[iOS基础控件 - 6.6.1] 展示团购数据代码
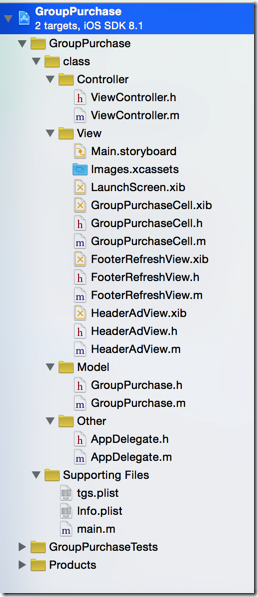
- 1 //
- 2 // ViewController.m
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import "ViewController.h"
- 10 #import "GroupPurchase.h"
- 11 #import "GroupPurchaseCell.h"
- 12 #import "FooterRefreshView.h"
- 13 #import "HeaderAdView.h"
- 14
- 15
- 16 @interface ViewController () <FooterRefreshViewDelegate>
- 17
- 18 @property(nonatomic, strong) NSArray *groupPurchases;
- 19
- 20 @end
- 21
- 22 @implementation ViewController
- 23
- 24 - (void)viewDidLoad {
- 25 [super viewDidLoad];
- 26 // Do any additional setup after loading the view, typically from a nib.
- 27
- 28 // 创建尾部控件
- 29 FooterRefreshView *footerView = [FooterRefreshView footerRrefreshViewWithDelegate:self];
- 30
- 31 // 设置尾部控件
- 32 self.tableView.tableFooterView = footerView;
- 33
- 34
- 35 //设置头部广告
- 36 HeaderAdView *adView = [self genAdView]; // 手动拼装广告图片数据
- 37 self.tableView.tableHeaderView = adView;
- 38 }
- 39
- 40 - (void)didReceiveMemoryWarning {
- 41 [super didReceiveMemoryWarning];
- 42 // Dispose of any resources that can be recreated.
- 43 }
- 44
- 45 #pragma mark - 状态栏
- 46 - (BOOL)prefersStatusBarHidden {
- 47 return YES;
- 48 }
- 49
- 50
- 51 #pragma mark - 数据方法
- 52 /** 延迟数据加载到model */
- 53 - (NSArray *) groupPurchases {
- 54 if (nil == _groupPurchases) {
- 55 NSArray *dictArray = [NSArray arrayWithContentsOfFile:[[NSBundle mainBundle] pathForResource:@"tgs.plist" ofType:nil]];
- 56
- 57 NSMutableArray *mdictArray = [NSMutableArray array];
- 58 for (NSDictionary *dict in dictArray) {
- 59 GroupPurchase *groupPurchase = [GroupPurchase groupPurchaseWithDictionary:dict];
- 60 [mdictArray addObject:groupPurchase];
- 61 }
- 62
- 63 _groupPurchases = mdictArray;
- 64 }
- 65
- 66 return _groupPurchases;
- 67 }
- 68
- 69 /** section数 */
- 70 - (NSInteger)numberOfSectionsInTableView:(UITableView *)tableView {
- 71 return 1;
- 72 }
- 73
- 74 /** 行数 */
- 75 - (NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section {
- 76 return self.groupPurchases.count;
- 77 }
- 78
- 79 /** 内容 */
- 80 - (UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath {
- 81
- 82 // 1.创建cell
- 83 GroupPurchaseCell *cell = [GroupPurchaseCell groupPurchaseCellWithTableView:self.tableView];
- 84
- 85 // 2.给cell传递model数据
- 86 cell.groupPurchase = self.groupPurchases[indexPath.row];
- 87
- 88 return cell;
- 89 }
- 90
- 91 /** 行高 */
