pandas dataframe 读取 xlsx 文件
refer to:
https://medium.com/@kasiarachuta/reading-and-writingexcel-files-in-python-pandas-8f0da449cc48
dframe = pd.read_excel(“file_name.xlsx”)
dframe = pd.read_excel(“file_name.xlsx”, sheetname=”Sheet_name”)
dframe = pd.read_excel(“file_name.xlsx”, sheetname=number)
原文如下:
//////////////////////////////////////////////////////////////////////////////
Reading and writingExcel files in Python pandas
In data science, you are very likely to mostly work with CSV files. However, knowing how to import and export Excel files is also very useful.
In this post, a Kaggle dataset on 2016 US Elections was used (https://www.kaggle.com/benhamner/d/benhamner/2016-us-election/primary-results-sample-data/output). This dataset has been converted from a CSV file to an Excel file and two sheets have been added with votes for Hilary Clinton (HilaryClinton) and Donald Trump (DonaldTrump). The first sheet (All) contains the original dataset.
Reading Excel files
dframe = pd.read_excel(“file_name.xlsx”)
Reading Excel files is very similar to reading CSV files. By default, the first sheet of the Excel file is read.
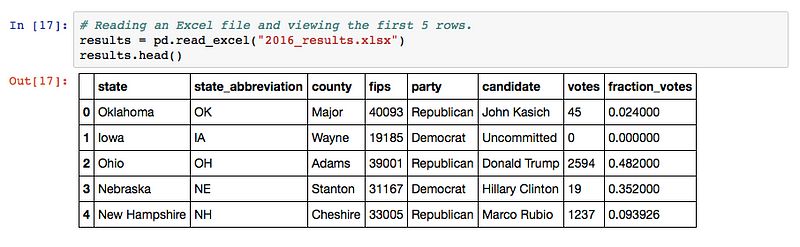
I’ve read an Excel file and viewed the first 5 rows
dframe = pd.read_excel(“file_name.xlsx”, sheetname=”Sheet_name”)
Passing the sheetname method allows you to read the sheet of the Excel file that you want. It is very handy if you know its name.

I picked the sheet named “DonaldTrump”
dframe = pd.read_excel(“file_name.xlsx”, sheetname=number)
If you aren’t sure what are the names of your sheets, you can pick them by their order. Please note that the sheets start from 0 (similar to indices in pandas), not from 1.

I read the second sheet of the Excel file
dframe = pd.read_excel(“file_name.xlsx”, header=None)
Sometimes, the top row does not contain the column names. In this case, you pass the argument of header=None.

The first row is not the header — instead, we get the column names as numbers
dframe = pd.read_excel(“file_name.xlsx”, header=n)
Passing the argument of header being equal to a number allows us to pick a specific row as the column names.

I pick the second row (i.e. row index 1 of the original dataset) as my column names.
dframe = pd.read_excel(“file_name.xlsx”, index_col=number)
You can use different columns for the row labels by passing the index_col argument as number.

I now use the county as the index column.
dframe = pd.read_excel(“file_name.xlsx”, skiprows=n)
Sometimes, you don’t want to include all of the rows. If you want to skip the first n rows, just pass the argument of skiprows=n.

