【WPF】 OxyPlot图表控件学习
最近在学习OxyPlot图表控件,一些基本的学习心得,在这里记录一下,方便以后进行查找。

- xmlns:oxy="http://oxyplot.org/wpf"
- 1 <Grid>
- 2 <oxy:PlotView Model="{Binding Model}" />
- 3 </Grid>
- 1 private PlotModel model;
- 2 public PlotModel Model
- 3 {
- 4 get { return model; }
- 5 set { SetProperty(ref model, value); }
- 6 }
- 1 Private void GetLine()
- 2 {
- 3 var tmp = new PlotModel
- 4 {
- 5 Title = "Demo", //图表的Titile
- 6 Subtitle = "直线" //图表的说明
- 7 };
- 8 var series2 = new LineSeries
- 9 {
- 10 Title = "Series 2", //线的说明
- 11 MarkerType = MarkerType.Square //标记点 的类型、形状
- 12 };
- 13 series2.Points.Add(new DataPoint(0, 0));//添加线的第一个点坐标
- 14 series2.Points.Add(new DataPoint(4, 4));//添加线的第二个点的坐标
- 15 tmp.Series.Add(series2);//将线添加到图标的容器中
- 16 this.Model = tmp;//赋值
- 17 }
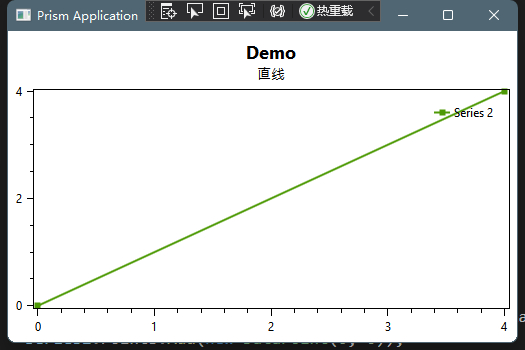
- 1 Private void GetSpline()
- 2 {
- 3 var lineSeries1 = new LineSeries { Title = "Series 1", MarkerType = MarkerType.Circle };
- 4 //画曲线主要是需要将线的 InterpolationAlgorithm 属性 设置为 CanonicalSpline 就可变成曲线
- 5 lineSeries1.InterpolationAlgorithm = InterpolationAlgorithms.CanonicalSpline;
- 6 lineSeries1.Points.Add(new DataPoint(0, 20));
- 7 lineSeries1.Points.Add(new DataPoint(10, 21));
- 8 lineSeries1.Points.Add(new DataPoint(20, 24));
- 9 lineSeries1.Points.Add(new DataPoint(30, 22));
- 10 lineSeries1.Points.Add(new DataPoint(40, 17));
- 11 lineSeries1.Points.Add(new DataPoint(50, 21));
- 12 lineSeries1.Points.Add(new DataPoint(60, 23));
- 13 lineSeries1.Points.Add(new DataPoint(70, 27));
- 14 lineSeries1.Points.Add(new DataPoint(80, 27));
- 15 lineSeries1.Points.Add(new DataPoint(90, 22));
- 16 lineSeries1.Points.Add(new DataPoint(100, 25));
- 17 tmp.Series.Add(lineSeries1);
- 18 this.Model = tmp;
- 19 }
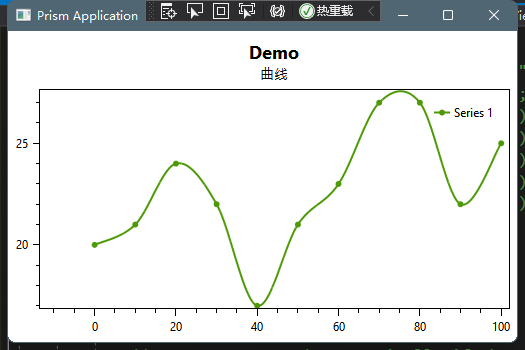
- 1 Private void GetSpline()
- 2 {
- 3 //将线进行填充 ,主要是将线的类型改为 AreaSeries 即可 但是此时是不会显示Mark点
- 4 var lineSeries1 = new AreaSeries { Title = "Series 1", MarkerType = MarkerType.Circle };
- 5
- 6 //画曲线主要是需要将线的 InterpolationAlgorithm 属性 设置为 CanonicalSpline 就可变成曲线
- 7 lineSeries1.InterpolationAlgorithm = InterpolationAlgorithms.CanonicalSpline;
- 8 lineSeries1.Points.Add(new DataPoint(0, 20));
