Ants(二分图最佳完美匹配)
Ants
Time Limit: 5000MS | Memory Limit: 65536K | |||
Total Submissions: 6904 | Accepted: 2164 | Special Judge |
Description
Young naturalist Bill studies ants in school. His ants feed on plant-louses that live on apple trees. Each ant colony needs its own apple tree to feed itself.
Bill has a map with coordinates of n ant colonies and n apple trees. He knows that ants travel from their colony to their feeding places and back using chemically tagged routes. The routes cannot intersect each other or ants will get confused and get to the wrong colony or tree, thus spurring a war between colonies.
Bill would like to connect each ant colony to a single apple tree so that all n routes are non-intersecting straight lines. In this problem such connection is always possible. Your task is to write a program that finds such connection.
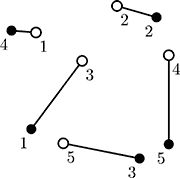
On this picture ant colonies are denoted by empty circles and apple trees are denoted by filled circles. One possible connection is denoted by lines.
Input
The first line of the input file contains a single integer number n (1 ≤ n ≤ 100) — the number of ant colonies and apple trees. It is followed by n lines describing n ant colonies, followed by n lines describing n apple trees. Each ant colony and apple tree is described by a pair of integer coordinates x and y (−10 000 ≤ x, y ≤ 10 000) on a Cartesian plane. All ant colonies and apple trees occupy distinct points on a plane. No three points are on the same line.
Output
Write to the output file n lines with one integer number on each line. The number written on i-th line denotes the number (from 1 to n) of the apple tree that is connected to the i-th ant colony.
Sample Input
5
-42 58
44 86
7 28
99 34
-13 -59
-47 -44
86 74
68 -75
-68 60
99 -60
Sample Output
4
2
1
5
3
Source
//# include <bits/stdc++.h>
# include <iostream>
# include <stdio.h>
# include <string.h>
# include <math.h>
using namespace std;
# define eps 1e-
# define LL long long
# define INF 1e20
# define MX
struct Node{
double x,y;
}ant[MX], tree[MX]; int n;
double W[MX][MX];
double Lx[MX], Ly[MX];
int link[MX]; //右边匹配左的
bool S[MX], T[MX]; double dist(int x,int y){
return sqrt((ant[x].x-tree[y].x)*(ant[x].x-tree[y].x)+(ant[x].y-tree[y].y)*(ant[x].y-tree[y].y));
} bool match(int p)
{
S[p]=;
for (int i=;i<=n;i++)
{
if (!T[i]&&(fabs(Lx[p]+Ly[i]-W[p][i])<=eps))
{
T[i]=;
if (link[i]==- || match(link[i]))
{
link[i]=p;
return ;
}
}
}
return ;
} void update()
{
double del = INF;
for (int i=;i<=n;i++) if(S[i])
for (int j=;j<=n;j++) if (!T[j])
if (Lx[i]+Ly[j]-W[i][j] < del - eps)
del = Lx[i]+Ly[j]-W[i][j];
for (int i=;i<=n;i++)
{
if (S[i]) Lx[i]-=del;
if (T[i]) Ly[i]+=del;
}
} void KM()
{
memset(link,-,sizeof(link));
for (int i=;i<=n;i++)
{
Ly[i]=0.0;
Lx[i]=-INF;
for (int j=;j<=n;j++)
if (W[i][j] > Lx[i] + eps)
Lx[i] = W[i][j];
}
for (int i=;i<=n;i++)
{
while ()
{
memset(S,,sizeof(S));
memset(T,,sizeof(T));
if (match(i)) break;
else update();
}
}
} int main()
{
while (scanf("%d",&n)!=EOF)
{
for (int i=;i<=n;i++)
scanf("%lf%lf",&ant[i].x, &ant[i].y);
for (int i=;i<=n;i++)
scanf("%lf%lf",&tree[i].x, &tree[i].y);
for (int i=;i<=n;i++)
for(int j=;j<=n;j++)
W[i][j] = -dist(i,j);
KM();
for(int i = ; i <= n; ++i)
{
for(int j = ; j <= n; ++j)
{
if(link[j] == i)
{
printf("%d\n",j);
break;
}
}
}
}
return ;
}
Ants(二分图最佳完美匹配)的更多相关文章
- UVa1349 Optimal Bus Route Design(二分图最佳完美匹配)
UVA - 1349 Optimal Bus Route Design Time Limit: 3000MS Memory Limit: Unknown 64bit IO Format: %lld & ...
- UVa 11383 少林决胜(二分图最佳完美匹配)
https://vjudge.net/problem/UVA-11383 题意: 给定一个N×N矩阵,每个格子里都有一个正整数W(i,j).你的任务是给每行确定一个整数row(i),每列也确定一个整数 ...