- 92 - (CGFloat)tableView:(UITableView *)tableView heightForRowAtIndexPath:(NSIndexPath *)indexPath {
- 93 return 80;
- 94 }
- 95
- 96 /** 实现FooterRefreshViewDelegate协议 */
- 97 - (void)footerRefreshViewClickedFooterRefreshButton:(FooterRefreshView *)footerRefreshView {
- 98 //增加团购记录
- 99 GroupPurchase *groupPurchase = [GroupPurchase groupPurchase];
- 100 groupPurchase.icon = @"M2Mini.jpg";
- 101 groupPurchase.title = @"增加用例";
- 102 groupPurchase.price = @"88";
- 103 groupPurchase.buyCount = @"32";
- 104
- 105 // 加入新数据
- 106 NSMutableArray *marray = [NSMutableArray arrayWithArray:self.groupPurchases];
- 107 [marray addObject:groupPurchase];
- 108 self.groupPurchases = marray;
- 109
- 110 // 刷新数据
- 111 [self.tableView reloadData];
- 112 }
- 113
- 114 // 配置一些头部广告的数据
- 115 - (HeaderAdView *) genAdView {
- 116 HeaderAdView *adView = [HeaderAdView headerAdView];
- 117
- 118 NSMutableArray *adImages = [NSMutableArray array];
- 119 for (int i=0; i<5; i++) {
- 120 [adImages addObject:[NSString stringWithFormat:@"ad_%02d", i]];
- 121 }
- 122
- 123 adView.ads = adImages;
- 124
- 125 return adView;
- 126 }
- 127
- 128 @end

- 1 //
- 2 // GroupPurchaseCell.m
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import "GroupPurchaseCell.h"
- 10 #import "GroupPurchase.h"
- 11
- 12 @implementation GroupPurchaseCell
- 13
- 14 // 界面被初始化的时候调用 awakeFromNib
- 15 - (void)awakeFromNib {
- 16 // Initialization code
- 17 }
- 18
- 19 - (void)setSelected:(BOOL)selected animated:(BOOL)animated {
- 20 [super setSelected:selected animated:animated];
- 21
- 22 // Configure the view for the selected state
- 23 }
- 24
- 25 /** 自定初始化的类方法,传入model数据 */
- 26 + (instancetype) groupPurchaseCellWithTableView:(UITableView *) tableView {
- 27
- 28 static NSString *ID = @"groupPurchase";
- 29
- 30 // 从缓存池寻找
- 31 GroupPurchaseCell *cell = [tableView dequeueReusableCellWithIdentifier:ID];
- 32
- 33 // 若缓存池没有,则创建一个
- 34 if (nil == cell) {
- 35 cell = [[[NSBundle mainBundle] loadNibNamed:@"GroupPurchaseCell" owner:nil options:nil] lastObject];
- 36 }
- 37
- 38 return cell;
- 39 }
- 40
- 41 /** 加载Model数据,初始化界面 */
- 42 - (void) setGroupPurchase:(GroupPurchase *) groupPurchase {
- 43 if (nil != groupPurchase) {
- 44 self.titleLabel.text = groupPurchase.title;
- 45 self.iconImageView.image = [UIImage imageNamed:groupPurchase.icon];
- 46 self.priceLabel.text = [NSString stringWithFormat:@"¥%@", groupPurchase.price];