- 9 lineSeries1.Points.Add(new DataPoint(10, 21));
- 10 lineSeries1.Points.Add(new DataPoint(20, 24));
- 11 lineSeries1.Points.Add(new DataPoint(30, 22));
- 12 lineSeries1.Points.Add(new DataPoint(40, 17));
- 13 lineSeries1.Points.Add(new DataPoint(50, 21));
- 14 lineSeries1.Points.Add(new DataPoint(60, 23));
- 15 lineSeries1.Points.Add(new DataPoint(70, 27));
- 16 lineSeries1.Points.Add(new DataPoint(80, 27));
- 17 lineSeries1.Points.Add(new DataPoint(90, 22));
- 18 lineSeries1.Points.Add(new DataPoint(100, 25));
- 19 tmp.Series.Add(lineSeries1);
- 20 this.Model = tmp;
- 21 }
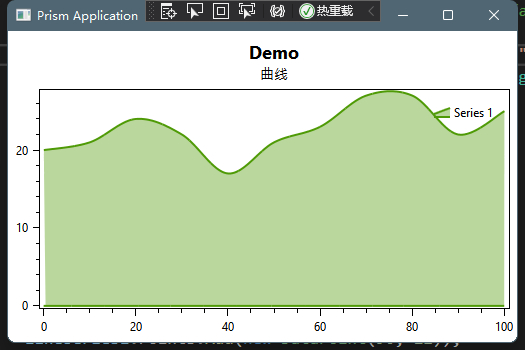
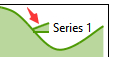
- 1 var tmp = new PlotModel { Title = "Demo", Subtitle = "曲线" };
- 2 tmp.LegendBackground = OxyColor.FromArgb(200, 255, 255, 255);//设置背景颜色
- 3 tmp.LegendBorder = OxyColors.Transparent;//设置边框颜色
- 4 tmp.LegendOrientation = LegendOrientation.Horizontal;//设置标识对其方式
- 5 tmp.LegendPlacement = LegendPlacement.Outside;//设置标识在图标中的相对位置 是在里面还是在外面
- 6 tmp.LegendPosition = LegendPosition.BottomLeft;//设置标识在整个容器中的位置 此时是左下角
- 7
- 8 //将线进行填充 ,主要是将线的类型改为 AreaSeries 即可 但是此时是不会显示Mark点
- 9 var lineSeries1 = new AreaSeries { Title = "Series 1", MarkerType = MarkerType.Circle };
- 10 //画曲线主要是需要将线的 InterpolationAlgorithm 属性 设置为 CanonicalSpline 就可变成曲线
- 11 lineSeries1.InterpolationAlgorithm = InterpolationAlgorithms.CanonicalSpline;
- 12 lineSeries1.Points.Add(new DataPoint(0, 20));
- 13 lineSeries1.Points.Add(new DataPoint(10, 21));
- 14 lineSeries1.Points.Add(new DataPoint(20, 24));
- 15 lineSeries1.Points.Add(new DataPoint(30, 22));
- 16 lineSeries1.Points.Add(new DataPoint(40, 17));
- 17 lineSeries1.Points.Add(new DataPoint(50, 21));
- 18 lineSeries1.Points.Add(new DataPoint(60, 23));
- 19 lineSeries1.Points.Add(new DataPoint(70, 27));
- 20 lineSeries1.Points.Add(new DataPoint(80, 27));
- 21 lineSeries1.Points.Add(new DataPoint(90, 22));
- 22 lineSeries1.Points.Add(new DataPoint(100, 25));
- 23 tmp.Series.Add(lineSeries1);
- 24 this.Model = tmp;
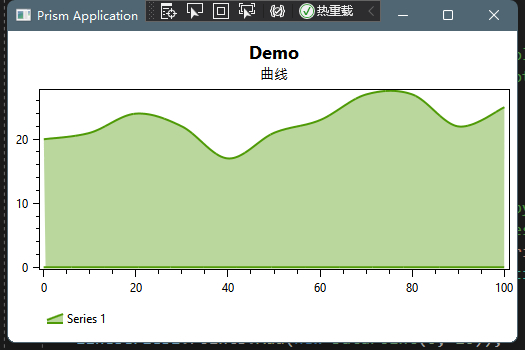
- 1 var tmp = new PlotModel { Title = "Demo", Subtitle = "曲线" };
