[ACM_搜索] ZOJ 1103 || POJ 2415 Hike on a Graph (带条件移动3盘子到同一位置的最少步数 广搜)
Description
In the sixties ("make love not war") a one-person variant of the game emerged. In this variant one person moves all the three pieces, not necessarily one after the other, but of course only one at a time. Goal of this game is to get all pieces onto the same location, using as few moves as possible. Find out the smallest number of moves that is necessary to get all three pieces onto the same location, for a given board layout and starting positions.
Input
Output
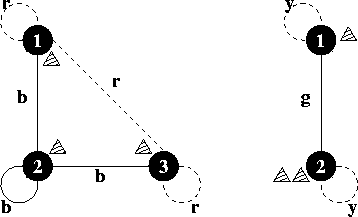
Sample Input
3 1 2 3
r b r
b b b
r b r
2 1 2 2
y g
g y
0
Sample Output
2
impossible
Source
题目大意:有一张图,上面的路径都是着色的,开始的时候有3个盘子在确定的点上,现在让你按要求沿图中的路径移动盘子(一步只能移动一只盘子),问是否能将3个盘子都移到同一个点上,如果可以,输出需要的最少步数,否则输出“impossible”。>_<移动条件是:每个盘子只能沿着这样一条路移动,这条路的颜色和另外的两个盘子之间的路径上标记的颜色是一样的。
解题思路:因为这道题给的是完全图,也就是说图上每两个点之间都有路径存在,它们标记的都有颜色。所以直接BFS就AC啦!
相关链接:过程详解 http://www.cnblogs.com/pcoda/archive/2012/09/02/2667987.html
#include<iostream>
#include<queue>
#include<string>
#include<string.h>
using namespace std;
int n,p1,p2,p3;
char map[][];//存储图
int ans[][][];//保存a[i][j][k]表示3个盘子在i,j,k位置时的最小步数
int ok;//记录最终3个盘子的位置,如果为0则imposible
struct state{
int a,b,c;
}temp;//3个盘子的位置
void read(){
cin>>p1>>p2>>p3;
for(int i=;i<=n;i++){
map[i][]='#';//在这填充个字符,不然就报错
for(int j=;j<=n;j++)
cin>>map[i][j];
map[i][n+]='\0';//在行尾加一个结束符,便于后面操作
}
}
void bfs(){
ok=;
fill(&ans[][][],&ans[][][]+**,);//将ans初始化很大
ans[p1][p2][p3]=;//令刚开始位置为0(不要少了)
queue<state> Q;
temp.a=p1;temp.b=p2;temp.c=p3;
Q.push(temp);
while(!Q.empty()){
state top=Q.front();Q.pop();
int x=top.a,y=top.b,z=top.c;
if(x==y && y==z){//如果3盘到一点就跳出
ok=x;
break;
}else{
int cur_ans=ans[x][y][z];
cur_ans++; char bc_color=map[y][z];
string str_a=map[x];//(与a连的所有路径)
for(int i=;i<=n;i++){
//遍历所有路径,如果不是自己,且满足移动条件,且ans[i][y][z]>cur_ans就移动
if(i!=x && str_a[i]==bc_color && ans[i][y][z]>cur_ans){
ans[i][y][z] = cur_ans;
temp.a=i;temp.b=y;temp.c=z;
Q.push(temp);
}
}//a盘的移动 char ac_color=map[x][z];
string str_b=map[y];
for(int i=;i<=n;i++){
if(i!=y && str_b[i]==ac_color && ans[x][i][z]>cur_ans){
ans[x][i][z]=cur_ans;
temp.a=x;temp.b=i;temp.c=z;
Q.push(temp);
}
}//b盘的移动 char ab_color=map[x][y];
string str_c=map[z];
for(int i=;i<=n;i++){
if(i!=z && str_c[i]==ab_color && ans[x][y][i]>cur_ans){
ans[x][y][i]=cur_ans;
temp.a=x;temp.b=y;temp.c=i;
Q.push(temp);
}
}//c盘的移动
}
}
}
int main(){
while(cin>>n && n){
read();
bfs();
if(ok)cout<<ans[ok][ok][ok]<<'\n';
else cout<<"impossible\n";
}return ;
}
[ACM_搜索] ZOJ 1103 || POJ 2415 Hike on a Graph (带条件移动3盘子到同一位置的最少步数 广搜)的更多相关文章
- 广搜,深搜,单源最短路径,POJ(1130),ZOJ(1085)
题目链接: http://acm.zju.edu.cn/onlinejudge/showProblem.do?problemId=85 http://poj.org/problem?id=1130 这 ...
