Programming Entity Framework CodeFirst -- 约定和属性配置
Data Annotation
|
MinLength(nn)
MaxLength(nn)
StringLength(nn)
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName).HasMaxLength(nn)
|
在SQL Server中,string会转换为nvarchar(max),bit会转换为varbinary(max) 如果是SQL CE这里的最大值会变成4000(毕竟人家是嵌入式数据库). 通过MinLength等来限制字段在数据库中的长度。MinLength和MaxLength会受到EntityFramework的验证,不会影响到数据库。
[StringLength(,MinimumLength= )]
public string Description { get; set; }
Data Type
Data Annotation
|
Column(TypeName=“xxx”)
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName)
.HasColumnType (“xxx”)
|
[Column(TypeName = "image")]
public byte[] Photo { get; set; }
Nullability
Data Annotation
|
Required
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName).IsRequired
|
[Required]
public string Name { get; set; }
Keys
Data Annotation
|
Key
|
Fluent
|
Entity<T>.HasKey(t=>t.PropertyName)
|
EF需要每个实体必须拥有一个Key,context会根据key来跟踪对象。而且默认是自动递增的。
Database-Generated
Data Annotation
|
DatabaseGenerated(DatabaseGeneratedOption)
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName)
.HasDatabaseGeneratedOption(DatabaseGeneratedOption)
|
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int DestinationId { get; set; }
DatabaseGeneratedOption 为None可以关闭自动增加。 TimeStamp或RowVersion
Data Annotation
|
TimeStamp
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName).IsRowVersion()
|
timestamp和rowversion是同一个数据类型的不同叫法,不同的数据库显示不同
[Timestamp]
public byte[] RowVersion { get; set; }
时间戳:数据库中自动生成的唯一二进制数字,与时间和日期无关的, 通常用作给表行加版本戳的机制,当行数据发生修改,时间戳会自动增加。存储大小为 8个字节。一个表只有一个时间戳列,不适合做主键,因为其本身是变化的。
在控制并发时起到作用:
用户A/B同时打开某条记录开始编辑,保存是可以判断时间戳,因为记录每次被更新时,系统都会自动维护时间戳,所以如果保存时发现取出来的时间戳与数据库中的时间戳如果不相等,说明在这个过程中记录被更新过,这样的话可以防止别人的更新被覆盖.
Non-Timestamp for Concurrency
Data Annotation
|
ConcurrencyCheck |
Fluent
|
Entity<T>.Property(t=>t.PropertyName).IsConcurrencyToken() |
对于那些没有时间戳的数据库中也提供并发检查。避免造成同时修改造成的冲突。和上面的Timestamp一样,当模型发生改变写入到数据库的时候,数据库不但通过key找到了这一行数据,还会比对标记了Concurrency字段的值是否是原来的值。
public class Person
{
public int PersonId { get; set; }
[ConcurrencyCheck]
public int SocialSecurityNumber { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
} //....
private static void UpdatePerson()
{
using (var context = new BreakAwayContext())
{
var person = context.People.FirstOrDefault();
person.FirstName = "Rowena";
context.SaveChanges();
}
}
SQL:
exec sp_executesql N'update [dbo].[People]
set [FirstName] = @0
where (([PersonId] = @1) and ([SocialSecurityNumber] = @2))
',N'@0 nvarchar(max) ,@1 int,@2 int',@0=N'Rowena',@1=1,@2=12345678
Non-Unicode Database Types
Data Annotation
|
unavailable
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName).IsUnicode(boolean)
|
Property(l => l.Owner).IsUnicode(false);
默认情况下,所以string都会映射成数据库中的Unicode类型。data Annotation 不支持。
Precision and Scale of Decimals
精度定义:
Data Annotation
|
unavailable
|
Fluent
|
Entity<T>.Property(t=>t.PropertyName).HasPrecision(n,n)
|
Property(l => l.MilesFromNearestAirport).HasPrecision(, );
上面表示MilesFromNearestAirport是八位数,保留一位小数。data Annotation 不支持。
complex type
Complex Type需要满足3个条件:1.没有key(Id),2.complex类型只能包含原始属性(primitive properties),3.当它用到别的类中时,只能是非集合(non-collection)形式。
下面的Address就是一个complex type
public class Address
{
//public int AddressId { get; set; }
public string StreetAddress { get; set; }
public string City { get; set; }
public string State { get; set; }
public string ZipCode { get; set; }
}
public class Person
{
public int PersonId { get; set; }
public int SocialSecurityNumber { get; set; }
public string FirstName { get; set; }
public string LastName { get; set; }
public Address Address { get; set; }
}
生成的表如下。
[ComplexType]
public class Address
{
public int AddressId { get; set; }
public string StreetAddress { get; set; }
public string City { get; set; }
public string State { get; set; }
public string ZipCode { get; set; }
}
Api :
modelBuilder.ComplexType<Address>().Property(p => p.StreetAddress).HasMaxLength();
More Complicated Complex Types
public class PersonalInfo
{
public Measurement Weight { get; set; }
public Measurement Height { get; set; }
public string DietryRestrictions { get; set; }
}
public class Measurement
{
public decimal Reading { get; set; }
public string Units { get; set; }
} public PersonalInfo Info { get; set; }
Person包含PersonInfo,PersonInfo包含两个Measurement。先在构造函数中初始化:
public Person()
{
Address = new Address();
Info = new PersonalInfo
{
Weight = new Measurement(),
Height = new Measurement()
};
}
这时运行会报错,说PersonInfo 没有定义主键。因为他包含的两个Measurement不是原始类型(primitive types)。

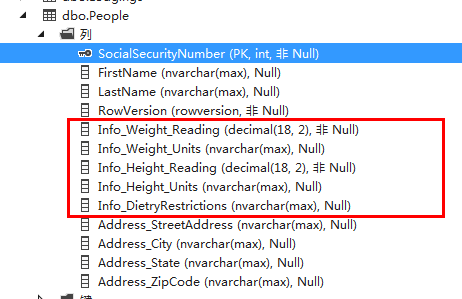
public class AddressConfiguration :
ComplexTypeConfiguration<Address>
{
public AddressConfiguration()
{
Property(a => a.StreetAddress).HasMaxLength();
}
}
再在OnModelCreating方法中加入
modelBuilder.Configurations.Add(new AddressConfiguration());
Programming Entity Framework CodeFirst -- 约定和属性配置的更多相关文章
- 【读书笔记】Programming Entity Framework CodeFirst -- 初步认识
以下是书<Programming Entity Framework Code First>的学习整理,主要是一个整体梳理. 一.模型属性映射约定 1.通过 System.Component ...