- 47 self.buyCountLabel.text = [NSString stringWithFormat:@"%@人已经购买", groupPurchase.buyCount];
- 48 }
- 49
- 50 _groupPurchase = groupPurchase;
- 51 }
- 52
- 53 @end
- 54
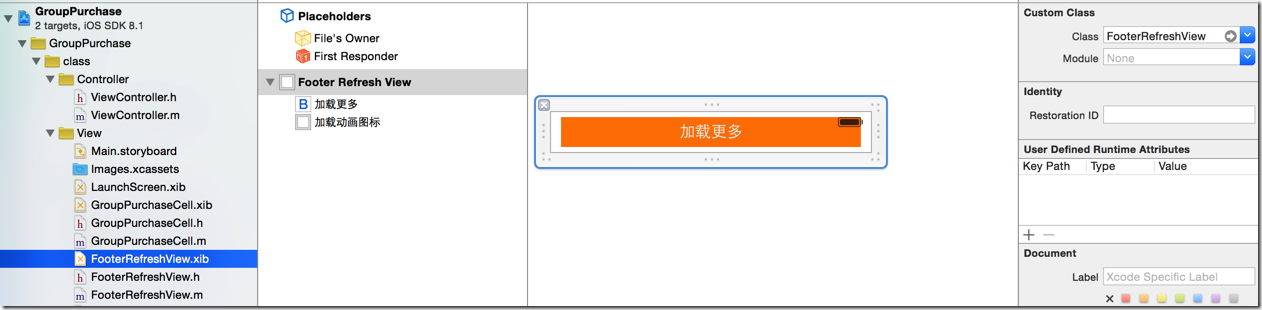
- 1 //
- 2 // FooterRefreshView.h
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import <UIKit/UIKit.h>
- 10
- 11 @class FooterRefreshView;
- 12
- 13 // 定义delegate协议
- 14 @protocol FooterRefreshViewDelegate <NSObject>
- 15
- 16 @optional
- 17 - (void) footerRefreshViewClickedFooterRefreshButton:(FooterRefreshView *) footerRefreshView;
- 18
- 19 @end
- 20
- 21 @interface FooterRefreshView : UIView
- 22
- 23 // 带代理的初始化方法
- 24 + (instancetype) footerRrefreshViewWithDelegate:(id<FooterRefreshViewDelegate>) delegate;
- 25
- 26 @end
- 1 //
- 2 // FooterRefreshView.m
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import "FooterRefreshView.h"
- 10
- 11 @interface FooterRefreshView()
- 12 /** 底部的“加载更多”按钮 */
- 13 @property (weak, nonatomic) IBOutlet UIButton *footerRefreshButton;
- 14
- 15 /** 加载动画图标 */
- 16 @property (weak, nonatomic) IBOutlet UIActivityIndicatorView *loadingImage;
- 17
- 18 /** 代理 */
- 19 @property(nonatomic, weak) id<FooterRefreshViewDelegate> delegate;
- 20
- 21 /** “加载更多”按钮点击事件 */
- 22 - (IBAction)onFooterRefreshButtonClicked;
- 23
- 24
- 25 @end
- 26
- 27 @implementation FooterRefreshView
- 28
- 29 #pragma mark - 初始化
- 30
- 31 /** 初始化方法 */
- 32 + (instancetype) footerRrefreshViewWithDelegate:(id<FooterRefreshViewDelegate>) delegate {
- 33 FooterRefreshView *footerRefreshView = [[[NSBundle mainBundle] loadNibNamed:@"FooterRefreshView" owner:nil options:nil] lastObject];
- 34
- 35 if (nil != delegate) {
- 36 footerRefreshView.delegate = delegate;
- 37 }
- 38
- 39 return footerRefreshView;
- 40 }
- 41
- 42 // xib控件的初始化调用方法
- 43 - (void)awakeFromNib {
- 44 self.loadingImage.hidden = YES;
- 45 }
- 46
- 47
- 48 #pragma mark - action
- 49 /** “加载更多”按钮点击事件 */
- 50 - (IBAction)onFooterRefreshButtonClicked {
- 51 NSString *footerButtonTitle = self.footerRefreshButton.currentTitle;
- 52
- 53 // 显示正在加载
- 54 self.loadingImage.hidden = NO;
- 55 [self.footerRefreshButton setTitle:@"拼命加载中..." forState:UIControlStateNormal];
- 56
- 57 // 暂时禁止按钮事件
- 58 self.footerRefreshButton.userInteractionEnabled = NO;
- 59
- 60 // 模拟延迟
- 61 dispatch_after(dispatch_time(DISPATCH_TIME_NOW, (int64_t)(1.0 * NSEC_PER_SEC)), dispatch_get_main_queue(), ^{
- 62 // 通知代理发生了点击事件
