(中等) POJ 1054 The Troublesome Frog,记忆化搜索。
Description
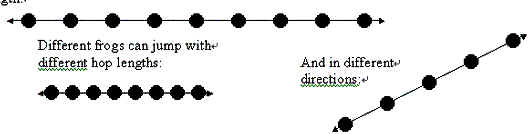
Your rice paddy has plants arranged on the intersection points of a grid as shown in Figure-1, and the troublesome frogs hop completely through your paddy, starting outside the paddy on one side and ending outside the paddy on the other side as shown in Figure-2:
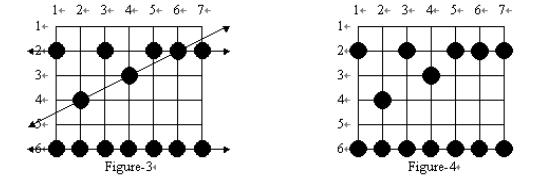
Many frogs can jump through the paddy, hopping from rice plant to rice plant. Every hop lands on a plant and flattens it, as in Figure-3. Note that some plants may be landed on by more than one frog during the night. Of course, you can not see the lines showing the paths of the frogs or any of their hops outside of your paddy ?for the situation in Figure-3, what you can see is shown in Figure-4:
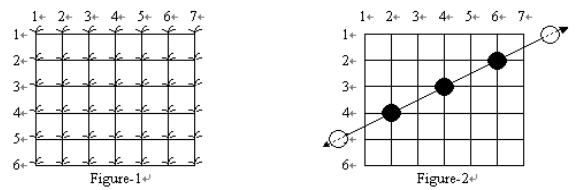
From Figure-4, you can reconstruct all the possible paths which the frogs may have followed across your paddy. You are only interested in frogs which have landed on at least 3 of your rice plants in their voyage through the paddy. Such a path is said to be a frog path. In this case, that means that the three paths shown in Figure-3 are frog paths (there are also other possible frog paths). The vertical path down column 1 might have been a frog path with hop length 4 except there are only 2 plants flattened so we are not interested; and the diagonal path including the plants on row 2 col. 3, row 3 col. 4, and row 6 col. 7 has three flat plants but there is no regular hop length which could have spaced the hops in this way while still landing on at least 3 plants, and hence it is not a frog path. Note also that along the line a frog path follows there may be additional flattened plants which do not need to be landed on by that path (see the plant at (2, 6) on the horizontal path across row 2 in Figure-4), and in fact some flattened plants may not be explained by any frog path at all.
Your task is to write a program to determine the maximum number of landings in any single frog path (where the maximum is taken over all possible frog paths). In Figure-4 the answer is 7, obtained from the frog path across row 6.
// ━━━━━━神兽出没━━━━━━
// ┏┓ ┏┓
// ┏┛┻━━━━━━━┛┻┓
// ┃ ┃
// ┃ ━ ┃
// ████━████ ┃
// ┃ ┃
// ┃ ┻ ┃
// ┃ ┃
// ┗━┓ ┏━┛
// ┃ ┃
// ┃ ┃
// ┃ ┗━━━┓
// ┃ ┣┓
// ┃ ┏┛
// ┗┓┓┏━━━━━┳┓┏┛
// ┃┫┫ ┃┫┫
// ┗┻┛ ┗┻┛
//
// ━━━━━━感觉萌萌哒━━━━━━ // Author : WhyWhy
// Created Time : 2015年07月20日 星期一 08时55分13秒
// File Name : 1054.cpp #include <stdio.h>
#include <string.h>
#include <iostream>
#include <algorithm>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <string>
#include <math.h>
#include <stdlib.h>
#include <time.h> using namespace std; const int MaxN=; int R,C;
short dp[MaxN][MaxN];
int N; struct Point
{
int x,y; bool operator < (const Point &b) const
{
if(x==b.x)
return y<b.y; return x<b.x;
}
}P[MaxN]; inline bool in(int x,int y)
{
return x<=R && x>= && y<=C && y>=;
} const int HASH=; struct HASHMAP
{
int head[HASH],next[MaxN],Hcou;
unsigned long long key[MaxN];
int rem[MaxN]; void init()
{
Hcou=;
memset(head,-,sizeof(head));
} void insert(unsigned long long val,int id)
{
int h=val%HASH; for(int i=head[h];i!=-;i=next[i])
if(val==key[i])
return; rem[Hcou]=id;
key[Hcou]=val;
next[Hcou]=head[h];
head[h]=Hcou++;
} int find(unsigned long long val)
{
int h=val % HASH; for(int i=head[h];i!=-;i=next[i])
if(val==key[i])
return rem[i]; return ;
}
}map1; int dfs(int u,int v)
{
if(dp[u][v])
return dp[u][v]; int dx=(P[v].x<<)-P[u].x,dy=(P[v].y<<)-P[u].y; if(!in(dx,dy))
return ; int rP=map1.find(dx*+dy); if(!rP)
{
dp[u][v]=-;
return -;
} dp[u][v]=dfs(v,rP)+; return dp[u][v];
} inline bool judge(int u,int v)
{
int dx=(P[u].x<<)-P[v].x,dy=(P[u].y<<)-P[v].y; return !in(dx,dy);
} int main()
{
//freopen("in.txt","r",stdin);
//freopen("out.txt","w",stdout); int ans=-; scanf("%d %d",&R,&C);
scanf("%d",&N); for(int i=;i<=N;++i)
scanf("%d %d",&P[i].x,&P[i].y); sort(P+,P+N+); map1.init(); for(int i=;i<=N;++i)
map1.insert(*P[i].x+P[i].y,i); for(int i=;i<=N;++i)
for(int j=i+;j<=N;++j)
if(judge(i,j))
ans=max(ans,dfs(i,j)); if(ans<)
ans=; printf("%d\n",ans); return ;
}
(中等) POJ 1054 The Troublesome Frog,记忆化搜索。的更多相关文章
- poj 3249(bfs+dp或者记忆化搜索)
题目链接:http://poj.org/problem?id=3249 思路:dp[i]表示到点i的最大收益,初始化为-inf,然后从入度为0点开始bfs就可以了,一开始一直TLE,然后优化了好久才4 ...
