2019 牛客多校第一场 F Random Point in Triangle
题目链接:https://ac.nowcoder.com/acm/contest/881/F
题目大意
给定二维平面上 3 个整数表示的点 A,B,C,在三角形 ABC 内随机选一点 P,求期望$E = max(S_{PAB}, S_{PAC}, S_{PBC})$。输出 36 * E。
分析
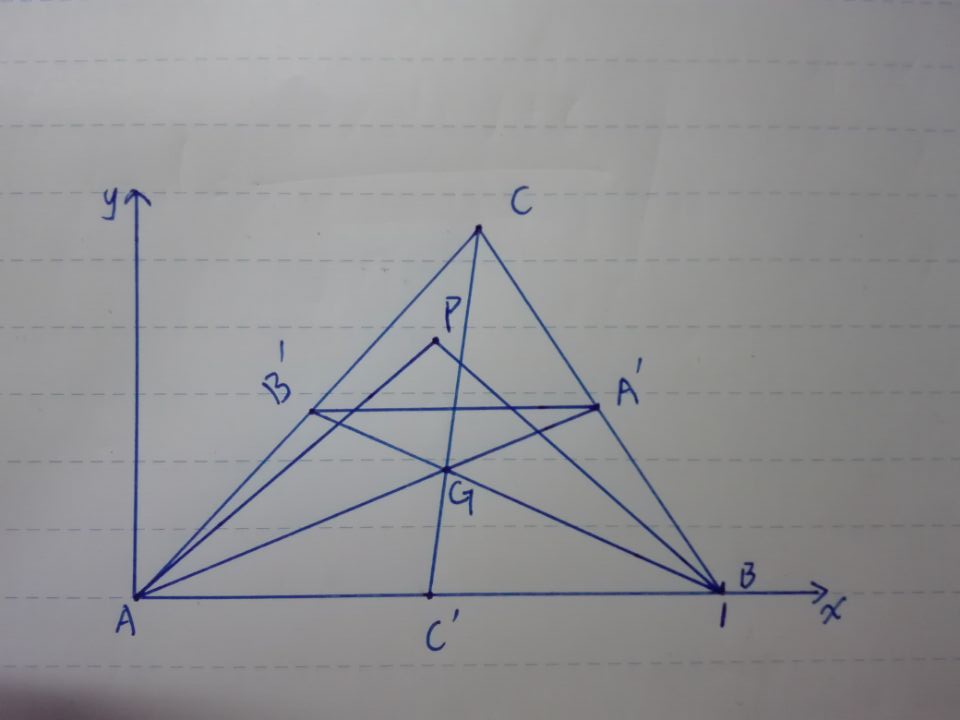
- G:$(\frac{a + 1}{3}, \frac{b}{3})$
- B':$(\frac{a}{2}, \frac{b}{2})$
- A':$(\frac{a + 1}{2}, \frac{b}{2})$
- B'A':$x = \frac{b}{2}$
- CA':$y = \frac{b}{a - 1}(x - 1)$
- CB':$y = \frac{b}{a}x$
- GB':$y = \frac{b}{a - 2}(x - 1)$
- GA':$y = \frac{b}{a + 1}x$
- $S_{PAB}$:$\frac{y}{2}$
- $S_{CB'GA'}$:$\frac{b}{6}$
- $S_{CB'A'}$:$\frac{b}{8}$
- $S_{GB'A'}$:$\frac{b}{24}$
于是$E = \frac{1}{S_{CB'GA'}} ((\int_{G_y}^{A'B'} dy \int_{GB'}^{GA'} S_{PAB} dx) + (\int_{A'B'}^{C_y} dy \int_{CB'}^{CA'} S_{PAB} dx))$
积出来得$E = \frac{11b}{36} = \frac{11}{18}S_{ABC}$
所以$36E = 22S_{ABC}$
代码如下
#include <bits/stdc++.h>
using namespace std; #define INIT() ios::sync_with_stdio(false);cin.tie(0);cout.tie(0);
#define Rep(i,n) for (int i = 0; i < (n); ++i)
#define For(i,s,t) for (int i = (s); i <= (t); ++i)
#define rFor(i,t,s) for (int i = (t); i >= (s); --i)
#define ForLL(i, s, t) for (LL i = LL(s); i <= LL(t); ++i)
#define rForLL(i, t, s) for (LL i = LL(t); i >= LL(s); --i)
#define foreach(i,c) for (__typeof(c.begin()) i = c.begin(); i != c.end(); ++i)
#define rforeach(i,c) for (__typeof(c.rbegin()) i = c.rbegin(); i != c.rend(); ++i) #define pr(x) cout << #x << " = " << x << " "
#define prln(x) cout << #x << " = " << x << endl #define LOWBIT(x) ((x)&(-x)) #define ALL(x) x.begin(),x.end()
#define INS(x) inserter(x,x.begin())
#define UNIQUE(x) x.erase(unique(x.begin(), x.end()), x.end())
#define REMOVE(x, c) x.erase(remove(x.begin(), x.end(), c), x.end()); // ?? x ?????? c
#define TOLOWER(x) transform(x.begin(), x.end(), x.begin(),::tolower);
#define TOUPPER(x) transform(x.begin(), x.end(), x.begin(),::toupper); #define ms0(a) memset(a,0,sizeof(a))
#define msI(a) memset(a,inf,sizeof(a))
#define msM(a) memset(a,-1,sizeof(a)) #define MP make_pair
#define PB push_back
#define ft first
#define sd second template<typename T1, typename T2>
istream &operator>>(istream &in, pair<T1, T2> &p) {
in >> p.first >> p.second;
return in;
} template<typename T>
istream &operator>>(istream &in, vector<T> &v) {
for (auto &x: v)
in >> x;
return in;
} template<typename T>
ostream &operator<<(ostream &out, vector<T> &v) {
Rep(i, v.size()) out << v[i] << " \n"[i == v.size()];
return out;
} template<typename T1, typename T2>
ostream &operator<<(ostream &out, const std::pair<T1, T2> &p) {
out << "[" << p.first << ", " << p.second << "]" << "\n";
return out;
} inline int gc(){
static const int BUF = 1e7;
static char buf[BUF], *bg = buf + BUF, *ed = bg; if(bg == ed) fread(bg = buf, , BUF, stdin);