- 63 if ([self.delegate respondsToSelector:@selector(footerRefreshViewClickedFooterRefreshButton:)]) {
- 64 [self.delegate footerRefreshViewClickedFooterRefreshButton:self];
- 65 }
- 66
- 67 // 恢复按钮状态
- 68 self.footerRefreshButton.userInteractionEnabled = YES;
- 69 self.loadingImage.hidden = YES;
- 70 [self.footerRefreshButton setTitle:footerButtonTitle forState:UIControlStateNormal];
- 71 });
- 72
- 73 }
- 74
- 75 @end
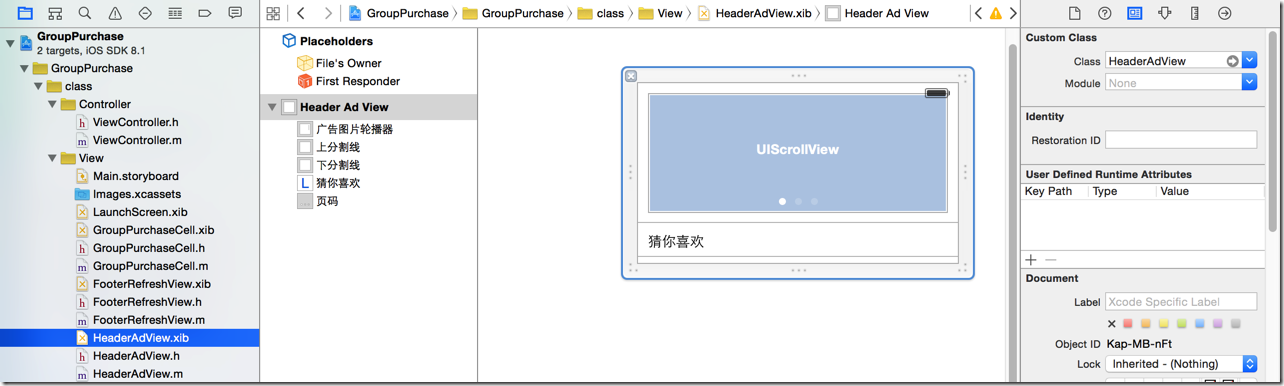
- 1 //
- 2 // HeaderAdView.h
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import <UIKit/UIKit.h>
- 10
- 11 @interface HeaderAdView : UIView
- 12 // 广告组
- 13 @property(nonatomic, strong) NSArray *ads;
- 14
- 15 + (instancetype) headerAdView;
- 16
- 17 @end
- 1 //
- 2 // HeaderAdView.m
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import "HeaderAdView.h"
- 10
- 11 #define AD_VIEW_WIDTH 300
- 12 #define AD_VIEW_HEIGHT 120
- 13
- 14 // 遵守UIScrollViewDelegate监控滚动事件
- 15 @interface HeaderAdView() <UIScrollViewDelegate>
- 16
- 17 /** scrollView控件 */
- 18 @property (weak, nonatomic) IBOutlet UIScrollView *scrollView;
- 19
- 20 /** 页码 */
- 21 @property (weak, nonatomic) IBOutlet UIPageControl *pageControl;
- 22
- 23 /** 计时器 */
- 24 @property(nonatomic, weak) NSTimer *timer;
- 25
- 26 @end
- 27
- 28 @implementation HeaderAdView
- 29
- 30 #pragma mark - 初始化方法
- 31 // 初始化headerAdView
- 32 + (instancetype) headerAdView {
- 33 return [[[NSBundle mainBundle] loadNibNamed:@"HeaderAdView" owner:nil options:nil] lastObject];
- 34 }
- 35
- 36 // 当控件从nib初始化
- 37 - (void)awakeFromNib {
- 38 self.scrollView.scrollEnabled = YES; // 开启滚动
- 39 self.scrollView.pagingEnabled = YES; // 开启翻页模式
- 40 self.scrollView.delegate = self;// 设置代理
- 41 }
- 42
- 43 /** 设置ads */
- 44 - (void) setAds:(NSArray *)ads {
- 45 if (nil != ads) {
- 46 CGFloat adImageWidth = AD_VIEW_WIDTH;
- 47 CGFloat adImageHeight = AD_VIEW_HEIGHT;
- 48 CGFloat adImageY = 0;
- 49
- 50 for (int i=0; i<ads.count; i++) {
- 51 // 计算当前图片的水平坐标
- 52 CGFloat adImageX = i * adImageWidth;
- 53
- 54 UIImageView *adImageView = [[UIImageView alloc] initWithFrame:CGRectMake(adImageX, adImageY, adImageWidth, adImageHeight)];
- 55 adImageView.image = [UIImage imageNamed:[NSString stringWithFormat:@"%@", ads[i]]];
- 56
- 57 [self.scrollView addSubview:adImageView];
- 58 }
- 59
- 60 // 设置滚动范围
- 61 self.scrollView.contentSize = CGSizeMake(ads.count * AD_VIEW_WIDTH, 0);
- 62
- 63 self.pageControl.numberOfPages = ads.count; // 总页数