- Entity Framework 系统约定配置
前言 Code First之所以能够让开发人员以一种更加高效.灵活的方式进行数据操作有一个重要的原因在于它的约定配置.现在软件开发越来越复杂,大家都试图将软件设计的越来越灵活,很多内容我们都希望是可配 ...
- Entity Framework Codefirst的配置步骤
Entity Framework Codefirst的配置步骤: (1) 安装命令: install-package entityframework (2) 创建实体类,注意virtual关键字在导航 ...
- 第三篇:Entity Framework CodeFirst & Model 映射 续篇 EntityFramework Power Tools 工具使用
上一篇 第二篇:Entity Framework CodeFirst & Model 映射 主要介绍以Fluent API来实作EntityFramework CodeFirst,得到了大家一 ...
- 第二篇:Entity Framework CodeFirst & Model 映射
前一篇 第一篇:Entity Framework 简介 我有讲到,ORM 最关键的 Mapping,也提到了最早实现Mapping的技术,就是 特性 + 反射,那Entity Framework 实现 ...
- Entity Framework CodeFirst数据迁移
前言 紧接着前面一篇博文Entity Framework CodeFirst尝试. 我们知道无论是“Database First”还是“Model First”当模型发生改变了都可以通过Visual ...
- [Programming Entity Framework] 第3章 查询实体数据模型(EDM)(一)
http://www.cnblogs.com/sansi/archive/2012/10/18/2729337.html Programming Entity Framework 第二版翻译索引 你可 ...
- entity framework codefirst 用户代码未处理DataException,InnerException基础提供程序在open上失败,数据库生成失败
警告:这是一个入门级日志,如果你很了解CodeFirst,那请绕道 背景:这篇日志记录我使用Entity FrameWork CodeFirst时出现的错误和解决问题的过程,虽然有点曲折……勿喷 备注 ...
- ADO.NET Entity Framework CodeFirst 如何输出日志(EF 5.0)
ADO.NET Entity Framework CodeFirst 如何输出日志(EF4.3) 用的EFProviderWrappers ,这个组件好久没有更新了,对于SQL执行日志的解决方案的需求 ...
随机推荐
- M2事后分析汇报总结
学霸网站项目Postmortem结果 M2之于M1的改进 文档和问答的整合 完成webservice 完成数据库触发器设计与完整性约束依赖(大规模) 优化学霸UI 资源的搜索 外部问题的搜索 文档的上 ...
- 阻塞非阻塞,同步异步四种I/O方式
举一个去书店买书的例子吧: (同步)阻塞: 你去书店买书,到柜台告诉店员,需要买一本APUE,然后一直在柜台等.(阻塞) 店员拿到书以后交给你. (同步)非阻塞: 你去书店买书,到柜台告诉店员A,需要 ...
- DDX_Text ()函数 C++
DDX_Text()函数管理着对话框.表格视或控件视对象中的编辑控件与对话框.表格视或控件视对象的CString型数据成员之间的int,UINT,long,DWORD,CString,float或do ...
- checkbox标签已有checked=checked属性但是不显示勾选
点击全选按钮,选中下面的列表,再次点击取消选择. 第一次的使用的方法是$("input[name=xxx]").attr('checked',true); 但是往往刷新页面第一次点 ...
- 使用my exclipse对数据库进行操作(3)
public class class3 { public static void main(String[] args) { // TODO Auto-generated method stub tr ...
- 自己对Extjs的Xtemplate的忽略
之前学习extjs Xtmeplate受一些书籍的误导,说Xtemplate不支持else ,今天仔细看了官网的示例,才恍然大悟,卧槽!不仅支持if-elseif-else结构 连switch都能够支 ...
- paip.语义分析--分词--常见的单音节字词 2_deDuli 单字词 774个
paip.语义分析--分词--常见的单音节字词 2_deDuli 单字词 774个 作者Attilax 艾龙, EMAIL:1466519819@qq.com 来源:attilax的专栏 地址 ...
- JS-随机排序
var arr = [ 1,2,3,4,5,6,7,8 ];arr.sort(function ( a, b ) { return Math.random() - 0.5;});alert( a ...
- shell循环
for循环 for循环一般格式为: for 变量 in 列表 do command1 command2 ... commandN done 列表是一组值(数字.字符串等)组成的序列,每个值通过空格分隔 ...
- A List of Social Tagging Datasets Made Available for Research
This list is not exhaustive - help expand it! Social Tagging Systems Research Group Source Year Obta ...