return *bg++;
} inline int ri(){
int x = , f = , c = gc();
for(; c<||c>; f = c=='-'?-:f, c=gc());
for(; c>&&c<; x = x* + c - , c=gc());
return x*f;
} template<class T>
inline string toString(T x) {
ostringstream sout;
sout << x;
return sout.str();
} inline int toInt(string s) {
int v;
istringstream sin(s);
sin >> v;
return v;
} //min <= aim <= max
template<typename T>
inline bool BETWEEN(const T aim, const T min, const T max) {
return min <= aim && aim <= max;
} typedef long long LL;
typedef unsigned long long uLL;
typedef pair< double, double > PDD;
typedef pair< int, int > PII;
typedef pair< int, PII > PIPII;
typedef pair< string, int > PSI;
typedef pair< int, PSI > PIPSI;
typedef set< int > SI;
typedef set< PII > SPII;
typedef vector< int > VI;
typedef vector< double > VD;
typedef vector< VI > VVI;
typedef vector< SI > VSI;
typedef vector< PII > VPII;
typedef map< int, int > MII;
typedef map< int, string > MIS;
typedef map< int, PII > MIPII;
typedef map< PII, int > MPIII;
typedef map< string, int > MSI;
typedef map< string, string > MSS;
typedef map< PII, string > MPIIS;
typedef map< PII, PII > MPIIPII;
typedef multimap< int, int > MMII;
typedef multimap< string, int > MMSI;
//typedef unordered_map< int, int > uMII;
typedef pair< LL, LL > PLL;
typedef vector< LL > VL;
typedef vector< VL > VVL;
typedef priority_queue< int > PQIMax;
typedef priority_queue< int, VI, greater< int > > PQIMin;
const double EPS = 1e-;
const LL inf = 0x7fffffff;
const LL infLL = 0x7fffffffffffffffLL;
const LL mod = 1e9 + ;
const int maxN = 2e5 + ;
const LL ONE = ;
const LL evenBits = 0xaaaaaaaaaaaaaaaa;
const LL oddBits = 0x5555555555555555; template<typename T>
struct Point{
T X,Y;
Point< T >(T x = ,T y = ) : X(x), Y(y) {} inline Point<T> operator* (const T& k) { return Point<T>(k*X, k*Y); }
inline Point<T> operator/ (const T& k) { return Point<T>(X/k, Y/k); }
inline Point<T> operator+ (const Point<T>& x) { return Point<T>(x.X+X,x.Y+Y); }
inline Point<T> operator- (const Point<T>& x) { return Point<T>(x.X-X,x.Y-Y); } T operator^(const Point<T> &x) const { return X*x.Y - Y*x.X; }
T operator*(const Point<T> &x) const { return X*x.X + Y*x.Y; }
}; template<typename T>
istream &operator>> (istream &in, Point<T> &x) {
in >> x.X >> x.Y;
return in;
} Point< LL > p[]; int main(){
//freopen("MyOutput.txt","w",stdout);
//freopen("input.txt","r",stdin);
//INIT();
while(cin >> p[] >> p[] >> p[]) cout << * abs((p[] - p[]) ^ (p[] - p[])) << endl;
return ;
}
2019 牛客多校第一场 F Random Point in Triangle的更多相关文章
- 2019牛客多校第一场 I Points Division(动态规划+线段树)
2019牛客多校第一场 I Points Division(动态规划+线段树) 传送门:https://ac.nowcoder.com/acm/contest/881/I 题意: 给你n个点,每个点有 ...
