Chapter 5(串)
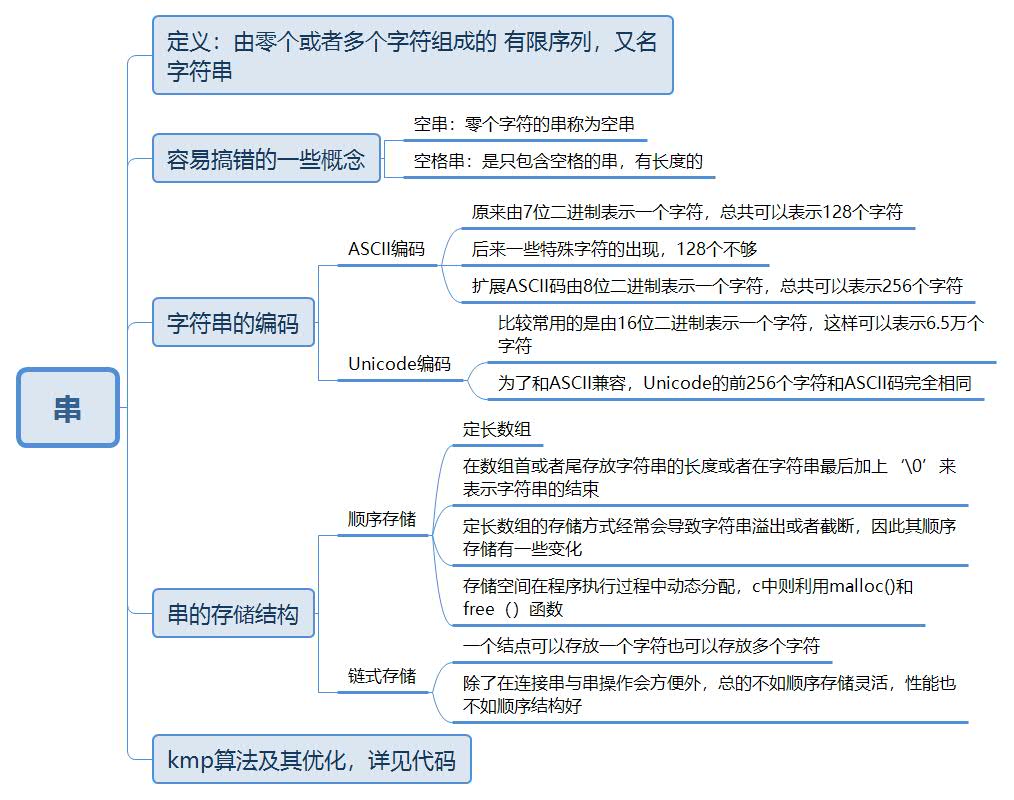
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
void get_nextval(char *str,int *nextval)
{
int i,j;
i = 0;
j = -1;
nextval[0] = -1;
int len = strlen(str);
while(i < len)
{
if(j==-1 || str[j]==str[i])//str[i]表示后缀的单个字符,str[j]表示前缀的单个字符
{
++i;
++j;
if(str[i] != str[j]) //若当前字符与前缀字符不同,则当前的j为nextvale在i位置的值
{
nextval[i] = j;
}
else //否则将前缀字符的nextval值赋给后缀字符的nextval
{
nextval[i] = nextval[j];
}
}
else
{
j = nextval[j];//若字符不同,则j值回溯
}
}
}
void get_next(char *str,int *next)
{
int i,j;
i = 0;
j = -1;
next[0] = -1;
int len = strlen(str);
while(i < len)
{
if(j==-1 || str[i]==str[j])
{
++i;
++j;
next[i] = j;
}
else
{
j = next[j];
}
}
}
int Index_KMP(char *strF,char *strS,int pos)
{
int i = pos;
int j = 0;//字串中当前位置下标值
int next[255];
get_nextval(strS,next);
int lenF = strlen(strF);
int lenS = strlen(strS);
while(i < lenF && j < lenS) //若i小于lenF且j小于lenS,循环继续
{
if(j == -1 || strF[i]==strS[j])//两字符相等或者j为-1则继续
{
++i;
++j;
}
else
{
j = next[j];//j回退合适的位置,i不变
}
}
if(j >= lenS)return i-lenS;//必须要由j使循环退出才说明找到了这个子串的位置
else return 0;
}
int main()
{
char *strF = "abcdecdefg";
char *strS = "defg";
printf("%d \n",Index_KMP(strF,strS,0));
return 0;
}
#include <stdio.h>
#include <stdlib.h>
#include <stdbool.h>
#include <string.h>
void get_nextval(char *str,int *nextval)
{
int i,j;
i = 0;
j = -1;
nextval[0] = -1;
int len = strlen(str);
while(i < len)
{
if(j==-1 || str[j]==str[i])//str[i]表示后缀的单个字符,str[j]表示前缀的单个字符
{
++i;
++j;
if(str[i] != str[j]) //若当前字符与前缀字符不同,则当前的j为nextvale在i位置的值
{
nextval[i] = j;
}
else //否则将前缀字符的nextval值赋给后缀字符的nextval
{
nextval[i] = nextval[j];
}
}
else
{
j = nextval[j];//若字符不同,则j值回溯
}
}
}
void get_next(char *str,int *next)
{
int i,j;
i = 0;
j = -1;
next[0] = -1;
int len = strlen(str);
while(i < len)
{
if(j==-1 || str[i]==str[j])
{
++i;
++j;
next[i] = j;
}
else
{
j = next[j];
}
}
}
int Index_KMP(char *strF,char *strS,int pos)
{
int i = pos;
int j = 0;//字串中当前位置下标值
int next[255];
get_nextval(strS,next);
int lenF = strlen(strF);
int lenS = strlen(strS);
while(i < lenF && j < lenS) //若i小于lenF且j小于lenS,循环继续
{
if(j == -1 || strF[i]==strS[j])//两字符相等或者j为-1则继续
{
++i;
++j;
}
else
{
j = next[j];//j回退合适的位置,i不变
}
}
if(j >= lenS)return i-lenS;//必须要由j使循环退出才说明找到了这个子串的位置
else return 0;
}
int main()
{
char *strF = "abcdecdefg";
char *strS = "defg";
printf("%d \n",Index_KMP(strF,strS,0));
return 0;
}
附件列表
Chapter 5(串)的更多相关文章
- 《深入理解计算机系统》 Chapter 7 读书笔记
<深入理解计算机系统>Chapter 7 读书笔记 链接是将各种代码和数据部分收集起来并组合成为一个单一文件的过程,这个文件可被加载(货被拷贝)到存储器并执行. 链接的时机 编译时,也就是 ...
