Painter's Problem
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 5378 | Accepted: 2601 |
Description
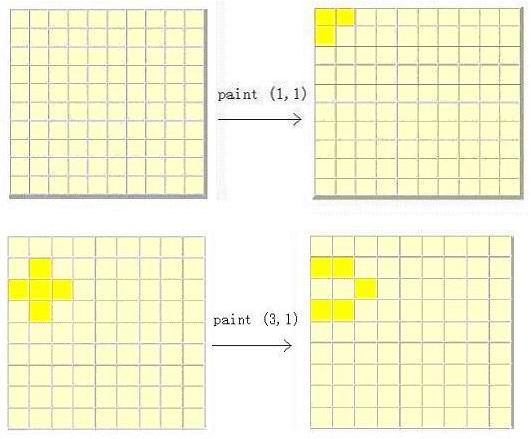
Input
Output
Sample Input
- 2
- 3
- yyy
- yyy
- yyy
- 5
- wwwww
- wwwww
- wwwww
- wwwww
- wwwww
Sample Output
0
15
题解:
构造矩阵高斯消元后可以得到一组解,但是题目中要求的是求出最小染色次数。所以要对其中不确定的方案进行枚举。
- #include<iostream>
- #include<cstdio>
- #include<cstdlib>
- #include<cmath>
- #include<algorithm>
- #include<cstring>
- #include<queue>
- #include<vector>
- using namespace std;
- int T,N,ANS;
- int a[][];
- bool gauss(){
- int now=;
- for(int i=;i<=N*N;i++){
- int to=now;
- while(to<=N*N&&a[to][i]==) to++;
- if(to>N*N) continue;
- if(to!=now){
- for(int j=;j<=N*N+;j++) swap(a[to][j],a[now][j]);
- }
- for(int j=;j<=N*N;j++){
- if(j!=now&&a[j][i]){
- for(int k=;k<=N*N+;k++){
- a[j][k]^=a[i][k];
- }
- }
- }
- now++;
- }
- for(int i=now;i<=N*N;i++)
- if(a[i][N*N+]!=) return false;
- return true;
- }
- int v[],cnt;
- void dfs(int x){
- if(cnt>=ANS) return ;//已经比目前的答案大了,没有必要再搜
- if(x==){
- ANS=min(cnt,ANS);
- return ;
- }
- if(a[x][x]!=){
- int num=a[x][N*N+];//num表示第x块砖染色不染色
- for(int i=x+;i<=N*N;i++){
- if(a[x][i]!=) num=num^v[i];//已经枚举过的x+1~N*N中某块砖如果可以对x产生影响且已染色,就让num改变一次
- }
- v[x]=num;
- if(num==) cnt++;
- dfs(x-);
- if(num==) cnt--;
- }
- else{//枚举按或不按两种情况
- v[x]=; dfs(x-);
- v[x]=; cnt++; dfs(x-); cnt--;
- }
- }
- int main(){
- scanf("%d",&T);
- while(T--){
- memset(a,,sizeof(a));
- scanf("%d",&N);
- for(int i=;i<=N*N;i++){
- a[i][i]=;
- if(i%N!=) a[i][i-]=;
- if(i%N!=) a[i][i+]=;
- if(i>=N+) a[i][i-N]=;
- if(i<=N*(N-)) a[i][i+N]=;
- }
- for(int i=;i<=N;i++){
- char s[];
- scanf("%s",s+);
- for(int j=;j<=N;j++){
- if(s[j]=='w') a[(i-)*N+j][N*N+]=;
- }
- }
- if(gauss()==false){
- puts("inf");
- continue;
- }
- ANS=<<;
- dfs(N*N);
- printf("%d\n",ANS);
- }
- return ;
- }
Painter's Problem的更多相关文章
- poj 1681 Painter's Problem
Painter's Problem 题意:给一个n*n(1 <= n <= 15)具有初始颜色(颜色只有yellow&white两种,即01矩阵)的square染色,每次对一个方格 ...