Skipping the first two rows (including the header)
Writing an Excel file
dframe.to_excel(‘file_name.xlsx’)
I wrote an Excel file called results.xlsx from my results DataFrame
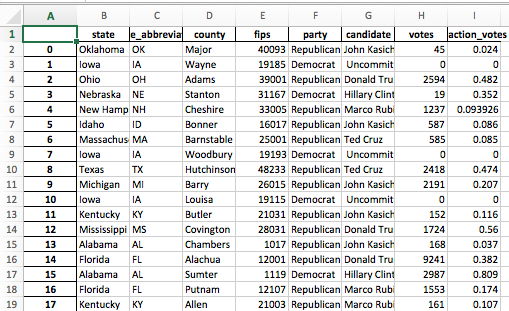
My exported Excel file
dframe.to_excel(‘file_name.xlsx’, index=False)
If you don’t want to include the index name (for example, here it is a number so it may be meaningless for future use/analysis), you can just pass another argument, setting index as False.
I don’t want index names in my Excel file
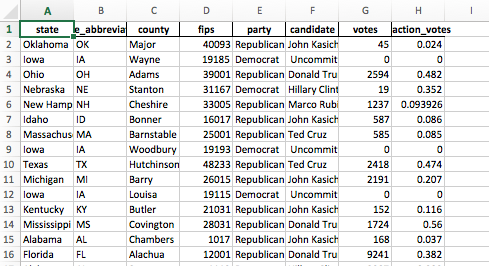
Excel file output with no index names
All of the code can be found on my GitHub: https://github.com/kasiarachuta/Blog/blob/master/Reading%20and%20writing%20Excel%20files.ipynb
pandas dataframe 读取 xlsx 文件的更多相关文章
- pandas-19 DataFrame读取写入文件的方法
pandas-19 DataFrame读取写入文件的方法 DataFrame有非常丰富的IO方法,比如DataFrame读写csv文件excel文件等等,操作很简单.下面在代码中标记出来一些常用的读写 ...
- 人工智能-机器学习之seaborn(读取xlsx文件,小提琴图)
我们不止可以读取数据库的内容,还可以读取xlsx文件的内容,这个库有在有些情况还是挺实用的 首先我们想读取这个文件的时候必须得现有个seaborn库 下载命令就是: pip install seab ...
- Python读取xlsx文件
Python读取xlsx文件 脚本如下: from openpyxl import load_workbook workbook = load_workbook(u'/tmp/test.xlsx') ...
- 读取xlsx文件的内容输入到xls文件中
package com.cn.peitest.excel; import java.io.File; import java.io.FileInputStream; import java.io.Fi ...
- C#读取xlsx文件Excel2007
读取Excel 2007的xlsx文件和读取老的.xls文件是一样的,都是用Oledb读取,仅仅连接字符串不同而已. 具体代码实例: public static DataTable GetExcelT ...
- C#基础知识之读取xlsx文件Excel2007
读取Excel 2007的xlsx文件和读取老的.xls文件是一样的,都是用Oledb读取,仅仅连接字符串不同而已. 具体代码实例: public static DataTable GetExcelT ...
- 使用POI读取xlsx文件,包含对excel中自定义时间格式的处理
package poi; import java.io.File; import java.io.FileInputStream; import java.io.FileNotFoundExcepti ...
- pandas read_csv读取大文件的Memory error问题
今天在读取一个超大csv文件的时候,遇到困难:首先使用office打不开然后在python中使用基本的pandas.read_csv打开文件时:MemoryError 最后查阅read_csv文档发现 ...
- Pandas dataframe数据写入文件和数据库
转自:http://www.dcharm.com/?p=584 Pandas是Python下一个开源数据分析的库,它提供的数据结构DataFrame极大的简化了数据分析过程中一些繁琐操作,DataFr ...
随机推荐
- Entity Framework 数据生成选项DatabaseGenerated(转)
在EF中,我们建立数据模型的时候,可以给属性配置数据生成选项DatabaseGenerated,它后有三个枚举值:Identity.None和Computed. Identity:自增长 None:不 ...
- 使用老毛桃安装Windows操作系统
首先必须知道什么是PE系统? 当电脑出现问题而不能正常进入系统时候的一种“紧急备用”系统,通常放在U盘中,设置启动项优先级,使得电脑启动的时候加载PE系统. 如何在U盘中安装老毛桃(PE工具箱)? h ...
- 正在尝试解析依赖项“MvvmLightLibs (≥ 5.2.0.0)”。 “MvvmLightLibs”已拥有为“CommonServiceLocator”定义的依赖项
正在尝试解析依赖项"MvvmLightLibs (≥ 5.2.0.0)". "MvvmLightLibs"已拥有为"CommonServiceLoca ...
- TED #01#
Laura Vanderkam: How to gain control of your free time 1.我们总是不缺乏时间去做重要的事情,即便我们再忙; “我没时间” 的同义词是“我不想做” ...
- Sublime Text 3 配置Python3.x
Sublime Text 3 配置Python3.x 一.Package Control 安装: 1,通过快捷键 ctrl+` 或者 View > Show Console 打开控制台,然后粘贴 ...
- Applet再学习
ZLYD团队Apllet学习笔记 Applet再学习 Applet是什么? Applet又称为Java小应用程序,是能够嵌入到一个HTML页面中,并且可通过Web浏览器下载和执行的一种Java类 .A ...
- HBase优化相关
1.HBase预分区 HBase在创建表时,默认会自动创建一个Region分区.在导入数据时,所有客户端都向这个Region写数据,直到这个Region足够大才进行切分.这样在大量数据并行写入时,容易 ...
- Maven .m2文件夹创建
settings.xml存在于两个地方: 1.安装的地方:$M2_HOME/conf/settings.xml 2.用户的目录:${user.home}/.m2/settings.xml 前者又被叫做 ...
- github上fork别人的代码之后,如何保持和原作者同步的更新
1.从自己fork之后的版本库clone $ git clone -o chucklu https://github.com/chucklu/Hearthstone-Deck-Tracker.git ...
- UVa 10534 波浪子序列(快速求LIS)
https://vjudge.net/problem/UVA-10534 题意:给定一个长度为n的整数序列,求一个最长子序列(不一定连续),使得该序列的长度为2k+1,前k+1个数严格递增,后k+1个 ...