HDU 1710-Binary Tree Traversals(二进制重建)
Binary Tree Traversals
Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 3340 Accepted Submission(s): 1500
or ordered. They are preorder, inorder and postorder. Let T be a binary tree with root r and subtrees T1,T2.
In a preorder traversal of the vertices of T, we visit the root r followed by visiting the vertices of T1 in preorder, then the vertices of T2 in preorder.
In an inorder traversal of the vertices of T, we visit the vertices of T1 in inorder, then the root r, followed by the vertices of T2 in inorder.
In a postorder traversal of the vertices of T, we visit the vertices of T1 in postorder, then the vertices of T2 in postorder and finally we visit r.
Now you are given the preorder sequence and inorder sequence of a certain binary tree. Try to find out its postorder sequence.
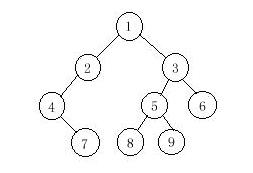
they are always correspond to a exclusive binary tree.
9
1 2 4 7 3 5 8 9 6
4 7 2 1 8 5 9 3 6
7 4 2 8 9 5 6 3 1今天刷二叉树专题,搞了半天最终对重建有了一点认识,首先说一下由先序和中序重建二叉树,大体思路是大概是这种:由于先序(pre)的第一个字母肯定是根节点。所以要在中序(ins)序列中找到根节点的位置k,然后递归重建根节点的左子树和右子树。此时要把左子树和右子树当成根节点,然后表示出它们的位置,左子树非常好说,pre+1就是它的位置。对于右子树来说,pre+k+1为它的位置。如图
对于由中序和后序重建,思路跟上面大体一样,详细写法有点区别。这个就要在中序序列中找到后序的最后一个字母(即根节点),然后递归重建左子树和右子树,依然是把左子树和右子树当成根节点。左子树的位置为pos+k-1,但传的參数应该是pos (由于我们要找的字母是pos[n-1]),而右子树的位置在后序的倒数第二个字母。传參数为pos+k。(不是pos+k+1,想明确这个就算真明确了)
另为这篇没写由后序和中序重建的代码,曾经写过,在这点击打开链接#include <cstdio>
#include <iostream>
#include <algorithm>
#include <cstring>
#include <cctype>
#include <cmath>
#include <cstdlib>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
using namespace std;
const int INF=1<<27;
const int maxn=5100;
typedef struct node
{
int data;
node *l,*r;
}*T;
void rbuild(T &root,int *pre,int *ins,int n)
{
if(n<=0)
root=NULL;
else
{
root=new node;
int k=find(ins,ins+n,pre[0])-ins;
root->data=pre[0];
rbuild(root->l,pre+1,ins,k);
rbuild(root->r,pre+k+1,ins+k+1,n-k-1);
}
}
int ans[maxn],p;
void last(T root)
{
if(root)
{
last(root->l);
last(root->r);
ans[p++]=root->data;
}
}
int main()
{
int n,i;
while(cin>>n){
T root;int pre[maxn],ins[maxn];
for(i=0;i<n;i++)
cin>>pre[i];
for(i=0;i<n;i++)
cin>>ins[i];
rbuild(root,pre,ins,n);
p=0;
last(root);
for(i=0;i<p;i++)
if(i!=p-1)
cout<<ans[i]<<" ";
else
cout<<ans[i]<<endl;
}
return 0;
}
版权声明:本文博客原创文章,博客,未经同意,不得转载。
HDU 1710-Binary Tree Traversals(二进制重建)的更多相关文章
- hdu 1710 Binary Tree Traversals 前序遍历和中序推后序
题链;http://acm.hdu.edu.cn/showproblem.php?pid=1710 Binary Tree Traversals Time Limit: 1000/1000 MS (J ...
- HDU 1710 Binary Tree Traversals (二叉树遍历)
Binary Tree Traversals Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/O ...
