uVa 714 (二分法)
Description
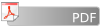
Before the invention of book-printing, it was very hard to make a copy of a book. All the contents had to be re-written by hand by so called scribers. The scriber had been given a book and after several months he finished its copy. One of the most famous scribers lived in the 15th century and his name was Xaverius Endricus Remius Ontius Xendrianus (Xerox). Anyway, the work was very annoying and boring. And the only way to speed it up was to hire more scribers.
Once upon a time, there was a theater ensemble that wanted to play famous Antique Tragedies. The scripts of these plays were divided into many books and actors needed more copies of them, of course. So they hired many scribers to make copies of these books. Imagine you have m books (numbered ) that may have different number of pages (
) and you want to make one copy of each of them. Your task is to divide these books among k scribes,
. Each book can be assigned to a single scriber only, and every scriber must get a continuous sequence of books. That means, there exists an increasing succession of numbers
such that i-th scriber gets a sequence of books with numbers between bi-1+1 and bi. The time needed to make a copy of all the books is determined by the scriber who was assigned the most work. Therefore, our goal is to minimize the maximum number of pages assigned to a single scriber. Your task is to find the optimal assignment.
Input
The input consists of N cases. The first line of the input contains only positive integer N. Then follow the cases. Each case consists of exactly two lines. At the first line, there are two integers m and k, . At the second line, there are integers
separated by spaces. All these values are positive and less than 10000000.
Output
For each case, print exactly one line. The line must contain the input succession divided into exactly k parts such that the maximum sum of a single part should be as small as possible. Use the slash character (`/') to separate the parts. There must be exactly one space character between any two successive numbers and between the number and the slash.
If there is more than one solution, print the one that minimizes the work assigned to the first scriber, then to the second scriber etc. But each scriber must be assigned at least one book.
Sample Input
2
9 3
100 200 300 400 500 600 700 800 900
5 4
100 100 100 100 100
Sample Output
100 200 300 400 500 / 600 700 / 800 900
100 / 100 / 100 / 100 100
Miguel A. Revilla
2000-02-15
程序分析:题目大意是要抄N本书,编号为1,2,3……N,每本书有1<=x<=10000000页,把这些书分配给K个抄写员,要求分配给某个抄写员的那些书的编号必须是连续的,每个抄写员的速度是相同的,求所有书抄完所用最少时间的分配方案。此题不但用到的二分法,同时在输出的时候也用到的贪心算法,还有一个值得注意的地方就是如果把X,Y,MID定义成int型会导致数据的溢出所以我们应该使用long long型。
程序代码:
#include <cstdio>
#include <cstdlib>
#include <iostream>
#include <cstring>
using namespace std;
const int MAXN = ;
int a[MAXN], use[MAXN];
long long low_bound, high_bound;
int n, m;
void init()
{
low_bound = -;
high_bound = ;
memset(use, , sizeof(use));
} int solve(int mid)
{
int sum = , group = ;
for(int i = n-; i >= ; i--)
{
if(sum + a[i] > mid)
{
sum = a[i];
group++;
if(group > m) return ;
}
else sum += a[i];
}
return ;
} void print(int high_bound)
{
int group = , sum = ;
for(int i = n-; i >= ; i--)
{
if(sum + a[i] > high_bound)
{
use[i] = ;
sum = a[i];
group++;
}
else sum += a[i];
if(m-group == i+)
{
for(int j = ; j <= i; j++)
use[j] = ;
break;
}
}
for(int z = ; z< n-; z++)
{
printf("%d ", a[z]);
if(use[z]) printf("/ ");
}
printf("%d\n", a[n-]);
} int main()
{
int T;
scanf("%d%*c", &T);
while(T--)
{
init();
scanf("%d%d", &n, &m);
for(int i = ; i < n; i++)
{
scanf("%d", &a[i]);
if(low_bound < a[i]) low_bound = a[i];
high_bound += a[i];
}
long long x = low_bound, y = high_bound;
while(x <= y)
{
long long mid = x+(y-x)/;
if(solve(mid)) y = mid-;
else x = mid+;
}
print(x);
}
return ;
}
uVa 714 (二分法)的更多相关文章
- uva 714 Copying Books(二分法求最大值最小化)
题目连接:714 - Copying Books 题目大意:将一个个数为n的序列分割成m份,要求这m份中的每份中值(该份中的元素和)最大值最小, 输出切割方式,有多种情况输出使得越前面越小的情况. 解 ...
