A Deep Dive Into Draggable and DragTarget in Flutter
https://medium.com/flutter-community/a-deep-dive-into-draggable-and-dragtarget-in-flutter-487919f6f1e4
This article is the sixth in a series of articles which take an in-depth look at Flutter’s built in widgets.
In this article, we will look at Draggable
and DragTarget
.
Exploring Draggable and DragTargets
Draggable
and DragTarget
allows us drag a widget across screen. First we will look at the basics of Draggables
and DragTargets
then dive into details of customizing them.
Draggable
A “Draggable” makes a widget movable around the screen. Let’s use a chess knight as our example.

Using a DragTarget to drag a chess knight around
The code for this is relatively straightforward:
Draggable(
child: WhiteKnight(
size: 90.0,
),
feedback: WhiteKnight(
size: 90.0,
),
childWhenDragging: Container(),
),
There are three main parts to the Draggable
widget:
- Child: The child parameter is the widget that will be displayed when the draggable is stationary
- Feedback: The feedback is the widget that will be displayed when the widget is being dragged.
- Child When Dragging: The childWhenDragging parameter takes the widget to display in the original place of
child
when the widget is being dragged.
In the example above,we have a white knight as the child
.When the knight is being dragged, we show an empty Container
in its place.
Let’s customize the widget
First, let’s change the feedback parameter.
In the example below, we change the feedback parameter to a white bishop. Now, when the widget is not being dragged, a white knight is shown however as you start dragging, our bishop is shown.
Draggable(
child: WhiteKnight(
size: 90.0,
),
feedback: WhiteBishop(
size: 90.0,
),
childWhenDragging: Container(),
),
Result of the above code:
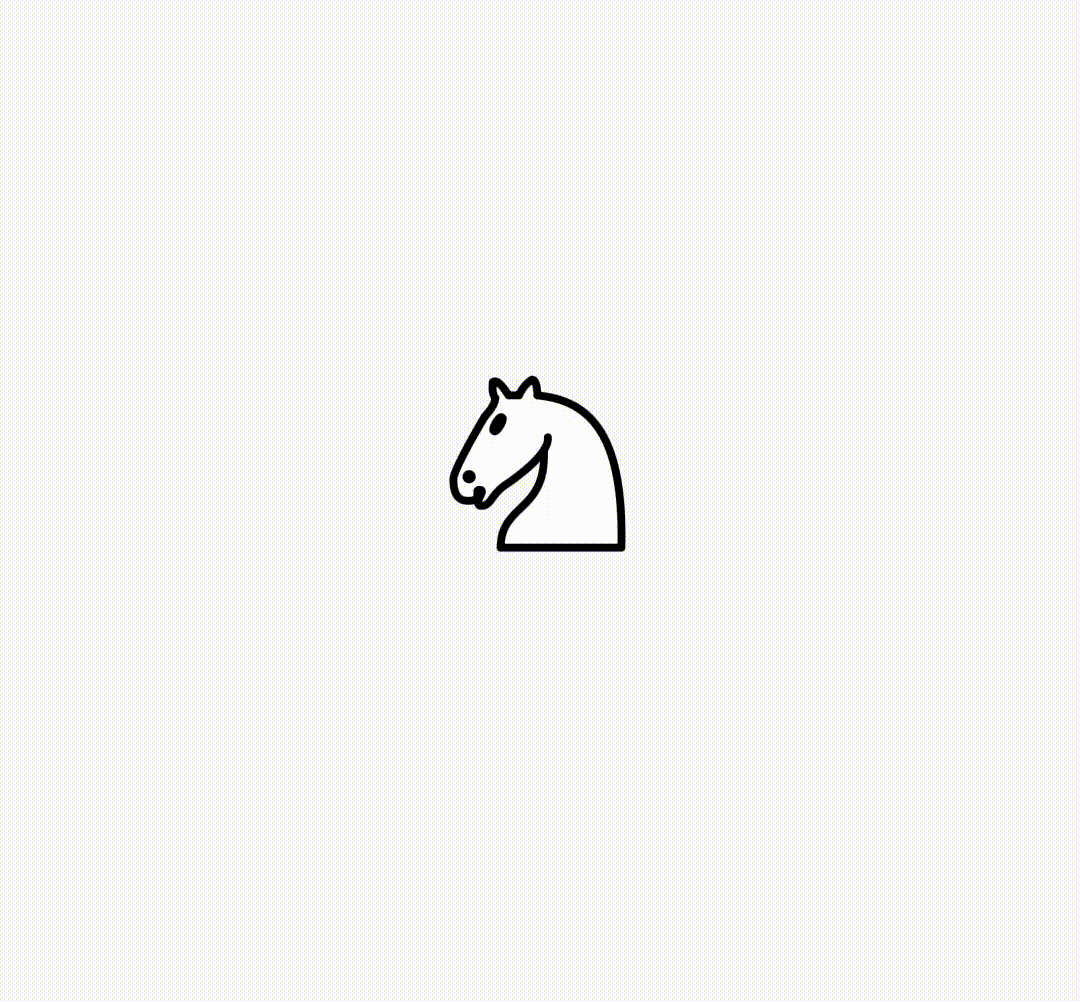
Now let’s move on to the third parameter, childWhenDragging
. This takes a widget to be displayed in the original position of child
as the widget is being dragged.
