【Spring Boot学习之二】WEB开发
环境
Java1.8
Spring Boot 1.3.2
一、静态资源访问
动静分离:动态服务和前台页面图片分开,静态资源可以使用CDN加速;
Spring Boot默认提供静态资源目录位置需置于classpath下,目录名需符合如下规则:
/static
/public
/resources
/META-INF/resources
举例:我们可以在src/main/resources/目录下创建static,在该位置放置一个图片文件。启动程序后,尝试访问http://localhost:8080/d.jpg。如能显示图片,配置成功。
二、全局异常捕获
首先定义一个切面处理类 用来处理运行时异常报错:
package com.wjy.controller; import java.util.HashMap;
import java.util.Map; import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody; @ControllerAdvice
public class GlobalExceptionHandler { @ExceptionHandler(RuntimeException.class)
@ResponseBody
public Map<String, Object> resultError() {
Map<String, Object> result = new HashMap<String, Object>();
result.put("errorCode", "500");
result.put("errorMsg", "系统错误!");
return result;
} }
@ControllerAdvice 是controller的一个辅助类,最常用的就是作为全局异常处理的切面类;
@ControllerAdvice 可以指定扫描范围;
@ControllerAdvice 约定了几种可行的返回值:
返回 String,表示跳到某个 view
返回 modelAndView
返回 model + @ResponseBody:将model类转换成json格式
然后写一个方法抛出异常:
package com.wjy.controller; import java.util.HashMap;
import java.util.Map; import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController; @EnableAutoConfiguration
@RestController
public class HelloController { @RequestMapping("/hello")
public String hello() {
return "hello world";
} @RequestMapping("/getMap")
public Map<String, Object> getMap() {
Map<String, Object> result = new HashMap<String, Object>();
result.put("errorCode", "200");
result.put("errorMsg", "成功..");
int a = 1/0;
return result;
} }
验证:
三、渲染WEB页面
Spring Boot整合页面建议优先选择模板引擎,不建议选用JSP,若一定要使用JSP将无法实现Spring Boot的多种特性。
模板引擎:使用伪HTML作动态页面模板,方便开发,另外提高搜索引擎搜索,Spring Boot提供了默认配置的模板引擎主要有以下几种:
• Thymeleaf
• FreeMarker
• Velocity
• Groovy
• Mustache
当你使用上述模板引擎中的任何一个,它们默认的模板配置路径为:src/main/resources/templates。
1、使用Freemaker渲染WEB视图
(1)POM引入依赖
<!-- 引入freeMarker的依赖包. -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-freemarker</artifactId>
</dependency>
(2)在src/main/resources/创建一个templates文件夹,里面创建动态页面模板,后缀为*.ftl
比如:index.ftl
<!DOCTYPE html>
<html>
<head lang="en">
<meta charset="UTF-8" />
<title>首页</title>
</head>
<body>
${name}
<#if sex==1>
男
<#elseif sex==2>
女
<#else>
其他 </#if>
<#list userlist as user>
${user}
</#list>
</body>
</html>
(3)创建后台代码
package com.wjy.controller; import java.util.ArrayList;
import java.util.List;