- 2019牛客多校第一场E ABBA(DP)题解
链接:https://ac.nowcoder.com/acm/contest/881/E 来源:牛客网 ABBA 时间限制:C/C++ 2秒,其他语言4秒 空间限制:C/C++ 524288K,其他语 ...
- 2019 牛客多校第一场 D Parity of Tuples
题目链接:https://ac.nowcoder.com/acm/contest/881/D 看此博客之前请先参阅吕凯飞的论文<集合幂级数的性质与应用及其快速算法>,论文中很多符号会被本文 ...
- 2019牛客多校第一场A-Equivalent Prefixes
Equivalent Prefixes 传送门 解题思路 先用单调栈求出两个序列中每一个数左边第一个小于自己的数的下标, 存入a[], b[].然后按照1~n的顺序循环,比较 a[i]和b[i]是否相 ...
- 2019牛客多校第一场 A.Equivalent Prefixes
题目描述 Two arrays u and v each with m distinct elements are called equivalent if and only if RMQ(u,l,r ...
- 2019 牛客多校第一场 B Integration
题目链接:https://ac.nowcoder.com/acm/contest/881/B 题目大意 给定 n 个不同的正整数 ai,求$\frac{1}{\pi}\int_{0}^{\infty} ...
- 2019 牛客多校第一场 E ABBA
题目链接:https://ac.nowcoder.com/acm/contest/881/E 题目大意 问有多少个由 (n + m) 个 ‘A’ 和 (n + m) 个 ‘B’,组成的字符串能被分割成 ...
- 2019 牛客多校第一场 C Euclidean Distance ?
题目链接:https://ac.nowcoder.com/acm/contest/881/C 题目大意 给定 m 和 n 个整数 ai,$-m \leq a_i \leq m$,求$\sum\limi ...
- 2019 牛客多校第一场 A Equivalent Prefixes
题目链接:https://ac.nowcoder.com/acm/contest/881/A 题目大意 定义 RMQ(u, L, R) 为 u 数组在区间 [L, R] 上最小值的下标. 如果有 2 ...
随机推荐
- 经典sql题练习50题
-- 1.查询"01"课程比"02"课程成绩高的学生的信息及课程分数 select a.* ,b.s_score as 01_score,c.s_score a ...
- AcWing 139. 回文子串的最大长度 hash打卡
如果一个字符串正着读和倒着读是一样的,则称它是回文的. 给定一个长度为N的字符串S,求他的最长回文子串的长度是多少. 输入格式 输入将包含最多30个测试用例,每个测试用例占一行,以最多1000000个 ...
- NX二次开发-基于NX开发向导模板的NX对Excel读写操作(OLE方式(COM组件))
在看这个博客前,请读者先去完整看完:NX二次开发-基于MFC界面的NX对Excel读写操作(OLE方式(COM组件))https://ufun-nxopen.blog.csdn.net/article ...
- undefined reference to `mysql_init'解决办法
命令行后面加入 -l mysqlclient 例如: 对mysqlQuery.c编译,使用gcc mysqlQuery.c -o mysqlQuery -l mysqlclient,即可编译成功.
- CSS:CSS 轮廓(outline)
ylbtech-CSS:CSS 轮廓(outline) 1.返回顶部 1. CSS 轮廓(outline) 轮廓(outline)是绘制于元素周围的一条线,位于边框边缘的外围,可起到突出元素的作用. ...
- webogic基本使用
文章目录 启动 注入 部署应用: 访问 启动 /root/Oracle/Middleware/user_projects/domains/weblogic/bin/startWebLogic.sh 上 ...
- sed 删除含有某个字符串的行 (在文件txt)
#删除a.txt中含有“aaa”的行 sed -i “/aaa/d” a.txt
- VIM编辑器进阶配置
vim自定义设置 可以选择需要的功能添加至 ~/.vimrc 打开注释使之生效. " 让 vim 关闭所有扩展的功能,尽量模拟 vi 的行为. set nocompatible ...
- pytest----fixture(1)--使用fixture执行配置及销毁逻辑
1使用fixture执行配 置及销毁;非常灵活 使用. 2数据共享:在 conftest.py配置里写方 法可以实现数据共享, 不需要import导入.可 以跨文件共享 3scope的层次及神 奇的y ...
- SpringMVC前后端参数交互
Controller中使用JSON方式有多种 关键在于ajax请求是将数据以什么形式传递到后台 HTTP请求中: 如果是get请求,那么表单参数以name=value&name1=value1 ...