- 2 tmp.LegendBackground = OxyColor.FromArgb(200, 255, 255, 255);//设置背景颜色
- 3 tmp.LegendBorder = OxyColors.Transparent;//设置边框颜色
- 4 tmp.LegendOrientation = LegendOrientation.Horizontal;//设置标识对其方式
- 5 tmp.LegendPlacement = LegendPlacement.Outside;//设置标识在图标中的相对位置 是在里面还是在外面
- 6 tmp.LegendPosition = LegendPosition.BottomLeft;//设置标识在整个容器中的位置 此时是左下角
- 7
- 8 //line1
- 9 //将线进行填充 ,主要是将线的类型改为 AreaSeries 即可 但是此时是不会显示Mark点
- 10 var lineSeries1 = new AreaSeries { Title = "Series 1", MarkerType = MarkerType.Circle };
- 11 //画曲线主要是需要将线的 InterpolationAlgorithm 属性 设置为 CanonicalSpline 就可变成曲线
- 12 lineSeries1.InterpolationAlgorithm = InterpolationAlgorithms.CanonicalSpline;
- 13 lineSeries1.Points.Add(new DataPoint(0, 20));
- 14 lineSeries1.Points.Add(new DataPoint(10, 21));
- 15 lineSeries1.Points.Add(new DataPoint(20, 24));
- 16 lineSeries1.Points.Add(new DataPoint(30, 22));
- 17 lineSeries1.Points.Add(new DataPoint(40, 17));
- 18 lineSeries1.Points.Add(new DataPoint(50, 21));
- 19 lineSeries1.Points.Add(new DataPoint(60, 23));
- 20 lineSeries1.Points.Add(new DataPoint(70, 27));
- 21 lineSeries1.Points.Add(new DataPoint(80, 27));
- 22 lineSeries1.Points.Add(new DataPoint(90, 22));
- 23 lineSeries1.Points.Add(new DataPoint(100, 25));
- 24 tmp.Series.Add(lineSeries1);
- 25
- 26 //Line2
- 27 var series2 = new LineSeries { Title = "Series 2", MarkerType = MarkerType.Square };
- 28 series2.Points.Add(new DataPoint(0, 0));
- 29 series2.Points.Add(new DataPoint(4, 4));
- 30 series2.Points.Add(new DataPoint(10, 12));
- 31 series2.Points.Add(new DataPoint(20, 16));
- 32 series2.Points.Add(new DataPoint(30, 25));
- 33 series2.Points.Add(new DataPoint(40, 5));
- 34 tmp.Series.Add(series2);
- 35
- 36 this.Model = tmp;
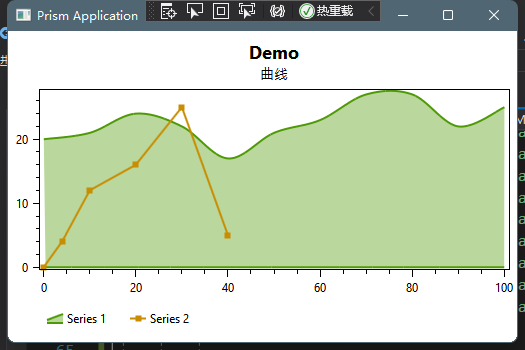
- 1 var tmp = new PlotModel { Title = "Demo", Subtitle = "曲线" };
- 2 tmp.LegendBackground = OxyColor.FromArgb(200, 255, 255, 255);
- 3 tmp.LegendBorder = OxyColors.Transparent;
- 4 tmp.LegendOrientation = LegendOrientation.Horizontal;
- 5 tmp.LegendPlacement = LegendPlacement.Outside;
- 6 tmp.LegendPosition = LegendPosition.BottomLeft;
- 7 tmp.LegendSymbolLength = 24;