import java.util.Map; import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping; //注意这里是 @Controller 不是@RestController
@Controller
public class FmindexController { @RequestMapping("/fmindex")
public String index(Map<String, Object> result) {
System.out.println("IndexController....index");
result.put("name", "yushengjun");
result.put("sex", 1);
List<String> list = new ArrayList<String>();
list.add("zhangsan");
list.add("lisi");
result.put("userlist", list);
return "index";
} }
注意:ftl的名字要和java 返回字符串的名字相同
验证:
(4)freemaker配置
使用application.properties文件,默认在src/main/resources目录下
########################################################
###FREEMARKER (FreeMarkerAutoConfiguration)
########################################################
spring.freemarker.allow-request-override=false
spring.freemarker.cache=true
spring.freemarker.check-template-location=true
spring.freemarker.charset=UTF-8
spring.freemarker.content-type=text/html
spring.freemarker.expose-request-attributes=false
spring.freemarker.expose-session-attributes=false
spring.freemarker.expose-spring-macro-helpers=false
#spring.freemarker.prefix=
#spring.freemarker.request-context-attribute=
#spring.freemarker.settings.*=
spring.freemarker.suffix=.ftl
spring.freemarker.template-loader-path=classpath:/templates/
#comma-separated list
#spring.freemarker.view-names= # whitelist of view names that can be resolved
2、使用JSP渲染WEB视图
(1)maven项目必须是war类型 eclipse 4.7 + jdk1.8
然后依次修改如下配置:
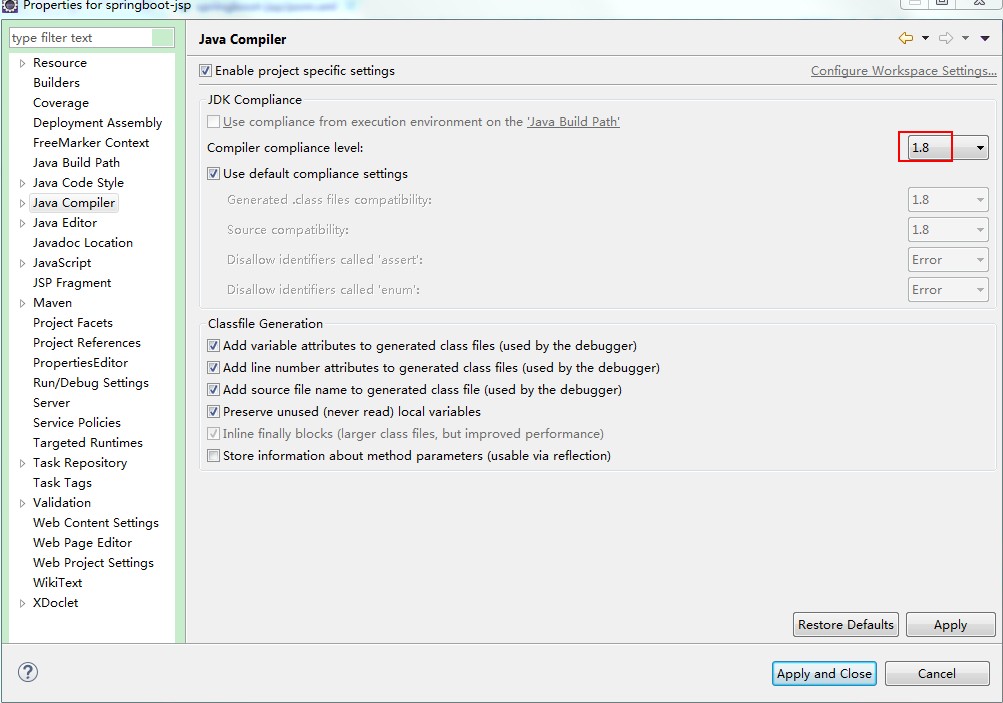
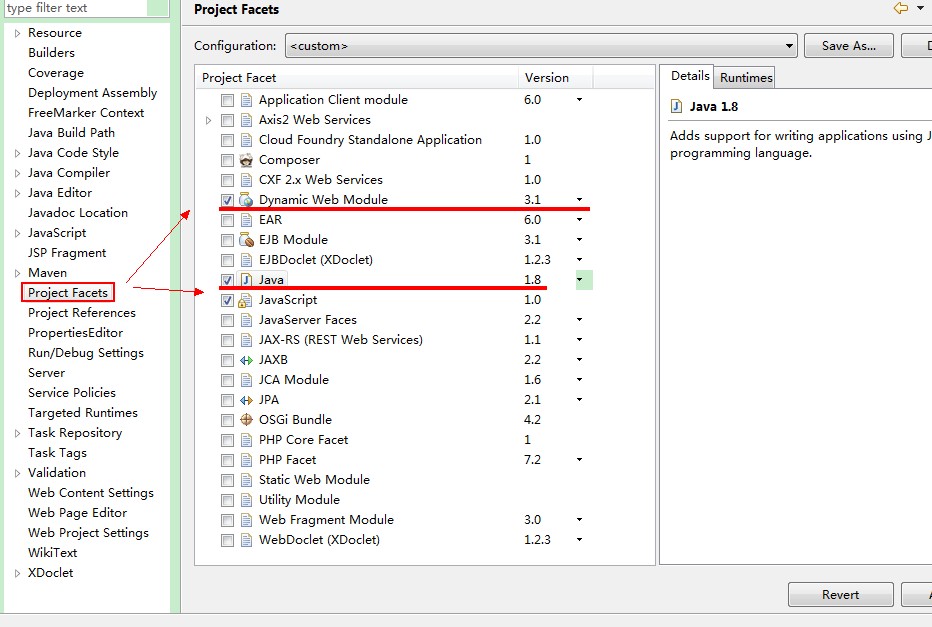
(2)POM引入依赖
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>1.3.3.RELEASE</version>
</parent>
<dependencies>
<!-- SpringBoot 核心组件 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
</dependency>
<dependency>
<groupId>org.apache.tomcat.embed</groupId>
<artifactId>tomcat-embed-jasper</artifactId>
</dependency>
<!-- 强制添加依赖 否则可能在解析JSP报错:No Java compiler available -->
<dependency>
<groupId>org.eclipse.jdt.core.compiler</groupId>
<artifactId>ecj</artifactId>
<version>4.6.1</version>
<scope>provided</scope>
</dependency>
</dependencies> <!-- 不加下面这个build 可能update project不起作用 -->
<build>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.1</version>
<configuration>
<source>1.8</source>
<target>1.8</target>
</configuration>
</plugin>
</plugins>
</build>
最后 maven --> Update Project...