- 64 self.pageControl.pageIndicatorTintColor = [UIColor blackColor]; // 其他页码颜色
- 65 self.pageControl.currentPageIndicatorTintColor = [UIColor redColor]; // 当前页码颜色
- 66
- 67 // 添加自动轮播
- 68 [self addTimer];
- 69 }
- 70
- 71 _ads = ads;
- 72 }
- 73
- 74 #pragma mark - 滚动事件
- 75 // 滚动动作监控,当滚动超过图片的一半的时候转变页码
- 76 - (void)scrollViewDidScroll:(UIScrollView *)scrollView {
- 77 CGFloat scrollViewWidth = self.scrollView.frame.size.width;
- 78 int page = (self.scrollView.contentOffset.x + 0.5 * scrollViewWidth) / scrollViewWidth;
- 79 self.pageControl.currentPage = page;
- 80 }
- 81
- 82 // 手动拖动广告图片轮播的时候,暂时销毁自动轮播器
- 83 - (void)scrollViewWillBeginDragging:(UIScrollView *)scrollView {
- 84 [self.timer invalidate];
- 85 self.timer = nil;
- 86 }
- 87
- 88 // 手动拖动结束,从新加上自动轮播器
- 89 - (void)scrollViewWillEndDragging:(UIScrollView *)scrollView withVelocity:(CGPoint)velocity targetContentOffset:(inout CGPoint *)targetContentOffset {
- 90 [self addTimer];
- 91 }
- 92
- 93 // 添加计时器
- 94 - (void) addTimer {
- 95 self.timer = [NSTimer scheduledTimerWithTimeInterval:2.0 target:self selector:@selector(nextImage) userInfo:nil repeats:YES];
- 96
- 97 // 计时器分享主线程资源
- 98 [[NSRunLoop currentRunLoop] addTimer:self.timer forMode:NSRunLoopCommonModes];
- 99 }
- 100
- 101 // 下一张图片动作
- 102 - (void) nextImage {
- 103 int page = 0;
- 104 // 如果是当前页码在最后一页,从新回到第一页
- 105 if (self.pageControl.currentPage == self.ads.count - 1) {
- 106 page = 0;
- 107 }
- 108 else {
- 109 page = self.pageControl.currentPage + 1;
- 110 }
- 111
- 112 // 滚动图片,带动画效果
- 113 [self.scrollView setContentOffset:CGPointMake(page * AD_VIEW_WIDTH, 0) animated:YES];
- 114 }
- 115 @end
- 1 //
- 2 // GroupPurchase.h
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import <Foundation/Foundation.h>
- 10
- 11 @interface GroupPurchase : NSObject
- 12
- 13 /** 图片 */
- 14 @property(nonatomic, copy) NSString *icon;
- 15
- 16 /** 标题 */
- 17 @property(nonatomic, copy) NSString *title;
- 18
- 19 /** 已经购买人数 */
- 20 @property(nonatomic, copy) NSString *buyCount;
- 21
- 22 /** 价格 */
- 23 @property(nonatomic, copy) NSString *price;
- 24
- 25 //初始化方法
- 26 - (instancetype) initWithDictionary:(NSDictionary *) dictionary;
- 27 + (instancetype) groupPurchaseWithDictionary:(NSDictionary *) dictionary;
- 28 + (instancetype) groupPurchase;
- 29
- 30 @end
- 1 //
- 2 // GroupPurchase.m
- 3 // GroupPurchase
- 4 //
- 5 // Created by hellovoidworld on 14/12/3.
