POJ 1691 Painting a Board(状态压缩DP)
Description
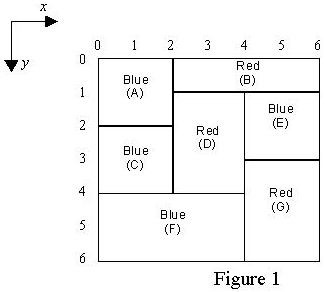
To color the board, the APM has access to a set of brushes. Each brush has a distinct color C. The APM picks one brush with color C and paints all possible rectangles having predefined color C with the following restrictions:
To avoid leaking the paints and mixing colors, a rectangle can only be painted if all rectangles immediately above it have already been painted. For example rectangle labeled F in Figure 1 is painted only after rectangles C and D are painted. Note that each rectangle must be painted at once, i.e. partial painting of one rectangle is not allowed.
You are to write a program for APM to paint a given board so that the number of brush pick-ups is minimum. Notice that if one brush is picked up more than once, all pick-ups are counted.
Input
Note that:
- Color-code is an integer in the range of 1 .. 20.
- Upper left corner of the board coordinates is always (0,0).
- Coordinates are in the range of 0 .. 99.
- N is in the range of 1..15.
Output
Sample Input
1
7
0 0 2 2 1
0 2 1 6 2
2 0 4 2 1
1 2 4 4 2
1 4 3 6 1
4 0 6 4 1
3 4 6 6 2
Sample Output
3
思路:
1. 拓扑排序加深搜
2. 拓扑排序加广搜
3. 状态压缩DP. 设 dp[s][i] 表示当前状态为 s 时, 刚画完第 i 个矩形所用的最少画笔数. s = [1, 1<<15), s 的二进制表示中, 第 i 位 为1 表示第 i 个矩形已经被涂完色.
dp[news][i] = min(dp[news][i], dp[olds][j]+1) if color[i] != color[j]
= min(dp[olds][j]) if color[i] == color[j]
其中, news = (olds | 1<<i)
上述状态转移方程的意思是, 要计算 dp[s][i] 的值, 那么考虑当前所有 dp[olds][j], 其中 s = (olds|1<<i), 假如 j 的颜色和 i 的颜色相同, 这不需要另拿画笔, 否则, 画笔数加 1
当然, 对 i 进行涂色需要满足 i 的直接前驱都已被涂完
总结:
1. 这道题近似于暴力破解, 枚举所有状态集合的所有状态, 在某个状态 s 下, 以 s 中以涂色的某个矩形为支点来更新一个还未被涂色的点
2. 第 48 行代码错过一次, 把 i 写成了 j
3. 第 45 行很精髓, 我本打算用一个 for 循环进行判断的
4. 第 44, 48 行, 体现了 (1) 的思想, 即以 k 为支点来更新 i
代码:
#include <iostream>
using namespace std; class tangle {
public:
int x1, y1, x2, y2;
int color;
tangle(int _x1, int _y1, int _x2, int _y2):x1(_x1), y1(_y1), x2(_x2), y2(_y2) {}
tangle() {
tangle(0, 0, 0, 0);
}
};
const int INF = 0X3F3F3F3F;
int M, N;
tangle tangles[20];
int dp[1<<15][20];
int up[20]; bool isUpper(int i, int j) {
if(tangles[i].x2 != tangles[j].x1) return false;
if(tangles[i].y1 >= tangles[j].y2) return false;
if(tangles[i].y2 <= tangles[j].y1) return false;
return true;
}
void pre_process() {
memset(up, 0, sizeof(up));
for(int i = 1; i <= N; i ++) {
for(int j = 1; j <= N; j ++) {
if(isUpper(i, j))
up[j] = (up[j]|(1<<(i-1)));
}
} memset(dp, 0x3f, sizeof(dp));
for(int i = 1; i <= N; i ++)
if(up[i] == 0)
dp[1<<(i-1)][i] = 1; }
int mainFunc() {
int END = (1<<N) -1;
for(int s = 1; s <= END; s ++) { // 从状态 s 导出 dp[s][i], 当前 s 第 i 个矩形不能被涂色
for(int i = 1; i <= N; i ++) { // 将要给第 i 个矩形涂色
if(s&(1<<(i-1)) ) continue; // 状态 s 中, 对应第 i 个矩形已经被涂完了
if((s&up[i]) != up[i]) continue; // 确保 i 的直接前驱都已涂完颜色
for(int k = 1; k <= N; k ++) {
if(!(s&(1<<(k-1)))) continue;
int news = (s|1<<(i-1)); // update 新的 dp[][]
if(tangles[i].color != tangles[k].color)
dp[news][i] = min(dp[news][i], dp[s][k]+1);
else
dp[news][i] = min(dp[news][i], dp[s][k]);
}
}
}
int ans = INF;
for(int i = 1; i <= N; i ++) {
ans = min(ans, dp[END][i]);
}
return ans;
}
int main() {
freopen("E:\\Copy\\ACM\\poj\\1691\\in.txt", "r", stdin);
cin >> M;
while( M -- >= 1) {
cin >> N;
for(int i = 1; i <= N; i ++) {
scanf("%d%d%d%d%d", &tangles[i].x1, &tangles[i].y1, &tangles[i].x2, &tangles[i].y2, &tangles[i].color);
}
pre_process();
cout << mainFunc() << endl;
}
return 0;
}
POJ 1691 Painting a Board(状态压缩DP)的更多相关文章
- poj 3311 floyd+dfs或状态压缩dp 两种方法
Hie with the Pie Time Limit: 2000MS Memory Limit: 65536K Total Submissions: 6436 Accepted: 3470 ...
