Examples For PLSQL Cursors - Explicit, Implicit And Ref Cursors
Explicit Cursors
Implicit Cursors
Ref Cursors
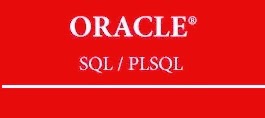
Examples For PLSQL Cursors - Explicit, Implicit And Ref Cursors的更多相关文章
- C#类型转换运算符之 explicit implicit
类型转换运算符 explicit和implicit用于声明用户定义的类型转换运算符,如果可以确保转换过程不会造成数据丢失,则可使用这两个关键字在用户定义的类型和其他类型之间进行转换. explicit ...
- Oracle PLSQL Demo - 20.弱类型REF游标[没有指定查询类型,也不指定返回类型]
declare Type ref_cur_variable IS REF cursor; cur_variable ref_cur_variable; v_ename ); v_deptno ); v ...
- Oracle PLSQL Demo - 16.弱类型REF游标[没有指定查询类型,已指定返回类型]
declare Type ref_cur_variable IS REF cursor; cur_variable ref_cur_variable; rec_emp scott.emp%RowTyp ...
- On Caching and Evangelizing SQL
http://www.oracle.com/technetwork/issue-archive/2011/11-sep/o51asktom-453438.html Our technologist ...
- C#中的Explicit和Implicit
今天在Review一个老项目的时候,看到一段奇怪的代码. if (dto.Payment == null) continue; var entity = entries.FirstOrDefault( ...
- Converting REF CURSOR to PIPE for Performance in PHP OCI8 and PDO_OCI
原文地址:https://blogs.oracle.com/opal/entry/converting_ref_cursor_to_pipe REF CURSORs are common in Ora ...
- Oracle PL/SQL Articles
我是搬运工....http://www.oracle-base.com/articles/plsql/articles-plsql.php Oracle 8i Oracle 9i Oracle 10g ...
- 游标-Oracle游标汇总
游标(Cursor):用来查询数据库,获取记录集合(结果集)的指针,可以让开发者一次访问一行结果集,在每条结果集上作操作. 游标可分为: <!--[if !supportLists] ...
- Miscellaneous Articles
标记一下,慢慢看 http://www.oracle-base.com/articles/misc/articles-misc.php Miscellaneous Articles DBA Deve ...
随机推荐
- 《OpenGL着色语言》理解点记录三
“帧缓冲区”中的“帧”的含义? “帧”是连续图像中的一幅,3D可视化程序最终都是转化为一幅幅的图像输出在显示器上,这一幅幅的图像叫做叫“帧”. 解释“glBlendFunc(GL_SRC_AL ...
- CSS 文字阴影(text-shadow)怎么用
textShadow="1px 1px 1px #ff0000" textShadow="水平位移 垂直位移 模糊半径 阴影颜色"
- Maven(一)
Maven学习总结(一)——Maven入门 一.Maven的基本概念 Maven(翻译为"专家","内行")是跨平台的项目管理工具.主要服务于基于Java平台的 ...
- PHPCMS V9 学习总结
在实现PHPCMS网站过程中,根据业务需求,我们遇到很多问题,特此总结如下,以便大家参考学习. [1]PHPCMS V9系统目录简析 在研究所有问题之前,请先了解一下系统的文件目录结构,具体如下图所示 ...
- STM32模拟I2C
之前为了测试, 拿最小板做了一个I2C的主发跟主读, 一开始当然是尝试用硬件I2C, 结果弄了很久, 时间紧迫, 只好用了模拟, 结果发现, 哎, 真特么挺好用的, 现在1片儿顶过去5片儿. 硬件I2 ...
- laravel5.1启动详解
laravel的启动过程 如果没有使用过类似Yii之类的框架,直接去看laravel,会有点一脸迷糊的感觉,起码我是这样的.laravel的启动过程,也是laravel的核心,对这个过程有一个了解,有 ...
- is_user_logged_in()
function is_user_logged_in() { $user = wp_get_current_user(); return $user->exists(); } wp_get_cu ...
- windows cmd color setup
设置颜色的话,一般可定会有foreground和background color设置:(其实color /?直接看一下就好了) Color Background Foreground Black 0 ...
- WIN7(VISTA)系统无法上网问题排查方法
WIN7(VISTA)系统无法上网问题排查方法 一.无法通过DHCP自动获取到IP 1. 确认正确配置路由器的DHCP功能 a.一般租期建议设置为1-3小时,推荐设置1小时. b.DHCP地址池不要和 ...
- WKWebView新特性及JS交互
引言 一直听说WKWebView比UIWebView强大许多,可是一直没有使用到,今天花了点时间看写了个例子,对其API的使用有所了解,为了日后能少走弯路,也为了让大家更容易学习上手,这里写下这篇文章 ...