- TIJ——Chapter One:Introduction to Objects
///:~容我对这个系列美其名曰"读书笔记",其实shi在练习英文哈:-) Introduction to Objects Object-oriented programming( ...
- WAP调用微信支付https://pay.weixin.qq.com/wiki/doc/api/wap.php?chapter=15_1
公司做的一个购物网站 之前微信版的网站要搬在webView上 可是微信支付是个问题 , 在外部浏览器怎么都发不起微信请求 , 原因是因为页面调用的微信浏览器自带JSAPI 在外部浏览器无法调用,但 ...
- Chapter 5. Label and Entry Widgets 标签和输入部件
Chapter 5. Label and Entry Widgets 标签和输入部件 有时候,你需要用户输入特定的信息,比如他们的名字,地址或者 甚至序号. 简单的方式来实现这个是使用Enry 部件 ...
- 梦殇 chapter three
chapter three 悲伤有N个层面.对于生命是孤独的底色,对于时间是流动的伤感,对于浪漫是起伏的变奏,对于善和怜悯是终生的慨叹…… 出去和舍友买完东西,刚回到宿舍,舍友就说,刚才有人给你打电话 ...
- (转)MapReduce Design Patterns(chapter 6 (part 1))(十一)
Chapter 6. Metapatterns 这种模式不是解决某个问题的,而是处理模式的关系的.可以理解为“模式的模式”.首先讨论的是job链,把几个模式联合起来解决复杂的,有多个阶段要处理的问题. ...
- C++ Primer(第五版)读书笔记 & 习题解答 --- Chapter 2
Chapter 2.1 1. 数据类型决定了程序中数据和操作的意义. 2. C++定义了一套基本数据类型,其中包括算术类型和一个名为void的特殊类型.算术类型包含了字符.整型.布尔值以及浮点数.vo ...
- 零元学Expression Blend 4 - Chapter 29 ListBox与Button结合运用的简单功能
原文:零元学Expression Blend 4 - Chapter 29 ListBox与Button结合运用的简单功能 本章所讲的是运用ListBox.TextBox与Button,做出简单的列表 ...
- 零元学Expression Blend 4 – Chapter 20 以实作案例学习Childwindow
原文:零元学Expression Blend 4 – Chapter 20 以实作案例学习Childwindow 本章将教大家如何运用Blend 4内建的假视窗原件-「ChildWindow」 Chi ...
随机推荐
- 亚马逊的客户服务和承诺 - Delay in shipping your Amazon.com order - Missed Fulfillment Promise
We encountered a delay in shipping your order. We apologize for the inconvenience. Since your packag ...
- killall命令详解
基础命令学习目录首页 原文链接:https://blog.csdn.net/tanga842428/article/details/52474250 Linux系统中的killall命令用于杀死指定名 ...
- (转)Django 数据库
转:https://blog.csdn.net/ayhan_huang/article/details/77575186 目录 数据库说明 配置数据库 在屏幕输出orm操作对应的s ...
- JS进阶系列之闭包
刚刚总结完作用域链,我觉得很有必要马上对闭包总结一下,因为,之前也写过自己对闭包的理解,那时候只知道,闭包就是可以访问别的函数变量的函数,就是在函数里面的函数就叫做闭包,可是并没有深入探究,为什么,可 ...
- 读书笔记之java编程思想2
今天将第一章余下的部分读完了,余下部分讲解了java单继承的特点,单继承保证了所有的子类都有一个基类,这使得java所实现的垃圾回收器的实现变得简单了很多,单继承保证了所有的对象都具有一些功能,使得参 ...
- imooc-c++学习感悟
imooc--慕课网c++课程链接:[课程链接](http://www.imooc.com/course/list?c=C+puls+puls) Imooc 慕课网c++学习感悟 1.课程名称:c++ ...
- Android开发--第一个活动
一.创建工程 1 项目名:MyActivity 包名:com.iflytek.myactivity 2 为了便于学习,不勾选Create Activity.然后finish,工程创建完成 END ...
- 【第十周】psp
代码累计 300+575+475+353+620+703=2926 随笔字数 1700+3000+3785+4210+4333+3032=20727 知识点 机器学习,支持向量机 数据库技术 Acm刷 ...
- 使用w3m访问页面执行函数
Ubuntu系统中 在计划任务中使用 w3m命令访问地址 locahost/index.php,或许使用curl "locahost/index.php"来访问地址
- Construct BST from given preorder traversal
Given preorder traversal of a binary search tree, construct the BST. For example, if the given trave ...