(3)application.properties创建以下配置
spring.mvc.view.prefix=/WEB-INF/jsp/
spring.mvc.view.suffix=.jsp
(4)代码
页面:
<%@ page language="java" contentType="text/html; UTF-8" pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
Spring Boot 整合 JSP
</body>
</html>
后台:
package com.wjy.app; import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.context.annotation.ComponentScan; @EnableAutoConfiguration
@ComponentScan(basePackages="com.wjy.controller")
public class App { public static void main(String[] args) {
SpringApplication.run(App.class, args);
} }
package com.wjy.controller; import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping; @Controller
public class IndexController { @RequestMapping("/index")
public String index() {
return "index";
}
}
验证:
【Spring Boot学习之二】WEB开发的更多相关文章
- Spring Boot学习记录(二)--thymeleaf模板 - CSDN博客
==他的博客应该不错,没有细看 Spring Boot学习记录(二)--thymeleaf模板 - CSDN博客 http://blog.csdn.net/u012706811/article/det ...
- Spring Boot学习笔记二
Spring Boot入门第二篇 第一天的详见:https://www.cnblogs.com/LBJLAKERS/p/12001253.html 同样是新建一个pring Initializer快速 ...
- Spring Boot学习(二):配置文件
目录 前言 方式1:通过配置绑定对象的方式 方式2:@Value("${blog.author}")的形式获取属性值 相关说明 注解@Value的说明 参考 前言 Spring B ...
- spring Boot 学习(二、Spring Boot与缓存)
一.概述1. 大多应用中,可通过消息服务中间件来提升系统异步通信.扩展解耦能力 2. 消息服务中两个重要概念: 消息代理(message broker)和目的地(destination) 当消息发送者 ...
- Spring Boot学习笔记:项目开发中规范总结
Spring Boot在企业开发中使用的很广泛,不同的企业有不同的开发规范和标准.但是有些标准都是一致的. 项目包结构 以下是一个项目常见的包结构 以上是一个项目的基本目录结构,不同的项目结构会有差异 ...
- Spring Boot学习笔记(二二) - 与Mybatis集成
Mybatis集成 Spring Boot中的JPA部分默认是使用的hibernate,而如果想使用Mybatis的话就需要自己做一些配置.使用方式有两种,第一种是Mybatis官方提供的 mybat ...
- Spring Boot学习(二)搭建一个简易的Spring Boot工程
第一步:新建项目 新建一个SpringBoot工程 修改项目信息 勾选项目依赖和工具 选择好项目的位置,点击[Finish] 第二步:项目结构分析 新建好项目之后的结构如下图所示,少了很多配置文件: ...
- Spring Boot学习总结二
Redis是目前业界使用最广泛的内存数据存储.相比memcached,Redis支持更丰富的数据结构,例如hashes, lists, sets等,同时支持数据持久化.除此之外,Redis还提供一些类 ...
- spring boot 学习笔记(二) 构建web支持jsp
一.必须将项目打包成war包 <packaging>war</packaging> 二.pom.xml加入依赖包 <dependency> <groupId& ...
随机推荐
- 转载>>去除inline-block元素间间距的N种方法《重》
一.现象描述 真正意义上的inline-block水平呈现的元素间,换行显示或空格分隔的情况下会有间距,很简单的个例子: <input /> <input type="su ...
- dump array
<?php //array_dump.php $a=array(); $a[]=1; $a[]=2; $a[]=3; $a[]=4; $a[]='a'; $a[]='b'; $a[]='c'; ...
- 多目标跟踪MOT综述
https://blog.csdn.net/u012435142/article/details/85255005 多目标跟踪MOT 1评价指标 https://www.cnblogs.com/YiX ...
- LeetCode 1249. Minimum Remove to Make Valid Parentheses
原题链接在这里:https://leetcode.com/problems/minimum-remove-to-make-valid-parentheses/ 题目: Given a string s ...
- Openwrt build env setup(9)
reference : https://openwrt.org/docs/guide-developer/quickstart-build-images Install dependence pack ...
- 【数论】[逆元,错排]P4071排列计数
题目描述 求有多少种长度为n的系列A,满足以下条件: 1~n这n个数在序列中各出现一次:若第i个数a[i]的值为i,则称i是稳定的.序列恰有m个数是稳定的. 输出序列个数对1e9+7取模的结果. So ...
- 自建 ca 及使用 ca 颁发证书
创建CA: 一.安装openssl [root@localhost ~]# yum install -y openssl 二.创建CA的相关文件及目录 mkdir /opt/root_ca & ...
- 【NOI2002】荒岛野人(信息学奥赛一本通 1637)(洛谷 2421)
题目描述 克里特岛以野人群居而著称.岛上有排列成环行的M个山洞.这些山洞顺时针编号为1,2,…,M.岛上住着N个野人,一开始依次住在山洞C1,C2,…,CN中,以后每年,第i个野人会沿顺时针向前走Pi ...
- Mac上安装Python3虚拟环境(VirtualEnv)教程
如果已经安装好pip3,那么执行命令安装virtualenv环境 pip3 install virtualenv 安装完成检测版本是否安装成功 virtualenv --version 创建新目录 M ...
- ImportError: DLL load failed while importing win32api: 找不到指定的模块。
这个是用pip install pywin32安装报的一个错误 据说直接使用pip install pypiwin32安装就不会有报错 但是遇到错误还是要尝试解决一下的 pip install pyw ...