- 6 // Copyright (c) 2014年 hellovoidworld. All rights reserved.
- 7 //
- 8
- 9 #import "GroupPurchase.h"
- 10
- 11 @implementation GroupPurchase
- 12
- 13 //初始化方法
- 14 - (instancetype) initWithDictionary:(NSDictionary *) dictionary
- 15 {
- 16 if (self = [super init]) {
- 17 // 使用KVC
- 18 [self setValuesForKeysWithDictionary:dictionary];
- 19 }
- 20
- 21 return self;
- 22 }
- 23
- 24 + (instancetype) groupPurchaseWithDictionary:(NSDictionary *) dictionary {
- 25 return [[self alloc] initWithDictionary:dictionary];
- 26 }
- 27
- 28 + (instancetype) groupPurchase {
- 29 return [self groupPurchaseWithDictionary:nil];
- 30 }
- 31
- 32 @end
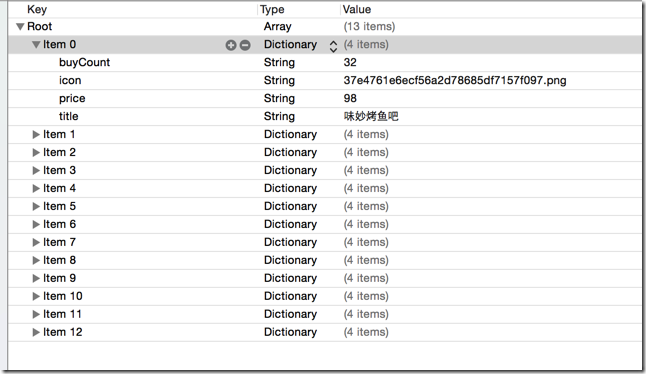
[iOS基础控件 - 6.6.1] 展示团购数据代码的更多相关文章
- [iOS基础控件 - 6.7] 微博展示 使用代码自定义TableCell(动态尺寸)
A.需求 1.类似于微博内容的展示 2.头像 3.名字 4.会员标志 5.内容 6.分割线 7.配图(可选,可有可无) code source: https://github.com/hellov ...
- [iOS基础控件 - 5.2] 查看大图、缩放图片代码(UIScrollView制作)
原图: 900 x 1305 拖曳滚动: 缩放: 主要代码: // // ViewController.m // ImageZoom // // Created by ...
- [iOS基础控件 - 5.5] 代理设计模式 (基于”APP列表"练习)
A.概述 在"[iOS基础控件 - 4.4] APP列表 进一步封装,初见MVC模式”上进一步改进,给“下载”按钮加上效果.功能 1.按钮点击后,显示为“已下载”,并且不 ...
- [iOS基础控件 - 6.7.1] 微博展示 代码
Controller: // // ViewController.m // Weibo // // Created by hellovoidworld on 14/12/4. // Copyrig ...
