power network 电网——POJ1459
Time Limit: 2000MS | Memory Limit: 32768K | |
Total Submissions: 27282 | Accepted: 14179 |
Description
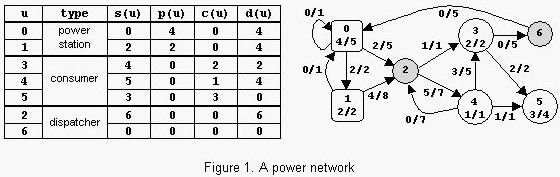
An example is in figure 1. The label x/y of power station u shows that p(u)=x and pmax(u)=y. The label x/y of consumer u shows that c(u)=x and cmax(u)=y. The label x/y of power transport line (u,v) shows that l(u,v)=x and lmax(u,v)=y. The power consumed is Con=6. Notice that there are other possible states of the network but the value of Con cannot exceed 6.
Input
Output
Sample Input
2 1 1 2 (0,1)20 (1,0)10 (0)15 (1)20
7 2 3 13 (0,0)1 (0,1)2 (0,2)5 (1,0)1 (1,2)8 (2,3)1 (2,4)7
(3,5)2 (3,6)5 (4,2)7 (4,3)5 (4,5)1 (6,0)5
(0)5 (1)2 (3)2 (4)1 (5)4
Sample Output
15
6
Hint
Source
while(scanf("%d%d%d%d",&n,&np,&nc,&m))
”
while(scanf("%d%d%d%d",&n,&np,&nc,&m)==4)
——————————————————————————————————————————————————————————
1 #include<cstdio>
2 #include<iostream>
3 #include<cstring>
4 #include<vector>
5 #include<queue>
6
7 using namespace std;
8 int n,np,nc,m;
9 int map[105][105];
10 bool vis[105];
11 int lays[105];
12 bool bfs()
13 {
14 queue<int>q;
15 memset(lays,-1,sizeof(lays));
16 q.push(n);
17 lays[n]=0;
18 while(!q.empty())
19 {
20 int u=q.front();
21 q.pop();
22 for(int i=0;i<=n+1;i++)
23 if(map[u][i]>0&&lays[i]==-1)
24 {
25 lays[i]=lays[u]+1;
26 if(i==n+1)return 1;
27 else
28 {
29 q.push(i);
30 }
31 }
32 }
33 return 0;
34 }
35 int dinic()
36 {
37 vector<int>q;
38 int maxf=0;
39 while(bfs())
40 {
41 q.push_back(n);
42 memset(vis,0,sizeof(vis));
43 vis[n]=1;
44 while(!q.empty())
45 {
46 int nd=q.back();
47 if(nd==n+1)
48 {
49 int minx=0x7fffffff,minn;
50 for(int i=1;i<q.size();i++)
51 {
52 int u=q[i-1],v=q[i];
53 if(map[u][v]<minx)
54 {
55 minx=map[u][v];
56 minn=u;
57 }
58 }
59 maxf+=minx;
60 for(int i=1;i<q.size();i++)
61 {
62 int u=q[i-1],v=q[i];
63 map[u][v]-=minx;
64 map[v][u]+=minx;
65 }
66 while(!q.empty()&&q.back()!=minn)
67 {
68 vis[q.back()]=0;
69 q.pop_back();
70 }
71 }
72 else
73 {
74 int i;
75 for(i=0;i<=n+1;i++)
76 {
77 if(map[nd][i]>0&&!vis[i]&&lays[i]==lays[nd]+1)
78 {
79 vis[i]=1;
80 q.push_back(i);
81 break;
82 }
83 }
84 if(i>n+1)q.pop_back();
85 }
86 }
87 }
88 return maxf;
89 }
90 int main()
91 {
92 char s[35];
93 while(scanf("%d%d%d%d",&n,&np,&nc,&m)==4)
94 {
95 memset(map,0,sizeof(map));
96 for(int i=0;i<m;i++)
97 {
98 int u,v,l;
99 scanf("%s",s);
100 sscanf(s,"(%d,%d)%d",&u,&v,&l);
101 map[u][v]+=l;
102 }
103 for(int i=0;i<np;i++)
104 {
105 int v,l;
106 scanf("%s",s);
107 sscanf(s,"(%d)%d",&v,&l);
108 map[n][v]+=l;
109 }
110 for(int i=0;i<nc;i++)
111 {
112 int v,l;
113 scanf("%s",s);
114 sscanf(s,"(%d)%d",&v,&l);
115 map[v][n+1]+=l;
116 }
117 printf("%d\n",dinic());
118 }
119
120 return 0;
121 }
power network 电网——POJ1459的更多相关文章
- POJ1459 Power Network —— 最大流
题目链接:https://vjudge.net/problem/POJ-1459 Power Network Time Limit: 2000MS Memory Limit: 32768K Tot ...