We’ll display a white rook when the widget is being dragged:
Draggable(
child: WhiteKnight(
size: 90.0,
),
feedback: WhiteBishop(
size: 90.0,
),
childWhenDragging: WhiteRook(
size: 90.0,
),
),
This becomes:
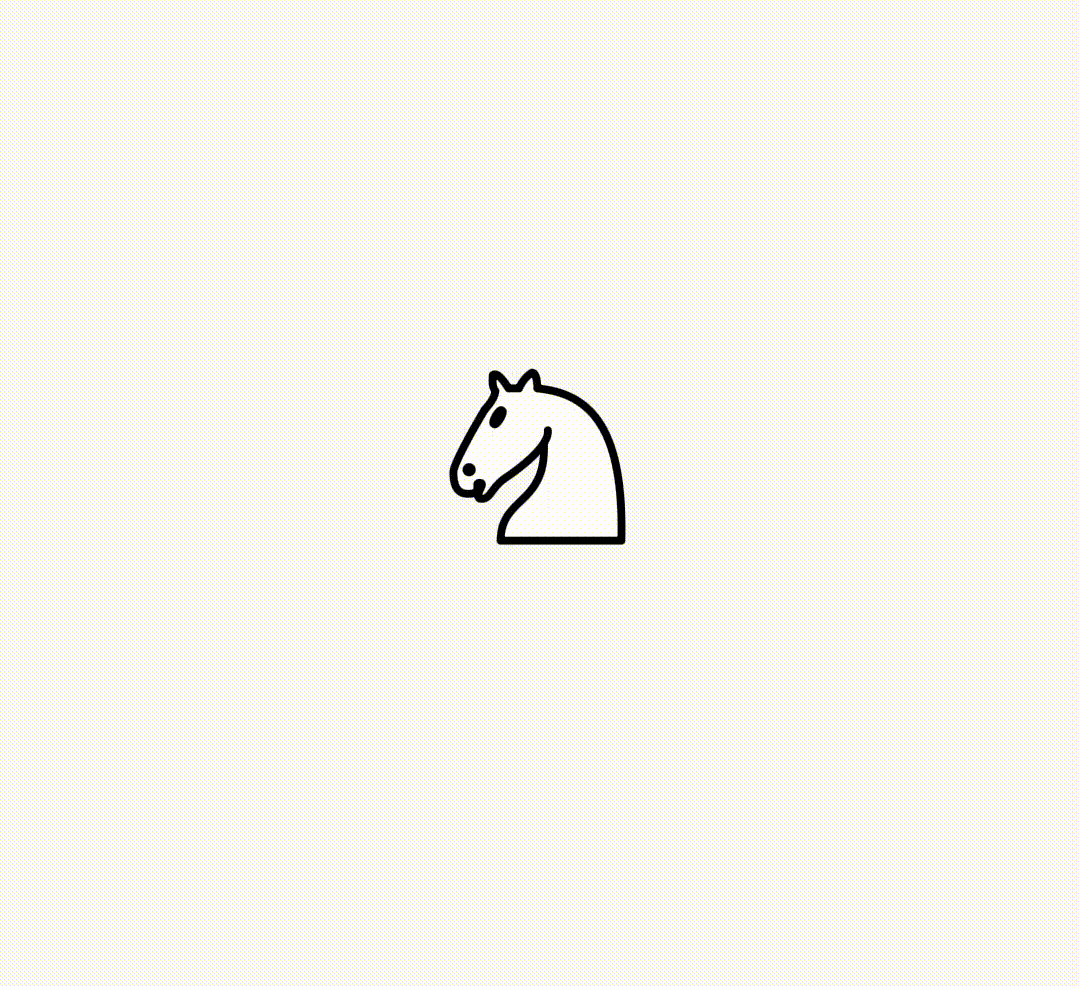
Restricting motion for Draggables
Setting the axis parameter helps us restrict motion in one dimension only.
