poj3122 binary search 实数区间
Time Limit: 1000MS | Memory Limit: 65536K | |||
Total Submissions: 14536 | Accepted: 4979 | Special Judge |
Description
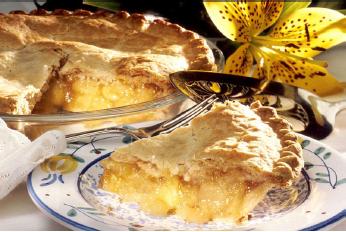
My friends are very annoying and if one of them gets a bigger piece than the others, they start complaining. Therefore all of them should get equally sized (but not necessarily equally shaped) pieces, even if this leads to some pie getting spoiled (which is better than spoiling the party). Of course, I want a piece of pie for myself too, and that piece should also be of the same size.
What is the largest possible piece size all of us can get? All the pies are cylindrical in shape and they all have the same height 1, but the radii of the pies can be different.
Input
- One line with two integers N and F with 1 ≤ N, F ≤ 10 000: the number of pies and the number of friends.
- One line with N integers ri with 1 ≤ ri ≤ 10 000: the radii of the pies.
Output
Sample Input
3
3 3
4 3 3
1 24
5
10 5
1 4 2 3 4 5 6 5 4 2
Sample Output
25.1327
3.1416
50.2655
题目大意:国人很多事情都追求公平,分饼也是如此,现在这里有n个饼,每一个饼都可以看做一个圆柱体,高都是1,但是半径不同,
每一个人都可以分到某个饼的一部分,但是只能要一部分,而不能要好几块饼,最终结果必须保证每个人分到的饼的体积(面积)相等,
问你每个人能够获得的饼的最大面积是多少。
思路分析:首先数据量很大,如果用暴力枚举肯定会超时,很显然我们应该采用二分逼近的方法来确定答案,但是在实际操作的时候还是
出了一些问题,首先,二分精度不能够太高,1e-5就可以,精度太高会超时,其次,关于π,如果写做3.1415926会被精度卡掉,将pi
定义为acos(-1.0)就可以了。
代码:
#include<map>
#include<queue>
#include<stack>
#include<cmath>
#include<cstdio>
#include<vector>
#include<string>
#include<cstdlib>
#include<cstring>
#include<climits>
#include<iostream>
#include<algorithm>
#include <cmath>
#define LL long long
using namespace std;
const int maxn=10000+100;
const double pi=acos(-1.0);
double a[maxn];
int n,f;
double s(double r)
{
return pi*r*r;
}
bool check(double x)
{
int t=0;
for(int i=0;i<n;i++)
{
double p=s(a[i]);
while(p>=x)
{
p-=x;
t++;
if(t>=f+1) return true;
}
}
return false;
}
int main()
{
int T;
scanf("%d", &T);
while(T--)
{
double sum=0.0;
scanf("%d%d",&n,&f);
for(int i=0;i<n;i++)
{
scanf("%lf",&a[i]);
sum+=s(a[i]);
}
sort(a,a+n);
double l=0.0,r=sum/(f+1)*1.0;
double ans=0;
while(l+0.000001<=r)
{
double mid=(l+r)/2;
if(check(mid)) ans=mid,l=mid;
else r=mid;
}
printf("%.4lf\n",ans);
}
return 0;
}
poj3122 binary search 实数区间的更多相关文章
- uva 10304 - Optimal Binary Search Tree 区间dp
题目链接 给n个数, 这n个数的值是从小到大的, 给出个n个数的出现次数. 然后用他们组成一个bst.访问每一个数的代价是这个点的深度*这个点访问的次数. 问你代价最小值是多少. 区间dp的时候, 如 ...
- Binary Search
Binary Search [原文见:http://www.topcoder.com/tc?module=Static&d1=tuto ...
- Algo: Binary search
二分查找的基本写法: #include <vector> #include <iostream> int binarySearch(std::vector<int> ...
- [数据结构]——二叉树(Binary Tree)、二叉搜索树(Binary Search Tree)及其衍生算法
二叉树(Binary Tree)是最简单的树形数据结构,然而却十分精妙.其衍生出各种算法,以致于占据了数据结构的半壁江山.STL中大名顶顶的关联容器--集合(set).映射(map)便是使用二叉树实现 ...
- [LeetCode] Verify Preorder Sequence in Binary Search Tree 验证二叉搜索树的先序序列
Given an array of numbers, verify whether it is the correct preorder traversal sequence of a binary ...
- [LeetCode] Unique Binary Search Trees II 独一无二的二叉搜索树之二
Given n, generate all structurally unique BST's (binary search trees) that store values 1...n. For e ...
- 【转】STL之二分查找 (Binary search in STL)
Section I正确区分不同的查找算法count,find,binary_search,lower_bound,upper_bound,equal_range 本文是对Effective STL第4 ...
- STL之二分查找 (Binary search in STL)
STL之二分查找 (Binary search in STL) Section I正确区分不同的查找算法count,find,binary_search,lower_bound,upper_bound ...
- 九章算法系列(#2 Binary Search)-课堂笔记
前言 先说一些题外的东西吧.受到春跃大神的影响和启发,推荐了这个算法公开课给我,晚上睡觉前点开一看发现课还有两天要开始,本着要好好系统地学习一下算法,于是就爬起来拉上两个小伙伴组团报名了.今天听了第一 ...
随机推荐
- SVN搭建本地版本控制仓库
1.安装TortoiseSVN 2.新建一个文件夹,比如F:\SvnProjectsCfg 3.在F:\SvnProjectsCfg新建一个文件夹project1,右键该文件夹选择“create re ...
- 在iis中调试asp.net程序
第一步,在iis中新建一个网站,名称为Langben,“物理路径”选择你的程序的根目录,端口你可以随便设置一个数,我这里设置为8888(后面要用到哦). 第二步,应用程序池设置一下 第三步,接下来,在 ...
- 解决jQuery中美元符号($)命名与别的js脚本库引用冲突方法
在Jquery中,$是JQuery的别名,所有使用$的地方也都可以使用JQuery来替换,如$('#msg')等同于JQuery('#msg') 的写法.然而,当我们引入多个js库后,在另外一个js库 ...
- C++第三课(2013.10.03 )
函数的默认参数: 1.函数的默认参数必须放在形参的右边而且在默认形参的右边不能出现没有无默认参数的形参 2.如果函数的声明给出了默认的参数,那么函数的实现就不能定义默认参 3.声明成员函数时没有给出默 ...
- php用get_meta_tags轻松获取网页的meta信息
之前没发现php还有这个函数,get_meta_tags()直接就可以获取文件中meta标签的属性值,返回数组: <?php $metas = get_meta_tags('http://www ...
- Quick Sort In-place Implementation
在线运行PHP http://www.compileonline.com/execute_php_online.php <?php function swap( &$a, &$b ...
- epoll函数及三种I/O复用函数的对比
epoll函数 #include <sys/epoll.h>int epoll_create(int size)int epoll_ctl(int epfd, int op, int fd ...
- 主题模型-LDA浅析
(一)LDA作用 传统判断两个文档相似性的方法是通过查看两个文档共同出现的单词的多少,如TF-IDF等,这种方法没有考虑到文字背后的语义关联,可能在两个文档共同出现的单词很少甚至没有,但两个文档是相似 ...
- xdebug及webgrind的联用
参考URL: http://www.tuicool.com/articles/ERFNva http://blog.sina.com.cn/s/blog_635833b3010127q5.html h ...
- mysql sql优化<1>
<pre name="code" class="html">explain SELECT t.* FROM ( SELECT t1.sn AS cl ...