- POJ 2686_Traveling by Stagecoach【状态压缩DP】
题意: 一共有m个城市,城市之间有双向路连接,一个人有n张马车票,一张马车票只能走一条路,走一条路的时间为这条路的长度除以使用的马车票上规定的马车数,问这个人从a出发到b最少使用时间. 分析: 状态压 ...
- poj 2411 Mondriaan's Dream_状态压缩dp
题意:给我们1*2的骨牌,问我们一个n*m的棋盘有多少种放满的方案. 思路: 状态压缩不懂看,http://blog.csdn.net/neng18/article/details/18425765 ...
- poj 1185 炮兵阵地 [经典状态压缩DP]
题意:略. 思路:由于每个大炮射程为2,所以如果对每一行状态压缩的话,能对它造成影响的就是上面的两行. 这里用dp[row][state1][state2]表示第row行状态为state2,第row- ...
- POJ 1038 Bug Integrated Inc(状态压缩DP)
Description Bugs Integrated, Inc. is a major manufacturer of advanced memory chips. They are launchi ...
- poj 2411 Mondriaan's Dream(状态压缩dP)
题目:http://poj.org/problem?id=2411 Input The input contains several test cases. Each test case is mad ...
- poj 2686 Traveling by Stagecoach ---状态压缩DP
题意:给出一个简单带权无向图和起止点,以及若干张马车车票,每张车票可以雇到相应数量的马. 点 u, v 间有边时,从 u 到 v 或从 v 到 u 必须用且仅用一张车票,花费的时间为 w(u, v) ...
- POJ 1185 炮兵阵地(状态压缩DP)
题解:nState为状态数,state数组为可能的状态 代码: #include <map> #include <set> #include <list> #inc ...
- POJ 3254 Corn Fields(状态压缩DP)
题目大意:给出一个M*N的矩阵,元素为0表示这个地方不能种玉米,为1表示这个地方能种玉米,现在规定所种的玉米不能相邻,即每行或者没列不能有相邻的玉米,问一共有多少种种植方法. 举个例子: 2 3 1 ...
随机推荐
- GIS+=地理信息+行业+大数据——基于云环境流处理平台下的实时交通创新型app
应用程序已经是近代的一个最重要的IT创新.应用程序是连接用户和数据之间的桥梁,提供即时訪问信息是最方便且呈现的方式也是easy理解的和令人惬意的. 然而,app开发人员.尤其是后端平台能力,一直在努力 ...
- json demo
package my.bigdata.movieTask.action; import com.alibaba.fastjson.JSON; import com.alibaba.fastjson.J ...
- 【Unity笔记】屏幕坐标和鼠标坐标
屏幕坐标:左下角为原点. void Update(){ // 获取物体的屏幕坐标,世界坐标->屏幕坐标 Vector3 screenPos = Camera.main.WorldToScreen ...
- C语言 · 整除问题
算法训练 整除问题 时间限制:1.0s 内存限制:512.0MB 问题描述 编写一个程序,输入三个正整数min.max和factor,然后对于min到max之间的每一个整数(包括mi ...
- SQL Server 大数据量insert into xx select慢的解决方案
最近项目有个需求,把一张表中的数据根据一定条件增删改到另外一张表.按理说这是个很简单的SQL.可是在实际过程中却出现了超级长时间的执行过程. 后来经过排查发现是大数据量insert into xx s ...
- JAVA 监控工具 VisualVM 插件路径配置地址
在使用VisualVM监控工具的时候,发现无法安装或者更新插件,或者报错,最后发现原来是插件中心的URL地址原装地址就不对.根据官方网站的地址配置后就正常了.下面的具体地址. VisualVMRele ...
- MVC出现错误:系统找不到指定文件(异常来自 HRSULT:0x80070002)
vs2013创建Web应用程序MVC出现错误:系统找不到指定文件(异常来自 HRSULT:0x80070002) 查到博客园VS2013新建Web Application时报错Exception fr ...
- MySql: ”Commands out of sync“Error (Connect/C++)
使用 Connector/C++ 查询 Mysql , 连续调用存储过程时 会出现如下: Commands out of sync; you can't run this command now,st ...
- 去掉CSS中的表达式Expression
在IE中,CSS是可以嵌入js表达式的,可以在CSS类中定义,但是将含有表达CSS类从DOM对象中移除,样式表达式是不会失效的. 经过研究找到了答案,需要使用js调用style对象的removeExp ...
- 利用百度地图API根据地址查询经纬度
传上来只是为了记录下三种jsonp方式,$.get(url, callback)方式不行,会出错 -- 必须指明返回类型为”json”才行. 或者使用$.getJSON()或者$.ajax({}). ...