Axis.horizontal makes the feedback widget on,y move in the horizontal axis.
Draggable(
axis: Axis.horizontal,
child: WhiteKnight(
size: 90.0,
),
feedback: WhiteBishop(
size: 90.0,
),
childWhenDragging: Container(),
),
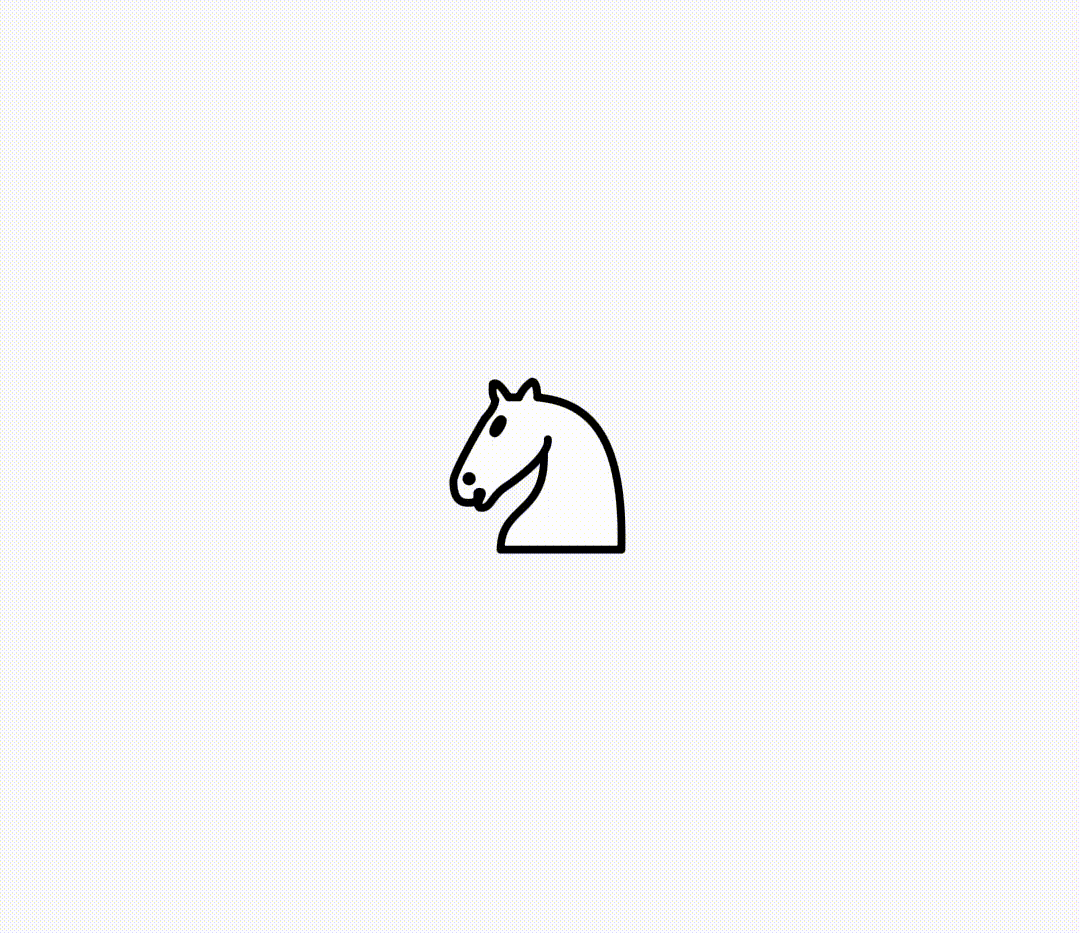
Similarly, we also have Axis.vertical for vertical movement.
