poj2299 Ultra-QuickSort(线段树求逆序对)
Description
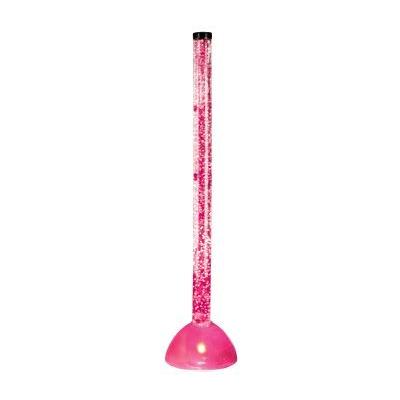
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
Output
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
题意:有一种排序,规则为如果相邻两数左比右大就交换他们,求最小交换次数?
题解:显然最小次数为逆序对数,至于逆序对,可以归并排序求,也可以树状数组/线段树求,自然是选择简单的喽!
代码如下:
#include<queue>
#include<string>
#include<cstdio>
#include<cstring>
#include<iostream>
#include<algorithm>
#define lson root<<1
#define rson root<<1|1
#define hi puts("hi!");
using namespace std; struct node
{
int kd,val;
}a[]; int n,m,cnt[];
long long tr[]; bool cmp(node a,node b)
{
return a.val<b.val;
} void push_up(int root)
{
tr[root]=tr[lson]+tr[rson];
} void build(int root,int l,int r)
{
if(l==r)
{
tr[root]=;
return;
}
int mid=(l+r)>>;
build(lson,l,mid);
build(rson,mid+,r);
push_up(root);
} void add(int root,int l,int r,int x,int p)
{ if(l==r)
{
tr[root]=;
return;
}
int mid=(l+r)>>;
if(p<=mid)
{
add(lson,l,mid,x,p);
}
if(p>mid)
{
add(rson,mid+,r,x,p);
}
push_up(root);
} long long query(int root,int l,int r,int x,int y)
{
long long ans=;
if(x<=l&&y>=r)
{
return tr[root];
}
int mid=(l+r)>>;
if(x<=mid)
{
ans+=query(lson,l,mid,x,y);
}
if(y>mid)
{
ans+=query(rson,mid+,r,x,y);
}
return ans;
} int main()
{
while(scanf("%d",&n)==&&n)
{
long long ans1=;
memset(tr,,sizeof(tr));
build(,,n);
for(int i=;i<=n;i++)
{
scanf("%d",&a[i].val);
a[i].kd=i;
}
sort(a+,a+n+,cmp);
for(int i=;i<=n;i++)
{
cnt[a[i].kd]=i;
}
for(int i=n;i>=;i--)
{
ans1+=query(,,n,,cnt[i]);
add(,,n,,cnt[i]);
}
printf("%lld\n",ans1);
}
}
poj2299 Ultra-QuickSort(线段树求逆序对)的更多相关文章
- 4163 hzwer与逆序对 (codevs + 权值线段树 + 求逆序对)
题目链接:http://codevs.cn/problem/4163/ 题目:
- BNU 2418 Ultra-QuickSort (线段树求逆序对)
题目链接:http://acm.bnu.edu.cn/bnuoj/problem_show.php?pid=2418 解题报告:就是给你n个数,然后让你求这个数列的逆序对是多少?题目中n的范围是n & ...
- HDU 4911 http://acm.hdu.edu.cn/showproblem.php?pid=4911(线段树求逆序对)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=4911 解题报告: 给出一个长度为n的序列,然后给出一个k,要你求最多做k次相邻的数字交换后,逆序数最少 ...
- SGU 180 Inversions(离散化 + 线段树求逆序对)
题目链接:http://acm.sgu.ru/problem.php?contest=0&problem=180 解题报告:一个裸的求逆序对的题,离散化+线段树,也可以用离散化+树状数组.因为 ...
- hdu1394(线段树求逆序对)
题目连接:http://acm.hdu.edu.cn/showproblem.php?pid=1394 线段树功能:update:单点增减 query:区间求和 分析:如果是0到n-1的排列,那么如果 ...
- HDU 1394 线段树求逆序对
Minimum Inversion Number Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java ...
- FZU2018级算法第五次作业 m_sort(归并排序或线段树求逆序对)
首先对某人在未经冰少允许情况下登录冰少账号原模原样复制其代码并且直接提交的赤裸裸剽窃行为,并且最终被评为优秀作业提出抗议! 题目大意: 给一个数组含n个数(1<=n<=5e5),求使用冒泡 ...
- POJ 2188线段树求逆序对
题目给的输入是大坑,算法倒是很简单-- 输入的是绳子的编号wire ID,而不是上(或下)挂钩对应下(或上)挂钩的编号. 所以要转换编号,转换成挂钩的顺序,然后再求逆序数. 知道了这个以后直接乱搞就可 ...
- HDU 1394 Minimum Inversion Number(线段树求逆序对)
题目链接: http://acm.hdu.edu.cn/showproblem.php?pid=1394 解题报告:给出一个序列,求出这个序列的逆序数,然后依次将第一个数移动到最后一位,求在这个过程中 ...
随机推荐
- (转)TextView 设置背景和文本颜色的问题
在做一个项目,突然遇到如下问题 比如:在color.xml中定义了几个颜色 <color name="white">#FFFFFF</color> < ...
- 解决spring 事务管理默认不支持SQLException等运行时异常
公司同事在定位一个bug时,发现spring默认的事务只支持运行时异常的回滚,对于像SQLException这样的非运行时异常,默认的事务机制不能处理,于是找了下解决的办法: 1.在捕获SQLE ...
- mybatis+druid+springboot 注解方式配置多个数据源
1\数据库配置 #test数据源 spring.datasource.test.url=jdbc:mysql:///db?useUnicode= spring.datasource.test.user ...
- OpenLiveWriter 这篇文章使用博客客户端撰写
OpenLiveWriter是非常方便的博客客户端,起码相比在浏览器写博客多了一种选择.而且借助于MetaWeblog接口,可以很方便地同步博客文章到多个博客地址.本站cms.xlongwei.com ...
- ExcelHandle
using System.Collections.Generic; using System.Data; using System.IO; using System.Linq; using Syste ...
- FTP协议完全详解
1. 介绍 FTP的目标是提高文件的共享性,提供非直接使用远程计算机,使存储介质对用户透明和可靠高效地传送数据.虽然我们也可以手工使用它,但是它的主要作用是供程序使用的.在阅读本文之前最好能够阅读TC ...
- 分享一道阿里巴巴(蚂蚁金服)Java笔试题
编写一个函数验证一个给定的9x9 整数矩阵是否符合数独的特性:a) 每个单元格数字为 1-9b) 每行的9个数不重复c) 每列的9个数不重复d) 如图中分割的9个小3x3矩阵,每个小矩阵里9个数不重复 ...
- 1107 Social Clusters
题意:给出n个人(编号为1~n)以及每个人的若干个爱好,把有一个或多个共同爱好的人归为一个集合,问共有多少个集合,每个集合里有多少个人? 思路:典型的并查集题目.并查集的模板init()函数,unio ...
- VC++使用TCHAR
#ifdef _UNICODE #define tcout wcout #define tcin wcin #else #define tcout cout #define tcin cin #end ...
- JeeSite基础知识(一)