- UVa 714 Copying Books(二分)
题目链接: 传送门 Copying Books Time Limit: 3000MS Memory Limit: 32768 KB Description Before the inventi ...
- 抄书 Copying Books UVa 714
Copying Books 题目链接:http://acm.hust.edu.cn/vjudge/contest/view.action?cid=85904#problem/B 题目: Descri ...
- uva 714 - Copying Books(贪心 最大值最小化 二分)
题目描写叙述开头一大堆屁话,我还细致看了半天..事实上就最后2句管用.意思就是给出n本书然后要分成k份,每份总页数的最大值要最小.问你分配方案,假设最小值同样情况下有多种分配方案,输出前面份数小的,就 ...
- UVa 714 Copying Books - 二分答案
求使最大值最小,可以想到二分答案. 然后再根据题目意思乱搞一下,按要求输出斜杠(这道题觉得就这一个地方难). Code /** * UVa * Problem#12627 * Accepted * T ...
- UVa 714 抄书(贪心+二分)
https://vjudge.net/problem/UVA-714 题意:把一个包含m个正整数的序列划分成k个非空的连续子序列,使得每个正整数恰好属于一个序列.设第i个序列的各数之和为S(i),你的 ...
- UVa 714 Copying books 贪心+二分 最大值最小化
题目大意: 要抄N本书,编号为1,2,3...N, 每本书有1<=x<=10000000页, 把这些书分配给K个抄写员,要求分配给某个抄写员的那些书的编号必须是连续的.每个抄写员的速度是相 ...
- 【NOIP提高组2015D2T1】uva 714 copying books【二分答案】——yhx
Before the invention of book-printing, it was very hard to make a copy of a book. All the contents h ...
- UVa 714 (二分) Copying Books
首先通过二分来确定这种最大值最小的问题. 假设每个区间的和的最大值为x,那么只要判断的时候只要贪心即可. 也就是如果和不超过x就一直往区间里放数,否则就开辟一个新的区间,这样来判断是否k个区间容得下这 ...
随机推荐
- apache域名重定向301跳转 .htaccess的写法
RewriteEngine on RewriteBase / RewriteCond %{HTTP_HOST} ^baidu.com$ [NC] RewriteRule ^(.*)$ http://w ...
- C# Convert an enum to other type of enum
Sometimes, if we want to do convert between two enums, firstly, we may think about one way: var myGe ...
- ASP.Net MVC3 - The easier to run Unit Tests by moq #Reprinted#
From: http://www.cnblogs.com/techborther/archive/2012/01/10/2317998.html 前几天调查完了unity.现在给我的任务是让我调查Mo ...
- 怪兽z主机豪华版 答问。
我的淘宝店里,怪兽z主机标准版,分经济版本,标准版,豪华版,三个版本.这里给大家详细介绍一下豪华版的概况. 淘宝购买地址:http://item.taobao.com/item.htm?id=3818 ...
- 转: object 和embed 标签播放flash
一.介绍: 我们要在网页中正常显示flash内容,那么页面中必须要有指定flash路径的标 签.也就是OBJECT和 EMBED标签.OBJECT标签是用于windows平台的IE浏览器的,而EMBE ...
- Correlation rule tuning
Lots of organizations are deploying SIEM systems either to do their due diligence or because it’s pa ...
- Protel99SE制作拼板的方法
制作步骤: 1.在PCB编辑里按快捷键 S/A全选复制源PCB全部内容,再按Ctrl+C看到十字光标.点击左键. 2.打开目标PCB文件,点击Edit菜单,在下拉菜单中点击Paste special( ...
- Windows Azure HDInsight 现已正式发布!
今天,我们宣布正式发布 Windows Azure HDInsight 服务.HDInsight 是 Microsoft 提供的基于 Hadoop 的服务,为云提供 100% 的 Apache Had ...
- Ext JS学习第十五天 Ext基础之 Ext.DomQuery
此文同来记录学习笔记 •Ext.dom.Query 嗯,这个类一看就是到时做什么事儿的,不用我去过多的解释了.这个类一共提供了8个方法供开发人员去使用. •要说最常用的方法,无非就是Ext.query ...
- js动画学习(四)
七.多属性封装函数 前面分别介绍了单独改变单一属性值得动画,从本节起开始介绍多属性封装函数,一个函数搞定多种属性值的改变. 首先介绍一个很重要的函数getStyle(),这个函数返回一个元素的当前属性 ...