Adding data to Draggable
Data can be attached to a Draggable
. This is useful to us since we use Draggables
and DragTargets
.
Considering our example, if we were to have multiple chess pieces, each piece would need to have unique data associated with it. Pieces would need properties such as color (White, Black) and type (ie: Rook, Bishop, Knight).
Below shows an example of how data can be attached to a Draggable
for use with DragTarget
.
We will take a look at this more in-depth in the DragTarget section.
Draggable(
child: WhiteKnight(
size: 90.0,
),
feedback: WhiteKnight(
size: 90.0,
),
childWhenDragging: Container(),
data: [
"White",
"Knight"
],
),
Callbacks
The draggable
widget supplies callbacks for actions on the widget.
These callbacks are:
onDragStarted : This is called when a drag is started on a widget.
onDragCompleted : When a draggable
is dragged to a DragTarget
and accepted, this callback is called. We will look atDragTarget
is in the next section.
onDragCancelled : When a draggable
does not reach a DragTarget
or is rejected, this callback is fired.
DragTarget
While a Draggable
allows a widget to be dragged, a DragTarget
provides a destination for the draggable.
For example, in chess, a chess piece is a draggable whereas a square box on the chessboard is a drag target.

Here, the knight is accepted by the DragTarget
.
The code for this example goes:
bool accepted = false;
DragTarget(builder: (context, List<String> candidateData, rejectedData) {
return accepted ? WhiteKnight(size: 90.0,) : Container();
}, onWillAccept: (data) {
return true;
}, onAccept: (data) {
accepted = true;
},),
NB: The DragTarget
is surrounded by a black colored container which is not shown in the code for brevity.
Let’s look at the parameters of the DragTarget
in more detail.
Note: The “data” in the following sections refers to the data parameter of the Draggable
.
Builder
The builder builds the actual widget inside the DragTarget
. This function is called every time a Draggable
is hovered over the DragTarget
or is dropped onto it.
This function has three parameters, context, candidateData and rejectedData.
candidateData is the data of a Draggable
whilst it is hovering over the DragTarget
, ready for acceptance by DragTarget
.
rejectedData is the data of a Draggable
hovering over a DragTarget
at moment it is not accepted.
Note that both of these are dealing with Draggables
which are hovering over the DragTarget
. At this point, they are not dropped onto it yet.
Now, how does the DragTarget
know what to accept?
onWillAccept
onWillAccept
is a function which gives us the Draggable
data for us to decide whether to accept or reject it.
This is where the data we attached onto the Draggable
becomes important. We use the data passed to accept or reject specific Draggables
.
For example if our Draggable is:
Draggable(
data: "Knight",
// ...
),
then we can do:
DragTarget(
// ...
onWillAccept: (data) {
if(data == "Knight") {
return true;
} else {
return false;
}
},
),
This will only accept the Draggable
if the data is “Knight”.
This function is called when the Draggable
is first hovered over the DragTarget
.
onAccept
If the Draggable
is dropped onto the DragTarget
and onWillAccept
returns true, then onAccept
is called.
onAccept
also gives us the data of the Draggable
and is usually used for storing the Draggable
dropped over DragTarget
.
onLeave
This was not implemented in the example. onLeave
is called when a Draggable
is hovered over the DragTarget
and leaves without being dropped.
A Demo
Let’s create a simple demo app where the user is given a number and they are required to sort it as either “even” or “odd’. Depending on the choice, we will then display a message stating whether the choice is correct or wrong.