- HDU 1710 Binary Tree Traversals(树的建立,前序中序后序)
Binary Tree Traversals Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/O ...
- HDU 1710 Binary Tree Traversals(二叉树)
题目地址:HDU 1710 已知二叉树先序和中序求后序. #include <stdio.h> #include <string.h> int a[1001], cnt; ty ...
- HDU 1710 Binary Tree Traversals(二叉树遍历)
传送门 Description A binary tree is a finite set of vertices that is either empty or consists of a root ...
- 【二叉树】hdu 1710 Binary Tree Traversals
acm.hdu.edu.cn/showproblem.php?pid=1710 [题意] 给定一棵二叉树的前序遍历和中序遍历,输出后序遍历 [思路] 根据前序遍历和中序遍历递归建树,再后续遍历输出 m ...
- HDU 1710 Binary Tree Traversals
题意:给出一颗二叉树的前序遍历和中序遍历,输出其后续遍历 首先知道中序遍历是左子树根右子树递归遍历的,所以只要找到根节点,就能够拆分出左右子树 前序遍历是按照根左子树右子树递归遍历的,那么可以找出这颗 ...
- hdu 1701 (Binary Tree Traversals)(二叉树前序中序推后序)
Binary Tree Traversals T ...
- HDU 1710 二叉树的遍历 Binary Tree Traversals
Binary Tree Traversals Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/O ...
- hdu1710(Binary Tree Traversals)(二叉树遍历)
Binary Tree Traversals Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/O ...
随机推荐
- 【Java探索道路安全系列:Java可扩展的安全架构】一间:Java可扩展的安全体系结构开始
笔者:郭嘉 邮箱:allenwells@163.com 博客:http://blog.csdn.net/allenwells github:https://github.com/AllenWell [ ...
- iOS_16_开关控制器_modal_代码方法
最后效果图: watermark/2/text/aHR0cDovL2Jsb2cuY3Nkbi5uZXQvcHJlX2VtaW5lbnQ=/font/5a6L5L2T/fontsize/400/fill ...
- 利用SVNKit进行版本库的树的导出
public List searchByTree(String userName,String passwd,String SVNServerUrl,String dirUrl){ //这里有点像 s ...
- Cloudera hadoop-2.3.0-cdh5.1.0 在Centos 6.5 下的安装
安装前准备 1. 虚拟机3个.安装Centos 6.5, 内存设置为4GB 2. 配置yum源为163(不配置,在安装软件时,慢的要死) 3. 关闭防火墙( iptables).disabled ...
- Spark1.0.0 属性配置
1:Spark1.0.0属性配置方式 Spark属性提供了大部分应用程序的控制项,而且能够单独为每一个应用程序进行配置. 在Spark1.0.0提供了3种方式的属性配置: Sp ...
- Xamarin 安装步骤
原文:Xamarin 安装步骤 1.下载并解压吾乐吧软件站提供的“Mono for Android 离线包” 安装:jdk-6u45-windows-i586.exe (就算你是64位系统,也要安装i ...
- 手把手教popupWindow从下往上,以达到流行效果
效果如图所看到的,点击開始button,popWindow从下往上出来,再点击popWindow外面,popWindow又从上往下消失 能够看出来,上面的popupWindow是半透明的,后面我会细说 ...
- linux下一个C语言flock功能使用 .
表头文件 #include<sys/file.h> 定义函数 int flock(int fd,int operation); 函数说明 flock()会依參数operation所指 ...
- C#新DataColumn类Type生成的方法类型参数
DataColumn有的需要等级Type构造类型的参数,如以下: // // 摘要: // 使用指定列名称和数据类型初始化 System.Data.DataColumn 类的新实例. // // 參数 ...
- ArcGIS SDE 10.1 for Postgresql 服务连接配置
去年写了ArcGIS 10.1 如何连接Postgresql 数据库(http://blog.csdn.net/arcgis_all/article/details/8202709)当时采用的也是Ar ...