- 8
- 9 var linearAxis1 = new LinearAxis();
- 10 linearAxis1.MajorGridlineStyle = LineStyle.Solid;
- 11 linearAxis1.MinorGridlineStyle = LineStyle.Dot;
- 12 linearAxis1.Title = "Y";
- 13 linearAxis1.Minimum = 0;
- 14 linearAxis1.Maximum = 35;
- 15 tmp.Axes.Add(linearAxis1);
- 16
- 17 var linearAxis2 = new LinearAxis();
- 18 linearAxis2.MajorGridlineStyle = LineStyle.Solid;
- 19 linearAxis2.MinorGridlineStyle = LineStyle.Dot;
- 20 linearAxis2.Position = AxisPosition.Bottom;
- 21 linearAxis2.Title = "X";
- 22 tmp.Axes.Add(linearAxis2);
- 23
- 24 var lineSeries1 = new LineSeries { Title = "Series 1", MarkerType = MarkerType.Circle };
- 25 lineSeries1.LabelFormatString = "{1}";
- 26 lineSeries1.Points.Add(new DataPoint(0, 20));
- 27 lineSeries1.Points.Add(new DataPoint(10, 21));
- 28 lineSeries1.Points.Add(new DataPoint(20, 24));
- 29 lineSeries1.Points.Add(new DataPoint(30, 22));
- 30 lineSeries1.Points.Add(new DataPoint(40, 17));
- 31 lineSeries1.Points.Add(new DataPoint(50, 21));
- 32 lineSeries1.Points.Add(new DataPoint(60, 23));
- 33 lineSeries1.Points.Add(new DataPoint(70, 27));
- 34 lineSeries1.Points.Add(new DataPoint(80, 27));
- 35 lineSeries1.Points.Add(new DataPoint(90, 22));
- 36 tmp.Series.Add(lineSeries1);
- 37
- 38 var series2 = new LineSeries { Title = "Series 2", MarkerType = MarkerType.Square };
- 39 series2.Points.Add(new DataPoint(0, 0));
- 40 series2.Points.Add(new DataPoint(4, 4));
- 41 series2.Points.Add(new DataPoint(10, 12));
- 42 series2.Points.Add(new DataPoint(20, 16));
- 43 series2.Points.Add(new DataPoint(30, 25));
- 44 series2.Points.Add(new DataPoint(40, 5));
- 45
- 46 tmp.Series.Add(series2);
- 47 this.Model = tmp;
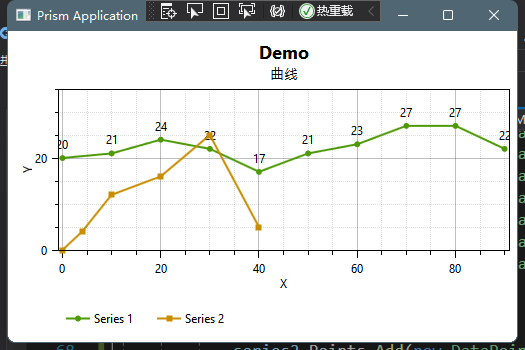
【WPF】 OxyPlot图表控件学习的更多相关文章
- WPF 曲线图表控件(自制)(二)
原文:WPF 曲线图表控件(自制)(二) 版权声明:本文为博主原创文章,未经博主允许不得转载. https://blog.csdn.net/koloumi/article/details/775218 ...
- WPF 曲线图表控件(自制)(一)
原文:WPF 曲线图表控件(自制)(一) 版权声明:本文为博主原创文章,未经博主允许不得转载. https://blog.csdn.net/koloumi/article/details/775092 ...
- WPF Visifire 图表控件
Visifire WPF 图表控件 破解 可能用WPF生成过图表的开发人员都知道,WPF虽然本身的绘图能力强大,但如果每种图表都自己去实现一次的话可能工作量就大了, 尤其是在开发时间比较紧的情况下.这 ...