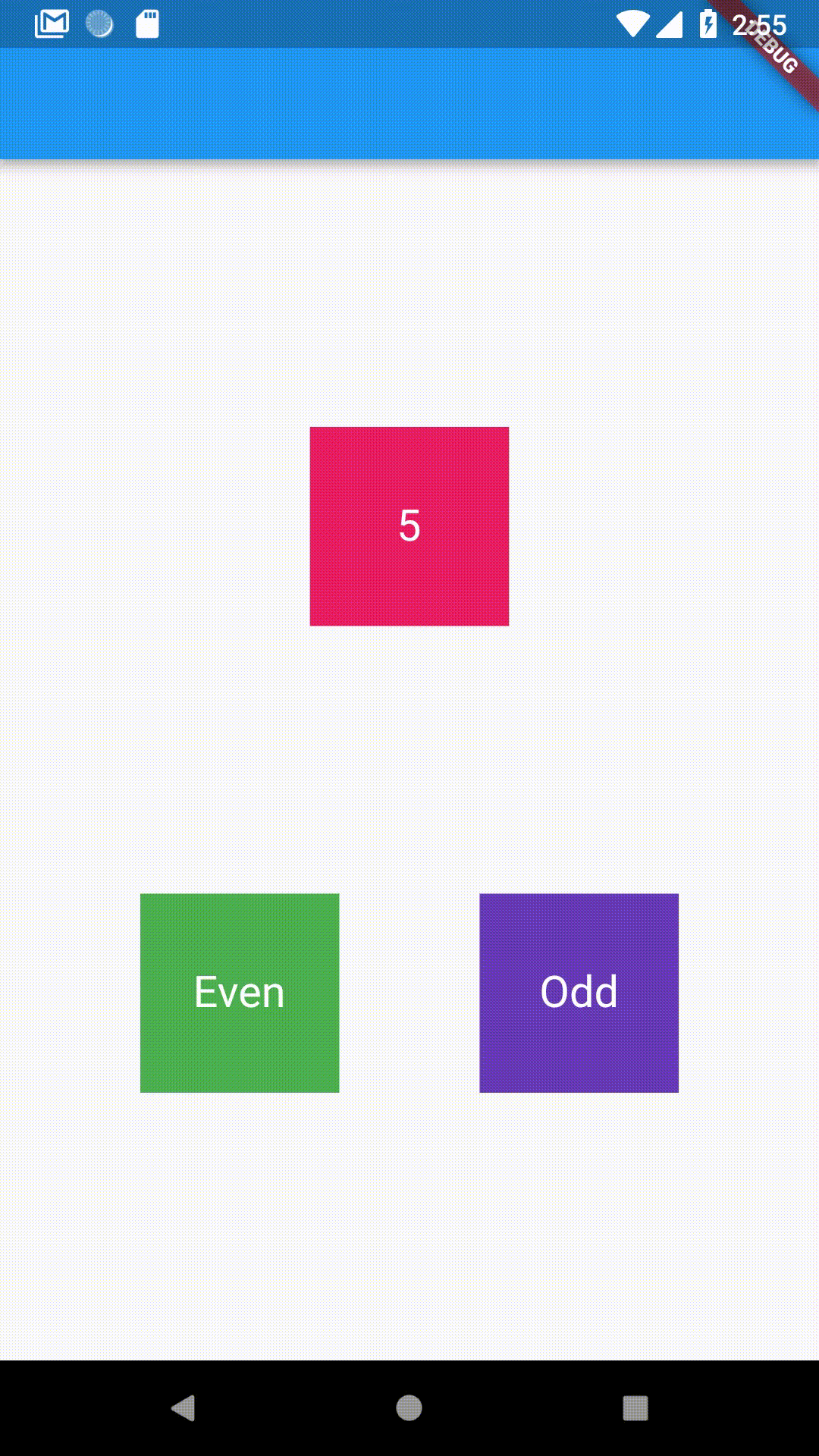
Source code:
A few more things
Draggable customisations
Draggable
gives us a few more customizations.
One such customization is maxSimultaneousDrags
. Multi-touch devicesare able to drag the same Draggable
with two or more pointers and hence we can have multiple feedback on screen. For instance if the user is using a second finger to drag a child while one drag is already progress, we receive two feedback widgets at the same time.
maxSimultaneousDrags lets us decide the maximum number of times a draggable
can be dragged at one time.
The feedbackOffset
property also lets us set the offset of the feedback widget that displays on the screen.
Common error: DragTarget not accepting data
In the builder function, consider giving a type to the parameter candidateData
. It returns a List
by default, so if the data you’re passing is a string, the type would be List<String>
.
builder: (context, List<String> candidateData, rejectedData) {
// ...
