[Algorithm] Array production problem
Given an array of integers, return a new array such that each element at index i
of the new array is the product of all the numbers in the original array except the one at i
.
For example, if our input was [1, 2, 3, 4, 5]
, the expected output would be [120, 60, 40, 30, 24]
. If our input was [3, 2, 1]
, the expected output would be [2, 3, 6]
.
For example [1,2,3,4,5]:
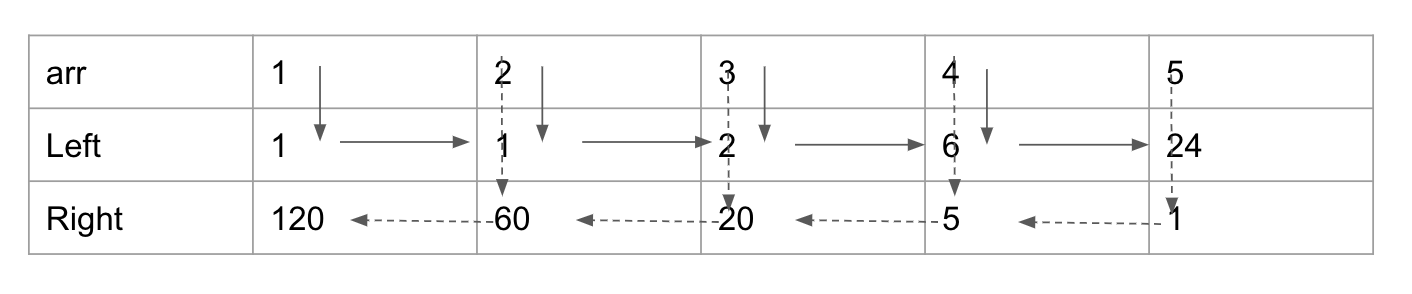
function productArr(arr) {
let left = [];
let right = [];
let prod = [];
left[0] = 1;
right[arr.length - 1] = 1; for (let i = 1; i <= arr.length -1; i++) {
left[i] = left[i-1] * arr[i-1];
} for (let j = arr.length - 2; j >=0; j--) {
right[j] = right[j+1] * arr[j+1];
} prod = left.map((l, i) => l * right[i]); return prod
} console.log(productArr([1, 2, 3, 4, 5])); // [120, 60, 40, 30, 24]
[Algorithm] Array production problem的更多相关文章
- [Codeforces 863D]Yet Another Array Queries Problem
Description You are given an array a of size n, and q queries to it. There are queries of two types: ...
- Yet Another Array Queries Problem CodeForces - 863D (暴力/思维)
You are given an array a of size n, and q queries to it. There are queries of two types: 1 li ri — p ...
- [Algorithm] Tower Hopper Problem
By given an array of number, each number indicate the number of step you can move to next index: For ...
- 863D - Yet Another Array Queries Problem(思维)
原题连接:http://codeforces.com/problemset/problem/863/D 题意:对a数列有两种操作: 1 l r ,[l, r] 区间的数字滚动,即a[i+1]=a[i] ...
- [Algorithm] Max Chars Problem
// --- Directions // Given a string, return the character that is most // commonly used in the strin ...
- Design and Analysis of Algorithms_Fundamentals of the Analysis of Algorithm Efficiency
I collect and make up this pseudocode from the book: <<Introduction to the Design and Analysis ...
- Co-variant array conversion from x to y may cause run-time exception
http://stackoverflow.com/questions/8704332/co-variant-array-conversion-from-x-to-y-may-cause-run-tim ...
- Leetcode 之 Kth Largest Element in an Array
636.Kth Largest Element in an Array 1.Problem Find the kth largest element in an unsorted array. Not ...
- 【LEETCODE】42、922. Sort Array By Parity II
package y2019.Algorithm.array; /** * @ProjectName: cutter-point * @Package: y2019.Algorithm.array * ...
随机推荐
- 将 Unity5.3 的老项目升级到 Unity 2018.3 遇到的些许问题。
删除 ParticleEmmiter 等废弃的接口: 删除 WindowsSecurityContext System.Security.Principal.WindowsIdentity 在 .Ne ...
- ssm+RESTful bbs项目后端主要设计
小谈: 帖主妥妥的一名"中"白了哈哈哈.软工的大三狗了,也即将找工作,怀着丝丝忐忑接受社会的安排.这是第一次写博客(/汗颜),其实之前在学习探索过程中,走了不少弯路,爬过不少坑.真 ...
- UIViewController的基本概念与生命周期
UIViewController是iOS顶层视图的载体及控制器,用户与程序界面的交互都是由UIViewController来控制的,UIViewController管理UIView的生命周期及资源的加 ...
- RabbitMQ (一)
学习RabbitMQ 可以先先了解一下AMQP 简单介绍: AMQP从一开始就设计成为开放标准,以解决众多的消息队列需求和拓扑结构问题,凭借开发,任何人都可以执行这一标准.针对标准编码的任何人都可以和 ...
- 【51Nod 1363】最小公倍数之和
http://www.51nod.com/onlineJudge/questionCode.html#!problemId=1363 \[ \begin{aligned} &\sum_{i=1 ...
- [CodeForces-178F]Representative Sampling
题目大意: 给你n个字符串,要求从中选出k个字符串,使得字符串两两lcp之和最大. 思路: 动态规划. 首先将所有的字符串排序,求出相邻两个字符串的lcp长度(很显然,对于某一个字符串,和它lcp最长 ...
- PHP链接sqlserver出现中文乱码
PHP通过dblib扩展链接sqlserver,使用的是freetds,出现中文乱码. 在freetds的配置文件中(/usr/local/freetds/etc/freetds.conf),[glo ...
- python开发_os.path
在python中,os.path模块在处理路径的时候非常有用 下面是我做的demo 运行效果: ========================================= 代码部分: ==== ...
- 常用SQL Server规范集锦及优化
原文地址:http://www.cnblogs.com/liyunhua/p/4526195.html
- CROC 2016 - Qualification C. Hostname Aliases map
C. Hostname Aliases 题目连接: http://www.codeforces.com/contest/644/problem/C Description There are some ...