- poj1459 Power Network (多源多汇最大流)
Description A power network consists of nodes (power stations, consumers and dispatchers) connected ...
- POJ1459 Power Network(网络最大流)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total S ...
- POJ1459 - Power Network
原题链接 题意简述 原题看了好几遍才看懂- 给出一个个点,条边的有向图.个点中有个源点,个汇点,每个源点和汇点都有流出上限和流入上限.求最大流. 题解 建一个真 · 源点和一个真 · 汇点.真 · 源 ...
- POJ1459:Power Network(多源点多汇点的最大流)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 31086 Accepted: 15986 题 ...
- POJ 1459 Power Network / HIT 1228 Power Network / UVAlive 2760 Power Network / ZOJ 1734 Power Network / FZU 1161 (网络流,最大流)
POJ 1459 Power Network / HIT 1228 Power Network / UVAlive 2760 Power Network / ZOJ 1734 Power Networ ...
- Power Network(网络流最大流 & dinic算法 + 优化)
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 24019 Accepted: 12540 D ...
- Power Network 分类: POJ 2015-07-29 13:55 3人阅读 评论(0) 收藏
Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 24867 Accepted: 12958 Descr ...
- poj 1459 Power Network : 最大网络流 dinic算法实现
点击打开链接 Power Network Time Limit: 2000MS Memory Limit: 32768K Total Submissions: 20903 Accepted: ...
随机推荐
- Daphile 安装手册 -- 官方文档译文 [原创]
Daphile 安装手册(Daphile Installation) 英文原文:https://www.daphile.com/download/DaphileInstallation.pdf 采集日 ...
- Eureka Server启动过程分析
1.首先,SpringCloud充分利用了SpringBoot的自动装配特点 eureka-server的jar包,发现在META-INF下面的配置文件spring.factories,里面记录了Sp ...
- TurtleBot3 Waffle (tx2版华夫)(7)底盘测试
说明:opencr本身带有自测底盘功能,通过按opencr的sw1和sw2来自检底盘是否正确安装和运行: 7.1.前进测试 1)测试前,先把小车架空,轮子不要着地: 2)接好电源后,打开opencr的 ...
- ThreadX应用笔记:内核初始化和任务调度
作者:zzssdd2 E-mail:zzssdd2@foxmail.com 一.前言 了解ThreadX的初始化流程有助于移植使用,掌握任务的的调度有助于更加得心应手地运用该实时操作系统. 二.初始化 ...
- .NET的并发编程(TPL编程)是什么?
写在前面 优秀软件的一个关键特征就是具有并发性.过去的几十年,我们可以进行并发编程,但是难度很大.以前,并发性软件的编写.调试和维护都很难,这导致很多开发人员为图省事放弃了并发编程.新版 .NET 中 ...
- mysql中的基本注入函数
1. 常见数据库注入函数: MYSQL: and length((user))>10 ACCESS: and (select count() from MSysAccessObject)> ...
- 【Docker】安装docker18.09.6后,无法启动
------------------------------------------------------------------------------------------------- | ...
- 【Linux】centos 7中,开机不执行rc.lcoal中的命令
最近将一些需要开机启动的命令添加到了rc.local中 本想着开机就启动了,很省事 但是一次意外的重启,发现rc.local中的全部命令都没有执行 发现问题后,及时查找 参考:https://blog ...
- 写给 Linux 初学者的一封信
大家好,我是肖邦. 这篇文章是写给 Linux 初学者的,我会分享一些作为初学者应该知道的一些东西,这些内容都是本人从事 Linux 开发工作多年的心得体会,相信会对初学者有所帮助.如果你是 Linu ...
- uni-app开发经验分享四: 实现文字复制到选择器中
这里分享一个我经常用到的一个方法,主要是用来复制文字内容,具体代码如下: var that=this; if(!document){ uni.setClipboardData({ data:复制的值, ...