- C# WPF DevExpress 图表控件之柱状图
说明:DevExpress版本是17.1.VS是2015. XAML: <!--#region 图表控件--> <dxc:ChartControl x:Name="char ...
- WPF 自定义UI控件学习
最近项目中运用到了WPF处理三维软件,在C/S结构中WPF做UI还是有很多优越性,简单的学了一点WPF知识,成功的完成项目目标.项目过度阶段对于WPF的一些基本特点有了进一步了解 .至此花费一点时间研 ...
- 图表控件== 百度 echarts的入门学习
花了3天的时间 去学习跟试用之前两款的图表控件 hightcharts(商业,人性化,新手非常方便试用,图表少了点) 跟chartjs==>搭配vue更好 控件,整体而言都还可以. http:/ ...
- Visifire For WPF 图表控件 如何免费
可能用WPF生成过图表的开发人员都知道,WPF虽然本身的绘图能力强大,但如果每种图表都自己去实现一次的话可能工作量就大了, 尤其是在开发时间比较紧的情况下.这时候有必要借助一种专业的图表工具. Vis ...
- 图表控件的学习===》hightChart 和 Chartjs的使用
hightChart : 比较旧的图表控件 商业需要授权 Chartjs 免费开源 刚开始使用了下 hightchart 然后参考示例 建了对应的参数配置的类, 也顺利的集合到后台动态传输. 后 ...
- ASP.NET Core MVC TagHelper实践HighchartsNET快速图表控件-开源
ASP.NET Core MVC TagHelper最佳实践HighchartsNET快速图表控件支持ASP.NET Core. 曾经在WebForms上写过 HighchartsNET快速图表控件- ...
随机推荐
- P5147-数学-随机数生成器
P5147-数学-随机数生成器 (洛谷第一篇题解说这是高一数学题,新高二感觉到被吊打) 我们设work(x)的期望值为\(f_x\) 注意\(f_1\)是边界.不过对下列式子没有影响.原因参照必修的数 ...
- SSM中如何上传图片
1.文件配置 2.jsp页面 文件的name值不能跟数据库列名一致 3.控制层收集数据转发到逻辑层 4.逻辑层处理把用户信息存到数据库 5.注册成功后跳到jsp页面进行展示
- odoo里API解读
Odoo自带的api装饰器主要有:model,multi,one,constrains,depends,onchange,returns 七个装饰器. multimulti则指self是多个记录的合集 ...
- java构造器级简单内存分析
java构造器的使用(基础篇) 构造方法也叫构造器,是创建对象时执行的特殊方法,一般用于初始化新对象的属性. 基本定义语法: 访问控制符 构造方法名([参数列表]){ 方法体 } 注:"访问 ...
- DS1515+
DS1515+ 2021年05月02日 0 2.4k aahk DS1515+ 2021年05月02日 这篇文章用于记录.改进.提高.分享我在使用群晖DS1515+网络存储服务器过程中的具体操作. ...
- HttpRunner3源码阅读:7.响应后处理 response.py
response 上一篇说的client.py来发送请求,这里就来看另一个response.py,该文件主要是完成测试断言方法 可用资料 jmespath[json数据取值处理]: https://g ...
- 100的累加和 while 循环
//100的累加和 while 循环 #include <stdio.h> int main() { int sum = 0; //5050 int i = 0; while(i < ...
- 使用Freemarker导出Word文档(包含图片)代码实现及总结
.personSunflowerP { background: rgba(51, 153, 0, 0.66); border-bottom: 1px solid rgba(0, 102, 0, 1); ...
- nagios介绍和安装
官方support文献: https://support.nagios.com/kb/ 1.Nagios的监控模式: 主动式检查:NCPA.NRPE nagios安装后默认使用主动检查方式,远程执行代 ...
- Linux从头学07:中断那么重要,它的本质到底是什么?
作 者:道哥,10+年的嵌入式开发老兵. 公众号:[IOT物联网小镇],专注于:C/C++.Linux操作系统.应用程序设计.物联网.单片机和嵌入式开发等领域. 公众号回复[书籍],获取 Linux. ...