}
This solves a few type errors that occasionally comes up when passing data.
A more complete real-world example using Draggable and DragTarget
Sometime ago, I wrote a complete chessboard package using Draggables
and DragTargets
.
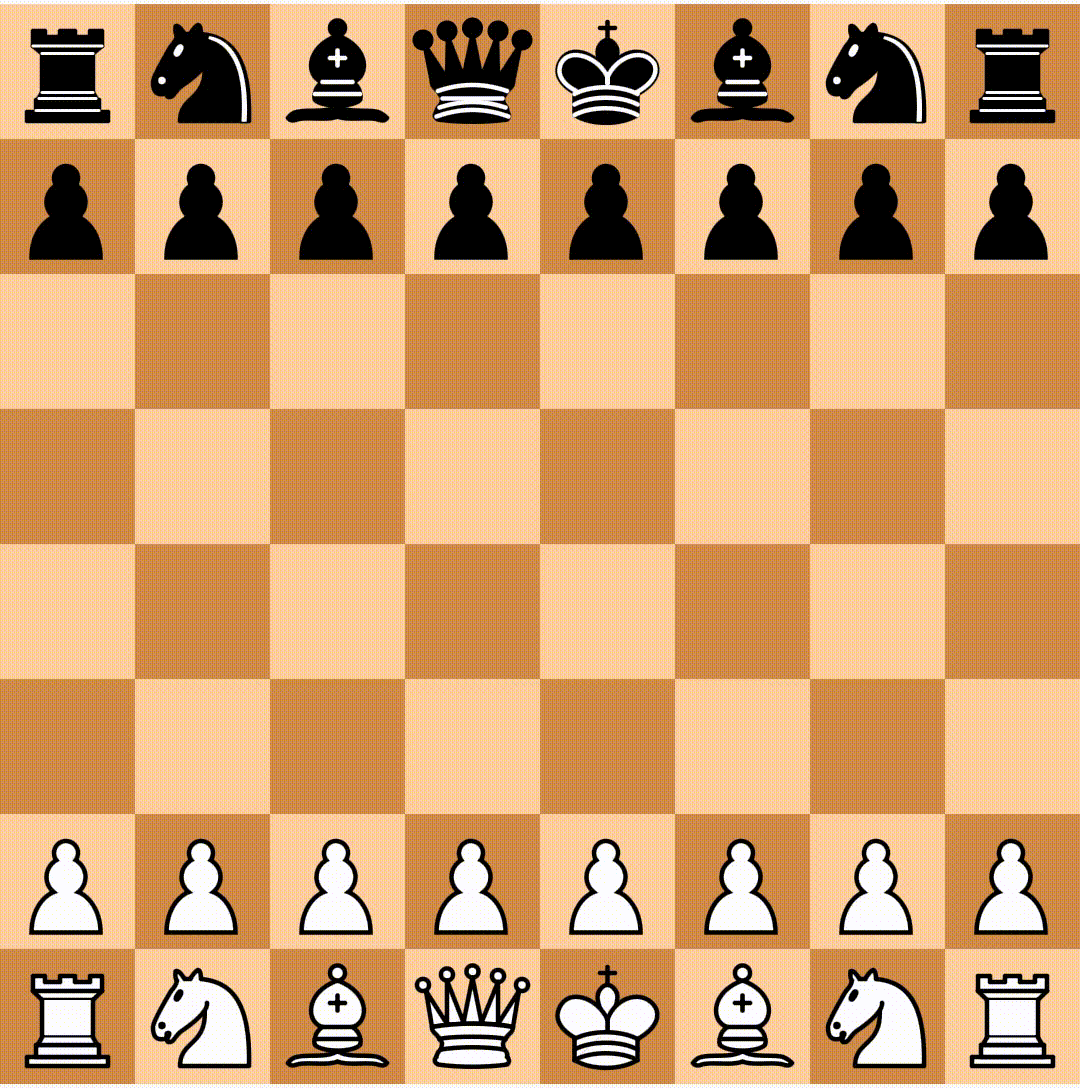
For the complete source code, you can check it out here.