- Painter's Problem poj1681 高斯消元法
Painter's Problem Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 4420 Accepted: 2143 ...
- POJ 1681 Painter's Problem 【高斯消元 二进制枚举】
任意门:http://poj.org/problem?id=1681 Painter's Problem Time Limit: 1000MS Memory Limit: 10000K Total ...
- [POJ1681]Painter's Problem(高斯消元,异或方程组,状压枚举)
题目链接:http://poj.org/problem?id=1681 题意:还是翻格子的题,但是这里有可能出现自由变元,这时候枚举一下就行..(其实这题直接状压枚举就行) /* ━━━━━┒ギリギリ ...
- OpenJudge 2813 画家问题 / Poj 1681 Painter's Problem
1.链接地址: http://bailian.openjudge.cn/practice/2813 http://poj.org/problem?id=1681 2.题目: 总时间限制: 1000ms ...
- Painter's Problem (高斯消元)
There is a square wall which is made of n*n small square bricks. Some bricks are white while some br ...
- POJ 1681 Painter's Problem(高斯消元+枚举自由变元)
http://poj.org/problem?id=1681 题意:有一块只有黄白颜色的n*n的板子,每次刷一块格子时,上下左右都会改变颜色,求最少刷几次可以使得全部变成黄色. 思路: 这道题目也就是 ...
- POJ 1681 Painter's Problem (高斯消元)
题目链接 题意:有一面墙每个格子有黄白两种颜色,刷墙每次刷一格会将上下左右中五个格子变色,求最少的刷方法使得所有的格子都变成yellow. 题解:通过打表我们可以得知4*4的一共有4个自由变元,那么我 ...
- POJ 1681 Painter's Problem (高斯消元 枚举自由变元求最小的步数)
题目链接 题意: 一个n*n 的木板 ,每个格子 都 可以 染成 白色和黄色,( 一旦我们对也个格子染色 ,他的上下左右 都将改变颜色): 给定一个初始状态 , 求将 所有的 格子 染成黄色 最少需要 ...
随机推荐
- 操作数组可以通过Array这个类来操作(不需要考虑数组的类型!!!)
这段代码通过Array这个类,把值取出来,存到collection里,不需要考虑数组的类型
- Ajax 常用资源
regular online:http://regex.larsolavtorvik.com/ json online:http://json.cn/ Prototype:http://prototy ...
- JUnit4 测试示例
1. JUnit4 测试示例 // Calculator.java public class Calculator{ public int add(int a, int b){ return a + ...
- Python数据分析(一):工具的简单使用
1.Numpy 安装:pip install numpy [root@kvm work]# cat numpy_test.py #!/usr/bin/env python #coding:utf-8 ...
- nginx 哈希表结构图
- Mysql索引长度和区分度
首先 索引长度和区分度是相互矛盾的, 索引长度太短,那么区分度就很低,吧索引长度加长,区分度就高,但是索引也是要占内存的,所以我们需要找到一个平衡点: 那么这个平衡点怎么来定? 比如用户表有个字段 ...
- maven仓库配置
apache官方提供的maven库下载速度比较慢,所以可以配置成aliyun的maven库,这样在构建项目的时候速度会提升很多,具体方法如下: vim /usr/local/maven/conf/se ...
- HDU1160:FatMouse's Speed(最长上升子序列,不错的题)
题目:http://acm.hdu.edu.cn/showproblem.php?pid=1160 学的东西还是不深入啊,明明会最长上升子序列,可是还是没有A出这题,反而做的一点思路没有,题意就不多说 ...
- 用tophat和cufflinks分析RNAseq数据[转载]
转自:http://blog.sciencenet.cn/home.php?mod=space&uid=635619&do=blog&id=884213 //今天看到一篇非常好 ...
- matplotlib显示栅格图片
参考自Matplotlib Python 画图教程 (莫烦Python)(13)_演讲•公开课_科技_bilibili_哔哩哔哩 https://www.bilibili.com/video/av16 ...