- ZOJ 1542 POJ 1861 Network 网络 最小生成树,求最长边,Kruskal算法
题目连接:problemId=542" target="_blank">ZOJ 1542 POJ 1861 Network 网络 Network Time Limi ...
- hdu Hike on a Graph
此题是道bfs搜索的题目.bfs的精髓就是找到下一步的所有可能然后存储起来,有点暴力的感觉,这题就是每步中 所有的可能都入队,然后一一 判断.这道题的题意是 : 给你一幅完全图,再给你三个盘,目的是把 ...
- 双向广搜 POJ 3126 Prime Path
POJ 3126 Prime Path Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 16204 Accepted ...
- 广搜+打表 POJ 1426 Find The Multiple
POJ 1426 Find The Multiple Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 25734 Ac ...
- poj 3026 Borg Maze 最小生成树 + 广搜
点击打开链接 Borg Maze Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 7097 Accepted: 2389 ...
- poj 3278 Catch That Cow (广搜,简单)
题目 以前做过,所以现在觉得很简单,需要剪枝,注意广搜的特性: 另外题目中,当人在牛的前方时,人只能后退. #define _CRT_SECURE_NO_WARNINGS //这是非一般的最短路,所以 ...
- (poj)3414 Pots (输出路径的广搜)
Description You are given two pots, having the volume of A and B liters respectively. The following ...
- POJ 3984 迷宫问题 记录路径的广搜
主要是学一下如何在广搜中记录路径:每找到一个点我就记录下这个点是由那个点得来的,这样我找到最后个点后,我就可以通过回溯得到我走过的路径,具体看代码吧~ #include<cstdio> # ...
随机推荐
- linux C学习笔记02--共享内存(进程同步)
system V下3中进程同步:共享内存(shared memory),信号量(semaphore)和消息队列(message queue) 调试了下午,终于调通啦! 运行./c.out 输出共享内存 ...
- spi controller
http://blog.csdn.net/droidphone/article/details/24353293 http://www.china-cpu.com/supports/article/0 ...
- 循序渐进Python3(七) --1-- 面向对象
Python 面向对象 什么是面向对象编程? 面向对象编程是一种程序设计范式 对现实世界建立对象模型 把程序看作不同对象的相互调用 Python从设计之初就已经是一门面向对象的语言,正因为如此,在Py ...
- Ubuntu 14.04 (32位)上搭建Hadoop 2.5.1单机和伪分布式环境
引言 一直用的Ubuntu 32位系统(准备下次用Fedora,Ubuntu越来越不适合学习了),今天准备学习一下Hadoop,结果下载Apache官网上发布的最新的封装好的2.5.1版,配置完了根本 ...
- JS控制鼠标点击事件
鼠标点击事件就是当鼠标点击元素时,就会出现另一个窗口,类似于百度首页中右上角的“登录”这个按钮,当鼠标点击 登录时,就会出现登录窗口.大体的意思就是这样,直接上代码了,简单易懂. <!DOCTY ...
- 【随笔】mOnOwall添加端口映射
mOnOwall是一个完整的嵌入防火墙软件包,当与一台嵌入个人电脑一起使用时,在免费使用自由软件的基础上,提供具备商业防火墙所有重要特性(包括易用). 这里通过配置mOnOwall的端口设置映射功能, ...
- 使用并行的方法计算斐波那契数列 (Fibonacci)
更新:我的同事Terry告诉我有一种矩阵运算的方式计算斐波那契数列,更适于并行.他还提供了利用TBB的parallel_reduce模板计算斐波那契数列的代码(在TBB示例代码的基础上修改得来,比原始 ...
- sqlite query用法
本文转自http://blog.csdn.net/double2hao/article/details/50281273,在此感谢作者 query(table, columns, selection, ...
- [Chapter 3 Process]Practice 3.8: Describe the differences among short-term, medium-term, long-term scheduling
3.8 Describe the differences among short-term, medium-term, and longterm scheduling. 答案: 长期调度决定哪些进程进 ...
- LeetCode OJ-- Word Ladder II ***@
https://oj.leetcode.com/problems/word-ladder-ii/ 啊,终于过了 class Solution { public: vector<vector< ...