Note: There is an update coming up to the package which includes a better architecture for the package, more boards, etc.
I used the chess_vectors_flutter package for the examples on this article.
A Deep Dive Into Draggable and DragTarget in Flutter的更多相关文章
- Deep Dive into Spark SQL’s Catalyst Optimizer(中英双语)
文章标题 Deep Dive into Spark SQL’s Catalyst Optimizer 作者介绍 Michael Armbrust, Yin Huai, Cheng Liang, Rey ...
- X64 Deep Dive
zhuan http://www.codemachine.com/article_x64deepdive.html X64 Deep Dive This tutorial discusses some ...
- 《Docker Deep Dive》Note - Docker 引擎
<Docker Deep Dive>Note Docker 引擎 1. 概览 graph TB A(Docker client) --- B(daemon) subgraph Docker ...
- 《Docker Deep Dive》Note - 纵观 Docker
<Docker Deep Dive>Note 由于GFW的隔离,国内拉取镜像会报TLS handshake timeout的错误:需要配置 registry-mirrors 为国内源解决这 ...
- Deep Dive into Neo4j 3.5 Full Text Search
In this blog we will go over the Full Text Search capabilities available in the latest major release ...
- 重磅解读:K8s Cluster Autoscaler模块及对应华为云插件Deep Dive
摘要:本文将解密K8s Cluster Autoscaler模块的架构和代码的Deep Dive,及K8s Cluster Autoscaler 华为云插件. 背景信息 基于业务团队(Cloud BU ...
- vue3 deep dive
vue3 deep dive vue core vnode vue core render / mount / patch refs https://www.vuemastery.com/course ...
- Flutter: Draggable和DragTarget 可拖动小部件
API class _MyHomeState extends State<MyHome> { List<Map<String, String>> _data1 = ...
- SQL optimizer -Query Optimizer Deep Dive
refer: http://sqlblog.com/blogs/paul_white/archive/2012/04/28/query-optimizer-deep-dive-part-1.aspx ...
随机推荐
- Video标签动态修改src地址播放问题
不管在React或Vue中,将一个变量赋值给src属性,当修改这个变量的值时,video播放的还是原来的视频. Vue中 <video id="root"> <s ...
- 4款五星级的3D模型资源包
HI,晚上好各位,今晚我们将为大家介绍4款五星级的3D模型资源包. ANIMALS FULL PACK ANIMALS FULL PACK包含了由PROTOFACTOR制作的24款高质量的动物模型,包 ...
- Eclipse中SpringBoot项目POM文件报UnKnown的解决方案
在项目中使用spring-boot-starter-parent的2.1.5.RELEASE版本时发现会出现POM错误(Unknown),其实这错误可以无视,但如果你实在看不下去可以在POM中添加如下 ...
- layer.confirm 防止post重复提交
layer.confirm("确定对该资源单的价格"+isa+value+"元吗!", function(index, layero){ $(layero).f ...
- nginx https 转发
add_header Content-Security-Policy upgrade-insecure-requests;
- 案例:执行 JavaScript 语句
隐藏百度图片 # coding=utf-8 from selenium import webdriver driver = webdriver.PhantomJS(executable_path=r' ...
- IntelliJ IDEA破解教程汇总
IDEA是一款很好用的工具,若资金允许,请点击https://www.jetbrains.com/idea/buy/购买正版,谢谢合作. 目前破解的方式主要有三种,注册机.破解补丁.注册码,下面分别介 ...
- 硬盘分区及Linux文件系统
1. 硬盘物理结构 硬盘物理上主要分为: 盘片 磁道 扇区 机械臂 磁头 主轴 磁道: 当硬盘盘片旋转时,磁头若固定在一个位置上,则磁头会在盘片表面划出一个圆形轨迹,这些圆形轨迹就叫做磁道.以盘片中心 ...
- nodejs调试工具 node-inspect
1.安装 npm install -g node-inspect 2.chrome设置 chrome://flags/#enable-devtools-experiments 3.测试 测试代码mai ...
- oracle全库查找是否有某个值
在scott用户下面,搜索含有'要找的值'的数据的表和字段穷举法: declare v_Sql ); v_count number; begin for xx in (select t.OWNER, ...