简单的思路我居然没想到,临场想了很多,以为有什么技巧,越想越迷...是思维方式有问题,遇到问题先分析最简单粗暴的办法,然后一 ...
- Express+Mongoose(MongoDB)+Vue2全栈微信商城项目全记录(一)
最近用vue2做了一个微信商城项目,因为做的比较仓促,所以一边写一下整个流程,一边稍做优化. 项目github地址:https://github.com/seven9115/vue-fullstack ...
- bzoj 3681 Arietta
一棵有根树,每个点有一个音高,有 $m$ 中弹奏方法,每种方法可以弹奏 $d$ 子树中音高在 $[l,r]$ 间的音符,每种方法最多弹 $t$ 次 求最多能弹出多少个音符 $n \leq 10000$ ...
- BerOS file system
The new operating system BerOS has a nice feature. It is possible to use any number of characters '/ ...
- swing之UI选择文件
package gui1; import java.awt.Container; import java.awt.FlowLayout; import java.awt.event.ActionEve ...
- RabbitMQ教程总结
[译]RabbitMQ教程一 主要通过Hello Word对RabbitMQ有初步认识 [译]RabbitMQ教程二 工作队列,即一个生产者对多个消费者 循环分发.消息确认.消息持久.公平分发 [译] ...
- 修改Linux安装软件镜像源为阿里云
CentOS系统更换软件安装源: 第一步:安装wget.如果你的系统已安装了wget可以直接跳到下一步. [root@local~]#yum install wget 第二步:备份你的原镜像文件,避免 ...
- VC 6.0下载 VC 6.0英文版下载 Visual C++ 6.0 英文企业版 集成SP6完美版(最新更新地址,百度网盘)
下载地址1:Visual.C++.6.EN 下载地址2:Visual.C++.6.EN 更新下载地址可用(百度网盘)Visual.C++.6.EN 转载请注明出处,有技术问题,欢迎互相交流,或者留言.