- UVA - 1045 The Great Wall Game(二分图最佳完美匹配)
题目大意:给出棋盘上的N个点的位置.如今问将这些点排成一行或者一列.或者对角线的最小移动步数(每一个点都仅仅能上下左右移动.一次移动一个) 解题思路:暴力+二分图最佳完美匹配 #include < ...
- 【LA4043 训练指南】蚂蚁 【二分图最佳完美匹配,费用流】
题意 给出n个白点和n个黑点的坐标,要求用n条不相交的线段把他们连接起来,其中每条线段恰好连接一个白点和一个黑点,每个点恰好连接一条线段. 分析 结点分黑白,很容易想到二分图.其中每个白点对应一个X结 ...
- Uva1349Optimal Bus Route Design(二分图最佳完美匹配)(最小值)
题意: 给定n个点的有向图问,问能不能找到若干个环,让所有点都在环中,且让权值最小,KM算法求最佳完美匹配,只不过是最小值,所以把边权变成负值,输出时将ans取负即可 这道题是在VJ上交的 #incl ...
- ZOJ-3933 Team Formation (二分图最佳完美匹配)
题目大意:n个人,分为两个阵营.现在要组成由若干支队伍,每支队伍由两个人组成并且这两个人必须来自不同的阵营.同时,每个人都有m个厌恶的对象,并且厌恶是相互的.相互厌恶的人不能组成一支队伍.问最多能组成 ...
- UVa 1349 - Optimal Bus Route Design(二分图最佳完美匹配)
链接: https://uva.onlinejudge.org/index.php?option=com_onlinejudge&Itemid=8&page=show_problem& ...
- POJ 3565 Ants(最佳完美匹配)
Description Young naturalist Bill studies ants in school. His ants feed on plant-louses that live on ...
- UVALive 4043 Ants 蚂蚁(二分图最佳完美匹配,KM算法)
题意: 有n个蚂蚁n棵树,蚂蚁与树要配对,在配对成功的一对之间连一条线段,要求所有线段不能相交.按顺序输出蚂蚁所匹配的树. 思路: 这个题目真是技巧啊,不能用贪心来为每个蚂蚁选择最近的树,这样很可能是 ...
随机推荐
- 程序员之---C语言细节24(段错误、类型提升、sizeof 'A')
主要内容:段错误.类型提升.sizeof 'A' #include <stdio.h> int main() { union test{ char a[10]; int b; }u; i ...
- Memory Barriers
这回该进入主题了. 上一文最后提到了 Memory Barriers ,即内存屏障.由于对一个 CPU 而言,a = 1; b = 1. 由于在中间加了内存屏障,在 X86 架构下,就 ...
- pytz 格式化北京时间 6分钟问题
使用datetime直接构造时间的时候,设置时区是没有北京时间的,一般来说习惯了linux的同志都会默认用上海时间来代替,这里却有一个问题,如果要进行时区转换,上海时间比北京时间差6分钟... 比如: ...
- hdu 1348 Wall (凸包模板)
/* 题意: 求得n个点的凸包.然后求与凸包相距l的外圈的周长. 答案为n点的凸包周长加上半径为L的圆的周长 */ # include <stdio.h> # include <ma ...
- jetty.xml解析
我们知道jetty有一种启动方式是在jetty的根目录中运行命令行:java -jar start.jar,这个命令会调用apache的XmlConfiguration工具类作为启动类,这个类会默认读 ...
- 联想电脑Win8升级win10后Wlan关闭无法开启解决办法
官网下载电源驱动,下载无线网上驱动 开启电脑 按fn+f5 电源管理界面就出来了 把无线网卡打开 就ok了 这样就开启了无线! 如果还不行,可进行如下尝试,希望有所帮助: 1.开机进bios(一般是按 ...
- 简体字丶冯|服务网关kong-docker安装
tags: kong ,服务网关,docker安装教程 grammar_cjkRuby: true --- 作为一名技术探索者,想了解一个未知系统的最有效方法就是去用.然而搭建一个陌生系统的最快捷方法 ...
- unity, UGUI Image shader
Image组件的Material成员默认是空,如果想为Image添加shader,只需新建material赋给Material即可. 另外注意,用于UI组件的shader都要包含一句:ZTest ...
- rxjs来啦
var text = document.querySelector('#text'); var inputStream = Rx.Observable.fromEvent(text, 'keyup') ...
- php使用N层加密eval gzinflate str_rot13 base64 破解方法汇总
php使用N层加密eval gzinflate str_rot13 base64 破解方法汇总 来源:本站转载 作者:佚名 时间:2011-02-14 TAG: 我要投稿 PHP使用eval(gzin ...