- [iOS基础控件 - 6.6] 展示团购数据 自定义TableViewCell
A.需求 1.头部广告 2.自定义cell:含有图片.名称.购买数量.价格 3.使用xib设计自定义cell,自定义cell继承自UITableViewCell 4.尾部“加载更多按钮”,以及其被点击 ...
- iOS 基础控件(下)
上篇介绍了UIButton.UILabel.UIImageView和UITextField,这篇就简短一点介绍UIScrollView和UIAlertView. UIScrollView 顾名思义也知 ...
- [iOS基础控件 - 6.9] 聊天界面Demo
A.需求 做出一个类似于QQ.微信的聊天界面 1.每个cell包含发送时间.发送人(头像).发送信息 2.使用对方头像放在左边,我方头像在右边 3.对方信息使用白色背景对话框,我方信息使用蓝色背景对话 ...
- [iOS基础控件 - 7.0] UIWebView
A.基本使用 1.概念 iOS内置的浏览器控件 Safari浏览器就是通过UIWebView实现的 2.用途:制作简易浏览器 (1)基本请求 创建请求 加载请求 (2)代理监听webView加载, ...
- [iOS基础控件 - 6.11.3] 私人通讯录Demo 控制器的数据传递、存储
A.需求 1.搭建一个"私人通讯录"Demo 2.模拟登陆界面 账号 密码 记住密码开关 自动登陆开关 登陆按钮 3.退出注销 4.增删改查 5.恢复数据(取消修改) 这个代码 ...
随机推荐
- c缺陷与陷阱笔记-第一章 词法陷阱
1.运算符的贪心性,匹配最长的运算符,例如 n-->0,从-开始,-是运算符,--是运算符,-->就不是,所以是 n -- > 0,--是 a---b,-是,--是,,---不是,所 ...
- A过的题目
1.TreeMap和TreeSet类:A - Language of FatMouse ZOJ1109B - For Fans of Statistics URAL 1613 C - Hardwood ...
- POJ3660——Cow Contest(Floyd+传递闭包)
Cow Contest DescriptionN (1 ≤ N ≤ 100) cows, conveniently numbered 1..N, are participating in a prog ...
- P66、面试题8:旋转数组的最小数字
题目:把一个数组最开始的若干个元素搬到数组的末尾,我们称之为数组的旋转.输入一个递增排序的数组的一个旋转,输出旋转数组的最小元素.例如数组{3,4,5,1,2}为{1,2,3,4,5}的一个旋转,该数 ...
- socket关闭动作以及socket状态的总结
主要部分,四次握手: 断开连接其实从我的角度看不区分客户端和服务器端,任何一方都可以调用close(or closesocket)之类的函数开始主动终止一个连接.这里先暂时说正常情况.当调用close ...
- Lambda表达式 =>(msdn)
using System; using System.Collections.Generic; using System.Linq; using System.Text; using System.T ...
- 1671. Anansi's Cobweb(并查集)
1671 并查集 对于询问删除边之后的连通块 可以倒着加边 最后再倒序输出 #include <iostream> #include<cstdio> #include<c ...
- innodb master主线程
http://wenku.baidu.com/link?url=MhY9yhHTgeOlyooWDvaVfPkW3cuVSX_rIZv2QtCu7GLeEuqSfYh_M7Yvl1N4IY08a3ws ...
- Java内省
什么是内省? Java语言对bean类属性.事件的一种缺省处理方法,例如类A中有属性name,那我们可以通过getName,setName来得到其值或者设置新的值. 什么是JavaBean? Java ...
- ajax withCredentials在firefox下问题的解释
1,起因: 跨域的问题一般有两种解决方式比较常用,一是使用jsonp,二是服务端配置cors策略.至于jsonp这里不再赘述,本文主要解释一下cors解决跨域产生的问题 2,cors跨域产生的问题 j ...