- poj 1661 Help Jimmy(记忆化搜索)
题目链接:http://poj.org/problem?id=1661 一道还可以的记忆化搜索题,主要是要想到如何设dp,记忆化搜索是避免递归过程中的重复求值,所以要得到dp必须知道如何递归 由于这是 ...
- poj 1085 Triangle War 博弈论+记忆化搜索
思路:总共有18条边,9个三角形. 极大极小化搜索+剪枝比较慢,所以用记忆化搜索!! 用state存放当前的加边后的状态,并判断是否构成三角形,找出最优解. 代码如下: #include<ios ...
- poj 1088 动态规划+dfs(记忆化搜索)
滑雪 Time Limit:1000MS Memory Limit:65536KB 64bit IO Format:%I64d & %I64u Description Mi ...
- poj 1579(动态规划初探之记忆化搜索)
Function Run Fun Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 17843 Accepted: 9112 ...
- POJ 3616 Milking Time ——(记忆化搜索)
第一眼看是线段交集问题,感觉不会= =.然后发现n是1000,那好像可以n^2建图再做.一想到这里,突然醒悟,直接记忆化搜索就好了啊..太蠢了.. 代码如下: #include <stdio.h ...
- POJ 1661 Help Jimmy ——(记忆化搜索)
典型的记忆化搜索问题,dfs一遍即可.但是不知道WA在哪里了= =,一直都没找出错误.因为思路是很简单的,肯定是哪里写挫了,因此不再继续追究了. WA的代码如下,希望日后有一天能找出错误= =: —— ...
- POJ 1054 The Troublesome Frog
The Troublesome Frog Time Limit: 5000MS Memory Limit: 100000K Total Submissions: 9581 Accepted: 2883 ...
- Poj 1054 The Troublesome Frog / OpenJudge 2812 恼人的青蛙
1.链接地址: http://poj.org/problem?id=1054 http://bailian.openjudge.cn/practice/2812 2.题目: 总时间限制: 10000m ...
随机推荐
- mysql 连接两列
以下划线符号,连接两列,作为查询结果: SELECT CONCAT(col_1,'_',col_2) FROM yourtable
- zf-关于评价器的开关所在的配置文件,与代码如何修改。
web.xml文件 把true改成false就是关
- JAVA-基本知识
1.JAVA跨平台 其实就是在每个平台上要安装对应该操作系统的JVM,JVM负责解析执行,即实现了跨平台.JVM是操作系统与java程序之间的桥梁. 2.JRE:java运行环境,包含JVM+核心类库 ...
- cURL: Learning..
CURL usage.. -v, -m, -H, -I, -s, --connect-timeout, -x, -X GET|POST, -d, -T, -o. --retry, -u curl [o ...
- surpersocket客户端
大家在学习surpersocket时候,都是拿telnet测试的吧,是不是没有 客户端 而感到 烦恼. 我么,就抽了一点时间 写了个简单的客户端代码. 针对QuickStart的 1-Basic 第一 ...
- AI 人工智能 探索 (八)
绑定下,用来释放内存 布局框架.链接:http://pan.baidu.com/s/1eQzSXZO 密码:25ir 这次 我采用 ngui 来设定界面.除工具栏模块外,其他各类ui模块都是 内存池动 ...
- 解决phpmyadmin 点击表结构时卡顿、一直加载、打不开的问题
本文内容是转自其它站点,亲测可用. 第一步,打开 ./version_check.php文件,找到以下代码: $save = true; $file ='http://www.phpmyadmin.n ...
- Android MediaScanner 详尽分析
[Innost]: http://blog.csdn.net/Innost/article/details/6083467 ====================================== ...
- Not a million dollars ——a certain kind of ingenuity, discipline, and proactivity that most people seem to lack
原文:http://ryanwaggoner.com/2010/12/you-dont-really-want-a-million-dollars/a certain kind of ingenuit ...
- js 鼠标事件
<html><head lang="en"> <meta charset="UTF-8"> <title>< ...